The PowerShell Profiler is a tool that helps users analyze the performance of their scripts by measuring execution time and resource usage of commands.
Measure-Command { Get-Process | Where-Object { $_.CPU -gt 0 } }
Understanding the Need for Profiling
Why Profile PowerShell Scripts?
Profiling is a crucial practice for any developer working with PowerShell. As scripts evolve in complexity and length, performance issues may arise unnoticed. Inefficient scripts can lead to longer execution times and increased resource usage, which may hinder user experience and productivity. By understanding and implementing profiling, you can identify and rectify these issues before they significantly impact your workflows.
Key Benefits of Using a Profiler
Improved Execution Times: Profiling helps pinpoint areas of the script that consume excessive time, allowing for optimizations that can drastically reduce execution durations.
Resource Optimization: With profiling, you can observe how your script utilizes system resources such as memory and CPU. This data enables you to make informed adjustments for resource efficiency.
Enhanced User Experience: Users expect quick and responsive scripts. Profiling ensures that your scripts perform optimally, meeting user needs effectively.
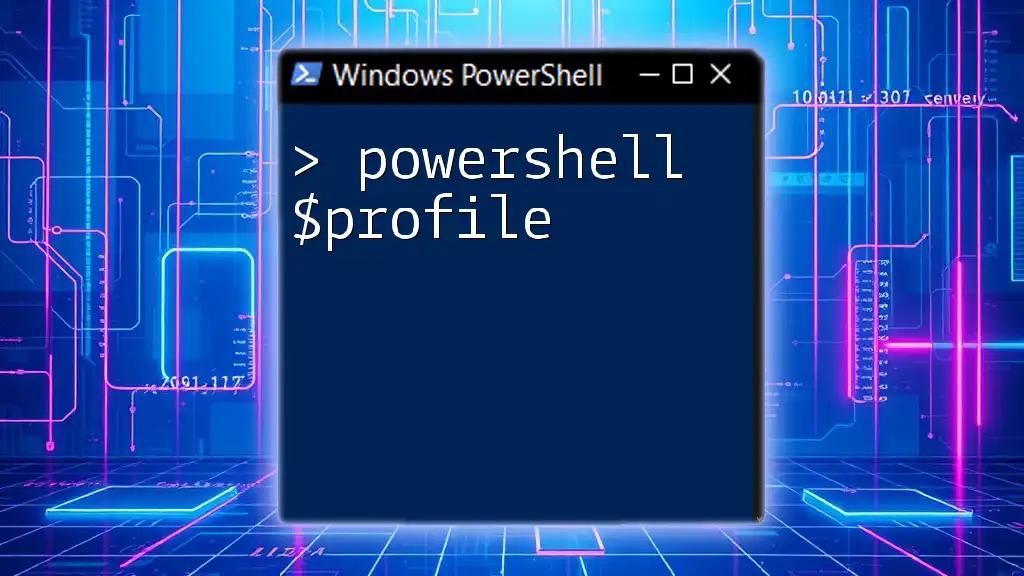
Getting Started with PowerShell Profiler
Prerequisites for Using the Profiler
Before diving into profiling, ensure that your PowerShell environment is configured correctly. You should have a version of PowerShell that supports profiling features; PowerShell 5.1 and later versions are ideal. Ensure that any necessary modules are installed, especially if you intend to use advanced profiling commands.
Basic Profiling Commands
PowerShell provides several commands that serve as a foundation for profiling your scripts. Key among them are:
- Measure-Command: This cmdlet allows you to measure the time it takes to run a block of PowerShell commands.
- Start-Transcript: This command is essential for logging output from your scripts, creating a record of all executed commands.
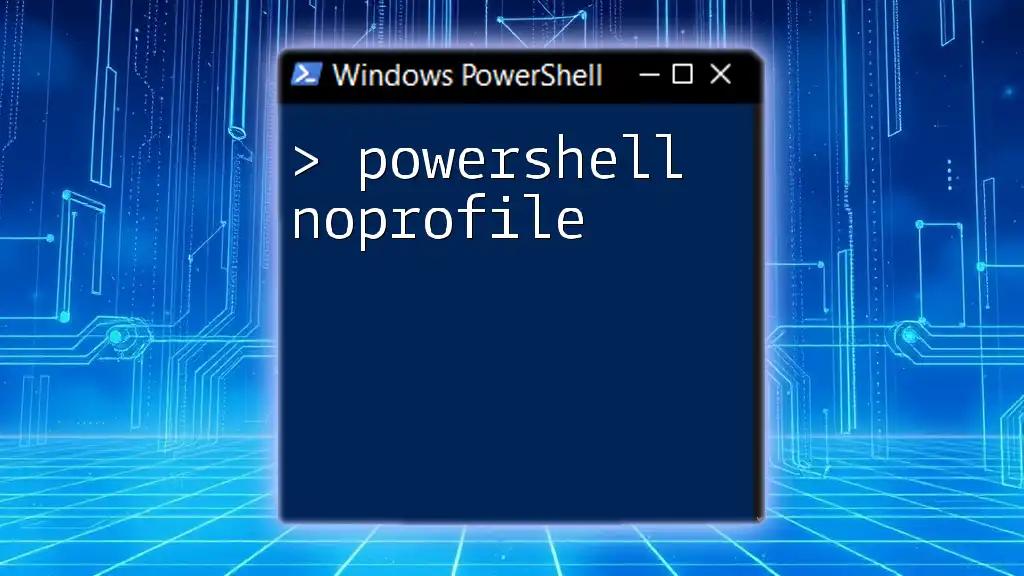
Detailed Analysis of PowerShell Profiler
Using Measure-Command
The `Measure-Command` cmdlet is straightforward and powerful. It essentially wraps around the commands you want to measure, providing the execution time for those commands. This allows for quick benchmarks to see how long particular operations take.
