To restart a service using PowerShell, you can use the `Restart-Service` cmdlet followed by the service name to quickly and effectively restart the specified service.
Here’s a code snippet:
Restart-Service -Name 'YourServiceName'
Understanding Windows Services
What is a Windows Service?
A Windows Service is a specialized type of application that operates in the background and does not require user interaction. Services are crucial for maintaining the operational integrity of the Windows operating system, as they manage various tasks like handling network requests, managing hardware processes, and even running scheduled tasks. Unlike standard applications, which run in user sessions, Windows Services can run without a user being logged into the system.
Importance of Restarting Services
Restarting services is often necessary for a variety of reasons:
- Performance Issues: Over time, services can consume more memory or CPU resources, which may lead to degraded performance.
- Updates and Configuration Changes: After installing updates or changing configuration settings, a service may need to be restarted for the changes to take effect.
- Error Recovery: Services may occasionally hang or become unresponsive. Restarting them can restore normal functionality.
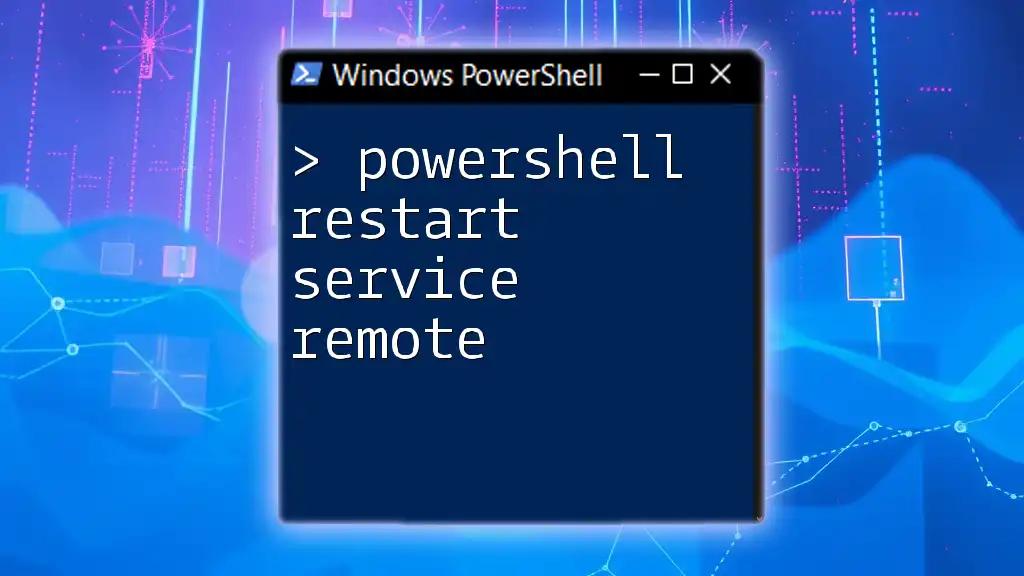
PowerShell Basics for Service Management
Introduction to PowerShell
PowerShell is a task automation framework that includes a command-line shell and a scripting language, primarily designed for system administration. It enables administrators to perform various tasks through cmdlets, which are specialized .NET classes.
Cmdlet Overview
When managing Windows Services through PowerShell, a few cmdlets are frequently utilized:
- `Get-Service`: Retrieves the status of services on the system.
- `Start-Service`: Starts a stopped service.
- `Stop-Service`: Stops a running service.
- `Restart-Service`: The focus of this article; it stops a running service and then starts it again.
