A PowerShell expression is a piece of code that evaluates to a value; it can be a simple command, a variable, or a combination of operators and functions.
Write-Host 'Hello, World!'
Understanding PowerShell Expressions
What is a PowerShell Expression?
A PowerShell expression is an instruction that calculates and produces a value. Expressions can include variables, operators, and literals, allowing for a dynamic evaluation in scripts. They are essential in scripting and automation, enabling users to perform calculations, manipulate strings, and evaluate conditions seamlessly. In many ways, expressions can be likened to the components found in other programming languages, yet PowerShell's syntax is designed to enhance readability and execution efficiency.
Types of PowerShell Expressions
Literal Expressions: These are the simplest forms of expressions consisting of constant values, such as numbers or strings. They are straightforward and are utilized when no calculations or manipulations are needed. For instance, the string `"Hello, World!"` is a literal expression.
Arithmetic Expressions: These expressions perform mathematical operations such as addition, subtraction, multiplication, and division. For example, if you want to calculate the sum of two numbers, you would write:
$result = 5 + 10
Write-Output "The result is: $result"
String Expressions: String expressions manipulate text data. You can concatenate multiple strings or format them using variables. For example, consider the following code snippet that concatenates strings:
$name = "John"
$greeting = "Hello, " + $name + "!"
Write-Output $greeting
Logical Expressions: These expressions evaluate conditions and return Boolean values (`True` or `False`). For instance, with logical operators like `-and`, `-or`, and `-not`, you can build conditions to make decisions in your scripts. For example:
$isAdult = $age -ge 18
Write-Output "Is the person an adult? $isAdult"
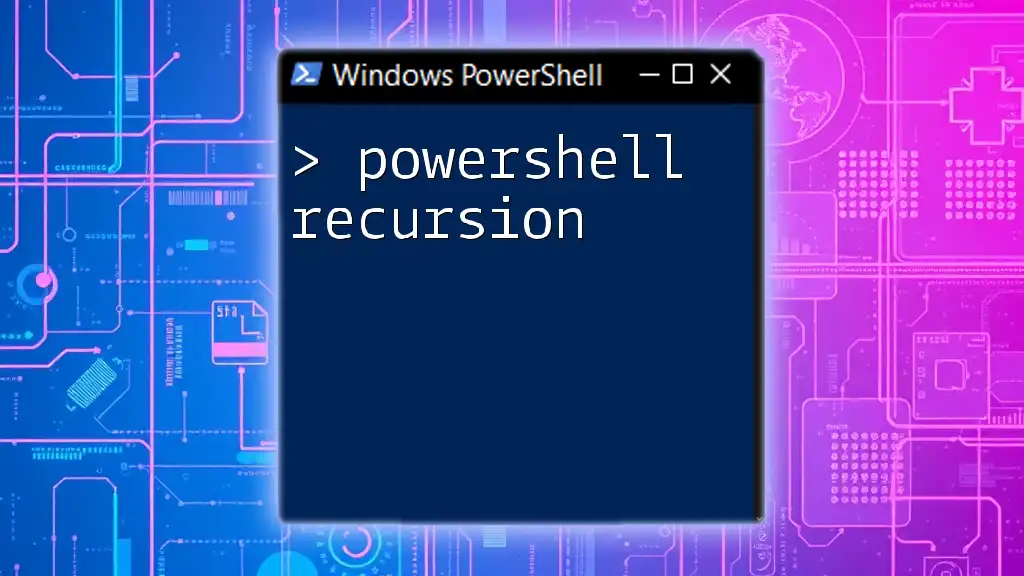
Building PowerShell Expressions
Syntax and Structure
Understanding the syntax is key to crafting effective PowerShell expressions. An expression typically consists of operators, variables, and values. For example, `$a + $b` combines two variables using the addition operator. The correct structure ensures that PowerShell can interpret the expression correctly.
