The `TrimStart` method in PowerShell is used to remove specified leading characters from a string, streamlining data input and ensuring clean output.
$string = " Hello, World! "
$trimmedString = $string.TrimStart()
Write-Host $trimmedString # Outputs "Hello, World! "
Understanding TrimStart in PowerShell
What is TrimStart?
`TrimStart` is a method in PowerShell that allows you to remove leading whitespace characters or specific characters from the beginning of a string. This is particularly useful when you're dealing with strings that may have unnecessary spaces, which can distort data processing or output.
Use Cases for Trimming
When working with user inputs, data from external sources, or file manipulations, leading whitespace can be a common issue. By effectively using `TrimStart`, you ensure that you're dealing with clean data, which makes it easier to process and reduces the risk of errors.
Syntax of TrimStart
The syntax for using `TrimStart` is straightforward. The method belongs to the string object and can be called directly.
Basic usage:
$string = " Hello, World! "
$trimmedString = $string.TrimStart()
Write-Output $trimmedString # Output: "Hello, World! "
In this example, there are three spaces before "Hello". By applying `TrimStart`, those leading spaces are removed, resulting in a cleaner string.
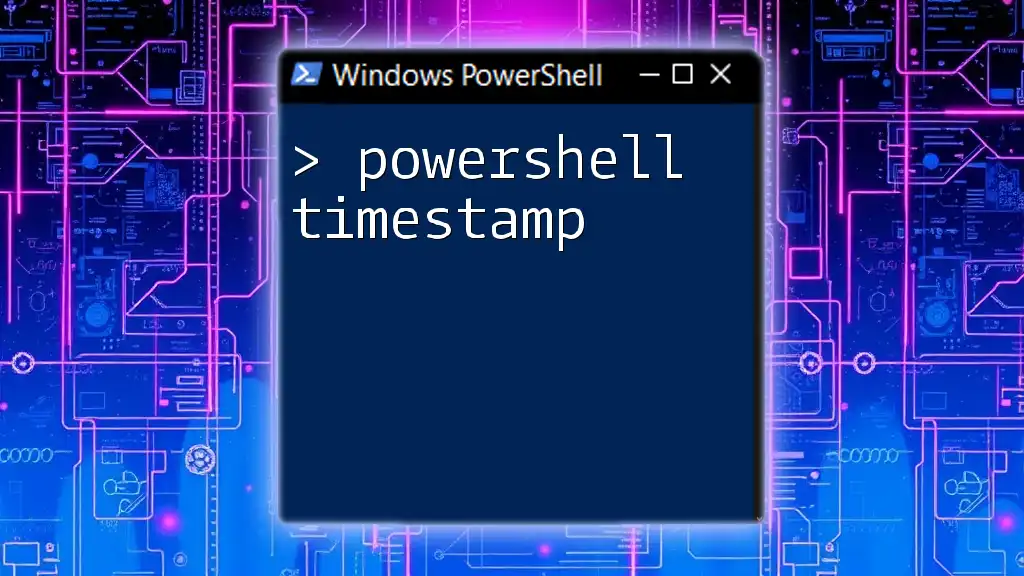
Practical Applications of TrimStart
Scenario 1: Cleaning User Input
User-generated input can often contain leading spaces, particularly if input is copied and pasted or if the user is not careful while typing. `TrimStart` helps in sanitizing this input.
Example Code Snippet:
$userInput = " Sample Input"
$cleanInput = $userInput.TrimStart()
Write-Output $cleanInput # Output: "Sample Input"
Here, the trailing text is sharply clear after using `TrimStart`. Such cleaning is essential when processing inputs for scripts or applications, where accuracy matters.
Scenario 2: Data Processing in CSV Files
Excel and other spreadsheet tools can produce CSV files that sometimes include unwanted spaces. When reading these files into PowerShell, using `TrimStart` can ensure that strings are processed correctly.
Example Code Snippet:
Import-Csv "data.csv" | ForEach-Object {
$_.ColumnName = $_.ColumnName.TrimStart()
# Further processing
}
In this scenario, we import a CSV and iterate over each record, applying `TrimStart` to a specific column. This method helps maintain data integrity for any further operations like filtering or exporting.
