PowerShell non-interactive mode allows scripts to run without requiring user input, making it ideal for automation and scheduled tasks.
Here's a simple example demonstrating non-interactive usage:
# This script runs without user interaction.
Write-Host 'Running in non-interactive mode...'
Start-Sleep -Seconds 5
Write-Host 'Done!'
What is PowerShell Non-Interactive Mode?
PowerShell non-interactive mode refers to the functionality of executing scripts and commands without direct user interaction, making it highly efficient for automation tasks. Unlike interactive mode, which requires user input, non-interactive mode allows for seamless execution of commands in the background or during scheduled tasks.
By utilizing non-interactive execution, system administrators can run scripts that perform maintenance, data processing, and other tasks without the need for active monitoring. This mode is particularly useful in the context of automation, where tasks often need to be performed repeatedly or in bulk without human intervention.
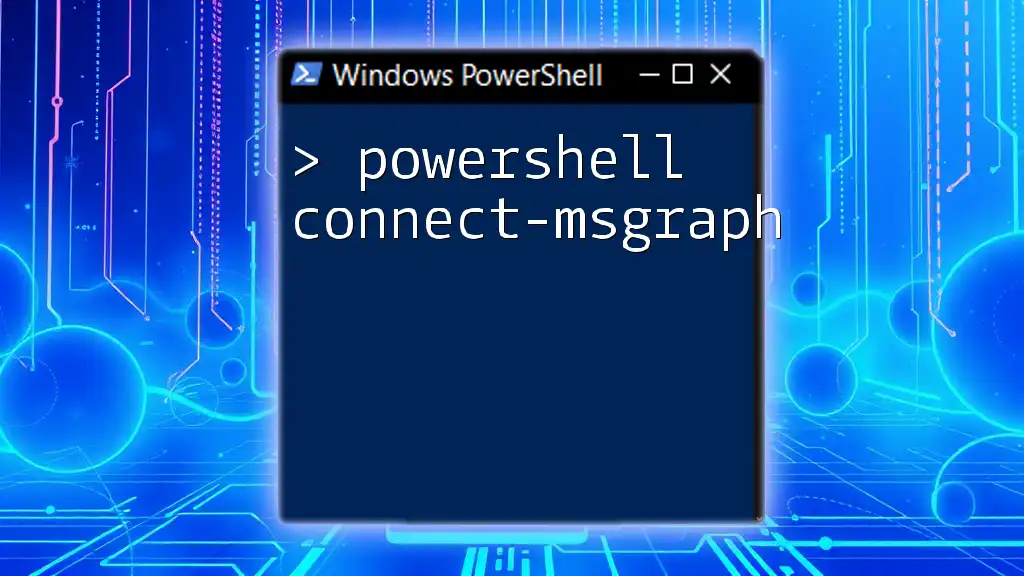
Why Use Non-Interactive Mode?
Benefits of non-interactive execution include increased efficiency and reduced potential for human error. Since scripts can run automatically, they can process data or handle system tasks unattended. Non-interactive scripts are ideal for:
- Scheduled tasks like backups or report generation.
- Running scripts during system maintenance windows.
- Automating processes that require repetitive actions.
In contrast to traditional interactive scripts, non-interactive scripts allow for batch processing and lend themselves well to integration into larger automation frameworks.
