PowerShell URL encoding is the process of converting special characters in a string into a format that can be transmitted over the internet, ensuring that the string remains intact during the transfer.
Here’s a simple code snippet to URL encode a string in PowerShell:
[System.Web.HttpUtility]::UrlEncode("Hello, World!")
What is URL Encoding?
URL encoding, also known as percent encoding, is a mechanism used to encode information in a Uniform Resource Identifier (URI) format. This is crucial as URLs can only be sent over the Internet using the ASCII character set. Certain characters, including spaces, punctuation, and non-ASCII characters, must be represented in a format that can be safely transmitted.
The process replaces unsafe ASCII characters with a "%" followed by two hexadecimal digits representing the character's ASCII code. For instance, a space is represented as `%20`. Understanding URL encoding is fundamental when building web applications or any automation involving web requests.
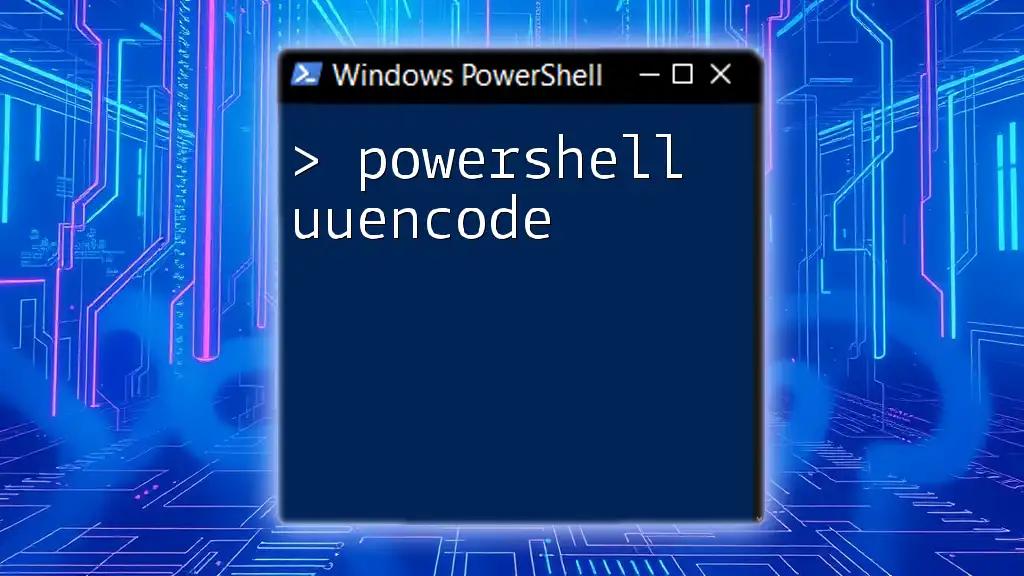
Why Use PowerShell for URL Encoding?
Using PowerShell for URL encoding provides a powerful scripting tool to automate the process efficiently. PowerShell can handle bulk URL encoding tasks, making it invaluable in scenarios such as:
- Automating the generation of URLs with parameters.
- Modifying existing URLs for compliance with web standards.
- Sending data via HTTP requests where precise encoding is essential for conveying the right format.

The Concept of URL Encoding in PowerShell
PowerShell natively supports URL encoding through various classes. The two most common classes utilized for encoding are `[System.Net.WebUtility]` and `[System.Uri]`. Each class has unique properties and methods for encoding URLs, making PowerShell a versatile tool for web developers and system administrators alike.
