The PowerShell pipeline allows you to pass the output of one command directly into another command, facilitating efficient data processing and manipulation.
Here’s a simple example:
Get-Process | Where-Object { $_.CPU -gt 50 } | Select-Object -Property Name, CPU
In this example, we retrieve all processes, filter those using more than 50 CPU seconds, and then select their name and CPU usage for display.
Understanding the PowerShell Pipeline
What is the PowerShell Pipeline?
The PowerShell pipeline serves as a mechanism for passing output from one cmdlet to another, allowing for the chaining of commands. Unlike traditional command execution where output is displayed or stored as text, the pipeline in PowerShell passes objects directly. This object-based approach makes it possible to work with complex data types and leverage the rich set of properties and methods that objects offer.
The Anatomy of a Pipeline
At its core, a pipeline consists of three essential components:
- Commands: The cmdlets that perform actions.
- Objects: The data being manipulated, which are instances of .NET objects.
- Pipe (`|`): The operator that connects commands.
To visualize a basic pipeline, consider the following structure:
Command1 | Command2 | Command3
This diagram shows how output from `Command1` flows directly into `Command2`, and then into `Command3`, allowing for seamless data manipulation.
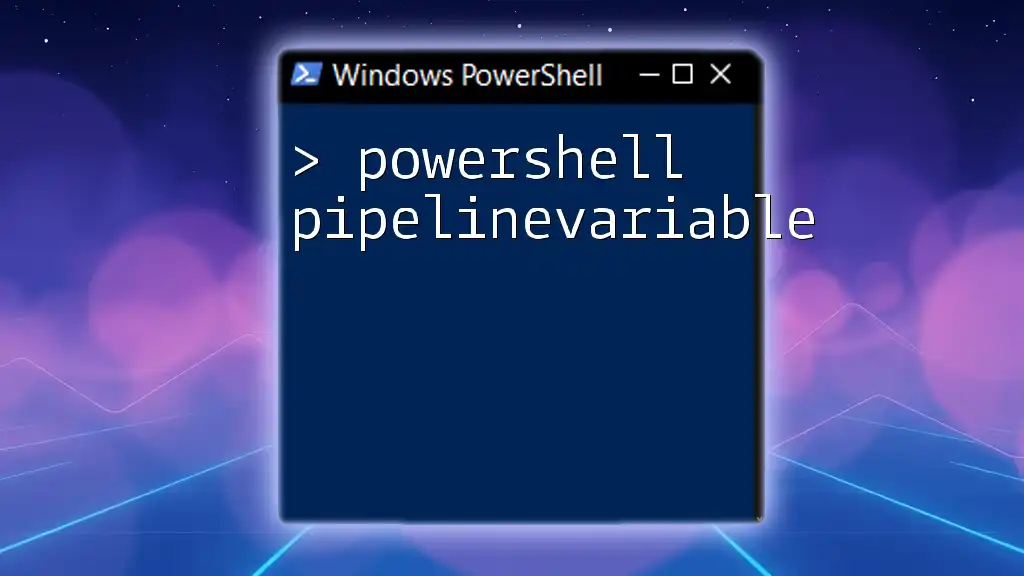
How the Pipeline Works
Data Flow in the Pipeline
Data moves through the PowerShell pipeline as objects rather than text output. This means that when you pass data from one cmdlet to another, you are not just dealing with raw strings; you are handling structured information that can be processed further. This feature makes the PowerShell pipeline a robust tool for automating tasks and managing system administration operations.
Common Cmdlets in the Pipeline
Several cmdlets frequently interact in the PowerShell pipeline. Some of these include:
- `Get-Command`: Retrieves all cmdlets, functions, and aliases.
- `Where-Object`: Filters objects based on specified conditions.
- `Select-Object`: Selects specified properties of an object or creates new computed properties.
For instance, when you want to filter and select specific processes running on your system, you might use a combination of cmdlets like this:
Get-Process | Where-Object { $_.CPU -gt 100 } | Select-Object Name, CPU
This command retrieves all processes, filters them to include only those using more than 100 CPU seconds, and then selects their names along with their CPU time.
