The `Round` function in PowerShell is used to round a numeric value to the nearest integer or to a specified number of decimal places.
# Rounding a number to the nearest integer
$number = 4.6
$roundedNumber = [math]::Round($number)
Write-Host "Rounded number: $roundedNumber" # Output: Rounded number: 5
Understanding Basic Rounding in PowerShell
What is Rounding?
Rounding is a mathematical process that involves reducing the number of digits in a number while retaining its value to a certain degree of accuracy. It's essential in various scenarios, such as financial calculations, data analysis, and reporting, where precision is key but excessive detail can be unnecessary.
Why Use Rounding in PowerShell?
In PowerShell, using the `powershell round` command enables users to streamline numerical outputs, making them more user-friendly and interpretable. Whether working with large datasets or performing calculations within scripts, rounding helps improve readability and accuracy in results.
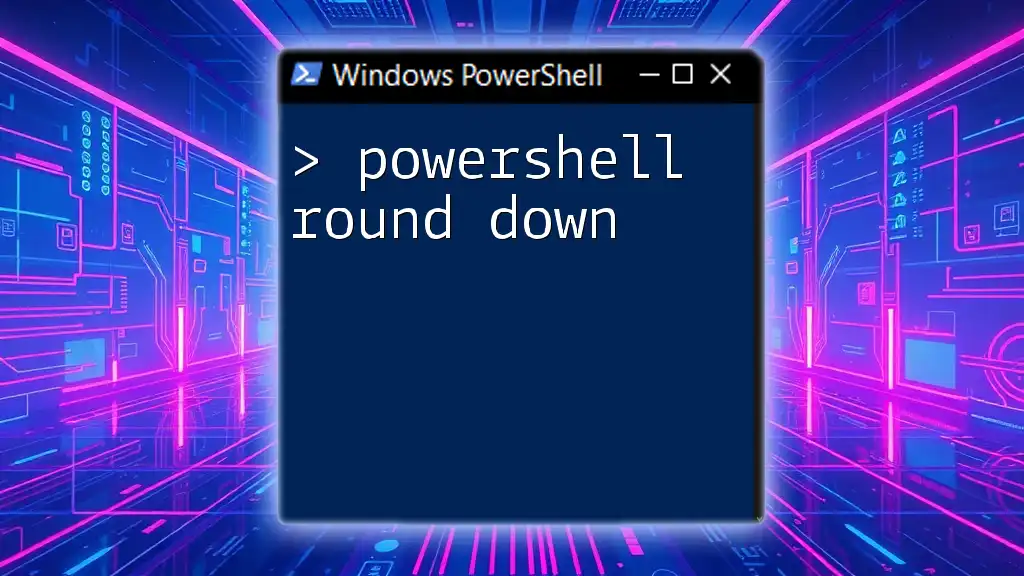
The `Round` Command in PowerShell
Introduction to the `Round` Command
The `Round` command in PowerShell is accessible through the `[math]` class, specifically through the `[math]::Round()` method. It is designed to round a number to the nearest whole number or to a specified number of decimal places.
How to Use the `Round` Command
Basic examples demonstrate how simple it is to round numbers in PowerShell. The syntax is straightforward, and the command can be used in various contexts.
$number = 5.7
$roundedNumber = [math]::Round($number)
Write-Output $roundedNumber # Output: 6
In this example, `5.7` rounds to `6`, showing the default behavior of rounding up.
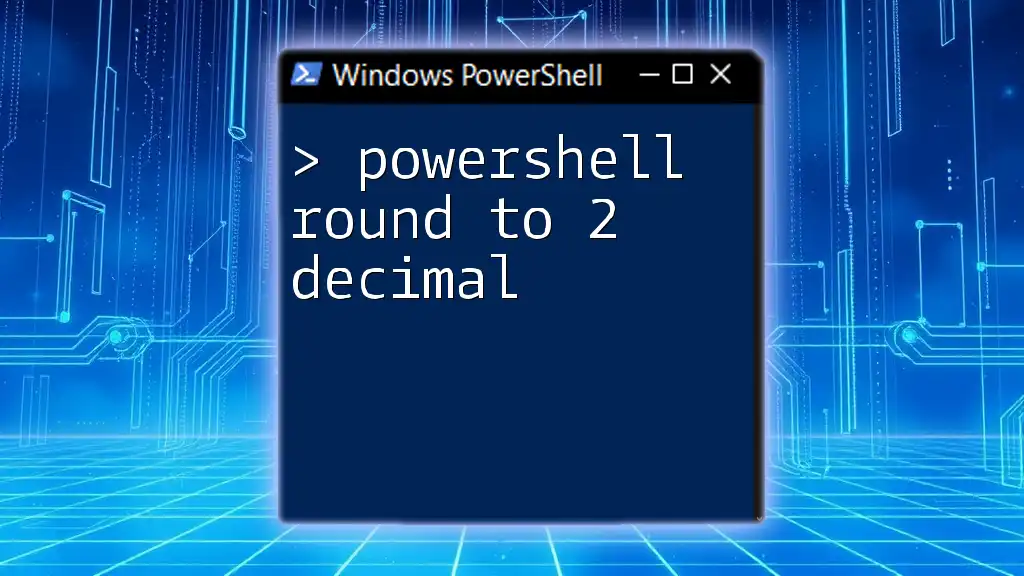
Rounding Methods in PowerShell
Rounding Up with `Round`
When discussing rounding, one vital aspect is distinguishing between rounding up and rounding down. PowerShell has built-in commands to accomplish both.