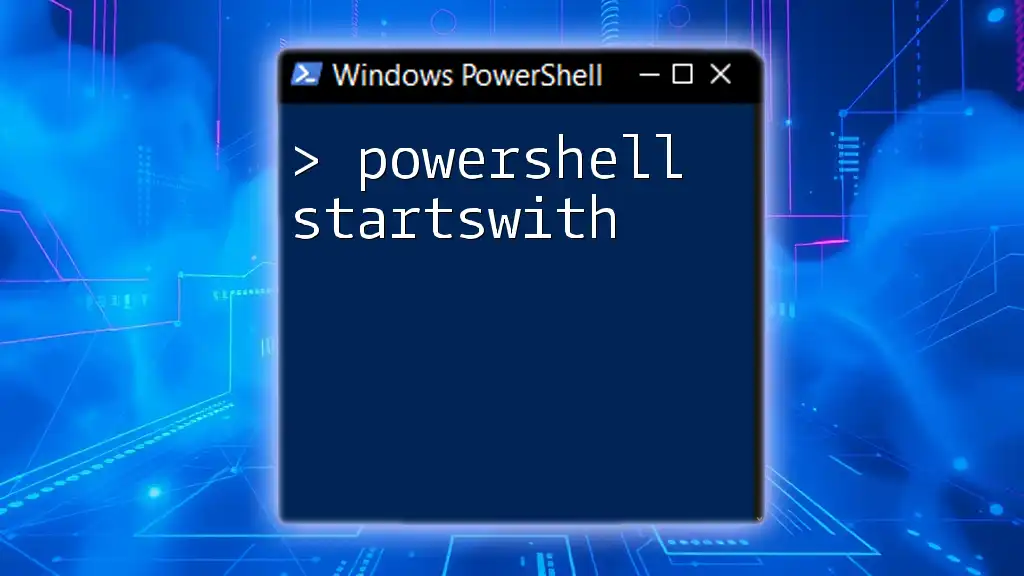
Comparing TrimStart and TrimEnd in PowerShell
What is TrimEnd?
Similar to `TrimStart`, the `TrimEnd` method removes trailing whitespace or specified characters from the end of a string. Understanding when to use these two methods is beneficial for precise string manipulation.
Usage Examples of TrimEnd
Using `TrimEnd` is also quite simple and effective.
Example Code Snippet:
$string = "Hello, World! "
$trimmedString = $string.TrimEnd()
Write-Output $trimmedString # Output: "Hello, World!"
In this example, the trailing spaces after "World!" are removed, leaving a cleaner output.
When to Use Which?
- Use `TrimStart` when you want to get rid of whitespace at the beginning of a string. This is generally more common when handling user inputs or data acquired from text processing that may mistakenly include spaces.
- Use `TrimEnd` when you need to clean up the end of your strings, such as when reading output from a file that may unintentionally include extra spaces.
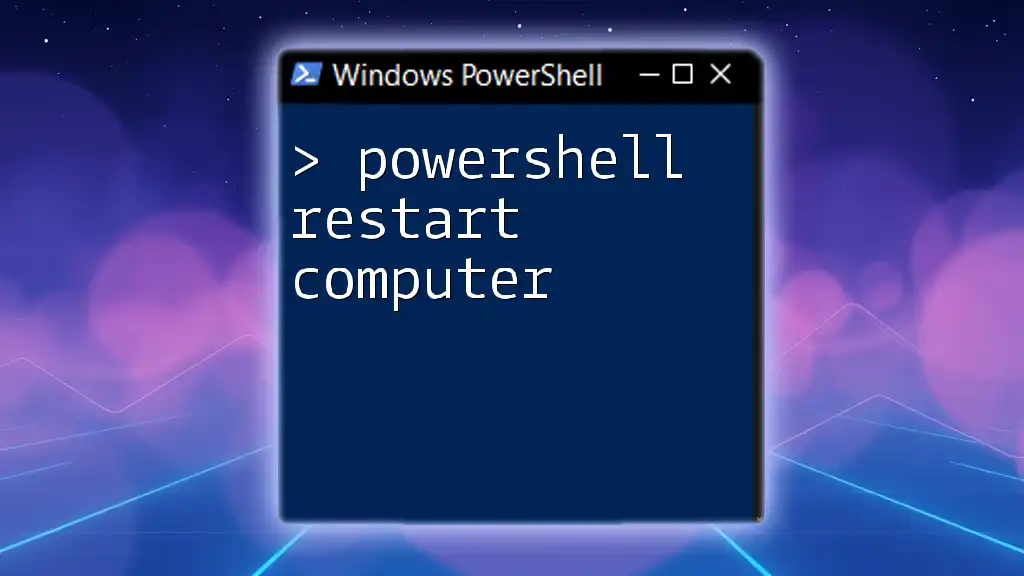
Combining TrimStart and TrimEnd for Comprehensive String Cleanup
Using Both Functions Together
Often, the most effective way to ensure your strings are clean is to apply both `TrimStart` and `TrimEnd`. This method allows you to remove both leading and trailing spaces simultaneously.