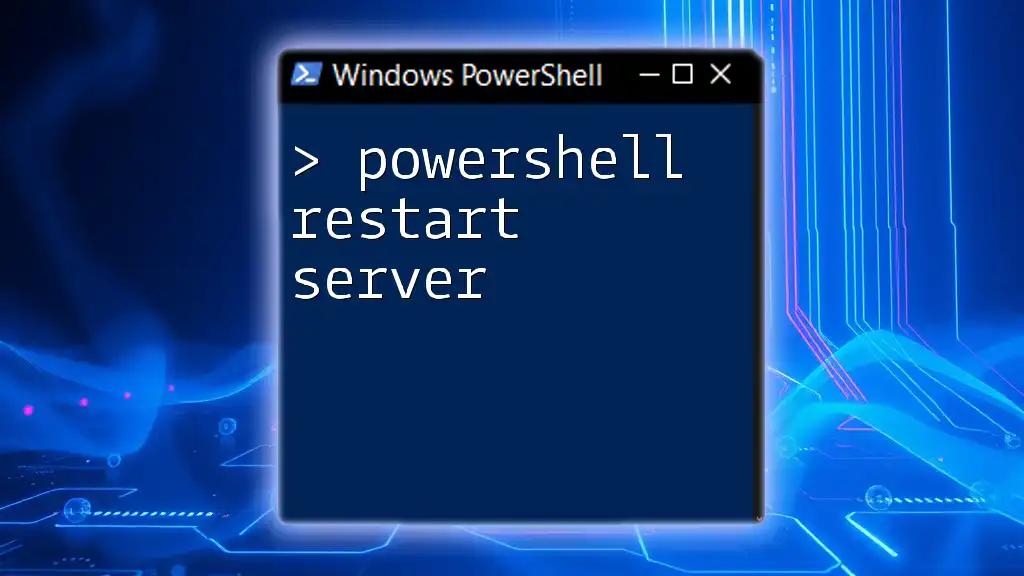
Restarting Services with PowerShell
The `Restart-Service` Cmdlet
The `Restart-Service` cmdlet is designed to stop and then start a Windows service in a single command. Its syntax is straightforward:
Restart-Service -Name "ServiceName"
This command requires the service's name as a parameter, and it’s important to use the exact name of the service as it appears in the Services management console.
Basic Usage of Restart-Service
To restart a service using PowerShell, the command can be quite simple. For example, if you want to restart the Windows Update service, you would use:
Restart-Service -Name "wuauserv"
This command sends a stop signal to the Windows Update service, and once it has stopped, it automatically issues a start signal. Understanding how this cmdlet works can help you effectively manage services without needing deep technical knowledge.
Restarting a Service with Options
Using the `Force` Parameter
In some cases, you may need to forcibly restart a service, especially if it is not responding to a standard stop request. The `-Force` flag can be added to the command:
Restart-Service -Name "Spooler" -Force
By using this parameter, you instruct PowerShell to disregard the service status and simply restart it, which can be useful in recovering hung services.
Using the `Delay` Parameter
If you would like to introduce a delay before restarting a service, the `-Delay` option allows for this control:
Restart-Service -Name "bits" -Delay 10
In this example, PowerShell will wait for 10 seconds after stopping the Background Intelligent Transfer Service before starting it again. This can help ensure that the service has completely stopped before it attempts to start again.
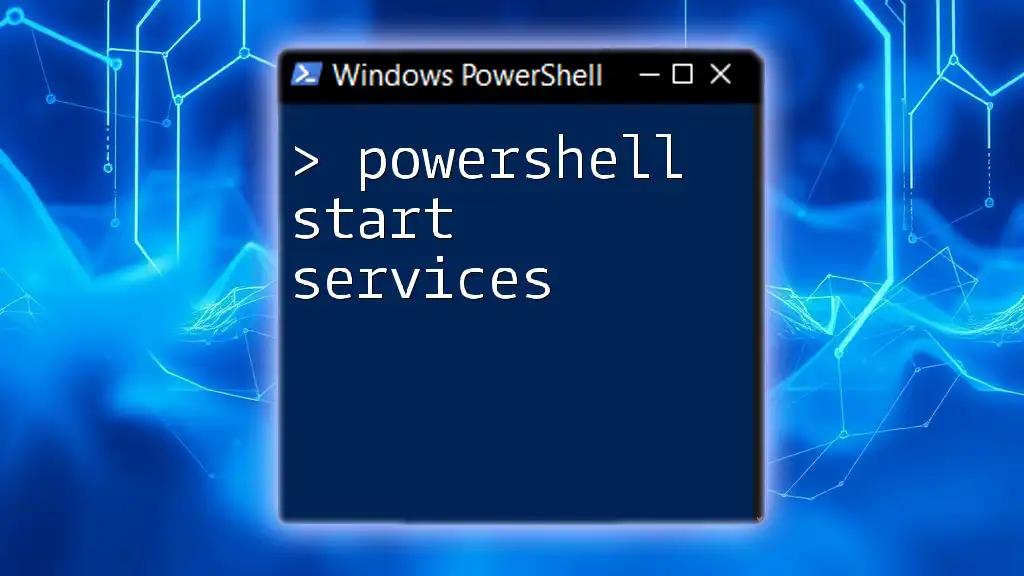
Advanced Techniques
Restarting Multiple Services
PowerShell excels at handling multiple items simultaneously. To restart more than one service at once, you can utilize a pipeline approach with the `ForEach-Object` cmdlet:
"Service1", "Service2" | ForEach-Object { Restart-Service -Name $_ }
This command effectively restarts each service specified in the list. It illustrates the power of PowerShell’s ability to handle collections of objects efficiently.