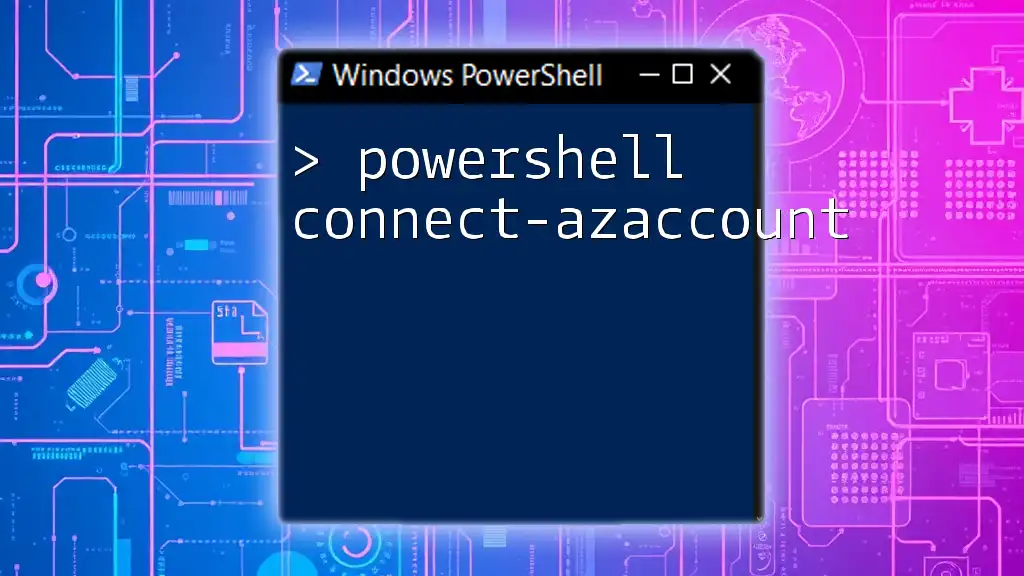
Setting Up PowerShell Non-Interactive Environment
Prerequisites
To effectively use PowerShell non-interactive mode, a few prerequisites should be in place:
- A fundamental understanding of PowerShell syntax and cmdlets.
- Ensure that PowerShell is properly installed and updated on your system.
- Familiarize yourself with execution policies, as they dictate the conditions under which scripts may be run.
How to Launch PowerShell in Non-Interactive Mode
PowerShell scripts can be executed non-interactively in various ways:
- Using Batch Files and Scripts: You can set up a batch file to execute a PowerShell script without requiring user input.
- Using Command-Line Arguments: Pass parameters directly in the command line when launching the script.
Here is an example of how to invoke a script non-interactively:
# Script Example: sample_script.ps1
param(
[string]$Message = "Hello, World!"
)
Write-Output $Message
To run this script non-interactively:
powershell -File "path\to\sample_script.ps1" -Message "Non-Interactive Mode"
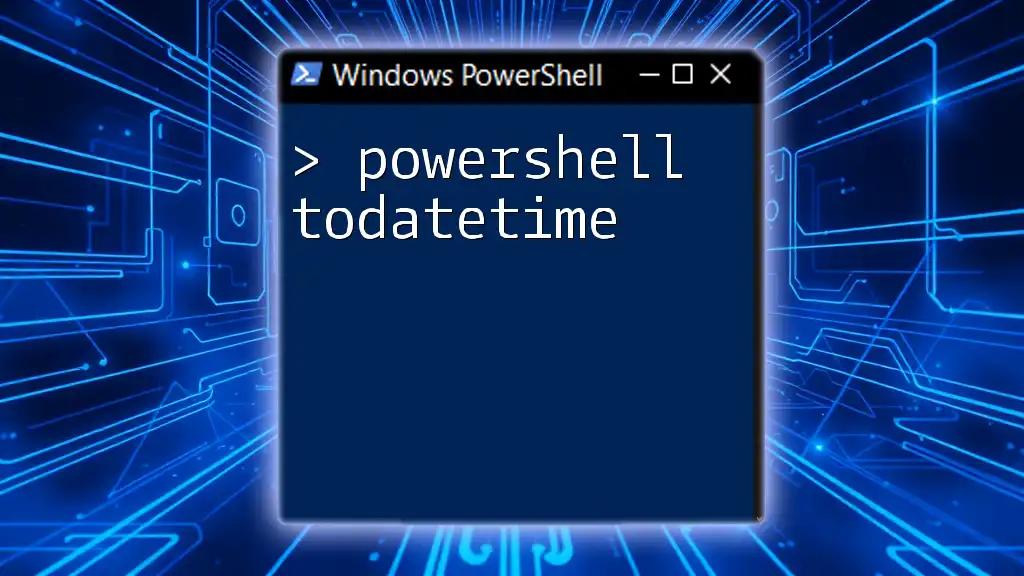
Common Commands and Techniques for Non-Interactive Scripting
Using Cmdlets in Scripts
When writing non-interactive scripts, it's crucial to utilize cmdlets that are designed for unattended operations. A few key points to consider include:
- Cmdlets like `Get-Process`, `Get-Service`, and file management cmdlets are often used in non-interactive scenarios.
