To restart a process in PowerShell, you can use the `Stop-Process` cmdlet followed by the `Start-Process` cmdlet to first stop the process and then start it again. Here's a code snippet demonstrating this:
Stop-Process -Name "notepad" -Force; Start-Process "notepad"
Understanding PowerShell Processes
What is a Process?
In computing, a process refers to an instance of a program that is being executed. It contains the program code and its current activity. Each process has its own memory space, system resources, and is identified by a unique Process ID (PID). PowerShell provides powerful cmdlets to manipulate processes, allowing you to manage them effectively right from your command line.
Common Use Cases for Restarting a Process
Understanding when to restart a process can significantly enhance your productivity and system performance. Here are some scenarios where it may be necessary:
- Application Freezes: When an application becomes unresponsive, you might need to restart it to restore functionality.
- Performance Issues: Over time, certain applications may consume excessive resources. Restarting them can free up memory and CPU.
- Updates: Many applications require a restart after updates are applied. Knowing how to automate this with PowerShell can save a lot of time.
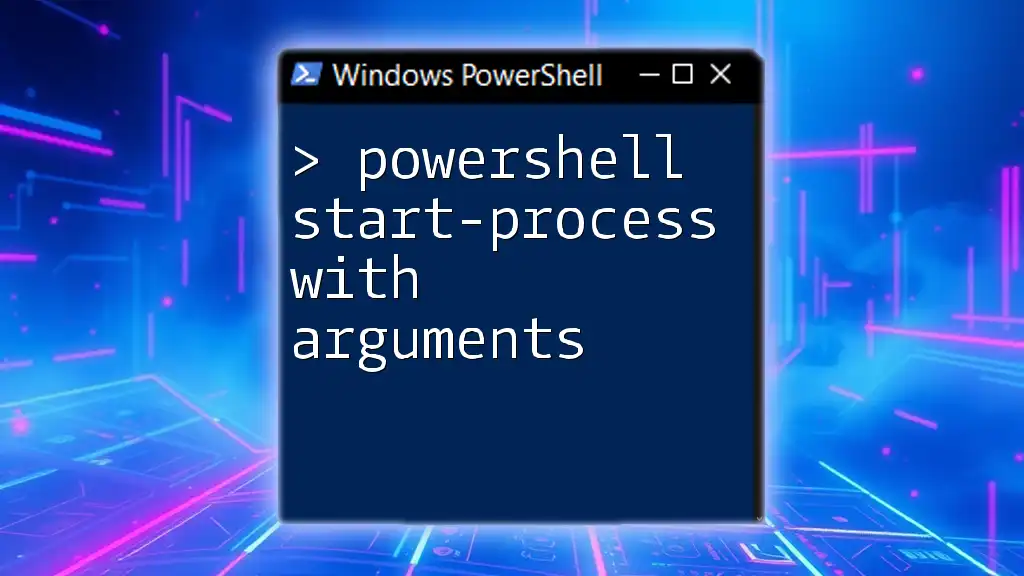
Preparation: Getting Started with PowerShell
Opening PowerShell
To unlock the capabilities of PowerShell, you first need to open it. There are several methods:
- Start Menu: Click on the Start Menu and type "PowerShell" to locate and launch it.
- Run Dialog: Press `Windows + R`, type "powershell", and hit Enter.
For administrative tasks, right-click on the PowerShell icon and choose Run as Administrator. This is crucial for executing commands that require elevated permissions.
PowerShell Basics
Before diving into the commands, it’s helpful to understand some basics of PowerShell:
Commandlets and Syntax: PowerShell commands, known as cmdlets, follow a verb-noun format, making them intuitive. For instance, `Get-Process` retrieves currently running processes. You can always use the `Get-Help` cmdlet to learn more about any cmdlet and its usage.