Checking Service Status Before Restarting
Before you attempt to restart a service, it is prudent to check its status. You can utilize the `Get-Service` cmdlet alongside a conditional statement:
$service = Get-Service -Name "MSSQLSERVER"
if ($service.Status -eq 'Running') { Restart-Service -Name "MSSQLSERVER" }
In this example, the script checks if the SQL Server service is running. If it is, then the command will proceed to restart it. This extra step can prevent unnecessary errors.
Creating a PowerShell Script to Restart a Service
Building a PowerShell script to automate the service restart process can save time and ensure consistency. Here’s how you can create a simple script:
param (
[string]$ServiceName
)
$service = Get-Service -Name $ServiceName
if ($service.Status -eq 'Running') {
Restart-Service -Name $ServiceName
} else {
Write-Output "Service $ServiceName is not running."
}
This script accepts the service name as a parameter, checks if it’s running, and then restarts it if necessary. If the service isn’t running, it outputs a user-friendly message. This approach allows you flexibility and reusability in service management.
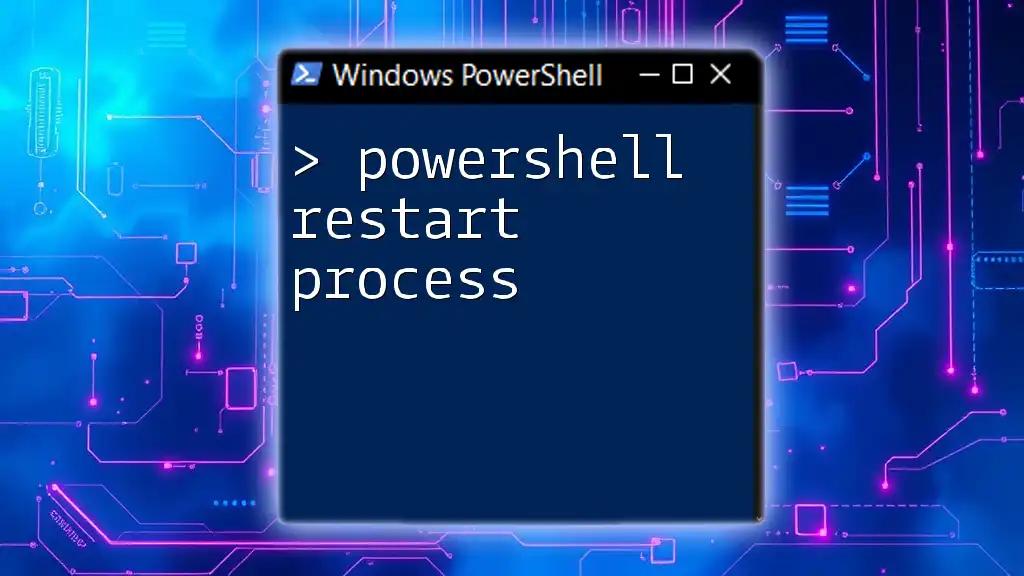
Troubleshooting Common Issues
Error Handling in PowerShell
When executing service restart commands, you may encounter various errors, such as permission issues or service dependency failures. Implementing error handling using try-catch blocks can provide more control:
try {
Restart-Service -Name "SomeService"
} catch {
Write-Output "Failed to restart service: $_"
}
This mechanism captures any error generated by the command and displays a helpful message, allowing for easier troubleshooting.
Logging and Monitoring Service Restarts
To maintain oversight of service management actions, it's useful to implement logging. You can log restart actions to a file for later review:
Restart-Service -Name "ServiceName" -Verbose | Out-File "C:\Logs\ServiceRestart.log" -Append
In the above command, the `-Verbose` switch provides detailed output which is then appended to a log file. This practice offers insight into your service management operations.