- Always ensure proper output handling, either by saving results to files or by logging, to maintain clear records of what the script executed.
For example, here’s how to retrieve a list of running processes and redirect the output to a file:
Get-Process | Out-File "processList.txt"
Handling Output and Errors
Effective error handling is critical in non-interactive mode, as there will often be no opportunity for user intervention. To capture output and errors, consider the following:
Utilize output redirection techniques, and leverage the `try-catch` blocks for error management. For example, here's how to handle errors gracefully:
try {
Get-Item "C:\NonExistentFile.txt" -ErrorAction Stop
} catch {
$_ | Out-File "errorLog.txt"
}
This method captures and logs errors into an error file, enabling post-execution analysis.
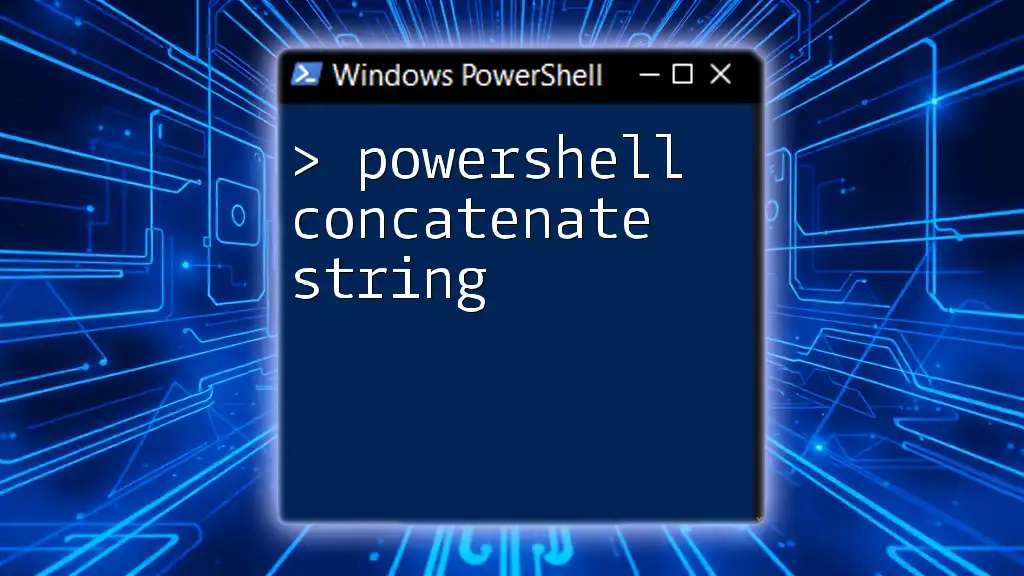
Advanced Concepts in PowerShell Non-Interactive Mode
Scheduled Tasks and Automation
PowerShell scripts can be scheduled to run at specific times using Windows Task Scheduler. This turns your scripts into automated solutions with minimal effort on your part. Setting up a scheduled task involves defining the action and the trigger. Here is a simple way to create a scheduled task that runs a PowerShell script daily:
$action = New-ScheduledTaskAction -Execute "powershell.exe" -Argument "-File C:\path\to\script.ps1"
$trigger = New-ScheduledTaskTrigger -Daily -At "08:00AM"
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName "DailyScript"
Using PowerShell Non-Interactive in Remote Environments
Remote execution is a powerful feature of PowerShell, enabling scripts to run on other machines without having to log in. To do this effectively, you must ensure remoting is enabled on the target machine. The following command demonstrates how to run a script on a remote machine:
Invoke-Command -ComputerName "RemotePC" -File "C:\path\to\nonInteractiveScript.ps1"
This command initiates the script specified on the remote computer, executing the script in non-interactive mode.
