In PowerShell, you can generate a random number using the `Get-Random` cmdlet, which allows you to customize the range of numbers if desired.
Get-Random -Minimum 1 -Maximum 100
Understanding Random Number Generation in PowerShell
What is Random Number Generation?
Random number generation is a crucial programming technique used to create numbers that do not follow a predictable pattern. In the world of computing, true randomness is challenging to achieve, as most algorithms create pseudo-random numbers—numbers that are generated based on initial values or "seeds." In PowerShell and other scripting languages, random numbers are often employed in various scenarios, such as testing, simulations, and game development.
Generating random numbers can help in creating diverse and unpredictable outcomes, making your scripts more dynamic and versatile.
Why Use PowerShell for Random Number Generation?
PowerShell is a powerful scripting tool that is not only straightforward to use but is also deeply integrated with the Windows operating system and other Microsoft services. Using PowerShell for random number generation offers several advantages:
- Quick scripting capabilities: You can run commands quickly without the overhead of compiled languages.
- Access to powerful cmdlets: PowerShell includes built-in cmdlets that simplify the process of generating random numbers.
- Integration: Random number generation can be seamlessly integrated with other PowerShell commands, enhancing automation and productivity in scripting tasks.
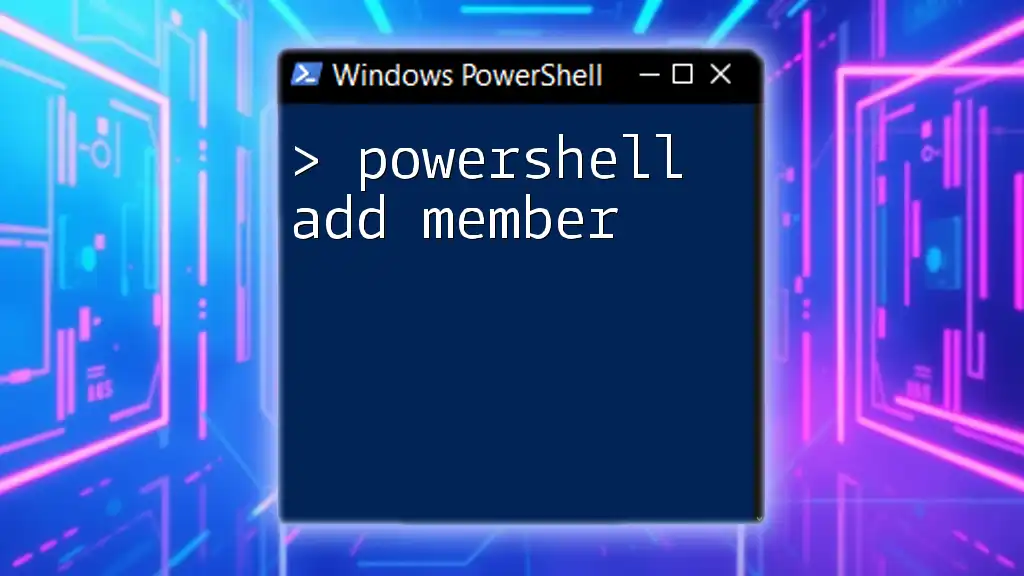
PowerShell Commands for Generating Random Numbers
The Get-Random Cmdlet
The Get-Random cmdlet is the primary means of generating random numbers in PowerShell. This cmdlet is incredibly versatile and easy to use.
To generate a simple random number, you can simply input:
Get-Random
When you run this command, you’ll receive an integer that is generated within the default range of 0 to 2147483647 (the maximum value for a 32-bit integer). The numbers are unpredictable and can serve various purposes depending on your needs.
Specifying Number Range
If you want to generate random numbers within a specific range, the Minimum and Maximum parameters permit you to set boundaries for your random numbers.
For example, if you want to generate a random integer between 1 and 100, you would write:
Get-Random -Minimum 1 -Maximum 100
In this case, the output will only display numbers between 1 and 99 (the maximum boundary is exclusive). This feature is beneficial for tasks that require randomization within specific limits.
Generating Multiple Random Numbers
You can also generate multiple random numbers in a single command. This is particularly useful if you need an array of random values for tasks such as simulating multiple outcomes in a game or generating a list of unique identifiers.
Use the -Count parameter to specify how many random numbers you want to generate:
Get-Random -Minimum 1 -Maximum 100 -Count 5
This command will output five random integers, each ranging between 1 and 99. It exemplifies how concise and efficient PowerShell can be for generating bulk random data.
