A PowerShell `PSObject` is a versatile data structure that allows you to create custom objects with properties and methods, making it ideal for organizing and manipulating data in scripts.
# Creating a PSObject with custom properties
$myObject = New-Object PSObject -Property @{
Name = 'John Doe'
Age = 30
Email = 'john.doe@example.com'
}
# Displaying the properties of the PSObject
$myObject | Format-List
Understanding PSObject
What is a PSObject?
A PSObject (PowerShell Object) is a flexible data structure in PowerShell that allows you to hold various types of information. It plays a pivotal role in scripting and data manipulation, serving as a tool for handling dynamic data. Unlike traditional objects in programming, PSObjects can be created on-the-fly with custom properties and methods, making them ideal for tasks such as storing configuration settings or managing script output.
Key Features of PSObject
PSObjects come with several notable features:
- Dynamic Properties and Methods: You can define custom properties and methods, enabling you to tailor the objects to suit your needs.
- Multi-Type Storage: PSObjects can store different data types, including strings, integers, and even other objects, allowing for great versatility.
- Compatibility: PSObjects are compatible with other PowerShell objects, making it easier to integrate them into your scripts.
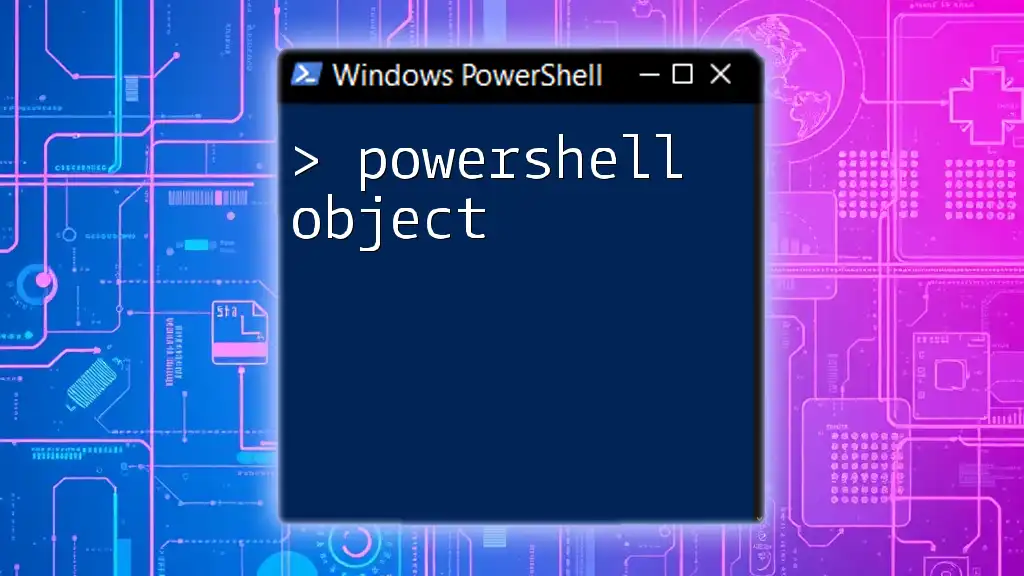
Creating a PSObject
Basic Syntax for Creating a PSObject
You can create a PSObject using the `New-Object` cmdlet. Here’s a simple example that defines a person with several properties:
$person = New-Object PSObject -property @{
Name = "John Doe"
Age = 30
Occupation = "Engineer"
}
In this snippet, a PSObject is created with three properties: Name, Age, and Occupation. This construction allows you to encapsulate related data into a single object.
Using the PSObject Constructor
Another efficient way to create a PSObject is by utilizing the PSCustomObject type. This approach is often preferred for its simplicity and enhanced performance. Here’s how you can do it:
$car = [PSCustomObject]@{
Make = "Toyota"
Model = "Camry"
Year = 2021
}
Using PSCustomObject builds a more efficient and memory-friendly object without unnecessary overhead. It’s a recommended best practice in modern PowerShell scripting.