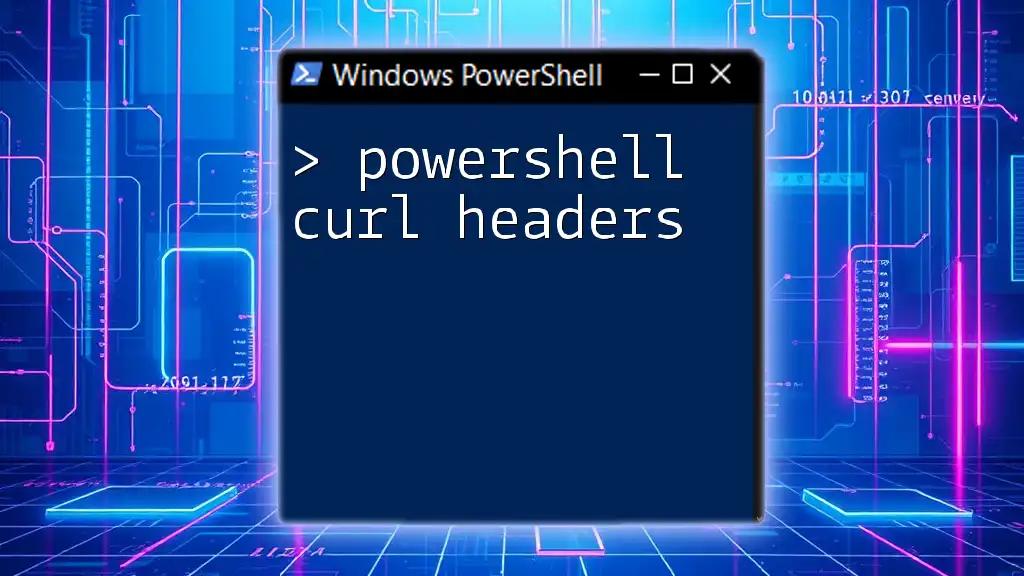
Common Use Cases for PowerShell URL Encoding
When working with web applications, there are various scenarios in which URL encoding is essential. Common use cases include:
- Sending HTTP GET requests with query parameters.
- Creating email links or hyperlinks that might contain special characters or spaces.
- Storing URLs in databases where encoding ensures compatibility.
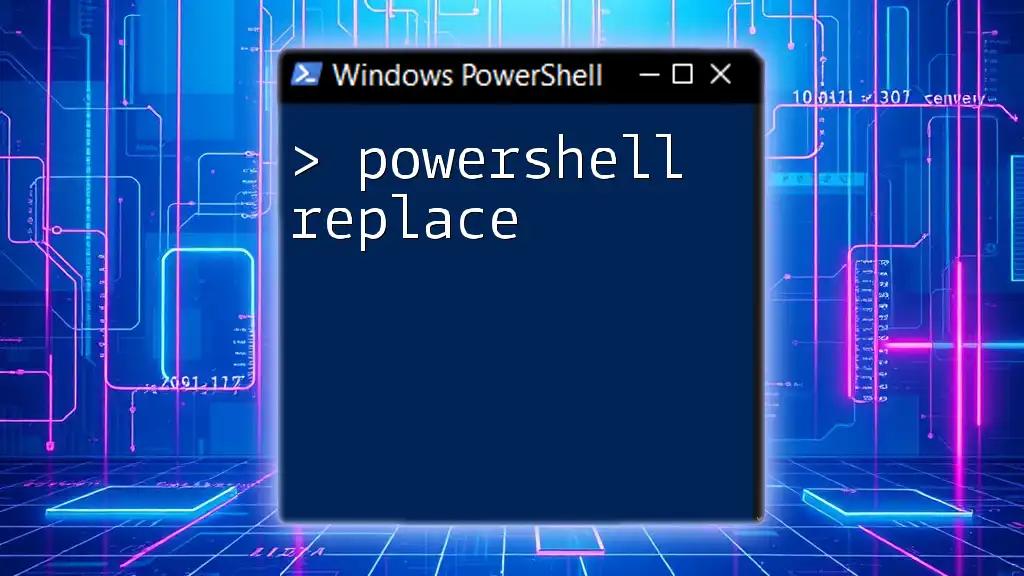
Using the [System.Net.WebUtility] Class
The `[System.Net.WebUtility]` class provides methods to encode and decode URLs efficiently. This class is often the first choice for developers due to its straightforward implementation.
Code Example using WebUtility
To URL encode a simple query string, use the `UrlEncode` method as follows:
$encodedUrl = [System.Net.WebUtility]::UrlEncode("https://example.com/search?q=hello world")
Write-Output $encodedUrl
In this example, the `UrlEncode` method converts the URL "https://example.com/search?q=hello world" into a proper encoded format. The output will be:
https%3A%2F%2Fexample.com%2Fsearch%3Fq%3Dhello+world
Explanation of the Code
- The method takes a string and outputs a new string encoded for safe transmission.
- Spaces are converted into `+`, and special characters like `:` become their hexadecimal percent-encoded counterparts.
