PowerShell selection allows you to choose specific objects or items from a collection using conditional statements to streamline your data processing.
Here's a simple example using the `Where-Object` cmdlet to filter a list of numbers:
$numbers = 1..10
$selectedNumbers = $numbers | Where-Object { $_ -gt 5 }
Write-Host "Selected Numbers: $selectedNumbers"
Understanding Conditional Statements
The `if` Statement
The `if` statement is the backbone of decision-making in PowerShell. It allows scripts to execute certain code only when specific conditions are met. The basic syntax is straightforward:
if ($condition) {
# Do something
}
For example, if you want to check if a directory exists before attempting to create it, you can use the `if` statement as follows:
if (-Not (Test-Path 'C:\MyDirectory')) {
New-Item -ItemType Directory -Path 'C:\MyDirectory'
}
Enhancing Decision-Making with `else` and `elseif`:
You can also use `else` and `elseif` to define alternate paths of execution. This allows for more complex decision trees. Here’s how it looks:
if ($condition1) {
# Action for condition1
} elseif ($condition2) {
# Action for condition2
} else {
# Action if none of the conditions are met
}
This enables a script to branch into multiple execution paths based on varying conditions, which increases its functionality.
Switch Statement
Overview of the Switch Statement:
When faced with multiple cases that depend on a single variable, the `switch` statement can be cleaner and more efficient than a series of `if` statements.
Basic Syntax:
switch ($variable) {
'option1' { # Action for option1 }
'option2' { # Action for option2 }
default { # Default action if no case matches }
}
Using Regular Expressions in Switch:
The `switch` statement can also utilize regular expressions for pattern matching. This is beneficial when you want to match a condition against various string patterns. For instance:
switch -Regex ($input) {
'^test' { Write-Host "Input starts with 'test'" }
'\d{3}' { Write-Host "Input contains three digits" }
}
This versatility makes the `switch` statement a powerful tool for managing multiple conditions effectively.
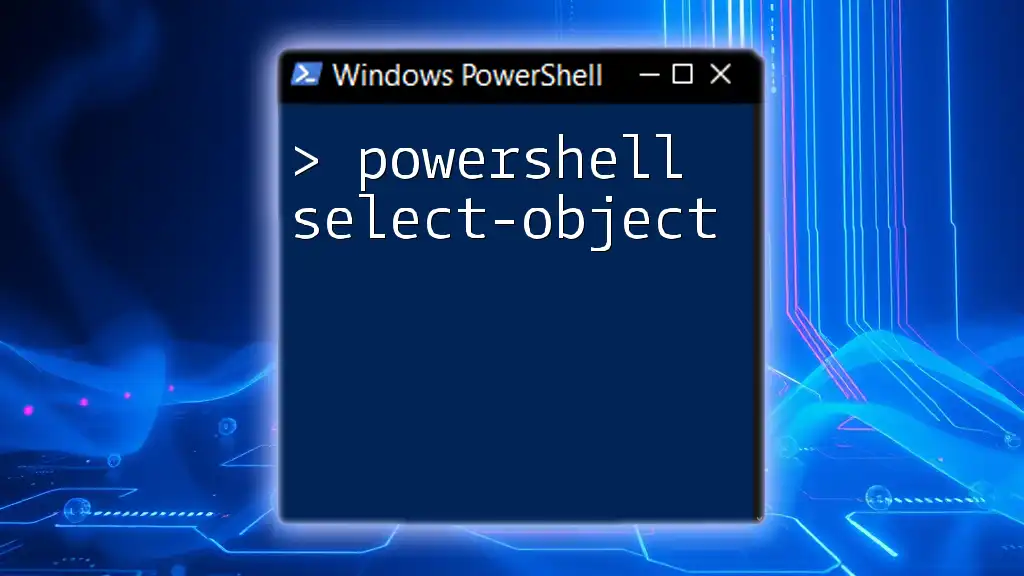
Looping Through Selections
ForEach Loop
Introduction to ForEach:
The `ForEach` loop is an essential construct for iterating through collections within your scripts, giving you the ability to process each item sequentially.
Basic Syntax:
$items = 1..5
foreach ($item in $items) {
Write-Host "Processing item: $item"
}
This example demonstrates how `ForEach` makes it easy to perform actions on each element in an array.