Example Code Snippet:
$string = " Hello, PowerShell! "
$cleanString = $string.TrimStart().TrimEnd()
Write-Output $cleanString # Output: "Hello, PowerShell!"
In this example, both the leading and trailing spaces are removed, giving you a clean, usable string that is free from confounding whitespace.
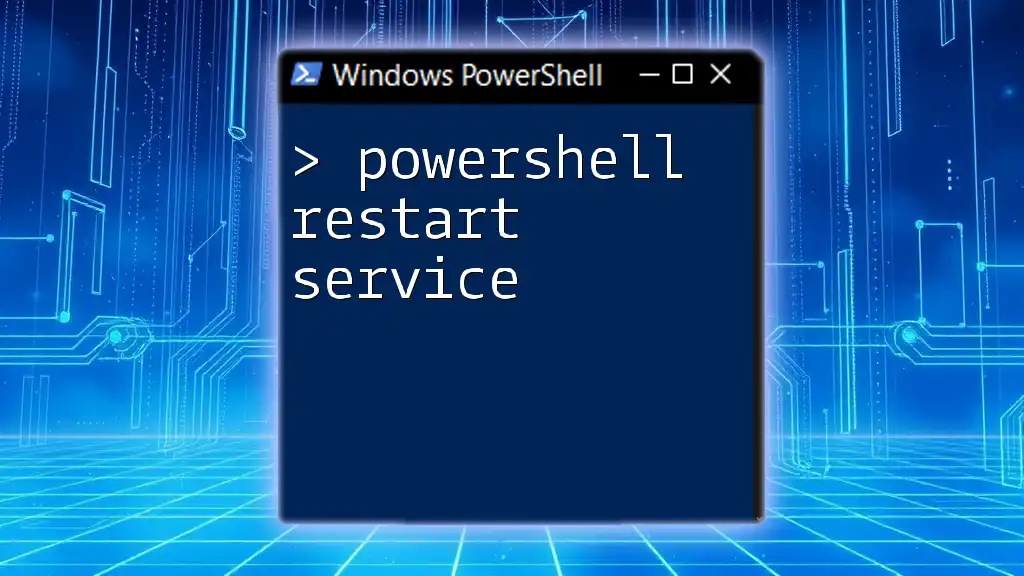
Common Pitfalls and Best Practices
Common Errors
When using `TrimStart`, users may forget that it only removes spaces at the beginning of the string. Expecting `TrimStart` to clean up trailing spaces will yield no changes. Therefore, it's essential to combine both `TrimStart` and `TrimEnd` when you're not sure about the purity of your string data.
Best Practices for String Trimming
- Always Validate Input Before Processing: Before using `TrimStart`, consider validating if the data indeed needs trimming. Excessive trimming can lead to loss of critical information in some cases.
- Chain Trimming Methods: Use both `TrimStart` and `TrimEnd` together for comprehensive cleanup if you're uncertain about your data's format.
- Document Your Code: When manipulating strings that may come from various sources, comment your code to clarify that you’ve intentionally included trimming methods.