Security Considerations
While non-interactive execution offers numerous benefits, it also comes with security responsibilities. It is essential to follow best practices to mitigate the risks associated with unattended scripts:
- Ensure scripts do not expose sensitive information such as plain-text passwords.
- Utilize `SecureString` for handling passwords to prevent them from being stored in plain text.
Example for securing passwords:
$securePassword = ConvertTo-SecureString "YourPassword" -AsPlainText -Force
By implementing these security measures, you can safeguard your automated processes against potential vulnerabilities.
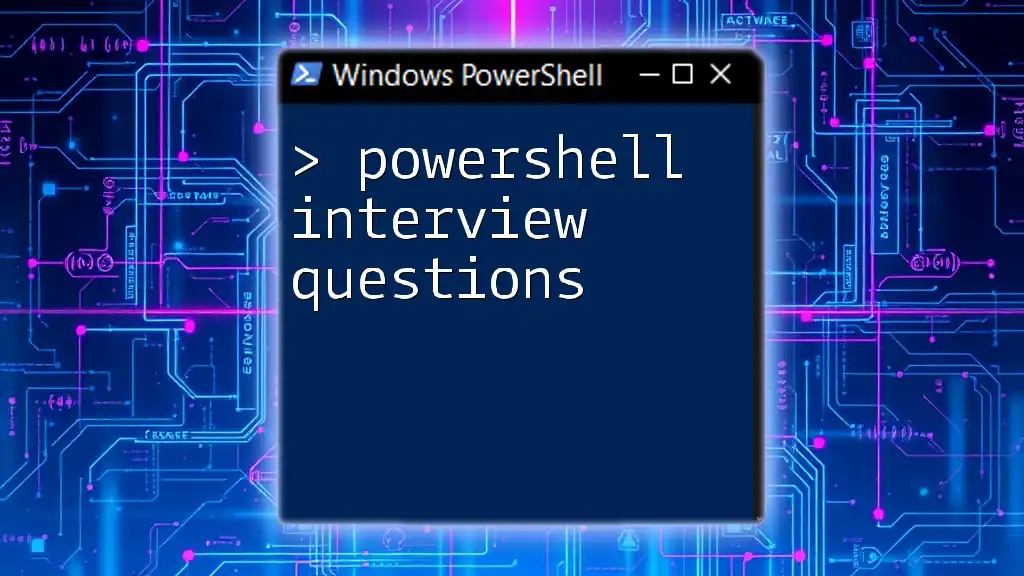
Troubleshooting Common Issues in Non-Interactive Mode
Common Errors and Fixes
Non-interactive scripts can sometimes encounter issues that may not arise in interactive mode. Common problems include permission issues, path errors, or missing cmdlets. To troubleshoot these, ensure:
- The executing user has the correct permissions to perform the actions defined in the script.
- File paths are absolute and correct.
- Dependencies and module requirements are addressed beforehand.
Debugging Non-Interactive PowerShell Scripts
Debugging non-interactive scripts requires the use of logging to track execution. This can be accomplished by using the `Start-Transcript` and `Stop-Transcript` cmdlets to capture all output from the session. Here’s how to implement basic logging in a script:
Start-Transcript -Path "C:\Logs\scriptLog.txt"
# Your PowerShell commands go here
Stop-Transcript
This approach provides a recorded log that can reveal what happened during script execution, helping to identify any issues that arose.