To round a number up—regardless of its decimal—use the `[math]::Ceiling()` method.
$number = 4.3
$roundedUp = [math]::Ceiling($number)
Write-Output $roundedUp # Output: 5
In this instance, `4.3` rounds up to `5`.
Rounding to Whole Numbers
To round a number to its nearest whole integer, you can use the `[math]::Round()` method directly. This command default to rounding values above .5 up and below .5 down.
$number = 2.9
$roundedToWhole = [math]::Round($number)
Write-Output $roundedToWhole # Output: 3
Here, `2.9` rounds up to `3`, showcasing how the command effectively handles numbers that are just below the mid-point.
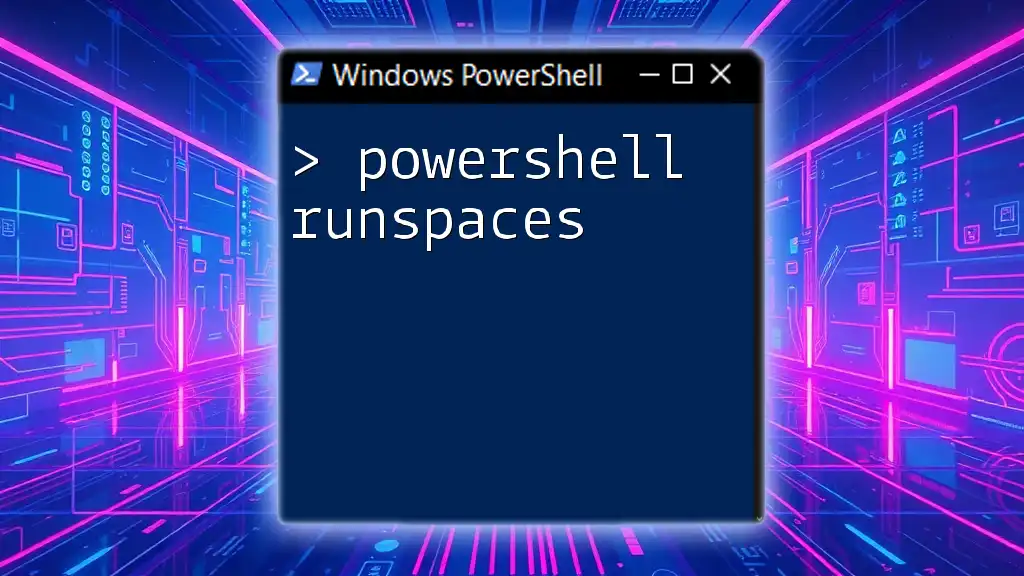
Advanced Rounding Techniques
Rounding to Specified Decimals
For more control over the rounding process, you can specify the number of decimal places. This allows for precise formatting in calculations where decimal points are essential.
$number = 1.2345
$roundedToTwoDecimals = [math]::Round($number, 2)
Write-Output $roundedToTwoDecimals # Output: 1.23
In this example, `1.2345` rounds to `1.23`, demonstrating how easy it is to manage numerical precision in PowerShell.
Usage of Different Rounding Modes
PowerShell supports various rounding behaviors, known as rounding modes. For instance, using `[midpointRounding]` allows more control over how PowerShell handles numbers that are precisely halfway between two potential values.
$numberHalfUp = 1.5
$roundedHalfUp = [math]::Round($numberHalfUp, 0, [midpointRounding]::ToPositiveInfinity)
Write-Output $roundedHalfUp # Output: 2
This command rounds `1.5` up to `2`, highlighting how the `ToPositiveInfinity` mode operates.