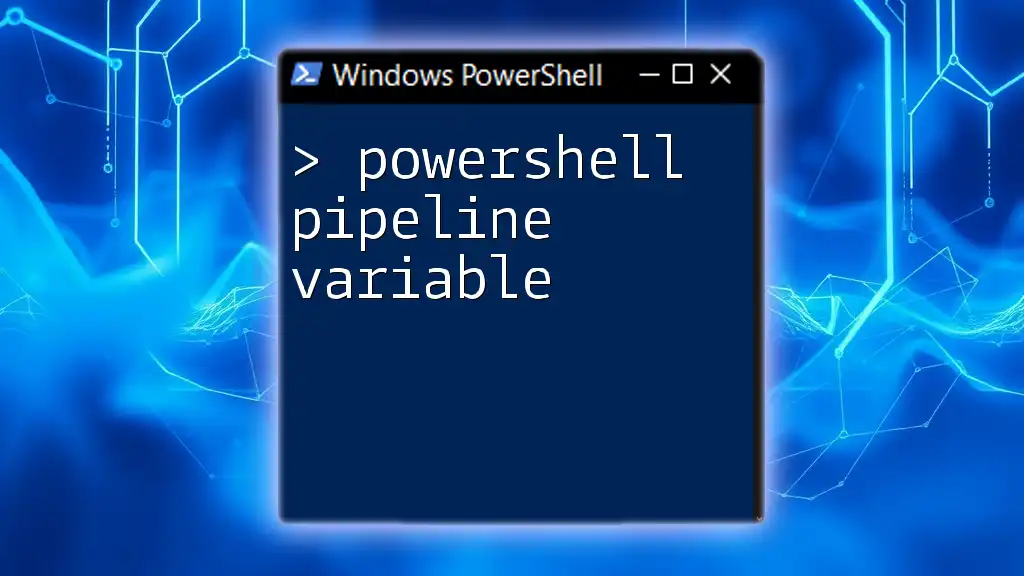
Creating Your First Pipeline
Basic Pipeline Syntax
Creating a basic pipeline is straightforward. The syntax revolves around placing commands and using the pipe operator to connect them. A simple example would look like this:
Get-Service | Where-Object Status -eq "Running"
In this example, the `Get-Service` cmdlet fetches all services, and the pipeline then filters it to show only those services that are currently running.
Example: Building a Simple Pipeline
Let’s walk through a simple pipeline step-by-step:
Get-Service | Where-Object Status -eq "Running" | Sort-Object DisplayName
- Get-Service: This cmdlet gathers all services on the system.
- Where-Object: Filters the results to show only services that are "Running."
- Sort-Object: Finally, it sorts those running services by their display names.
This straightforward approach to data manipulation via the pipeline highlights the ease of use PowerShell provides.
The Importance of Parameter Binding
PowerShell employs an automatic parameter binding mechanism. This means that when you pass an object through the pipeline, PowerShell intelligently binds the properties of that object to the parameters of the receiving cmdlet.
Take the following example:
Get-ChildItem | Measure-Object -Property Length -Sum
In this case, `Get-ChildItem` retrieves a list of files and directories, which is then piped to `Measure-Object` to compute the total size (in bytes) of all items by summing their lengths.
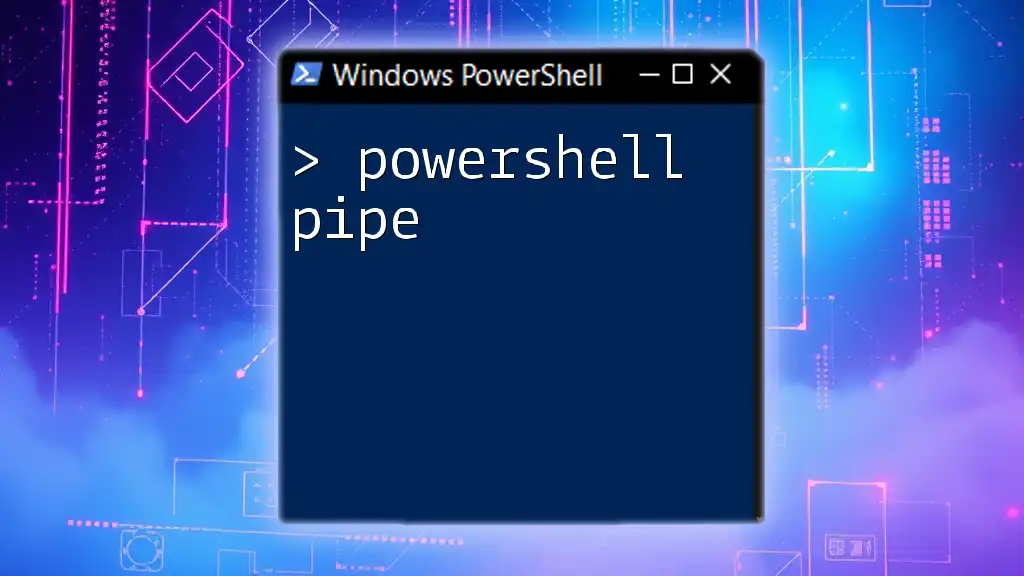
Advanced Pipeline Techniques
Using `ForEach-Object` in Pipelines
The `ForEach-Object` cmdlet is a powerful tool that can be utilized within a pipeline to perform operations on each object passed through.