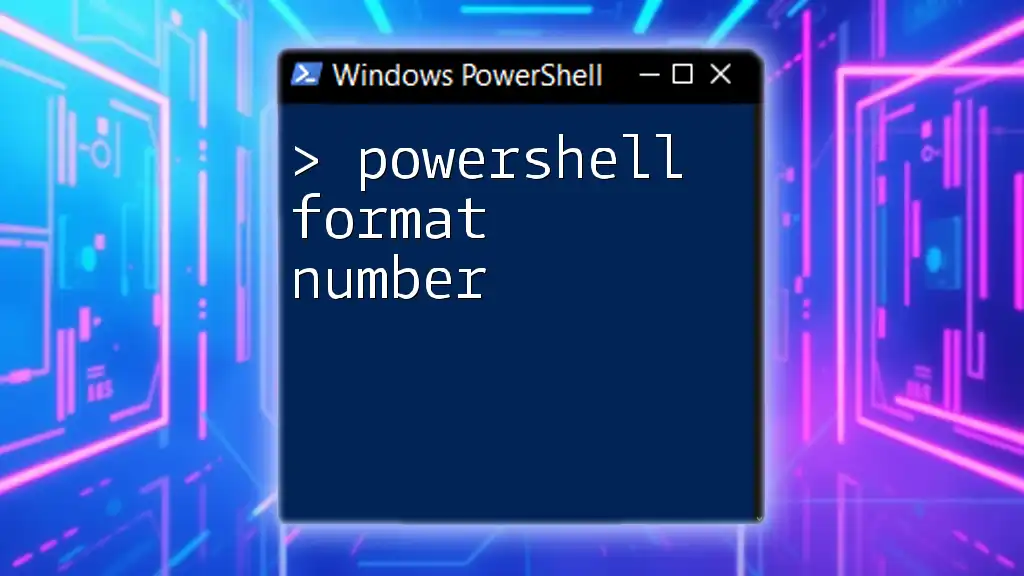
Advanced Techniques for Random Number Generation
Using Random Seed
The concept of a random seed is an essential part of random number generation. By initializing the random number generator with a specific value, you can produce the same sequence of random numbers every time you run your script. This can be particularly useful for testing purposes where you want consistent results.
Here’s how you can use a seed with the Get-Random cmdlet:
Get-Random -Minimum 1 -Maximum 100 -Seed 12345
With a specified seed, every time you run this command, you’ll get the same random number sequence. While this might seem contrary to the nature of randomness, it is a powerful tool for replicating scenarios in testing.
Generating Random Floating Point Numbers
In addition to integers, PowerShell can also generate random floating-point numbers. This extension broadens the scenarios where random numbers can be applicable—especially for simulations or calculations where precision is crucial.
Here's how you might generate a random floating-point number and round it to two decimal places:
$float = [math]::Round((Get-Random) * 100, 2)
In this example, we multiply the generated random number (which defaults to between 0 and 1) by 100 to scale it up. The use of `[math]::Round` allows us to specify the number of decimal places, making the output cleaner and more suited to applications requiring floating-point precision.
Custom Random Number Generator
To enhance reusability, you might want to create your own custom function for generating random numbers. Here’s an example of a simple PowerShell function that encapsulates the Get-Random cmdlet, allowing you to specify a minimum and maximum value:
function Generate-CustomRandom {
param (
[int]$min,
[int]$max
)
return Get-Random -Minimum $min -Maximum $max
}
By using this function, you can call `Generate-CustomRandom -min 5 -max 25` to get a random number in a user-defined range without rewriting the command each time. This approach promotes code reusability and keeps your scripts clean.
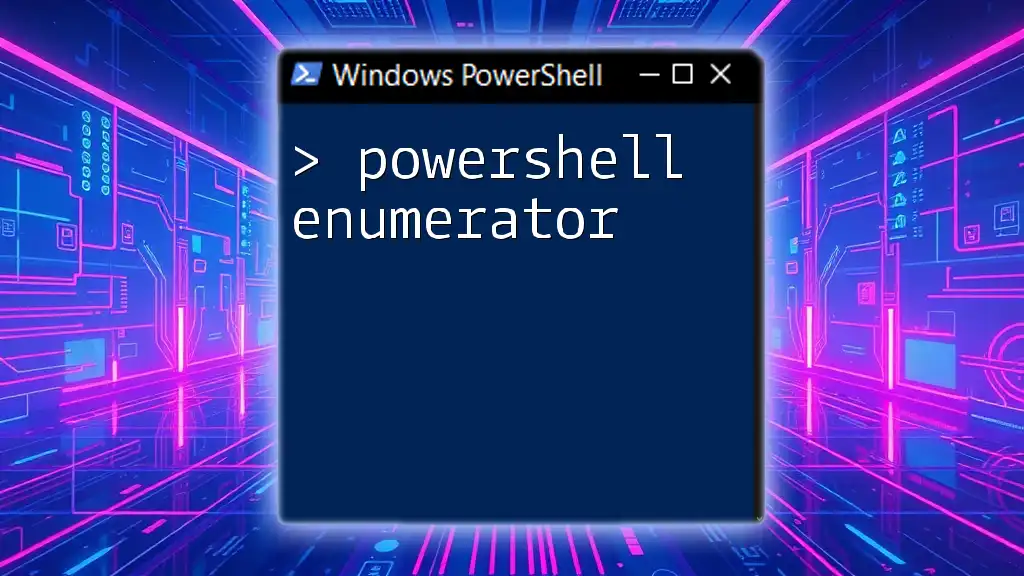
Practical Applications of Random Number Generation in PowerShell
Scripting for Game Development
In game development, random numbers often serve to create unpredictable elements, such as enemy movements, loot drops, or user interactions. For instance, you might simulate rolling dice in a text-based game using PowerShell:
$diceRoll = Get-Random -Minimum 1 -Maximum 7
Write-Host "You rolled a $diceRoll!"
