In PowerShell, a constant is a variable whose value cannot be changed after it has been assigned, ensuring data integrity throughout the script.
Here’s a simple code snippet to define a constant in PowerShell:
$constantName = "ConstantValue"
Set-Variable -Name constantName -Value $constantName -Option Constant
Understanding PowerShell Constants
Definition of PowerShell Constants
In programming, a constant is defined as a value that does not change during the execution of a program. In PowerShell, constants are similar—once you define a constant, its value remains fixed and cannot be modified. This characteristic makes constants particularly valuable, as they can help prevent accidental changes to critical values while ensuring code consistency.
Why Use PowerShell Constants?
Using PowerShell constants instead of variables provides several benefits. Unlike variables, which can be reassigned, constants maintain their integrity throughout the script’s execution. This immutability leads to improved code clarity and prevents unintended side effects that may arise when a value gets changed inadvertently. By using constants, you create self-documenting code, making it easier for other developers (and future you) to understand the intended values at a glance.
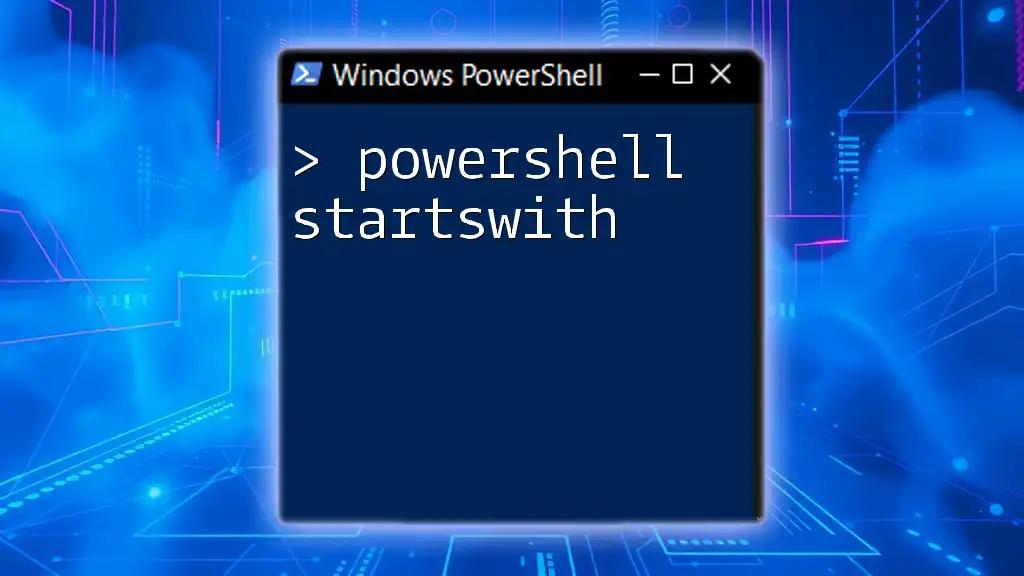
Creating Constants in PowerShell
The Syntax for Defining Constants
To define a constant in PowerShell, the `New-Variable` cmdlet is used with the `-Option` parameter set to `Constant`. Here is the basic syntax you would use:
New-Variable -Name 'YourConstantName' -Value 'YourValue' -Option Constant
Key Considerations When Defining Constants
When naming your constants, it’s essential to follow naming conventions to enhance readability. A common practice is to use uppercase letters and underscores to separate words, ensuring that constants stand out in your code.
Moreover, consider the scope of your constants—constants can be defined as global or local. A global constant can be accessed from anywhere in your script, while a local constant is confined to the scope where it is defined. This distinction can be important depending on how reusable you need your constants to be.
Example of Creating and Using a Constant
Let’s take a look at how to create a constant and use it within a script. The following example demonstrates creating a constant for the mathematical constant Pi:
New-Variable -Name 'PI' -Value 3.14159 -Option Constant
Write-Output "The value of PI is: $PI"
In this example, the value of `PI` remains static, and any future attempts to change it will result in an error.