For example:
Get-ChildItem | ForEach-Object { $_.Name }
This command lists the names of all items returned by `Get-ChildItem`, allowing for more granular control over the output.
Filtering and Sorting Output
Effective filtering and sorting are crucial for managing large datasets. The `Where-Object` cmdlet enables you to specify conditions, while `Sort-Object` organizes the output based on selected properties.
Here’s a combined example:
Get-Process | Where-Object { $_.CPU -gt 10 } | Sort-Object Memory -Descending
This command fetches processes consuming more than 10 CPU seconds and sorts them in descending order based on memory usage.
Creating Custom Objects with `Select-Object`
Using `Select-Object`, you can create custom outputs that focus on particular properties or create computed properties. Consider the following:
Get-Process | Select-Object Name, @{Name='Id';Expression={$_.Id}}, @{Name='Memory';Expression={($_.PrivateMemorySize / 1MB)}}
In this code, we create a custom object displaying each process's name, ID, and memory usage (in megabytes), demonstrating how to extract specific data easily.
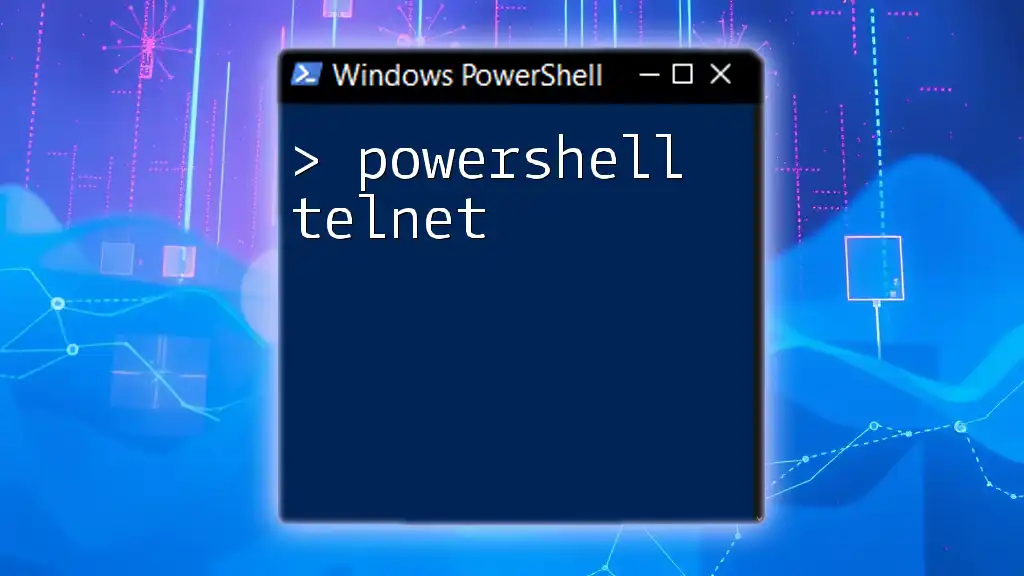
Best Practices for Using the PowerShell Pipeline
Keep Pipelines Concise
Maintaining a concise pipeline enhances readability. Avoid over-complicating pipelines with unnecessary commands. Simplicity makes it easier for anyone reviewing the script to understand its purpose.
Debugging Pipelines
Debugging can be challenging, especially when working with complex pipelines. Leverage cmdlets like `Get-Member` to examine the structure and properties of objects as they pass through the pipeline. For instance:
Get-Service | Get-Member
This command provides insight into the properties and methods available on the objects output by `Get-Service`.
Performance Optimization
Performance can be impacted by the complexity of your pipelines. To optimize, focus on minimizing the number of objects being passed through and avoid executing unnecessary cmdlets.