Using Variables within Expressions
Variables in PowerShell are defined using the `$` sign and can store various data types. To create expressions that incorporate variables, you would first declare the variables, then use them in calculations or evaluations. For example:
$number1 = 5
$number2 = 10
$sum = $number1 + $number2
Write-Output "The sum is: $sum"
Operator Precedence in PowerShell
Operator precedence determines the order in which operators in an expression are evaluated. Understanding this concept can prevent unintended results. For instance, in the expression `$a + $b * $c`, PowerShell evaluates multiplication before addition. To ensure expressions are evaluated in the desired order, parentheses can be used:
$result = ($a + $b) * $c
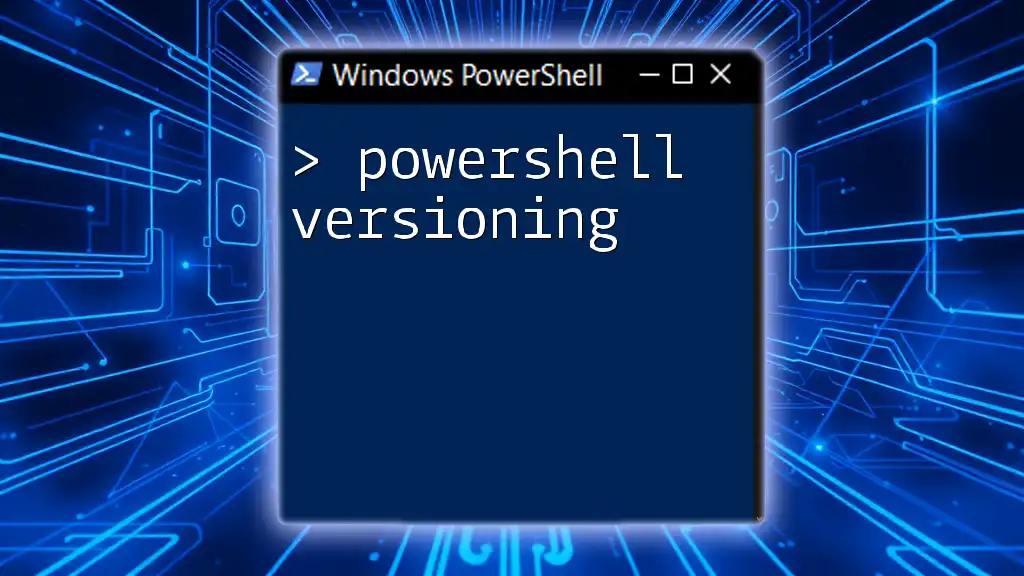
Evaluating PowerShell Expressions
The `Write-Output` Cmdlet
The `Write-Output` cmdlet is a fundamental way to display the results of expressions. By utilizing this cmdlet, you can effectively communicate the outcomes of your expressions, whether they are arithmetic results, evaluations, or string manipulations. For example:
$temperature = 100
$isFever = $temperature -gt 98
Write-Output "You have a fever: $isFever"
Logical Evaluation and Truthiness
PowerShell expressions evaluate Boolean values based on their logical constructs. An expression using comparison operators (like `-eq`, `-lt`, `-gt`) will yield either `True` or `False`. For instance:
$temperature = 100
$isHot = $temperature -gt 90
Write-Output "Is it hot? $isHot"
This simple expression evaluates the variable `$temperature` and determines if it is greater than 90.
Using `if` Statements with Expressions
Incorporating expressions into `if` statements allows for conditional logic in your scripts. Here’s a straightforward example of an if statement utilizing an expression:
$age = 16
if ($age -ge 18) {
Write-Output "You are an adult."
} else {
Write-Output "You are not an adult."
}
This script checks if the `$age` variable meets the condition and outputs a corresponding message.
