In PowerShell, a semicolon (`;`) is used to terminate commands and can be used to separate multiple commands on a single line for efficient execution.
Here's a code snippet illustrating its use:
Write-Host 'Command 1'; Write-Host 'Command 2'; Write-Host 'Command 3'
Understanding the PowerShell Semicolon
What is a Semicolon in PowerShell?
The semicolon in PowerShell acts as a command separator, allowing users to run multiple commands in a single line. This feature is particularly useful in scripting and automation tasks, where executing a series of commands consecutively can save time and improve efficiency.
When comparing PowerShell to other scripting languages, the use of semicolons is a common practice. For instance, in languages like JavaScript and C, semicolons serve a similar purpose, separating statements. However, unlike JavaScript, where omitting a semicolon might lead to unexpected behavior, PowerShell accepts new lines as command terminators, which offers some flexibility in how you structure your code.
The Role of the Semicolon
-
Command Separation: The primary function of the semicolon is to separate distinct commands that you want to run sequentially. For instance:
Get-Process; Get-Service; Get-EventLog
This command retrieves a list of running processes, services, and event logs, executing each command one after the other.
-
Logical Grouping: By grouping commands with a semicolon, you can create a streamlined command sequence. This logical structure enables better organization and flow, especially when dealing with complex tasks.
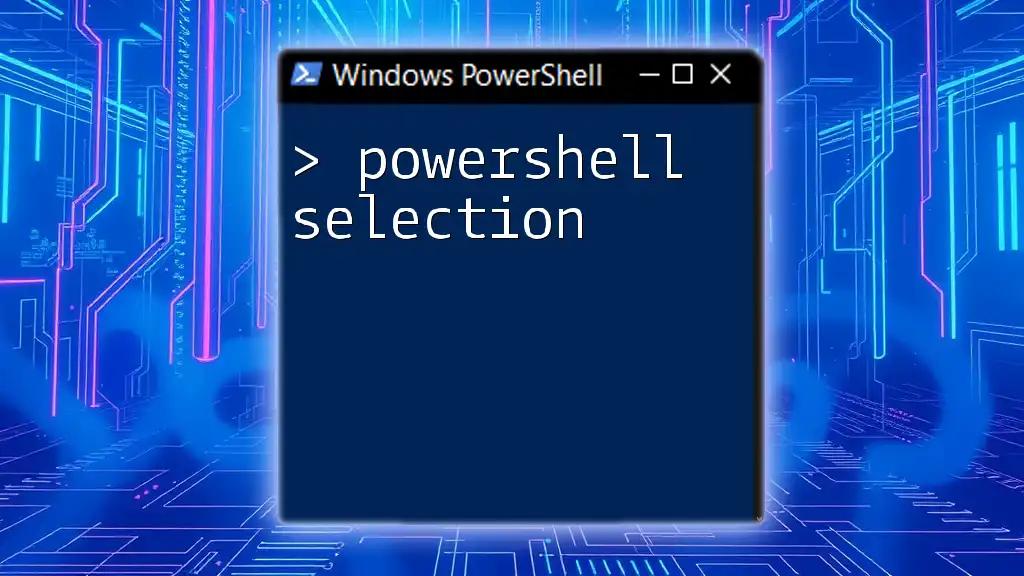
Using Semicolons in PowerShell
Basic Syntax
To effectively use the semicolon in PowerShell, it's essential to understand the basic command structure. You can place multiple commands on a single line, each separated by a semicolon. Consider this simple example:
Get-ChildItem; Clear-Host; Write-Host "Done listing files."
In this case, PowerShell will first list all files and directories, then clear the console, and finally print "Done listing files."
Combining Different Command Types
PowerShell allows you to combine various command types—such as cmdlets, function calls, and scripts—using semicolons. For example, combining several cmdlets can be done as follows:
Get-ChildItem; Clear-Host; Write-Host "Done listing files."
Here, `Get-ChildItem` retrieves the directory contents, `Clear-Host` removes the current output from the console, and `Write-Host` displays a message. Each command is executed in sequence, contributing to a fluid work process.