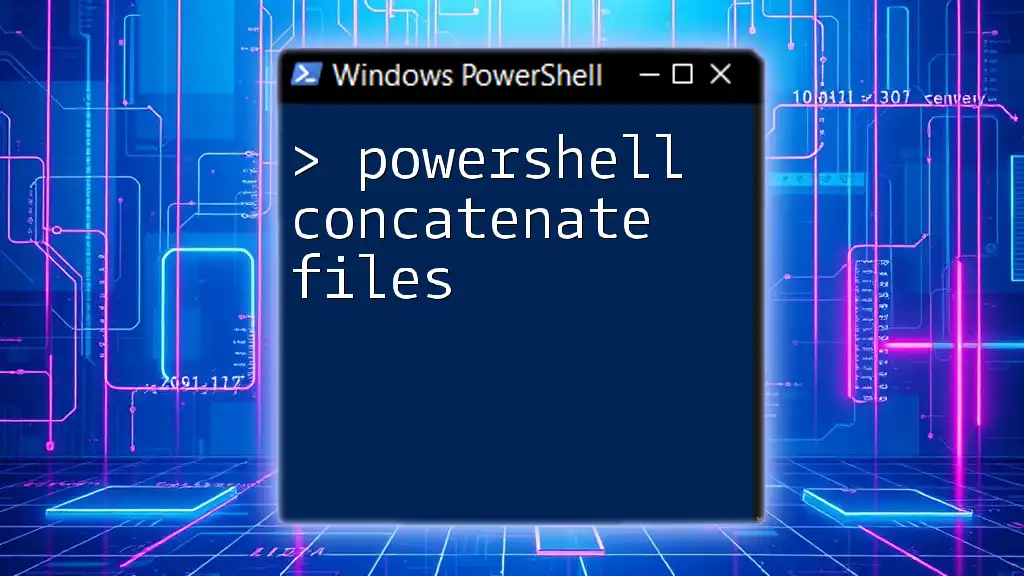
Conclusion
PowerShell non-interactive mode is a powerful feature that facilitates automation and efficiency in managing repetitive tasks, maintenance, and data processing. By understanding how to set up and utilize non-interactive scripts, you can significantly enhance your productivity and minimize the risk of human error.
By employing effective techniques for output handling, security practices, and scheduled execution, you can fully harness the potential of PowerShell non-interactive mode. Remember to continually explore and test your scripts, adapting them to match your evolving automation needs.
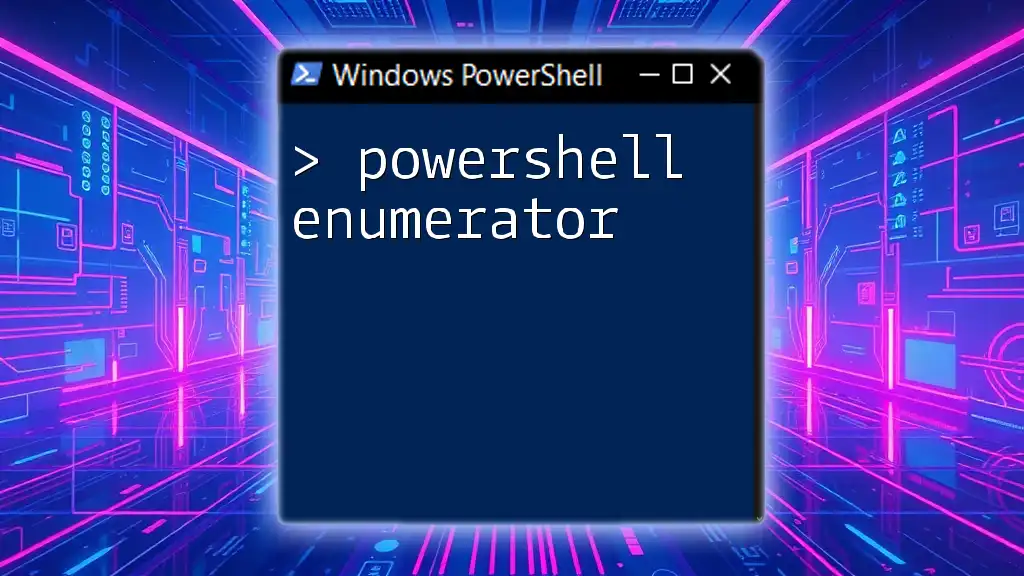
Call to Action
For more tutorials and tips on optimizing your PowerShell skills, subscribe to our updates and share your experiences with PowerShell non-interactive mode in the comments!