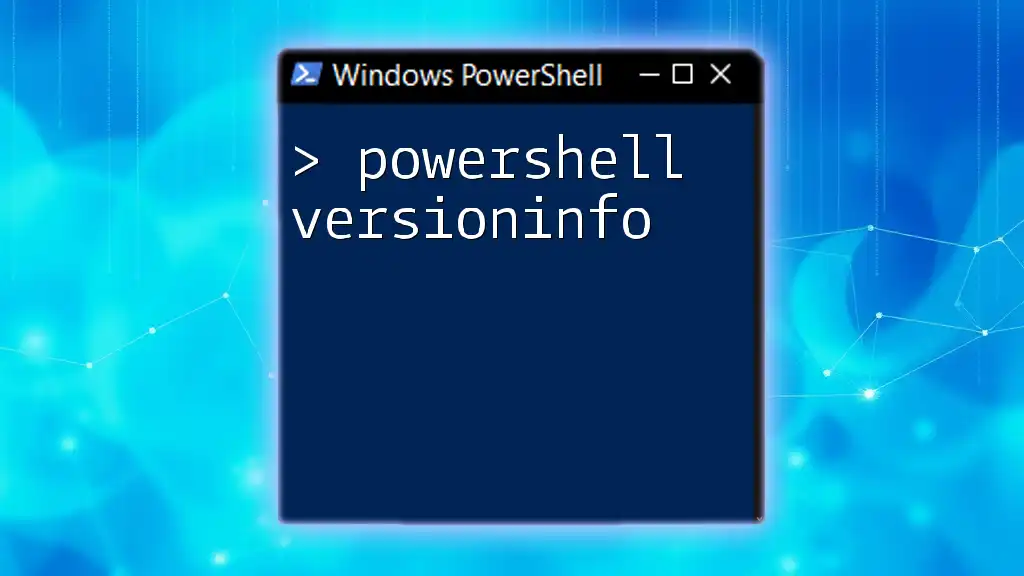
Advanced PowerShell Expressions
Using Nested Expressions
Nested expressions can evaluate multiple conditions within a single structure. They enhance script capabilities by allowing compound decisions. For example:
$score = 85
if ($score -ge 90) {
Write-Output "You got an A!"
} elseif ($score -ge 80 -and $score -lt 90) {
Write-Output "You got a B!"
} else {
Write-Output "You need to study harder."
}
Expression in Loops
Expressions are incredibly useful within loop structures for repeated evaluations. For example, a `for` loop can utilize expressions to perform a task multiple times:
for ($i = 1; $i -le 5; $i++) {
$square = $i * $i
Write-Output "The square of $i is: $square"
}
This loop iterates through numbers one to five and calculates the square of each.
Working with Arrays and Collections
Expressions also play a vital role in manipulating arrays and collections. The `Where-Object` cmdlet can filter data based on an expression. For instance, to extract even numbers from an array:
$numbers = 1..10
$evenNumbers = $numbers | Where-Object { $_ % 2 -eq 0 }
Write-Output "Even Numbers: $evenNumbers"
This expression checks each element in the array and filters those that meet the condition, creating a new array with only the even numbers.