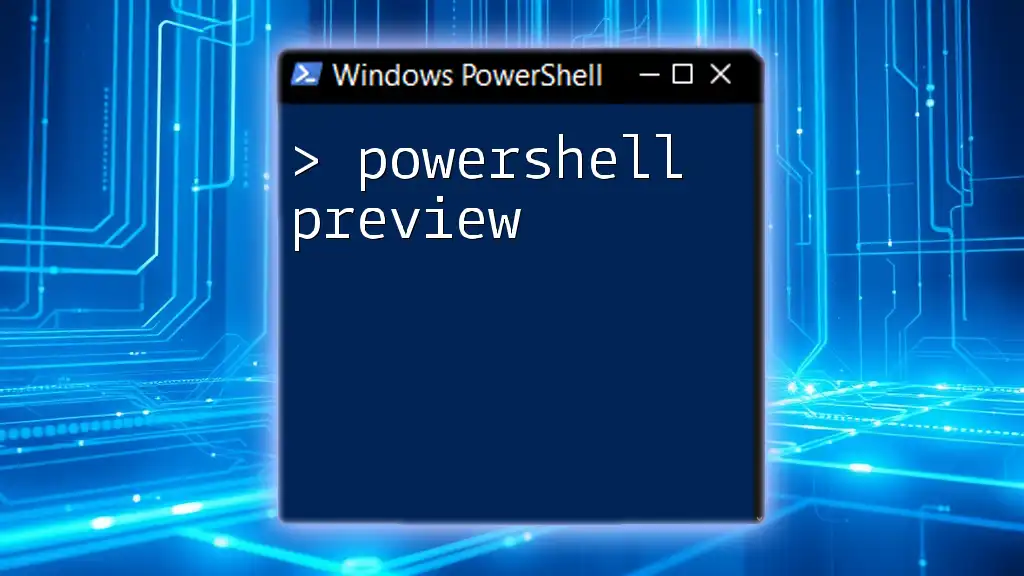
Conclusion
The PowerShell pipeline is a powerful feature that allows for seamless data manipulation and automation. By chaining cmdlets together, you can create complex workflows while maintaining clarity and readability. As you practice and experiment with pipelines, you'll discover their versatility and potential to streamline tedious tasks.
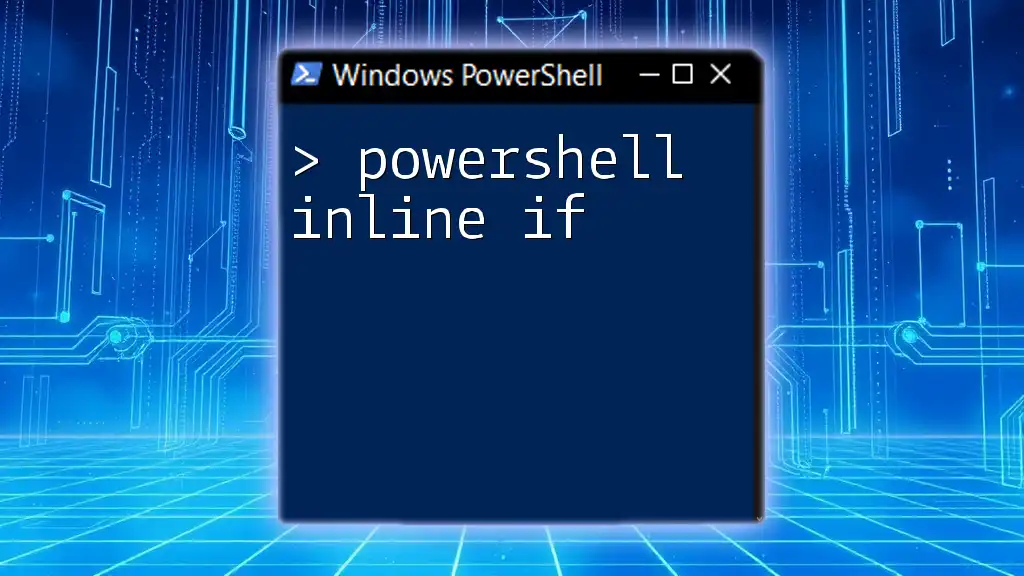
Additional Resources
For further learning and explorations into the world of PowerShell, refer to the official PowerShell documentation and consider exploring recommended books, courses, and community forums for discussions and support.
Call to Action
We invite you to share your experiences with PowerShell pipelines. What challenges have you faced, and what successes have you found? Stay tuned for more tips and tutorials as we continue to explore PowerShell's capabilities!