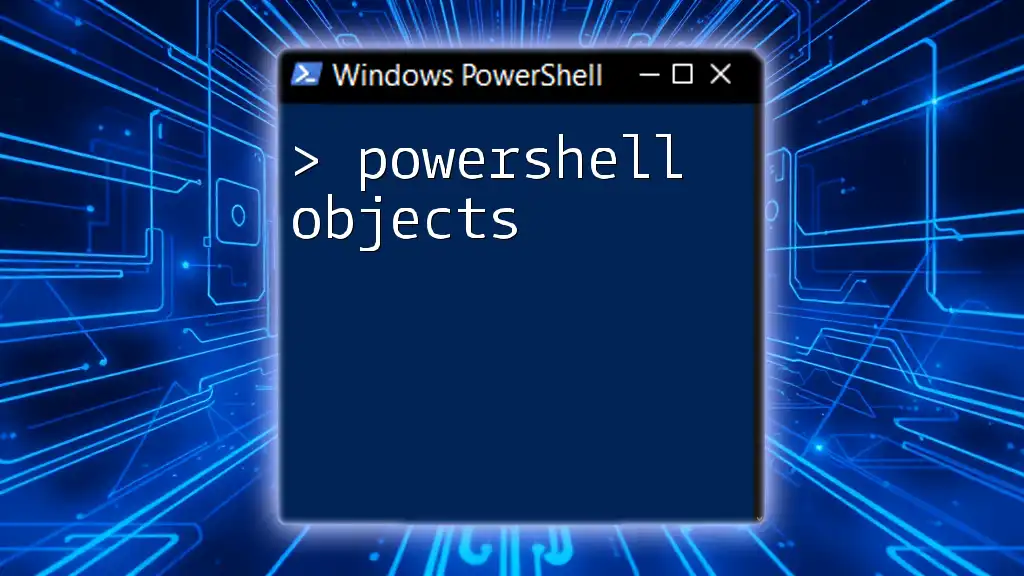
Accessing and Modifying PSObject Properties
Accessing Properties
Accessing properties of a PSObject is straightforward. You can simply use the dot notation:
$person.Name
$person.Age
The above commands will retrieve the values of the Name and Age properties, respectively.
Modifying Existing Properties
PowerShell allows for easy modification of properties. If you need to update the Age property, you can write:
$person.Age = 31
This line updates the Age of the $person object from 30 to 31. It showcases how flexible PSObjects can be.
Adding New Properties
Adding new properties dynamically is one of the strongest features of PSObjects. Here's how to add a Country property:
$person.Country = "USA"
With this simple line, a new Country property is created, making it easier to store additional relevant information without redefining the entire object.
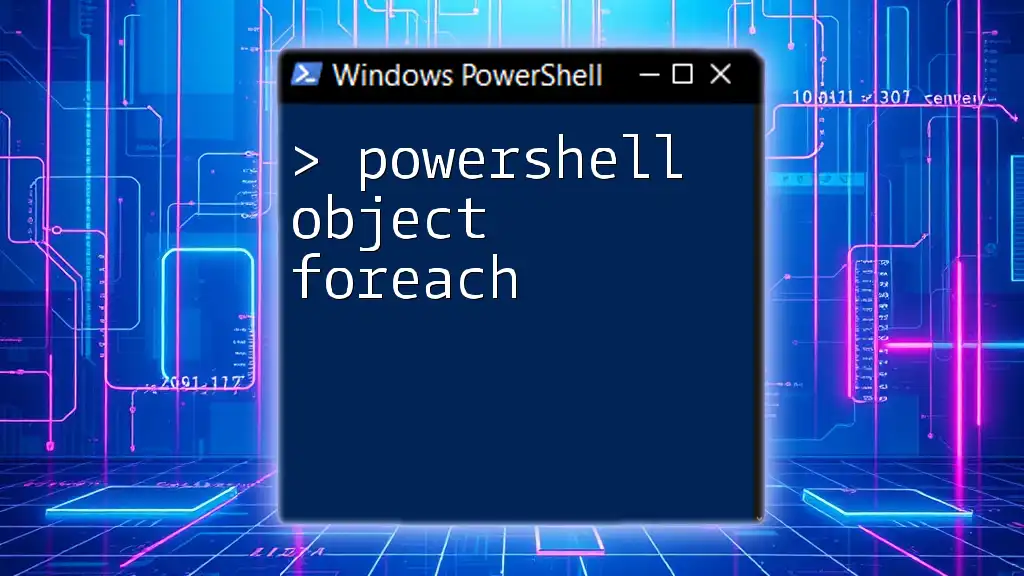
Working with Collections of PSObjects
Creating an Array of PSObjects
In PowerShell, you can create collections of PSObjects. Arrays of PSObjects can be incredibly useful, especially when dealing with multiple data entries. Here's an example:
$employees = @(
[PSCustomObject]@{ Name = "Alice"; Age = 28; Role = "Manager" },
[PSCustomObject]@{ Name = "Bob"; Age = 24; Role = "Developer" }
)
This code creates an array containing two employee objects, allowing for scalable data handling.