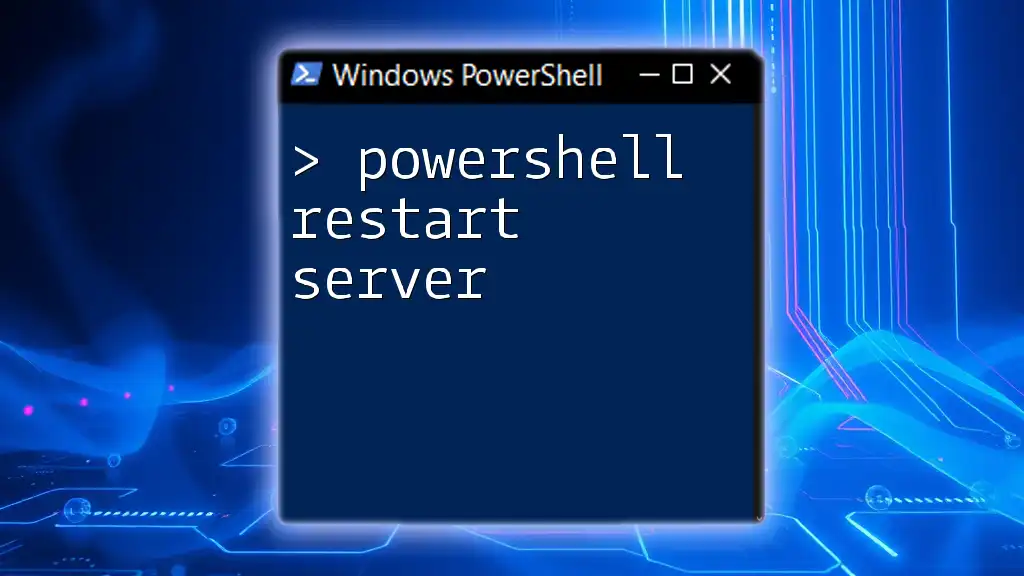
Conclusion
Understanding and effectively using `TrimStart` in PowerShell enhances your capability to manage string data efficiently. It is a simple yet powerful tool that, when combined with its counterpart `TrimEnd`, can lead to cleaner, more accurate data processing. Practice using these functions in various scenarios to solidify your understanding and improve your scripting skills. Exploring further capabilities in PowerShell string manipulation will expand your mastery and utility of this robust scripting language.
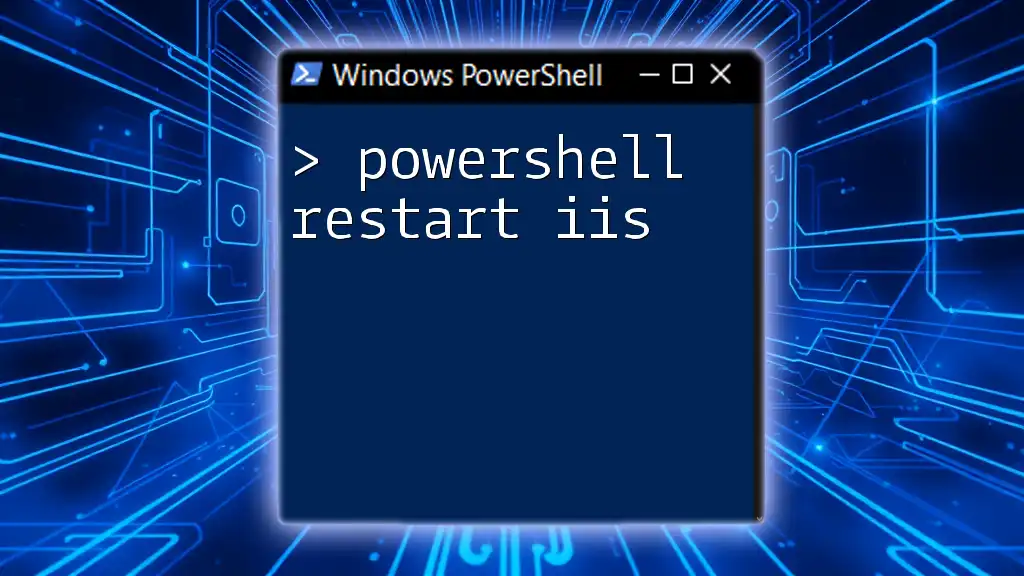
Additional Resources
For deeper insights, consider exploring the official PowerShell documentation for `TrimStart` and `TrimEnd`. Delving into related topics such as regex for precise string handling can also broaden your proficiency in string manipulation, enhancing your overall workflow.