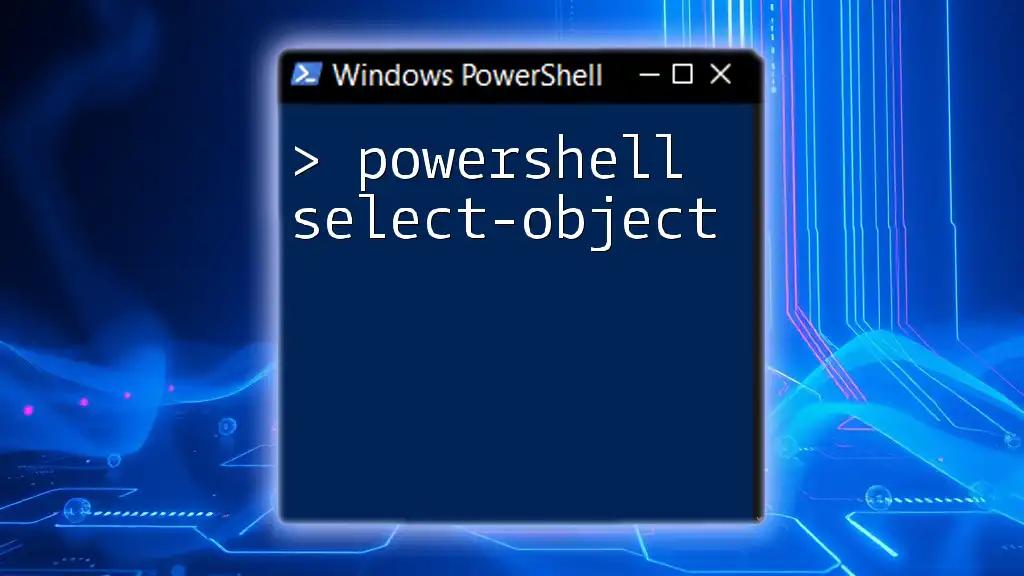
Using the [System.Uri] Class
Another powerful way to encode URLs in PowerShell is to utilize the `[System.Uri]` class. It provides a robust interface for creating URIs, which can be useful in an array of scenarios.
Code Example using Uri
Here’s a simple example of how to use the `[System.Uri]` class:
$uri = New-Object System.Uri("https://example.com/search?q=hello world")
$encodedUrl = $uri.AbsoluteUri
Write-Output $encodedUrl
Explanation of the Code
In this script, a new `Uri` object is created, and by accessing the `AbsoluteUri` property, the URL is automatically encoded. This method ensures that all parts of the URI conform to the necessary encoding rules.
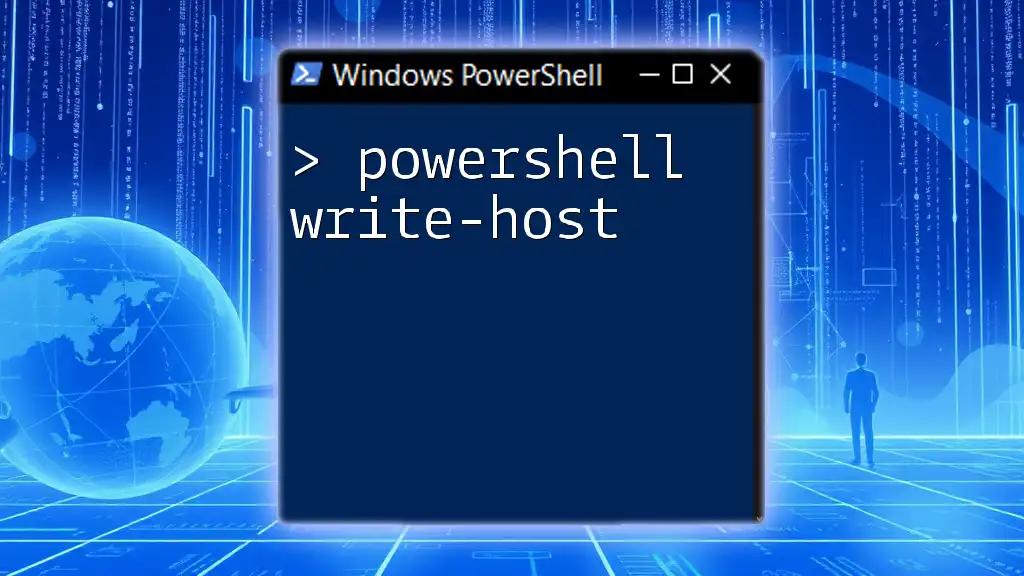
Understanding PowerShell URL Decoding
While encoding is essential, it's equally important to know how to decode URLs. URL decoding reverses the encoding process by converting encoded characters back to their original form.