Using ForEach-Object Cmdlet:
Another approach for iteration is using the `ForEach-Object` cmdlet in conjunction with the pipeline. This manner of processing is straightforward and fits naturally with the PowerShell object model:
1..5 | ForEach-Object { Write-Host "Processing item: $_" }
This method showcases how PowerShell's pipeline can interoperate with selection commands, streamlining your scripts.
Other Looping Constructs
While and Do-While Loops:
These loops provide even more flexibility in how you can handle iterations. A `while` loop continues executing as long as a specified condition remains true. Here’s an example:
$count = 0
while ($count -lt 5) {
Write-Host "Count is: $count"
$count++
}
Using looping constructs allows you to dynamically manage how many times a block of code is executed based on conditions.
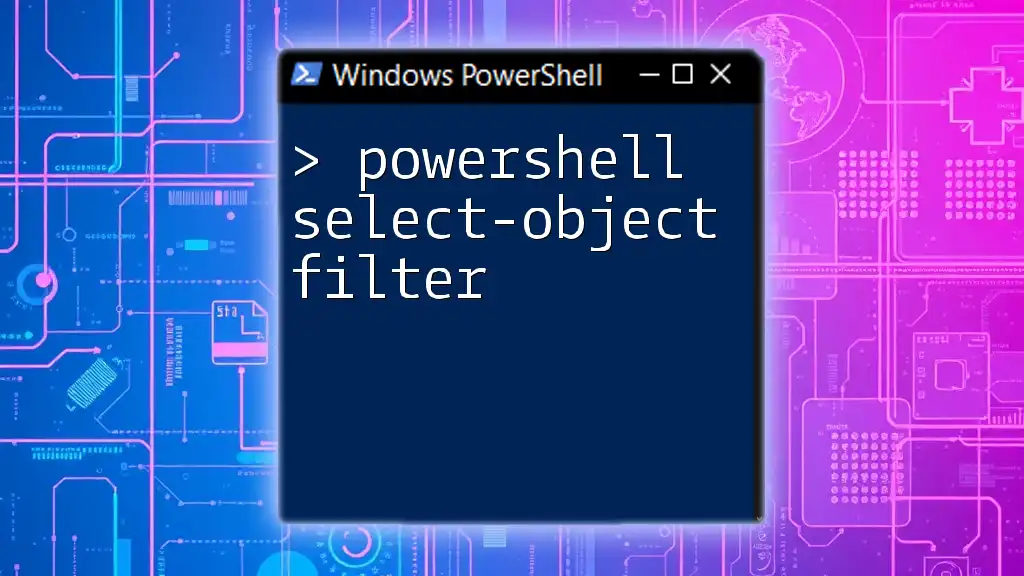
Testing and Comparing Conditions
Comparison Operators
PowerShell offers a range of comparison operators to evaluate conditions. These include:
- Equality (`-eq`)
- Inequality (`-ne`)
- Greater than (`-gt`) and Less than (`-lt`)
For example, you can use these operators to compare numeric values:
if ($x -gt 10) {
Write-Host "x is greater than 10"
}
Common Comparison Examples:
Use these operators within your scripts to handle user input, system states, and other dynamic conditions effectively.
Logical Operators
Combining Conditions:
Logical operators such as `-and`, `-or`, and `-not` enable you to combine multiple conditions into a single `if` statement. This allows for complex decision-making processes. For example:
if ($condition1 -and $condition2) {
Write-Host "Both conditions are true."
}
This capability lets scripts respond to a combination of variables or states seamlessly.