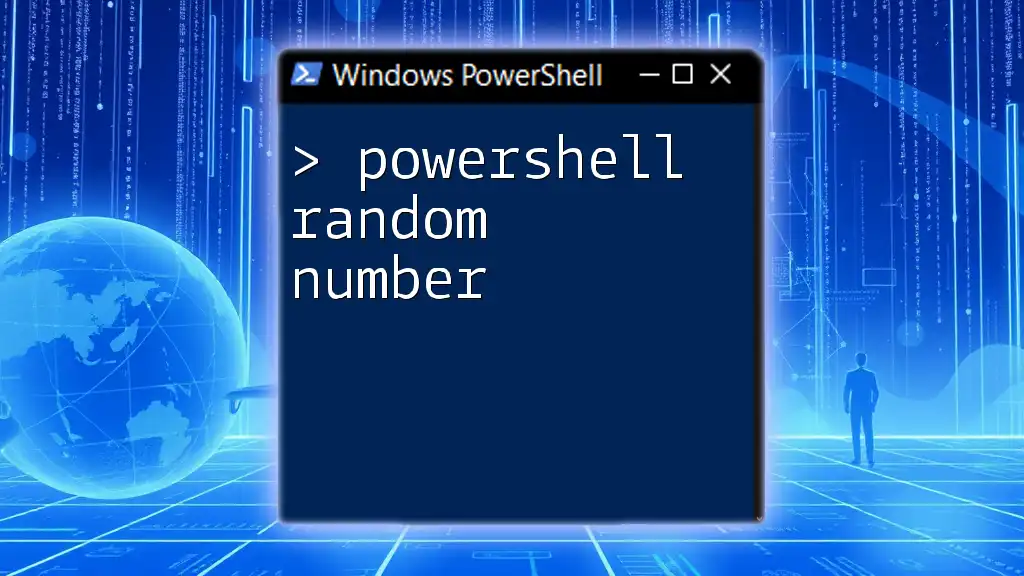
Common Rounding Pitfalls
Understanding Floating-Point Precision Issues
One common issue when working with rounding in PowerShell—or any programming language, for that matter—is floating-point precision. When performing arithmetic with floating points, results can sometimes be unexpectedly imprecise.
$num = 0.1 + 0.2
Write-Output $num # Output: 0.30000000000000004
This subtle discrepancy highlights why careful rounding is crucial when precision matters greatly in calculations.
Handling Negative Numbers
Rounding also behaves differently when negative numbers are involved, which is essential to understand to avoid misinterpretation of results.
$negativeNumber = -1.5
$roundedNegative = [math]::Round($negativeNumber)
Write-Output $roundedNegative # Output: -2
In this scenario, `-1.5` rounds down to `-2`, demonstrating how rounding operates with negative values.
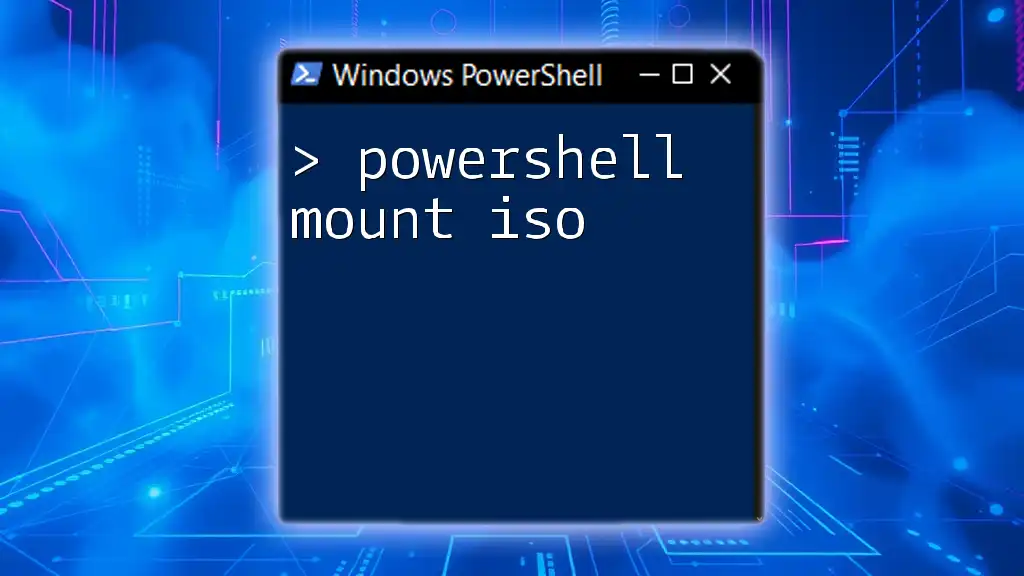
Practical Applications of Rounding in PowerShell
Use Cases for Rounding in Scripting and Automation
Rounding is particularly valuable in data manipulation, where converting precise values to comprehensible figures can enhance reporting and data representation. For example, round monetary numbers for financial reports or averages for statistical data, making information clearer and more actionable.
Integrating Rounding in Larger PowerShell Scripts
Imagine generating a report that summarizes sales data including total revenue. Using the `powershell round` command, one can ensure the final values are cleanly presented.