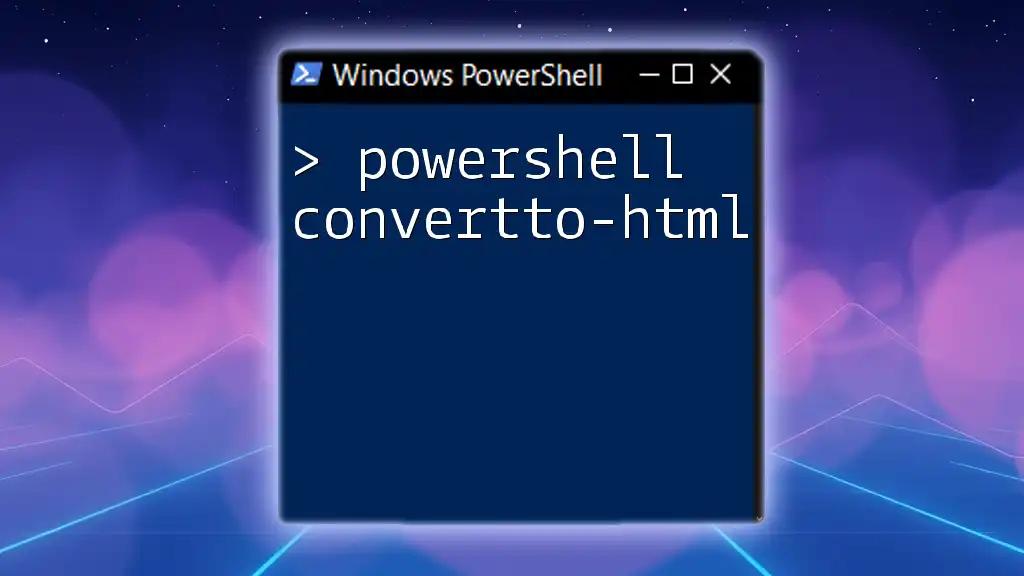
Practical Applications of PowerShell Constants
Using Constants for Configuration Settings
Constants are often employed to store configuration values that should not change during the execution of scripts. For instance, if you have a setting for maximum retry attempts in a web request, you can define it as a constant:
New-Variable -Name 'MaxRetryCount' -Value 5 -Option Constant
This constant can then be easily referenced throughout your script for various processes, ensuring consistency in your handling of retry logic.
Using Constants in Functions and Scripts
Another powerful application of constants is in functions. They can serve as predefined values that are reused across different parts of your script. Here’s an example of a function that utilizes a constant for determining the maximum retry count:
function Get-RetryCount {
return $MaxRetryCount
}
In this function, the constant `MaxRetryCount` ensures that any changes to retry logic need to happen in one place, preserving the integrity and clarity of the code.
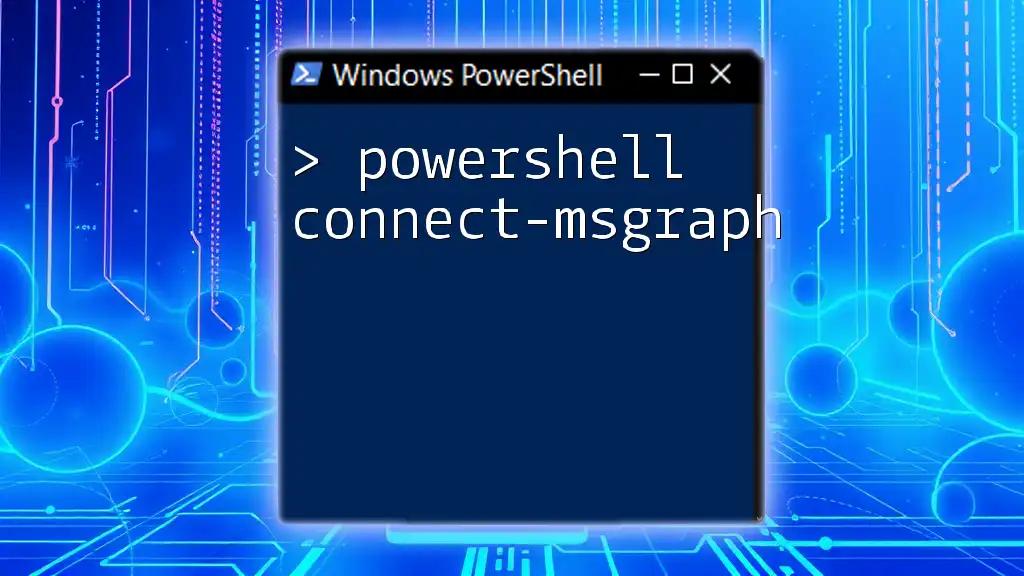
Limitations of PowerShell Constants
Understanding the Read-Only Aspect
One important aspect of constants in PowerShell is their read-only nature. Once a constant is defined, you cannot reassign it to a new value. While this is generally beneficial, it can also lead to limitations in certain scenarios. For example, if a constant needs to change based on user input or external configurations, you will have to consider alternatives, like using variables instead.