Iterating Over PSObjects
You can iterate through an array of PSObjects using the `ForEach-Object` cmdlet. For example:
$employees | ForEach-Object {
"$($_.Name) is a $($_.Role)"
}
This command will output lines indicating each employee's name and role, demonstrating how easy it is to work with collections.
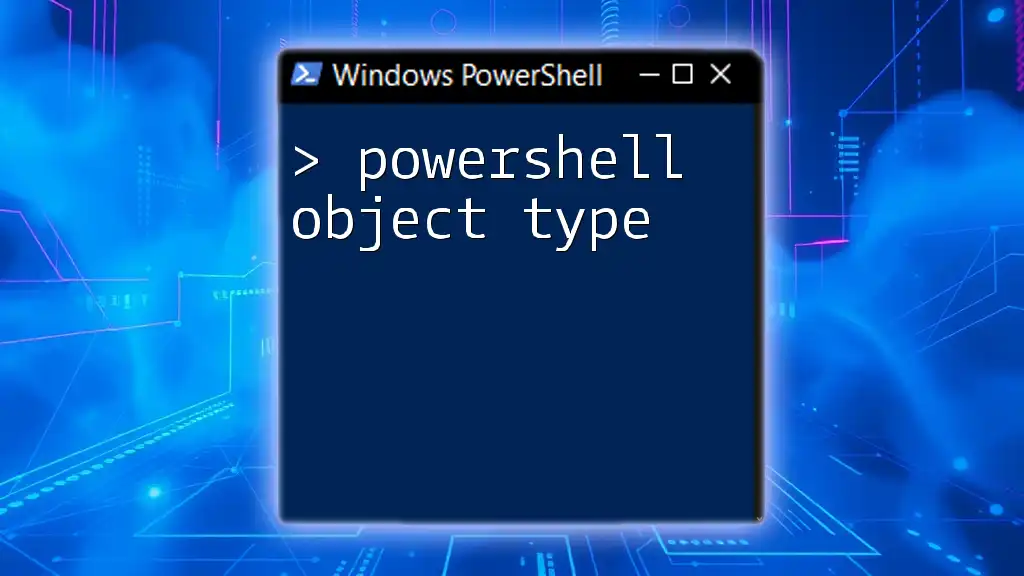
Common Use Cases for PSObjects
Data Representation
PSObjects are great for representing structured data. For example, if you were managing configurations, PSObjects can encapsulate all necessary parameters and settings.
Output Formatting
You can also use PSObjects to enhance output formatting in your scripts. Consider the following example:
Get-Process | Select-Object Name, ID, @{Name="Memory (MB)"; Expression={[math]::round($_.WorkingSet / 1MB, 2)}}
In this code snippet, a PSObject is used to format process information, showing the memory usage in a clear and concise manner.