Importance of URL Decoding
Decoding is crucial when receiving data from a URL where parameters have been encoded. It ensures that the data is in a readable format for further processing.
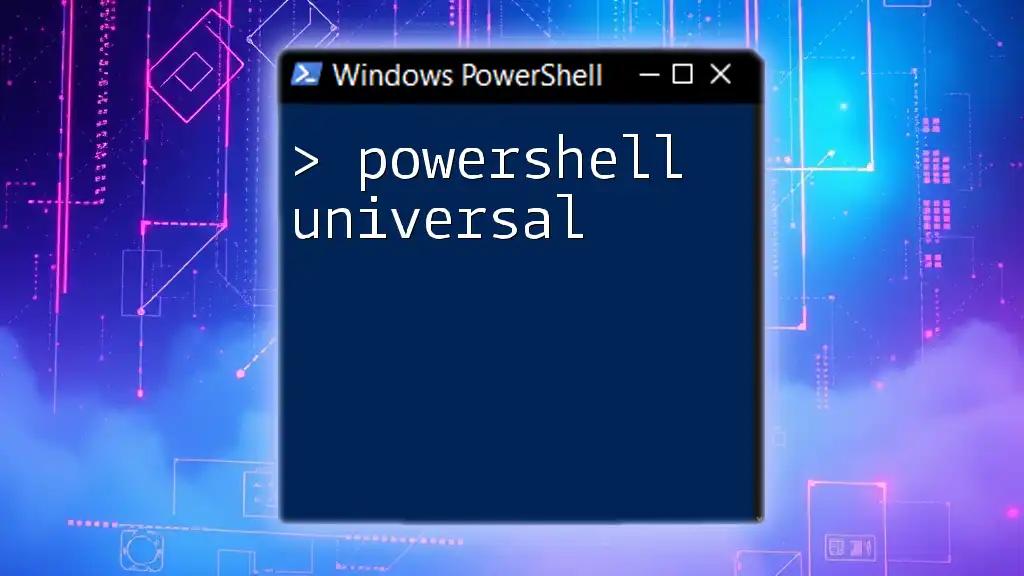
How to Perform URL Decoding in PowerShell
You can easily decode URLs in PowerShell by using the `UrlDecode` method from the `[System.Net.WebUtility]` class.
Code Example for URL Decoding
$decodedUrl = [System.Net.WebUtility]::UrlDecode("https%3A%2F%2Fexample.com%2Fsearch%3Fq%3Dhello%20world")
Write-Output $decodedUrl
Explanation of the Code
The `UrlDecode` method correctly translates the encoded URL back into a human-readable string, successfully converting `%3A` back to `:`, `%2F` to `/`, and so on.
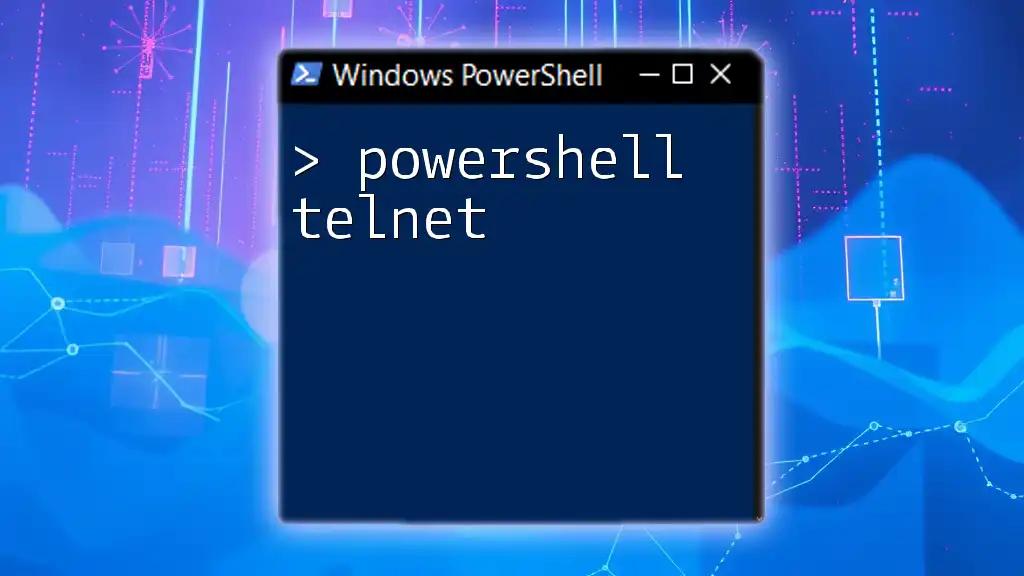
Advanced PowerShell URL Encoding Techniques
Handling Special Characters and Non-ASCII Characters
It's important to note that URL encoding does not simply stop at spaces or common special characters. Non-ASCII characters (such as emojis or special letters) also require encoding. PowerShell handles these seamlessly through the methods mentioned, ensuring that your URLs are safe for transmission.