This simple command can substantially enhance the player’s experience by introducing randomness and excitement into the game.
Testing and QA in Software Development
When developing software, generating random numbers can be invaluable for testing. You can create unique user IDs or test data sets that simulate real-world usage patterns:
$testUserID = Get-Random -Minimum 1000 -Maximum 9999
Write-Host "Generated User ID: $testUserID"
This approach ensures that your application withstands varied data scenarios, preparing it for real-world deployment.
Simulations and Modeling
Random numbers play a vital role in simulations, such as Monte Carlo methods where outcomes are modeled based on random sampling. For instance, if you are simulating lottery draws, having random numbers can create a fair and unpredictable experience:
$lotteryNumbers = Get-Random -Minimum 1 -Maximum 50 -Count 6
Write-Host "Your lottery numbers are: $($lotteryNumbers -join ', ')"
This command generates six distinct random numbers, ideal for simulating a lottery system where players pick numbers.
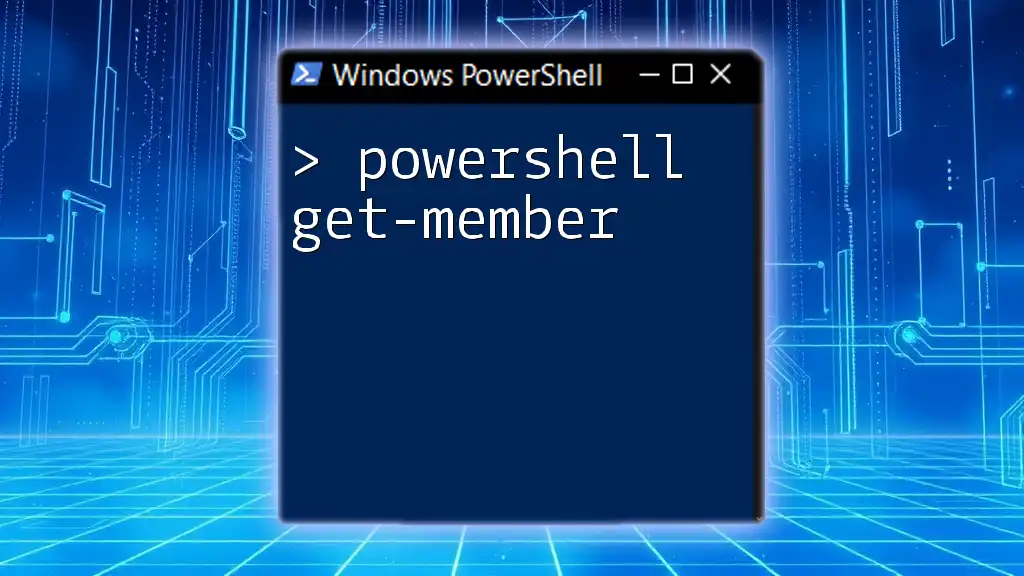
Conclusion
In summary, mastering the art of generating random numbers in PowerShell opens up a multitude of possibilities for automation and data manipulation. The Get-Random cmdlet is a versatile tool that can be employed in various ways—whether you’re generating simple integers, floating-point numbers, or creating custom functions to suit your needs. Randomization plays a crucial role in multiple domains, from game development to quality assurance in software testing.
With the knowledge you've gained from this article, you can confidently explore the randomness that PowerShell offers, enhancing your scripts and broadening your programming toolkit. For continuous improvement, consider learning more about PowerShell’s extensive capabilities through available resources and documentation.