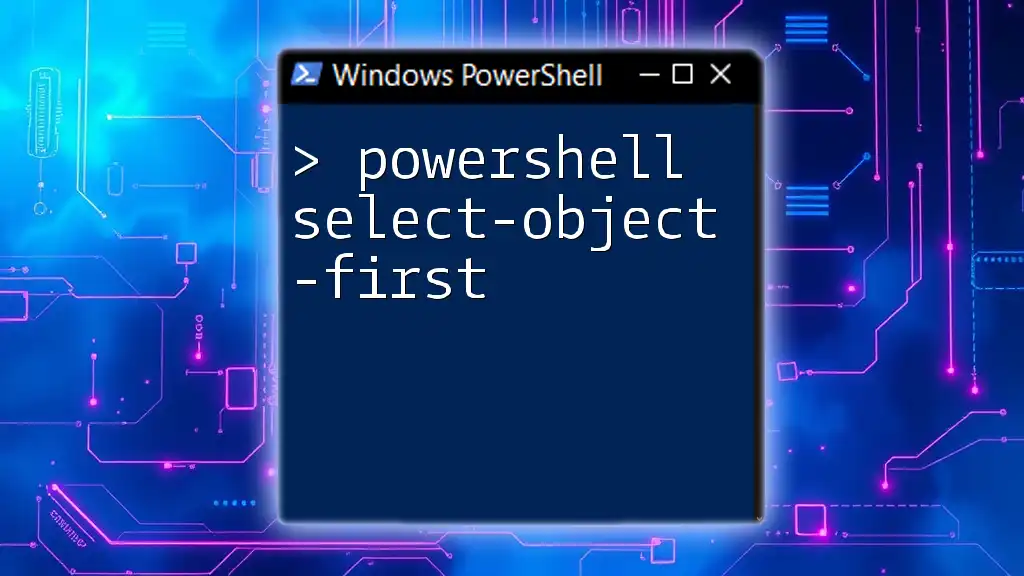
Error Handling with Selection
Try-Catch-Finally
Introduction to Error Handling in PowerShell:
Error handling is a critical aspect of scripting, especially when your scripts will be interacting with external systems or user inputs. The `try-catch-finally` construct is used to gracefully manage potential errors while executing a block of code.
Basic Syntax:
try {
# Code that may throw an error
Get-Content 'nonexistentfile.txt'
} catch {
Write-Host "An error occurred: $_"
} finally {
Write-Host "Execution completed."
}
This structure allows you to handle errors while ensuring that necessary cleanup or follow-up actions can be taken.
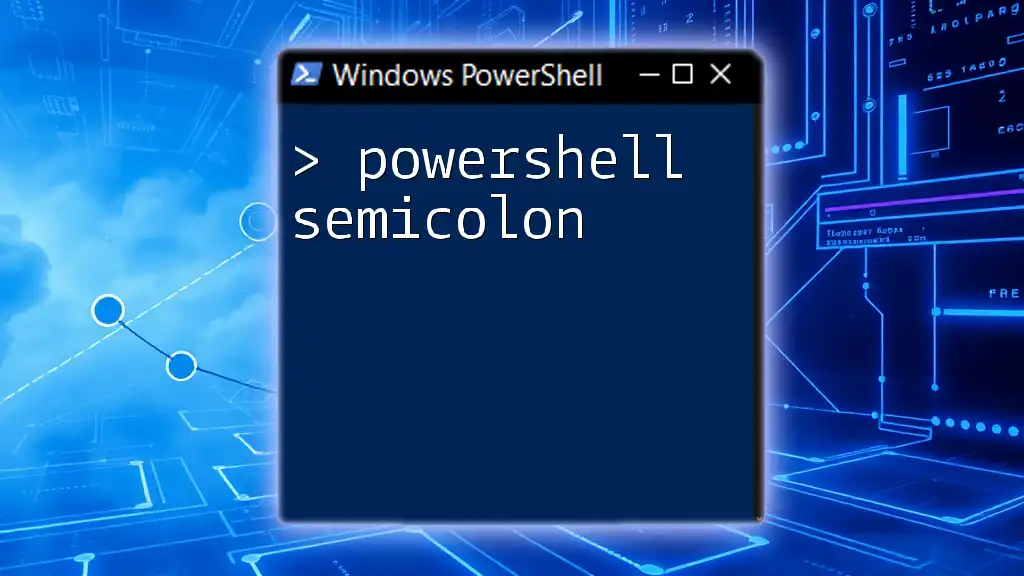
Real-World Use Cases of PowerShell Selection
Automating System Management
Consider a script that automates tasks based on system availability. You can check if a service is running and take action accordingly:
if (Get-Service -Name 'wuauserv' -ErrorAction SilentlyContinue) {
Write-Host "Windows Update Service is running."
} else {
Write-Host "Starting Windows Update Service..."
Start-Service -Name 'wuauserv'
}
This demonstrates how selection can be leveraged to save time by automating administrative tasks.
Script Validation
To further illustrate selection in action, consider a scenario where user input needs validation. You can prompt a user and respond based on their choice:
$userChoice = Read-Host "Enter your choice (A/B)"
switch ($userChoice) {
'A' { Write-Host "Executing action A..." }
'B' { Write-Host "Executing action B..." }
default { Write-Host "Invalid choice, please try again." }
}
This structure ensures that the script reacts appropriately based on user input, enhancing user experience and functionality.