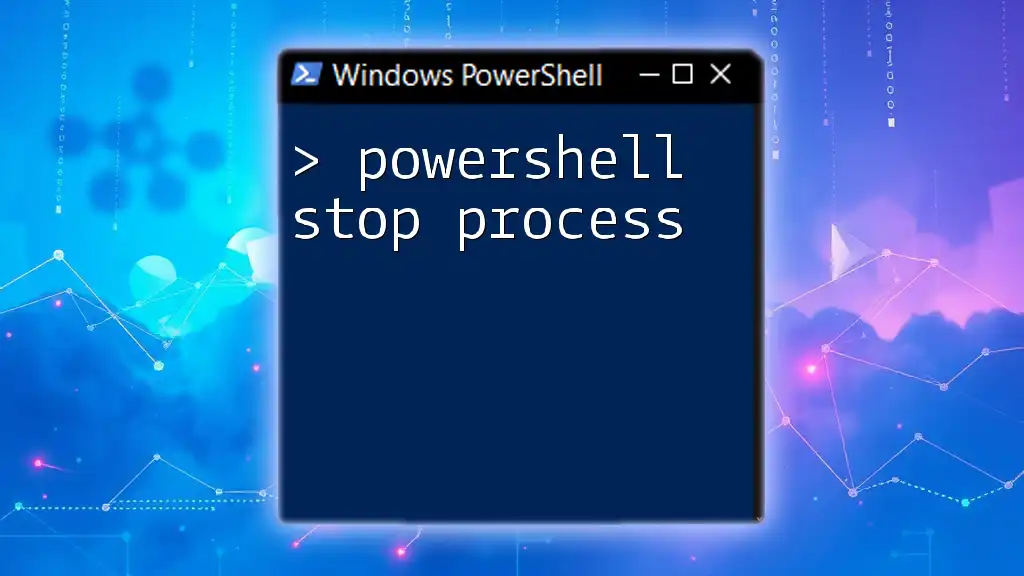
Restarting a Process in PowerShell
Using Stop-Process and Start-Process Cmdlets
Syntax Overview
The two main cmdlets you will use for restarting processes are `Stop-Process` and `Start-Process`. Their syntax is straightforward, which makes them convenient for managing processes.
Stopping a Process
To close a process, you can use the `Stop-Process` cmdlet. Here’s how it looks:
Stop-Process -Name "processName" -Force
-
-Name: Specifies the name of the process you wish to stop.
-
-Force: Closes the process immediately without prompting for confirmation, which is useful for unresponsive applications.
Starting a Process
Once you've stopped a process, you can easily start it again using the `Start-Process` cmdlet. The syntax is as follows:
Start-Process "processPath"
- "processPath": Replace this with the full path of the executable you want to start. If it's in your system PATH, you can just use the process name.
Example: Restarting Notepad
Let’s walk through a practical example of restarting Notepad. This scenario is useful when Notepad becomes unresponsive:
-
Stopping Notepad:
Stop-Process -Name "notepad" -Force
-
Starting Notepad:
Start-Process "notepad"
This sequence effectively refreshes the Notepad application, getting rid of any unresponsiveness it may have.
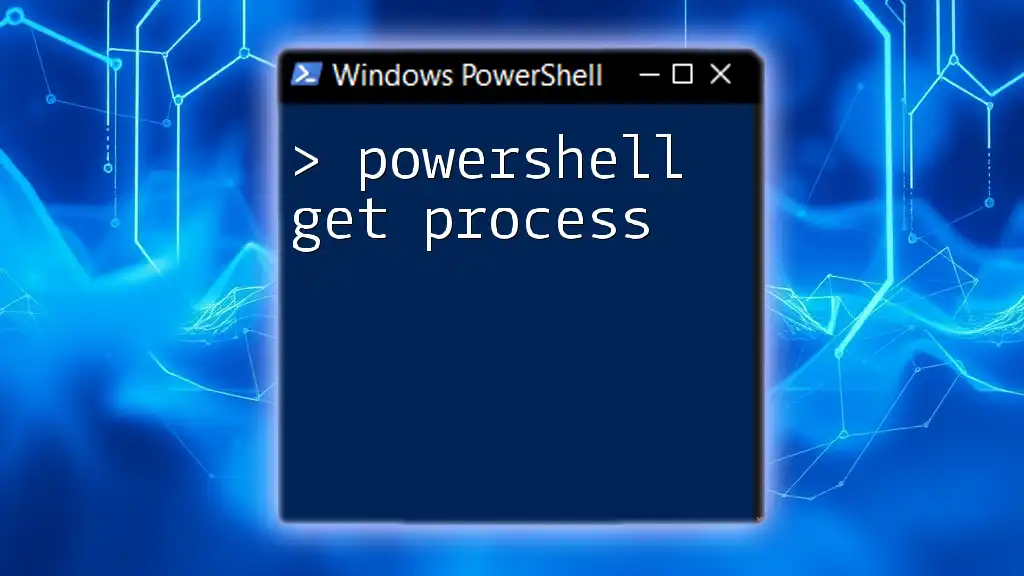
Advanced Techniques for Restarting Processes
Restart-Process Cmdlet
What is Restart-Process?