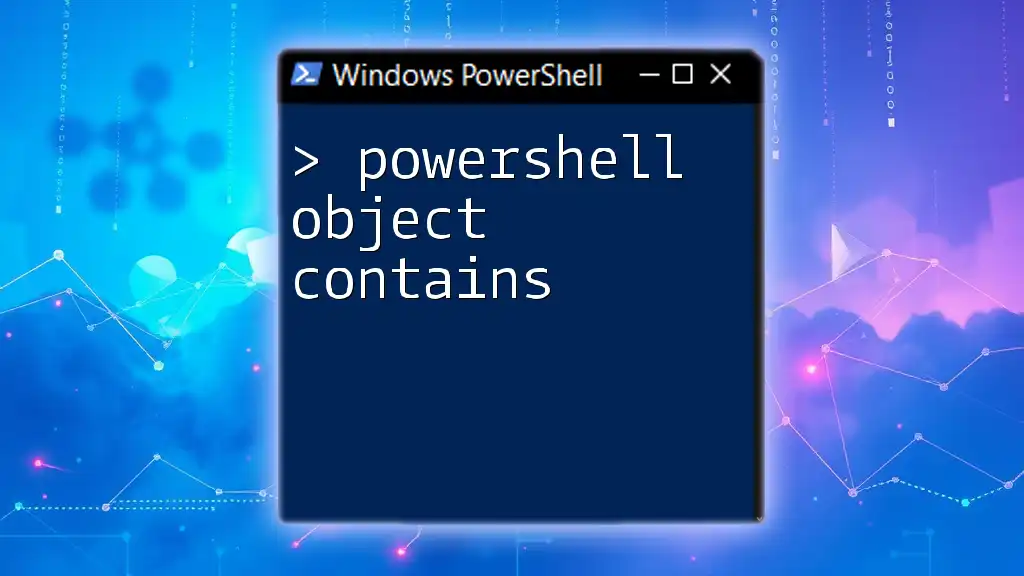
Best Practices for Using PSObjects
Naming Conventions
When creating PSObjects, consistent and descriptive naming conventions for properties are crucial. Meaningful names will improve code readability and maintainability.
Performance Considerations
While PSObjects are immensely powerful, consider their performance in large datasets. Utilizing PSCustomObject over New-Object generally results in better performance, especially when dealing with numerous instances.
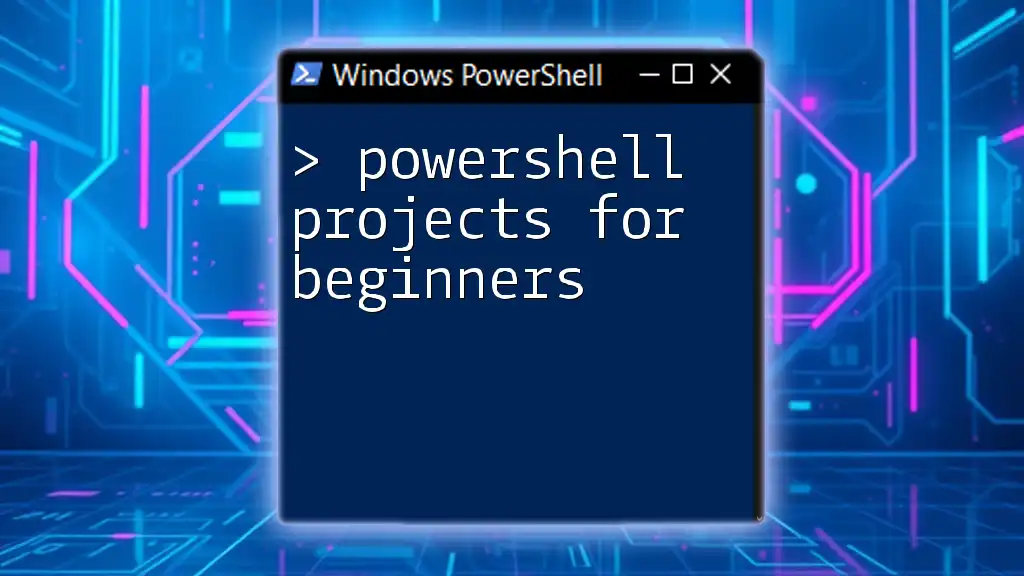
Troubleshooting Common Issues
Common Errors with PSObjects
When working with PSObjects, you may encounter some common issues:
-
Accessing Non-Existent Properties: Trying to retrieve a property that hasn’t been defined will result in an error. Always check property names for accuracy.
-
Type Mismatches: Since PSObjects can store diverse data types, ensure that you're using the right types, especially when making calculations.