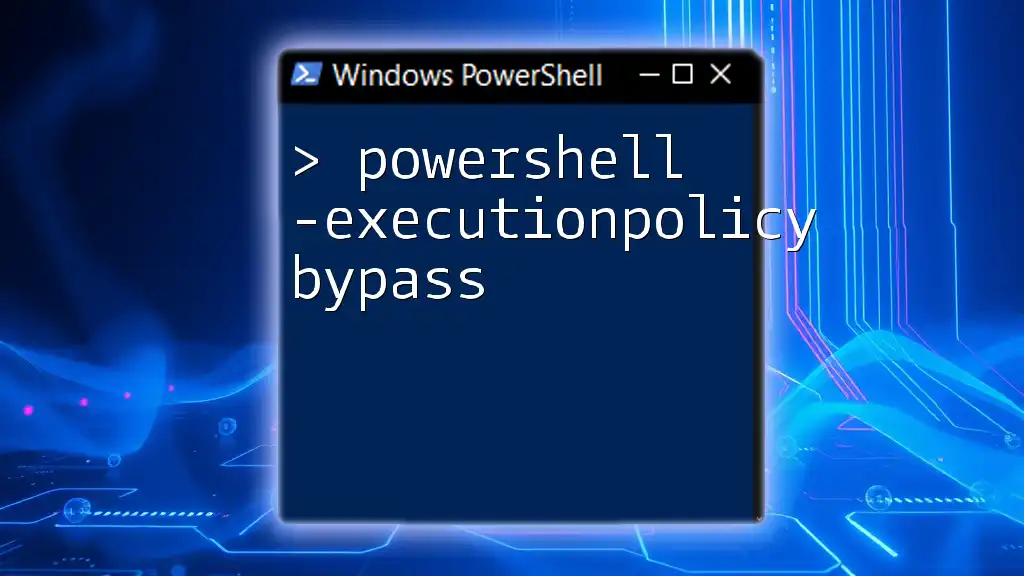
Conclusion
PowerShell selection is an essential component that allows scripts to make decisions based on dynamic conditions. By mastering if statements, switch statements, and various looping constructs, you create robust scripts that respond to different scenarios effectively. The combined power of comparison and logical operators, along with proper error handling, ensures that your automation tasks run smoothly without interruptions. Taking the time to practice and implement these techniques will undoubtedly elevate your PowerShell skills, allowing for the creation of more sophisticated and effective scripts.
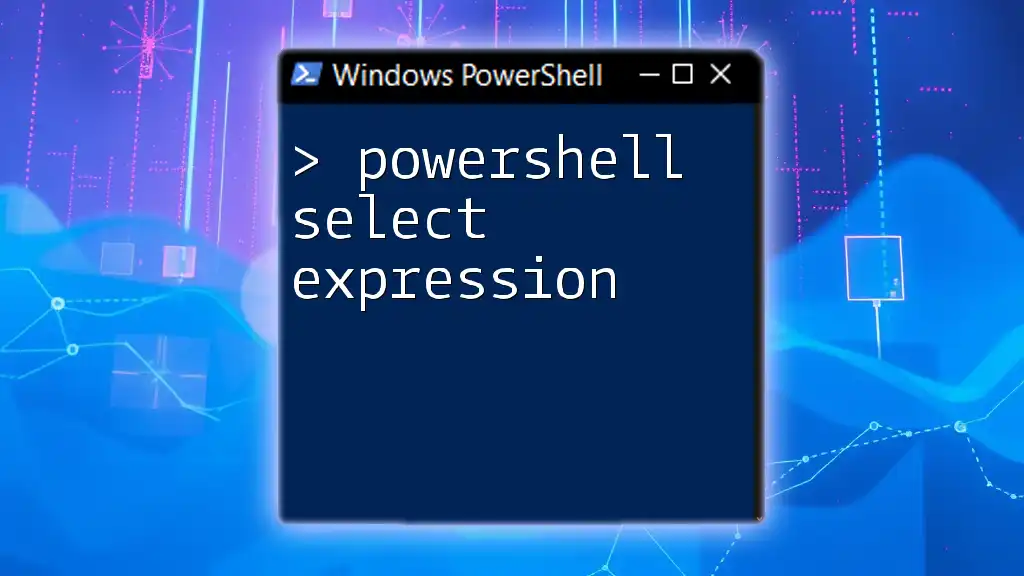
Additional Resources
There are various resources available for further exploration of PowerShell selection and conditional logic. Websites like Microsoft Docs, educational platforms, and dedicated forums can provide in-depth examples and communities to enhance your learning.