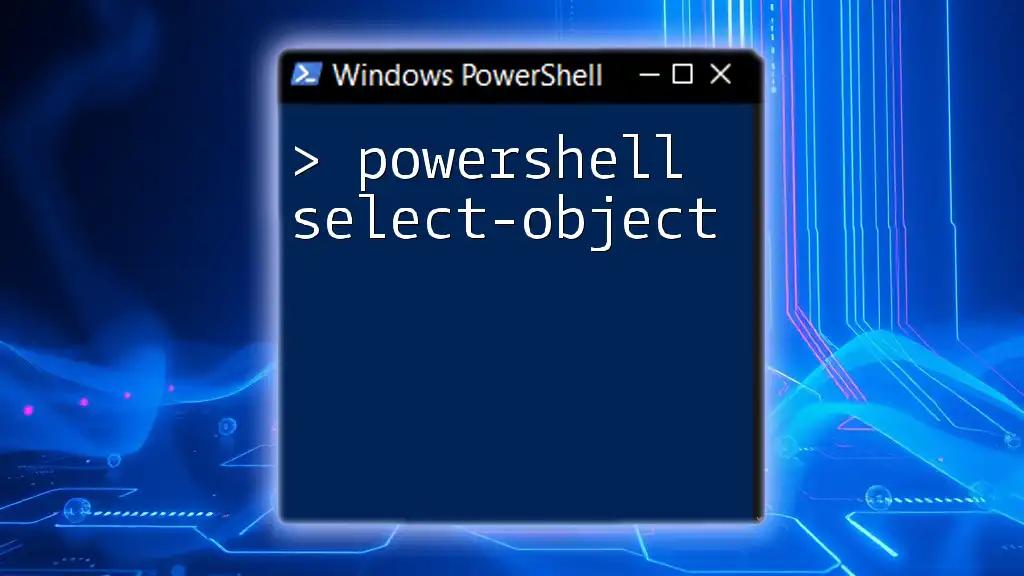
Best Practices for Using Semicolons
Readability and Maintainability
While it’s tempting to put many commands on a single line using semicolons, doing so can hinder readability and maintainability. A good practice is to keep commands clear and concise. Consider the following example:
Good Practice:
Get-Process;
Get-Service;
Get-EventLog -LogName Application
Poor Practice:
Get-Process;Get-Service;Get-EventLog -LogName Application;Write-Host "All command executed"
The second example, while functional, lacks clarity when compared to the first. Readable code is easier to debug and maintain in the long run, making it essential to structure PowerShell commands thoughtfully.
Example of Good vs. Poor Semicolon Use
Continuing from the earlier points, emphasizing readability shows how cohesive coding can vastly improve your scripts’ usability. When written clearly, your code communicates intent better, helping you or others understand its purpose upon revisiting it.
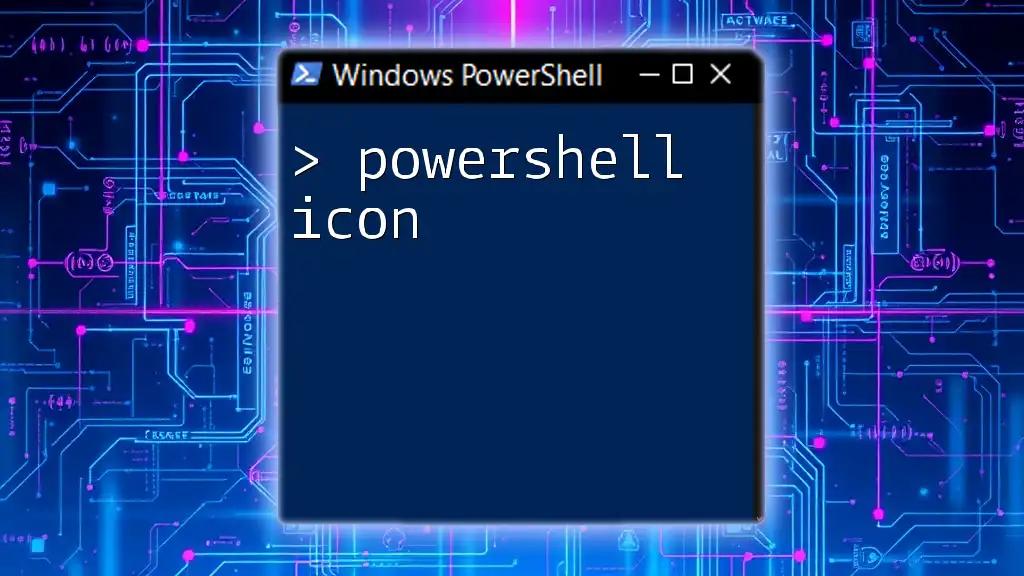
Error Handling with Semicolons
Utilizing Try-Catch Blocks with Semicolons
Incorporating error handling into semicolon-separated commands strengthens your scripts, ensuring that errors are gracefully managed. Using a `Try-Catch` block, you can effectively catch any exceptions that occur during execution. For example:
Try {
Get-Process; Get-Service; Get-EventLog
} Catch {
Write-Host "An error occurred: $_"
}
In this script, if any of the commands fail, the catch block will execute, displaying an error message. This practice reinforces the reliability of your scripts, especially for production environments.