How to Handle Mistakes with Constants
If mistakes occur when working with constants, it’s critical to manage these errors proactively. Always test constants in a controlled environment before deploying them into production to avoid runtime errors. Clear documentation will also assist teams in understanding the purpose of each constant, reducing the likelihood of misuse.
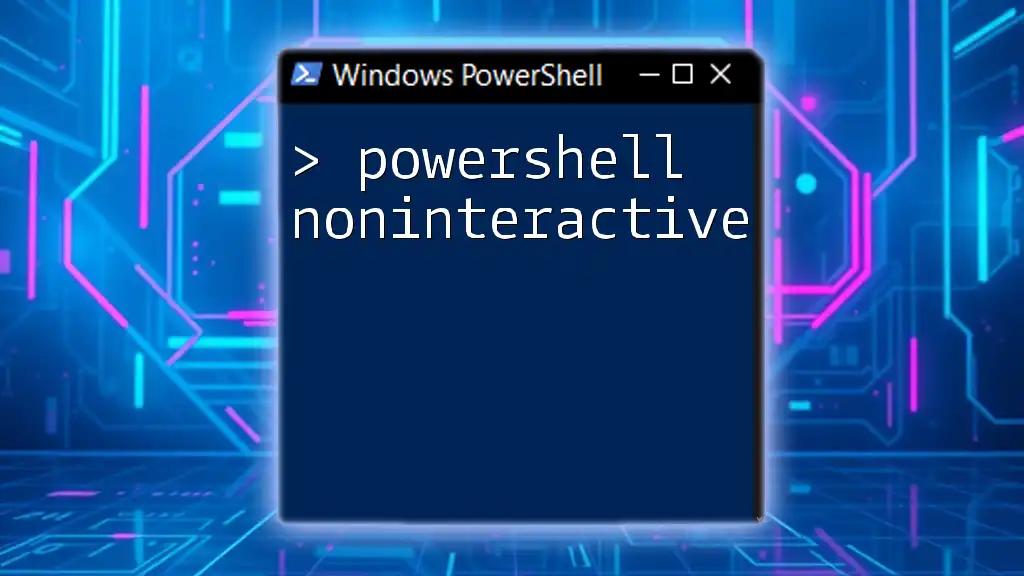
Best Practices for Using PowerShell Constants
Defining Meaningful Constant Names
It's vital to use clear and descriptive names when creating constants. This practice will significantly enhance code readability and prevent ambiguity. For example, use names like `DownloadsFolderPath` instead of generic names like `Path1`, which do not convey what the constant represents.
Organizing Constants in Your Scripts
Effective organization of constants within your scripts can lead to cleaner code. A common approach is to define all constants at the top of the script or, for larger projects, within a dedicated module. This structure allows for quick reference and management while keeping your scripts clean and easy to navigate.