Example Code Snippet:
$time = Measure-Command {
# Your script block goes here
Get-Process
}
Write-Output "Execution Time: $($time.TotalMilliseconds) ms"
In this example, you can see just how easy it is to measure the time taken by the `Get-Process` command. Such quick evaluations can help you determine if further optimization is required.
Using Start-Transcript for Logging
`Start-Transcript` is a powerful command that captures all output from a PowerShell session. It is particularly useful for logging purposes, as it captures both the commands that are being executed and their output.
Example Code Snippet:
Start-Transcript -Path "C:\Logs\PowerShellTranscripts.txt"
# Your script content
Stop-Transcript
Running this command starts the logging process. Once your commands are executed, especially in a complex script, you can stop the transcript. The resulting log file serves as a vital debugging tool and a record for performance profiling.
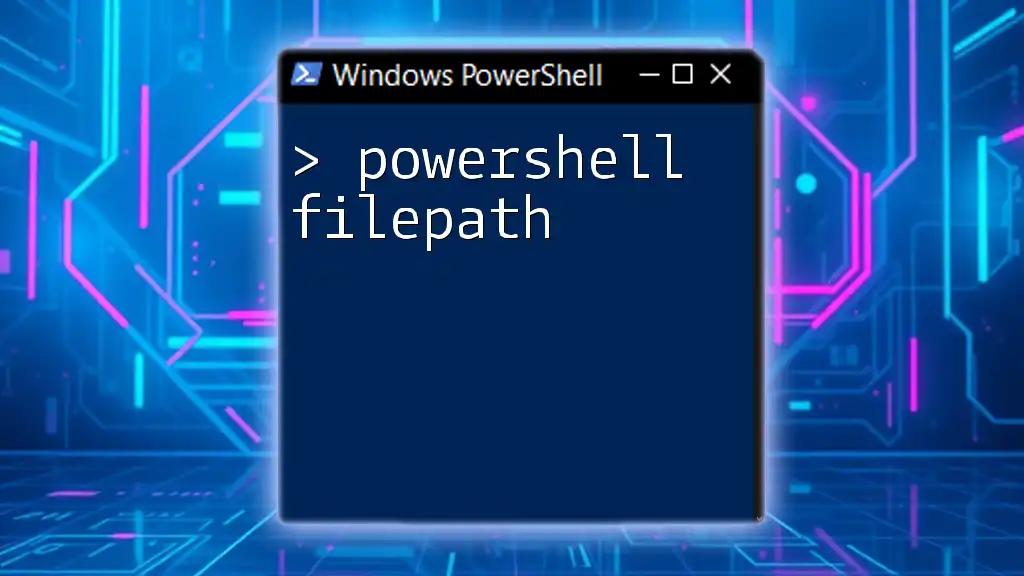
Advanced Profiling Techniques
Profiling with Trace-Command
For deeper insights, you may use `Trace-Command`. This cmdlet allows a user to trace specific actions or commands executed during the session. You can tailor this to monitor specific cmdlets or functions, providing detailed diagnostic information.
Code Example for Trace-Command:
Trace-Command -Name "CommandName" -Expression { Get-Service } -PSHost
This command traces the execution of `Get-Service`, providing visibility into its internal processing. Such traces can help identify unexpected behavior and performance bottlenecks.
Utilizing Performance Counters
Windows Performance Counters are an advanced way to monitor system performance. PowerShell provides access to these counters, enabling you to evaluate several aspects of system performance in real time.
Example Code Snippet:
Get-Counter -Counter "\Processor(_Total)\% Processor Time" -Continuous
This code allows continuous monitoring of CPU usage, giving you insights into how script execution may impact overall system performance. It’s especially useful when integrating scripts into larger systems or services.