The `Restart-Process` cmdlet combines the functionalities of both `Stop-Process` and `Start-Process`. This cmdlet is particularly beneficial because it simplifies the process of restarting applications with a single command.
Syntax and Parameters
A typical usage of the `Restart-Process` cmdlet is as follows:
Restart-Process -Name "processName" -Force
Again, the -Force parameter is included for immediate termination without confirmation, which is useful for unresponsive apps.
Example: Using Restart-Process
To illustrate, here’s how you would restart Google Chrome using the `Restart-Process` cmdlet:
Restart-Process -Name "chrome" -Force
This command quickly closes any running instances of Chrome and immediately relaunches it.
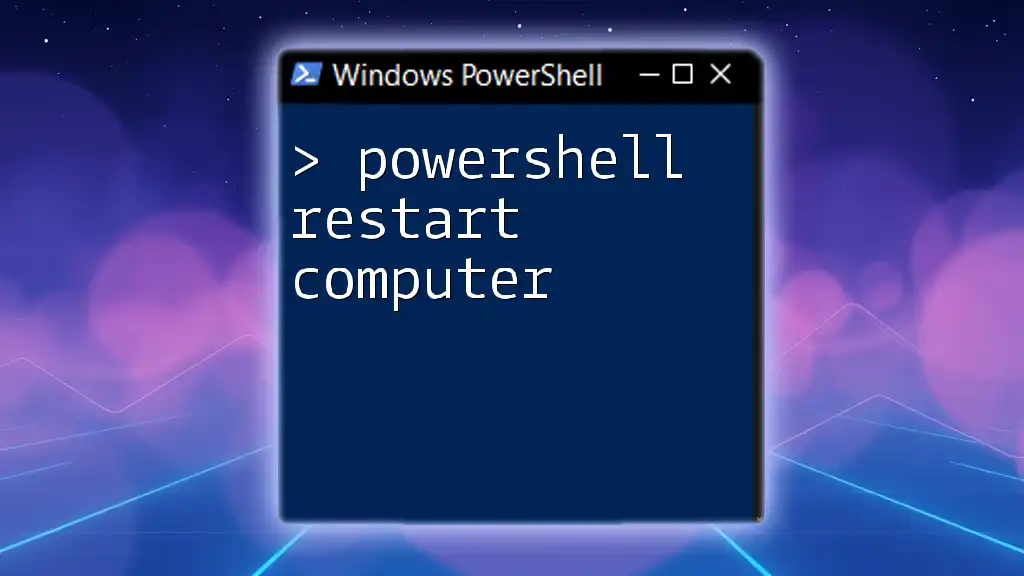
Using Filters and Conditions
Filtering Processes by Status
In some cases, you might want to restart a process only if it’s currently running. This prevents unnecessary errors from attempting to stop a non-existing process.
Here’s a classic way to achieve this:
$process = Get-Process -Name "myApp" -ErrorAction SilentlyContinue
if ($process) {
Stop-Process -Name "myApp" -Force
Start-Process "myApp"
}
In this example:
- Get-Process: Retrieves the specified process.
- -ErrorAction SilentlyContinue: Instructs PowerShell to continue without throwing an error if the process is not found.
- The `if` statement checks whether the process is active before attempting to stop it.