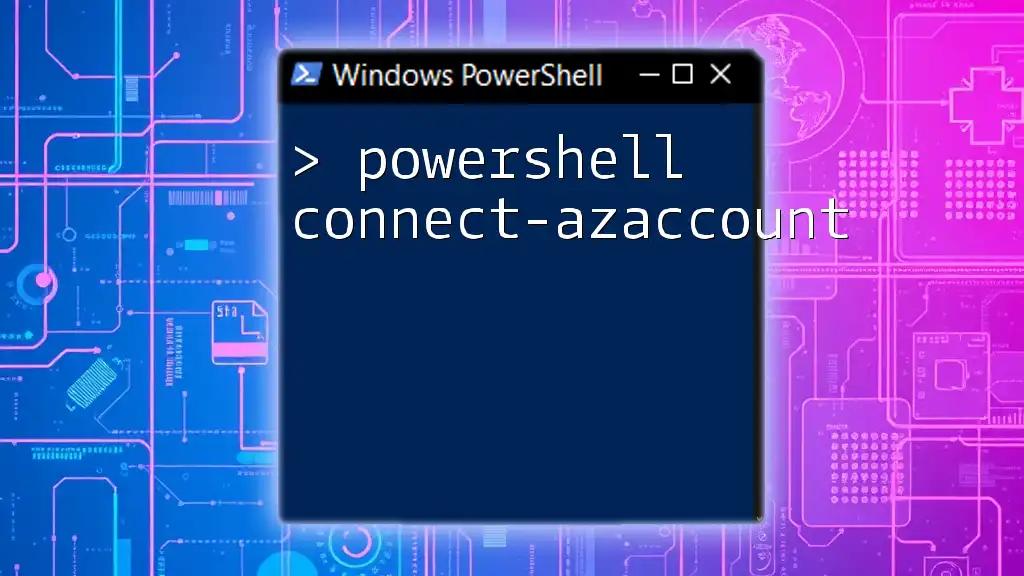
Conclusion
Utilizing PowerShell constants is an approach that promotes clarity, prevents accidental changes, and leads to better-maintained code. By implementing the practices outlined, you’ll harness the power of constants to create robust PowerShell scripts that stand the test of time.

Additional Resources
Documentation and References
For further reading, consult the official PowerShell documentation on constants. Additional resources can enhance your understanding and usage of PowerShell commands.
Community Contributions
If you have experiences or questions regarding PowerShell constants, consider joining community discussions. Engaging with others can provide insight and solutions that may enhance your learning journey.
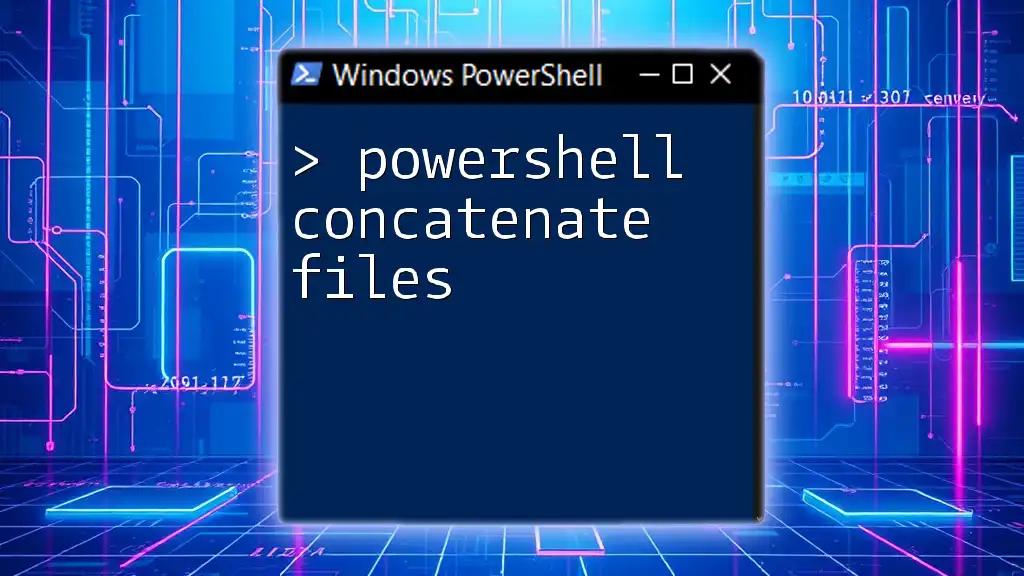
Call to Action
To continue expanding your knowledge of PowerShell commands, subscribe for more tips and tutorials. Additionally, a free guide on common PowerShell practices is available for our subscribers—don’t miss out!