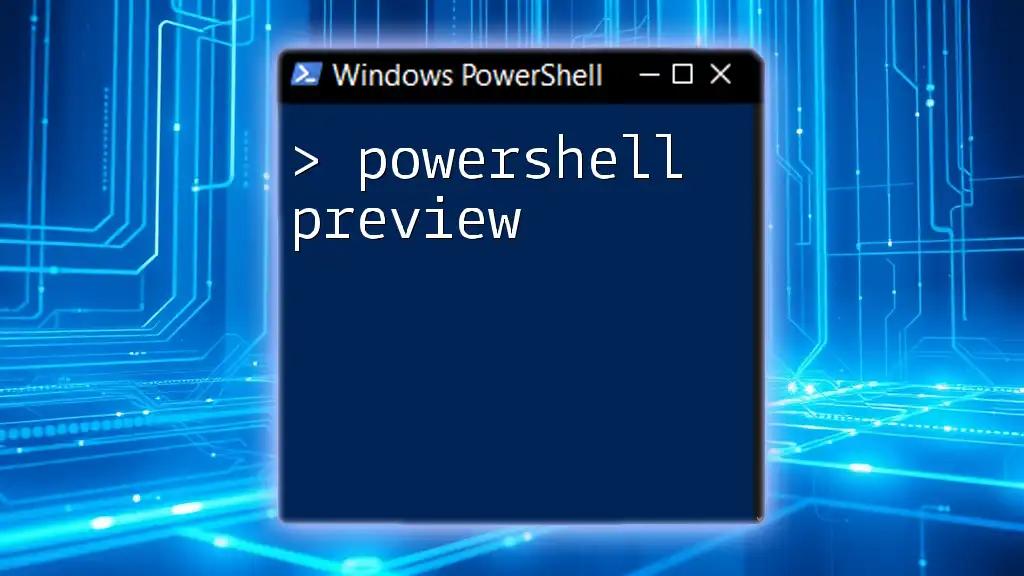
Analyzing Performance Data
Interpreting Profiler Outputs
Interpreting the data provided by profiling tools can be challenging. However, leverage existing visualization tools to help make sense of the data. Understanding these outputs enables you to spot trends, identify anomalies, and make data-driven adjustments to your scripts.
Identifying Performance Bottlenecks
Common signs of performance bottlenecks include high CPU usage, prolonged execution times, and resource contention errors. Identifying these issues through profiling output allows you to focus on optimizing specific parts of your PowerShell scripts.
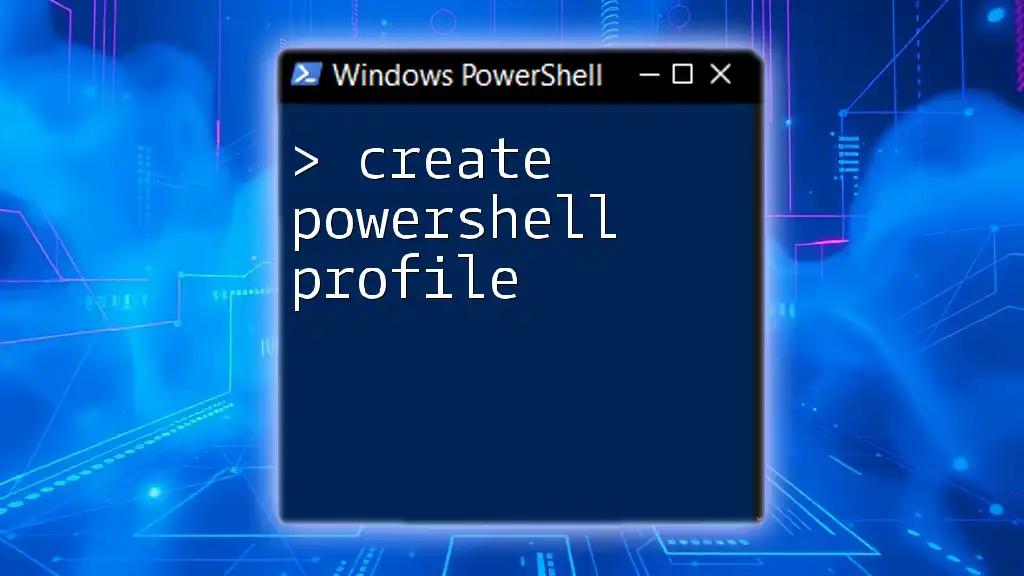
Best Practices for Efficient PowerShell Scripting
Writing Efficient PowerShell Scripts
Efficient scripting involves not just reducing execution time but also writing maintainable and clean code. Consider the following tips:
- Use pipeline operations: They often perform better than iterative loops.
- Avoid unnecessary cmdlets: If your script executes commands multiple times that yield the same results, cache the result for reuse.