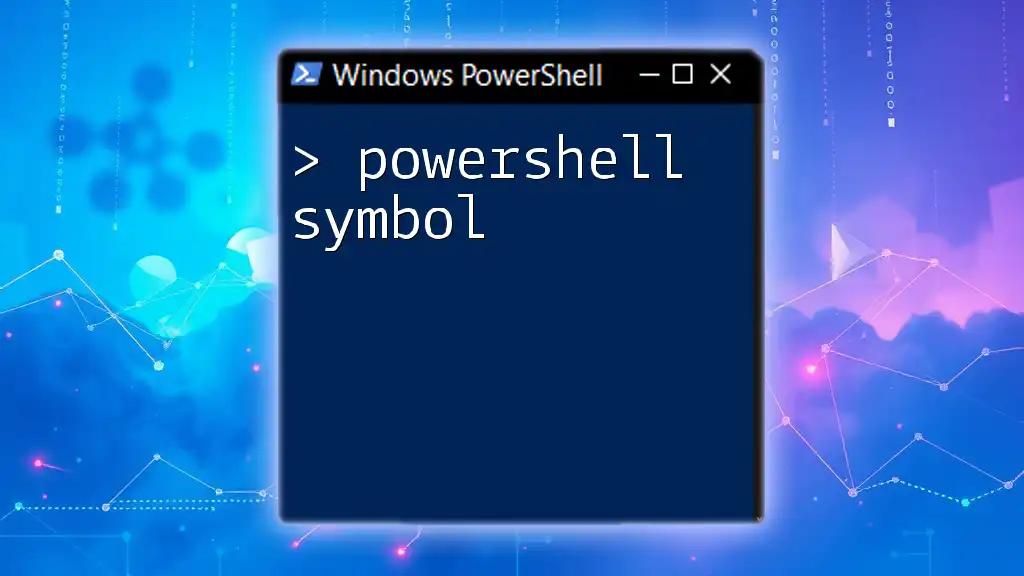
Performance Considerations
Efficiency of Command Execution
While semicolons streamline commands, it’s crucial to consider potential performance impacts. Executing numerous commands on one line can lead to chunked execution instead of concurrent processing. PowerShell often processes commands sequentially, which can result in slower execution times if not managed properly.
Example of Performance Testing
To illustrate this point, consider running commands with and without semicolons:
# Using semicolons
Get-Process; Get-Service; Get-ChildItem
versus:
Start-Job -ScriptBlock {Get-Process}; Start-Job -ScriptBlock {Get-Service}; Start-Job -ScriptBlock {Get-ChildItem}
In this example, using the semicolon may execute in a linear fashion, while the `Start-Job` commands run concurrently, offering faster overall execution. This highlights the flexibility of PowerShell in handling command execution.
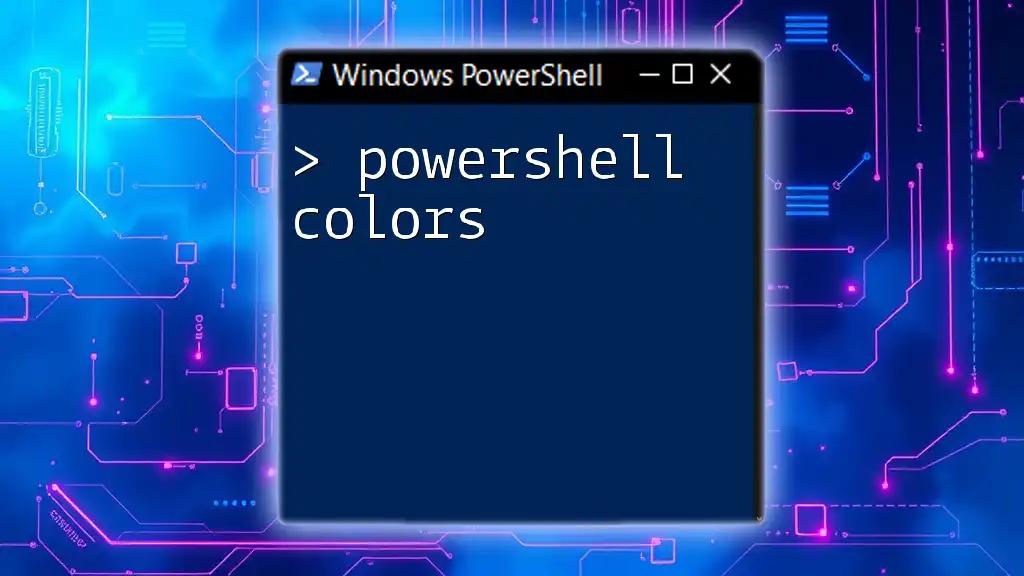
Alternatives to Semicolons
Using New Lines for Command Separation
Beyond semicolons, PowerShell allows for commands to be separated by new lines, treating each new line as a separate command. This approach often improves readability. For example:
Get-Process
Get-Service
Get-EventLog
This alternative can greatly enhance the clarity of your code without sacrificing functionality.