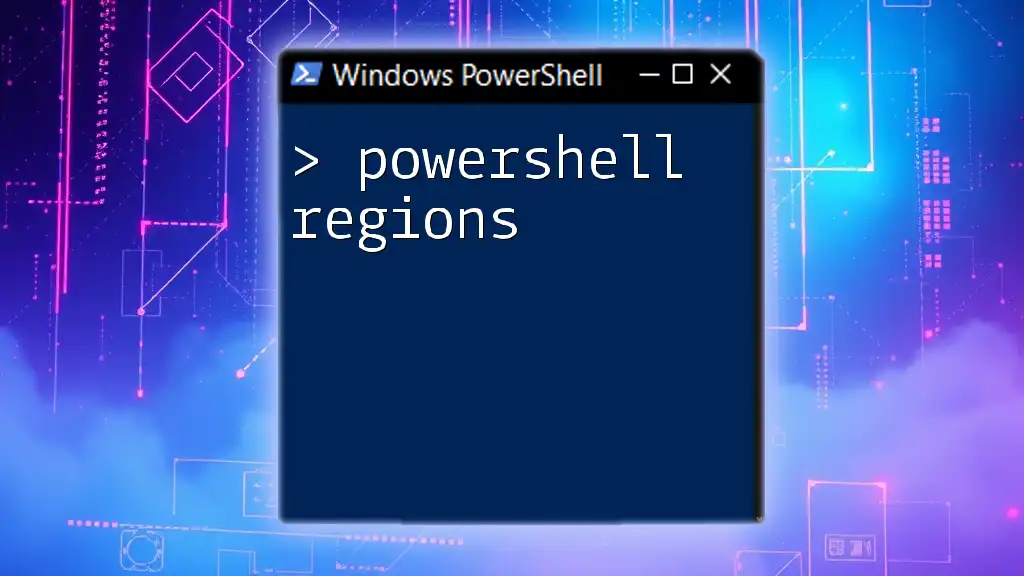
Practical Applications of PowerShell Expressions
Automating Administrative Tasks
PowerShell expressions are invaluable for automating repetitive administrative tasks. For example, checking the status of user accounts in Active Directory can be performed with succinct expressions, drastically reducing manual effort.
Scripting and Efficiency
PowerShell’s ability to perform complex evaluations in a concise manner means you can write more readable and efficient scripts. For instance, refactoring verbose code into compact expressions can significantly enhance script performance and maintainability.
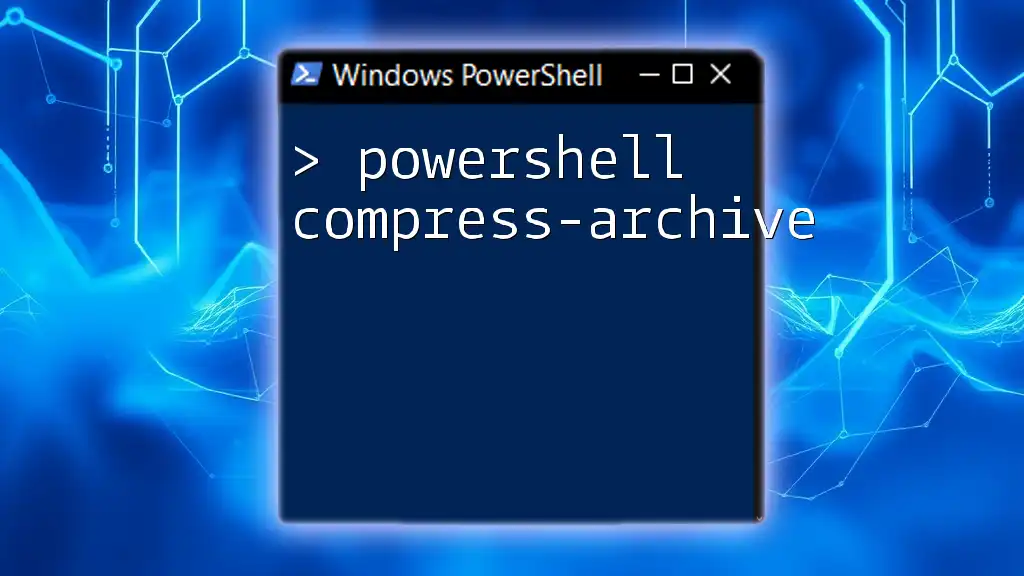
Conclusion
In this comprehensive guide, we've explored the concept of PowerShell expressions, delving into their structure, evaluation, and applications. Understanding and utilizing these expressions is essential for enhancing your scripting abilities and automating tasks effectively. As you continue learning PowerShell, remember to practice writing expressions, as they will become a fundamental part of your automation toolkit. Get started with simple expressions and gradually challenge yourself with more complex evaluations and scenarios!