- Implement error handling: Ensure your script handles errors gracefully to avoid performance dips during unexpected situations.
When to Use Profiling in Your Development Process
Incorporate profiling at multiple stages of your development process. Run profiling during the initial development phase to understand any potential issues, execute it again during major updates, and finally validate performance after significant changes. Ongoing performance assessments allow for continual improvements and optimizations.
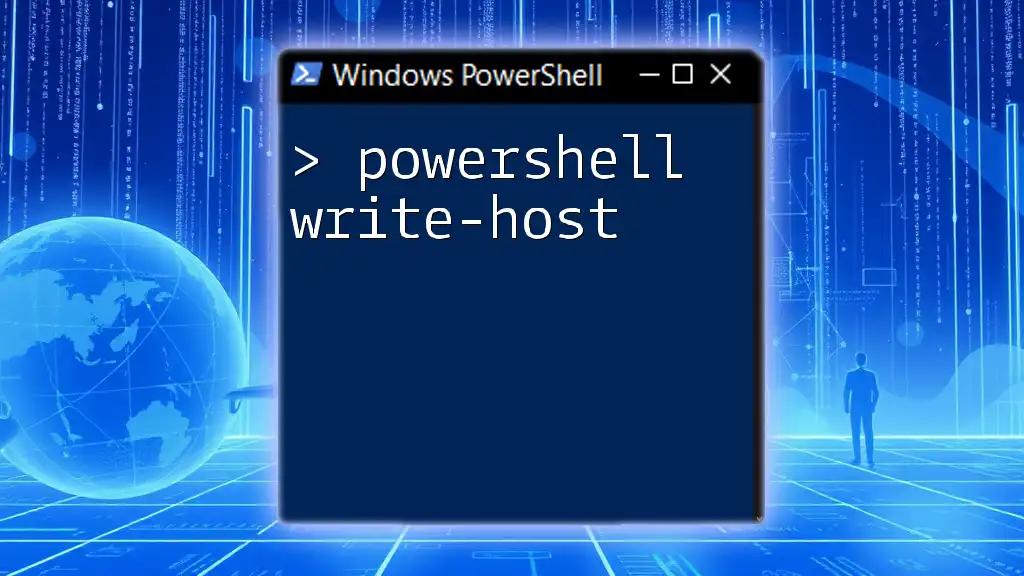
Conclusion
In summary, utilizing the PowerShell Profiler is essential for developing efficient PowerShell scripts. By understanding the tools and techniques for profiling, you can significantly optimize your script's performance, ensuring that your workflows remain effective and responsive.
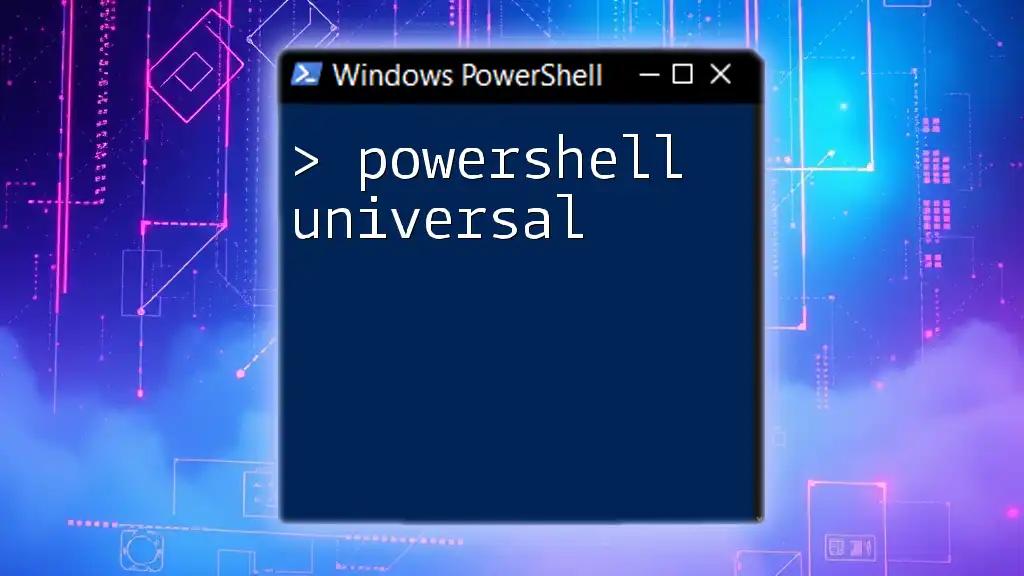
Resources and Further Reading
For those looking to expand their understanding, the official PowerShell documentation is a great starting point. Additionally, there are numerous books and online courses dedicated to PowerShell scripting and performance optimization. Engaging with communities in forums can also provide real-world examples and tips to enhance your skills.