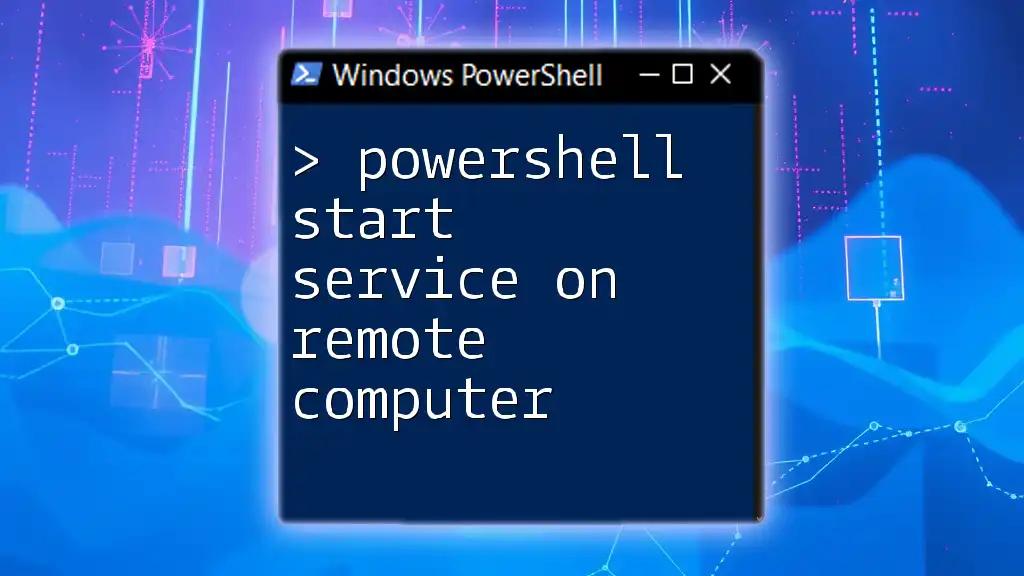
Best Practices
Timing Considerations
When scheduling service restarts, it’s best to consider timing. Performing restarts during off-peak hours can minimize impact on users and ensure that the services are restarted when they are least likely to be needed.
Automation Tips
To further streamline service management, consider using Task Scheduler in Windows to automate PowerShell scripts. By scheduling a script to run at specific intervals or events, you can ensure services are maintained without manual intervention, ultimately improving system reliability.
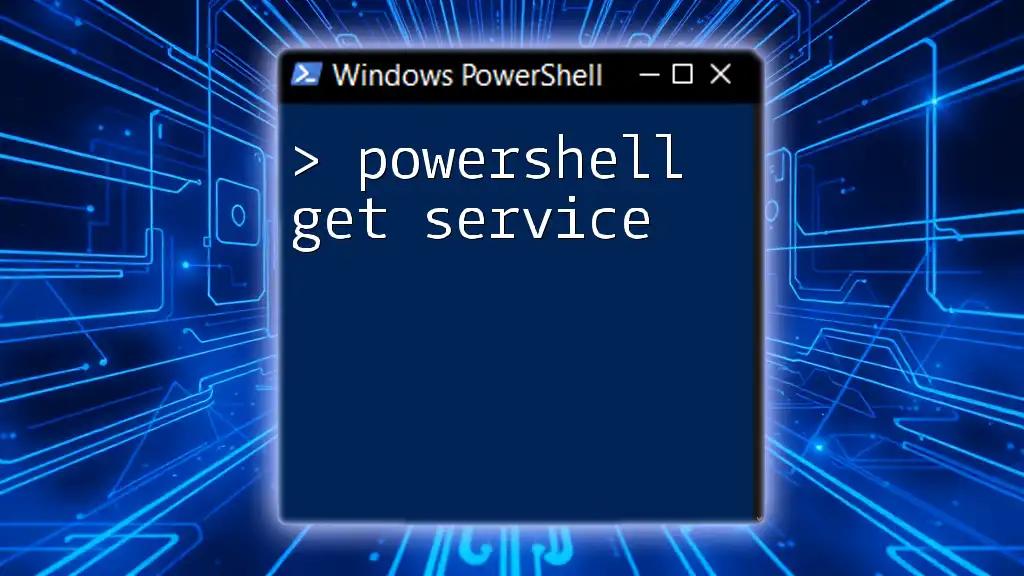
Conclusion
Utilizing PowerShell for managing Windows services, particularly the `Restart-Service` cmdlet, offers immense flexibility and efficiency. With a solid grasp of these commands and best practices, you can ensure a smoother operation of services within your environment, leading to improved performance and reduced downtime. Experimenting with these commands not only enhances your skills but also fosters a deeper understanding of the Windows operating system's service management capabilities.