When to Use Each Method
When working in PowerShell, choose the method of separation based on the context. While semicolons are convenient for short command sequences, the new line method is typically preferable for longer scripts, fostering better organization and easier debugging.
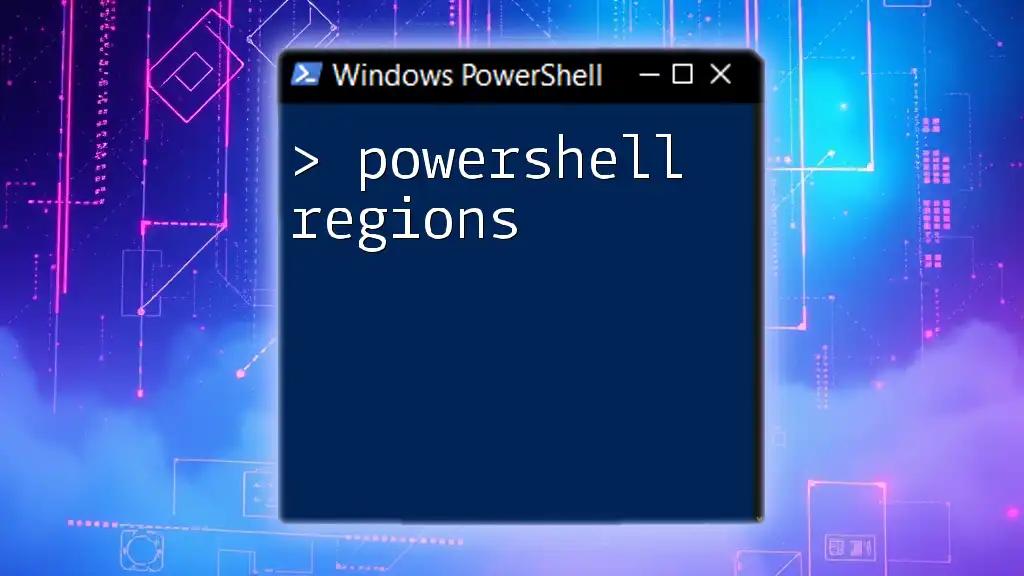
Conclusion
In summary, the PowerShell semicolon is a versatile command separator that plays a vital role in scripting and automation tasks. Understanding how to effectively use semicolons can enhance your scripting ability, streamline your workflow, and contribute to cleaner, more maintainable code. Embrace the practices discussed in this article as you continue to explore the vast capabilities of PowerShell. Happy scripting!
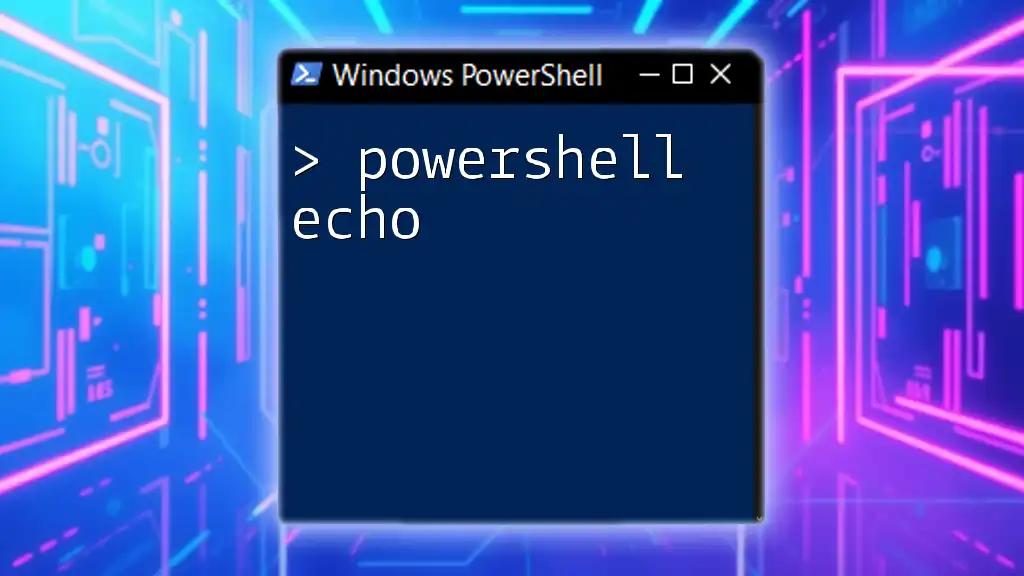
Additional Resources
For further learning, consider exploring the PowerShell documentation, engaging with community forums, and participating in online tutorials. This continued education can prepare you to tackle more advanced scripting techniques and improve your command line skills in the long run.