Creating a Custom Function for URL Encoding
You may encounter situations where you wish to build a more versatile solution for URL encoding. This can be accomplished by creating a custom function in PowerShell.
Code Example for a Custom Encoding Function
function Encode-Url {
param([string]$url)
return [System.Net.WebUtility]::UrlEncode($url)
}
Encode-Url "https://example.com/?query=hello world"
Explanation of the Function
This custom function, `Encode-Url`, takes a URL as input and returns the encoded version using the `UrlEncode` method. This encapsulation allows for reusable code across scripts – wherever URL encoding is necessary.
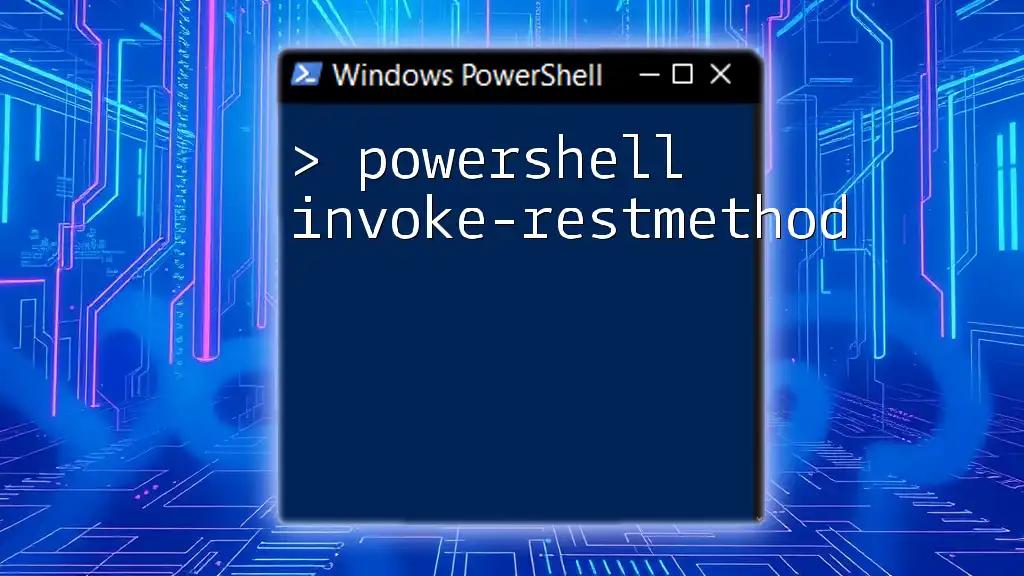
Common Pitfalls in PowerShell URL Encoding
Common Errors and Solutions
When performing URL encoding, it’s easy to overlook certain characters, especially when dealing with complex URLs. Typical mistakes include:
- Forgetting to encode spaces: They should be converted to `%20` or `+`.
- Assuming that all characters are safe: Always check if your URL contains characters that need encoding.
Performance Considerations
When encoding multiple URLs or operating under computational constraints, performance can be an issue. Use batch processing for bulk URL manipulations and ensure that unnecessary operations are avoided to maintain speed and efficiency in your PowerShell scripts.

Recap of PowerShell URL Encoding and Decoding
In summary, mastering PowerShell URL encode functionality is paramount for anyone involved in web development or automation tasks. Understanding how to encode and decode URLs effectively can save time and prevent errors in web applications, especially when dealing with dynamic parameters.