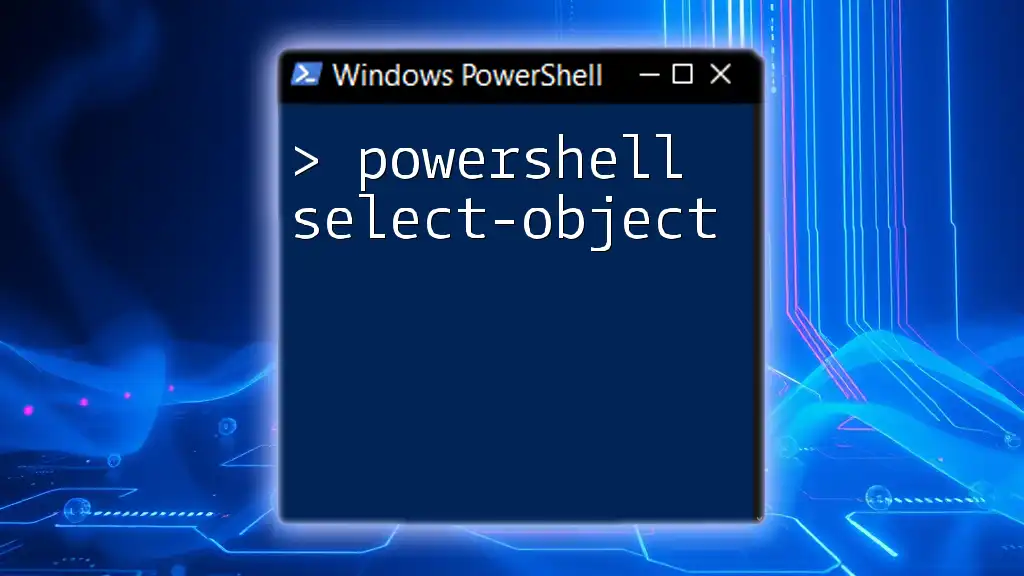
Conclusion
In summary, PowerShell PSObject is an essential concept that allows for dynamic manipulation of data in scripts. By understanding how to create, access, and modify PSObjects, as well as their practical applications, you can enhance your PowerShell scripting skills significantly. Practice creating and using PSObjects in your own scripts to see their versatility and power in action.
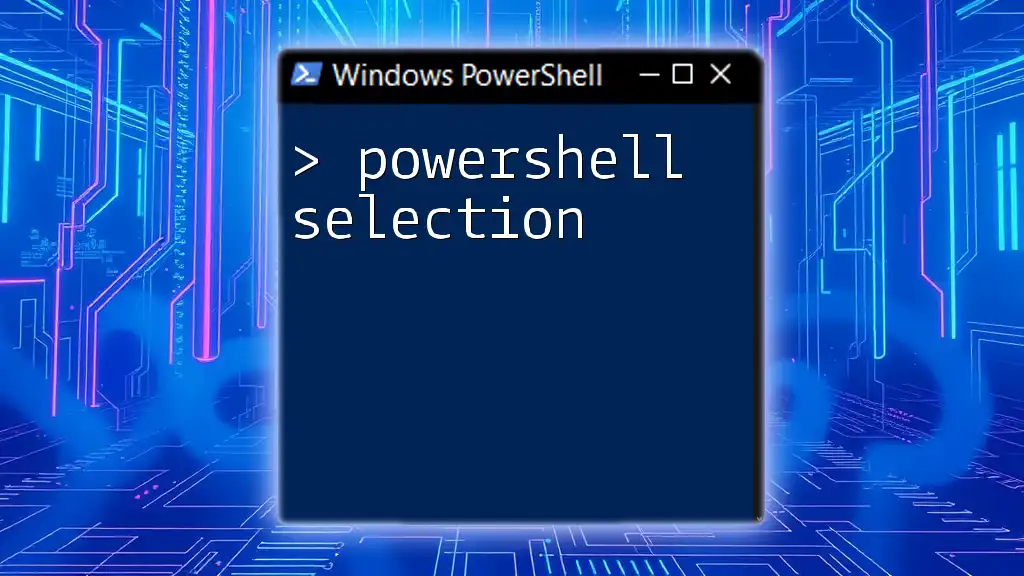
Additional Resources
To further enhance your understanding of PSObjects, explore the following resources:
- Useful commands and cmdlets related to PSObjects.
- Tutorials on PowerShell scripting best practices.
- Community forums and official documentation for additional learning.
By mastering PSObjects, you will equip yourself with a powerful tool that enhances your ability to manage and automate tasks within PowerShell effectively.