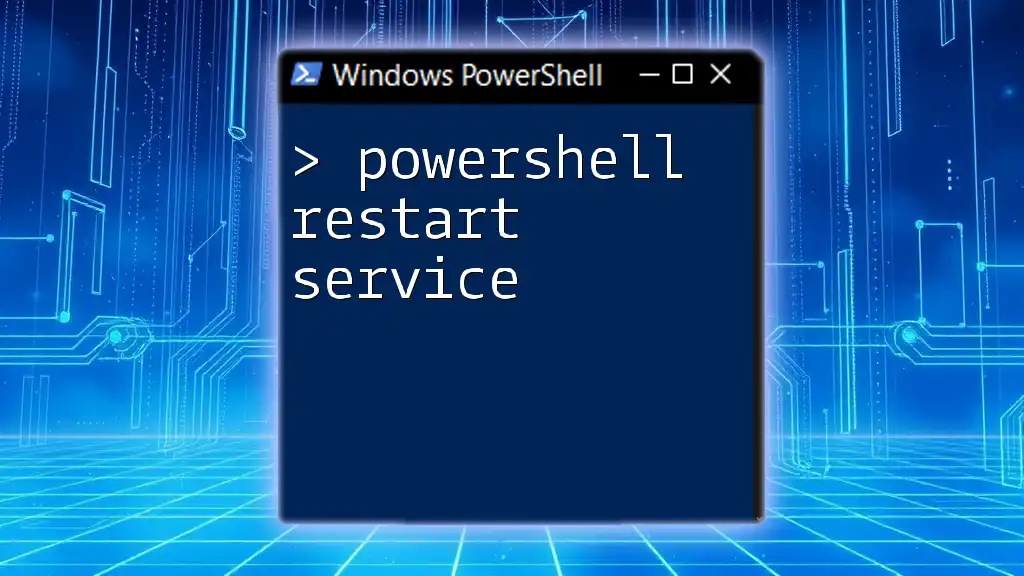
Automating Process Restarts
Creating a Function
Creating a custom function in PowerShell allows for reusability and efficiency. Here is a straightforward function to restart any given process:
function Restart-MyProcess {
param (
[string]$processName
)
Stop-Process -Name $processName -Force -ErrorAction SilentlyContinue
Start-Process -Name $processName
}
This function takes the process name as a parameter and manages the stopping and starting in one reusable command.
Scheduling Your Script with Task Scheduler
Once you have your script ready, you can automate its execution using Windows Task Scheduler:
- Open Task Scheduler and create a new task.
- In the "Actions" tab, select "Start a program" and specify your PowerShell script.
- Set triggers as per your requirements (e.g., every hour, daily, etc.).
This automates the restart of processes, thus enhancing system performance in the background.
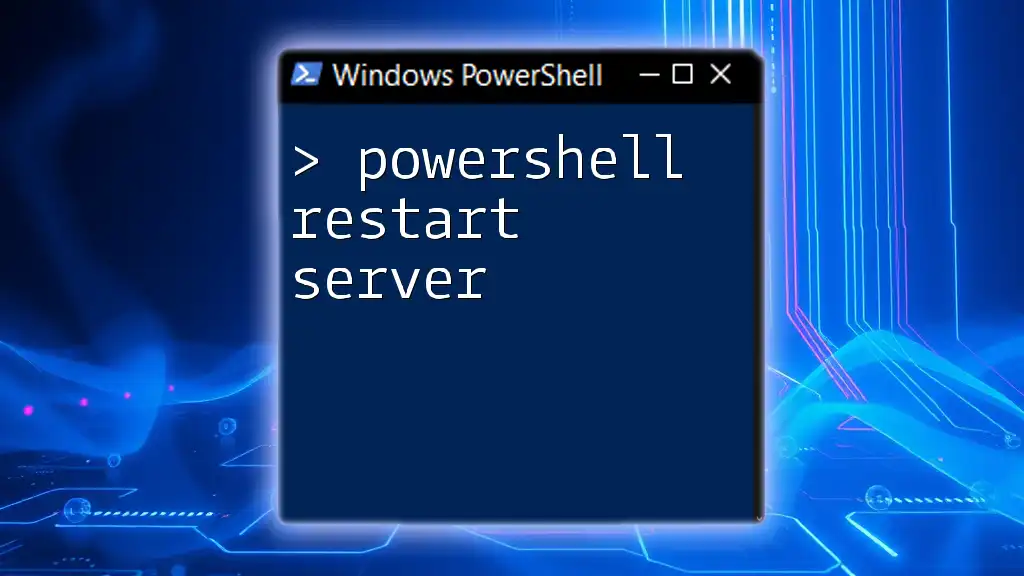
Troubleshooting Common Issues
Permission Denied Errors
Sometimes, you may face permission issues when trying to stop or start processes. Ensure that you are running PowerShell with elevated permissions. Use Run as Administrator to resolve these errors.
Process Not Found Errors
If you encounter a "process not found" error, it is often due to supplying an incorrect process name. Verify the name using the `Get-Process` cmdlet to ensure it matches exactly.