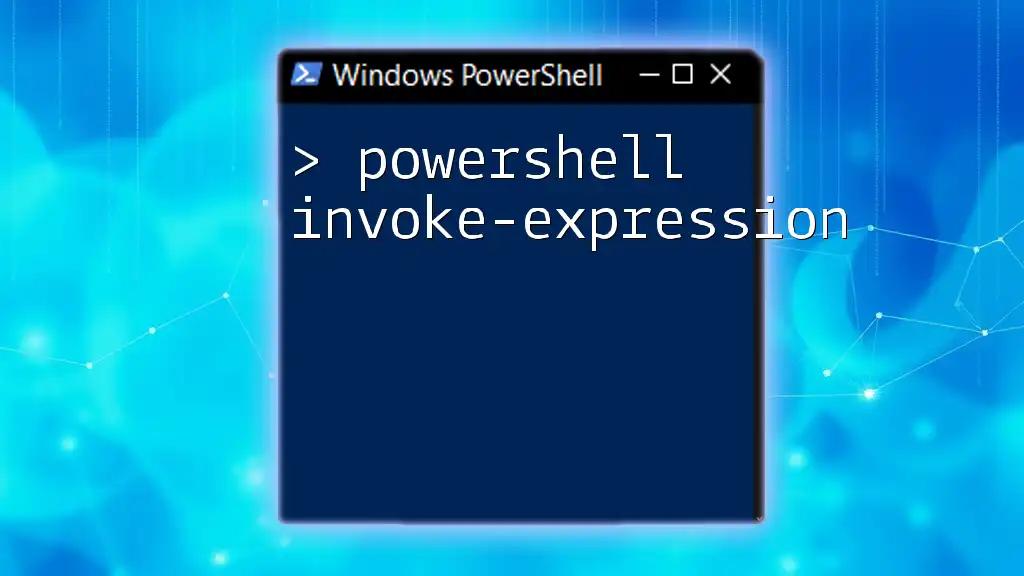
Where to Learn More About PowerShell
If you are eager to dive deeper into PowerShell, numerous resources are available. Engage with community forums, online courses, and documentation to enhance your knowledge. Leverage books and tutorials that focus on scripting best practices, and aim to keep your skills sharpened in this vital area.
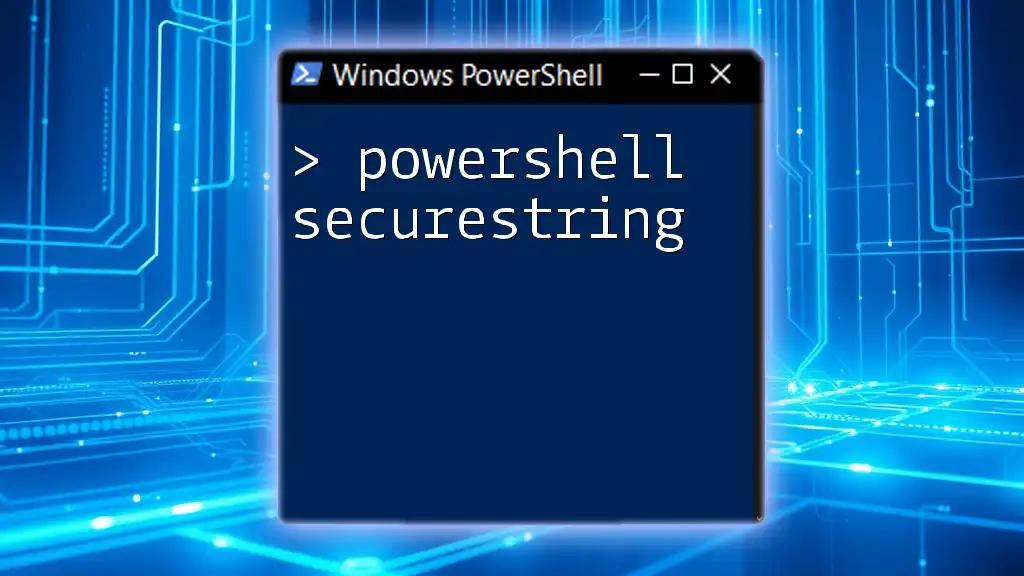
PowerShell Documentation and Community Support
Always refer to the official PowerShell documentation for authoritative guidance on classes and functions. Engaging with community forums can also provide insights and solutions to common problems.
By integrating these practices into your workflow, you’ll master PowerShell URL encoding and decoding, ultimately simplifying your automation and web integration tasks.