$totalRevenue = 1350.537
$roundedRevenue = [math]::Round($totalRevenue, 2)
Write-Output "Total Revenue: $roundedRevenue in USD" # Output: Total Revenue: 1350.54 in USD
This practical application of rounding ensures that users see economic values formatted to two decimal places, adhering to financial reporting conventions.
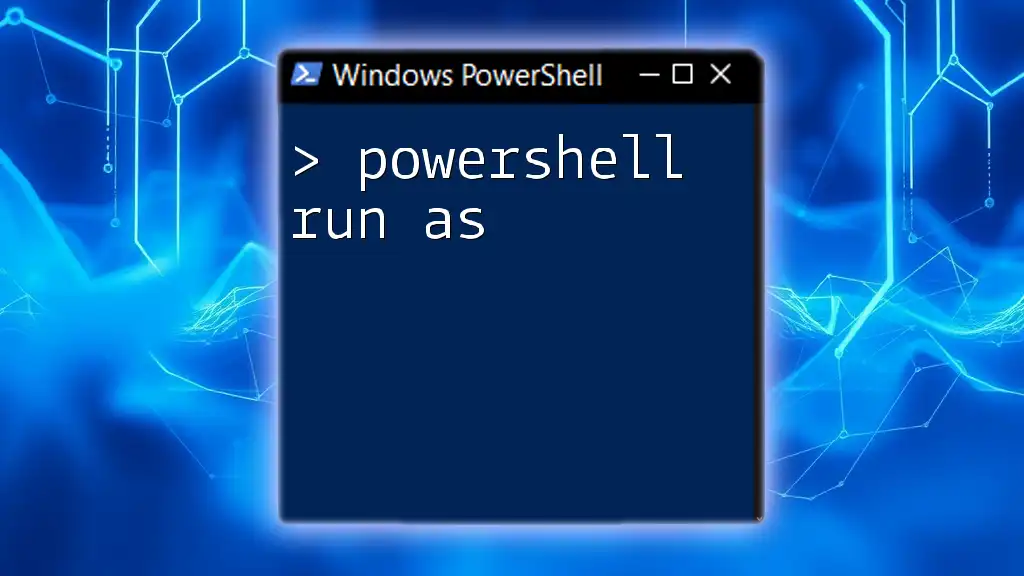
Conclusion
Rounding numbers in PowerShell through the `powershell round` command provides essential tools for clarity and precision in numerical reporting and calculations. Understanding how to properly utilize rounding commands allows for improved data presentation and script capabilities. Practice with the examples and consider how rounding can streamline your PowerShell scripts for better efficiency and readability.
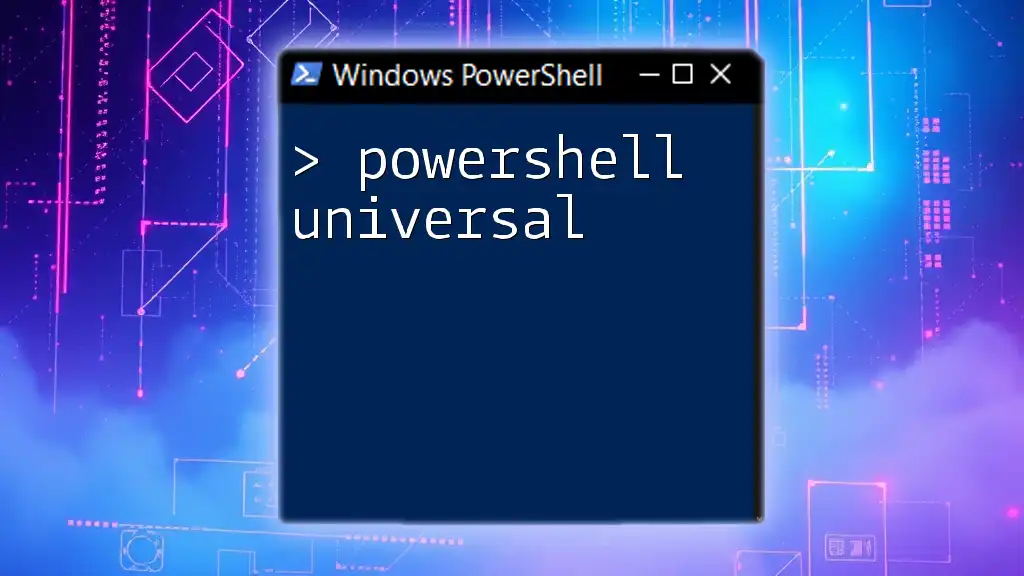
Additional Resources
For further study, consult the official PowerShell documentation and delve deeper into mathematical functions. Knowing how to effectively apply these principles can greatly enhance your coding capabilities and understanding of PowerShell’s versatile functionalities.