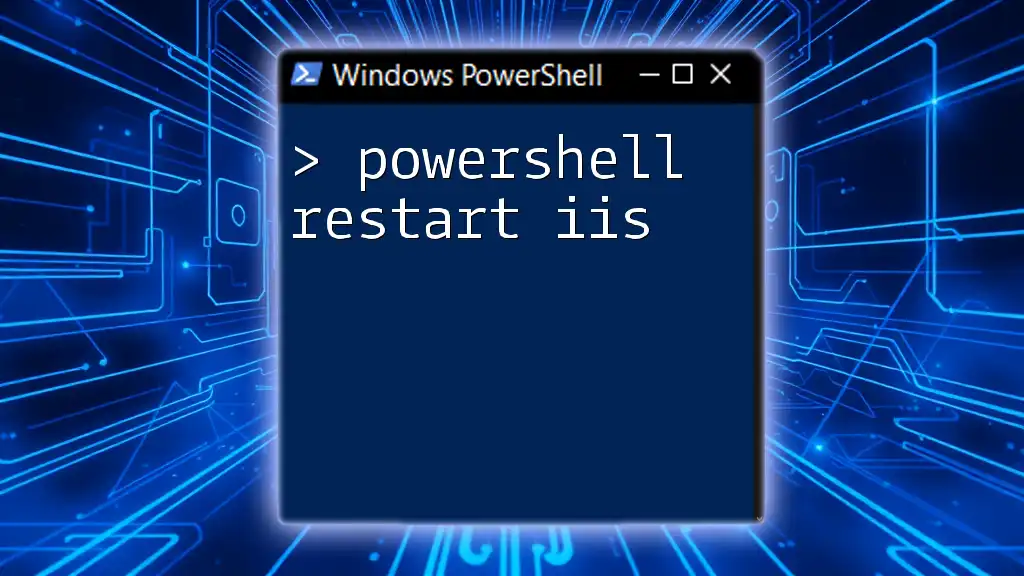
Best Practices for Process Management
To effectively manage processes through PowerShell, here are a few best practices:
- Test Your Commands: Always test commands in a safe environment before deploying them in a production setting.
- Use Comments: In your scripts, include comments to explain the purpose of each command for future reference.
- Regular Cleanup: Periodically monitor and clean running processes to ensure optimal system performance.
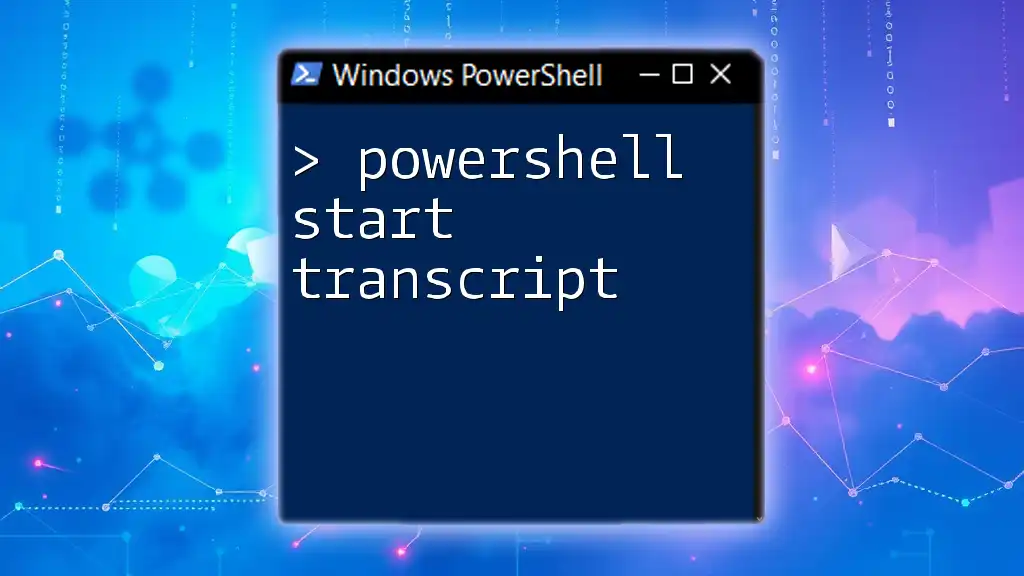
Conclusion
With the knowledge of PowerShell commands for restarting processes, you are now equipped to manage applications more efficiently. Practice these commands in various scenarios to deepen your understanding and streamline your workload with PowerShell's powerful capabilities. The ability to quickly restart processes will not only save time but also ensure that your systems remain robust and responsive.