The `PSCustomObject` in PowerShell is a flexible way to create custom objects that can hold multiple properties, allowing users to organize and manage data efficiently.
Here’s a code snippet that demonstrates how to create a `PSCustomObject`:
# Creating a PSCustomObject with two properties: Name and Age
$person = [PSCustomObject]@{
Name = 'John Doe'
Age = 30
}
Write-Host $person
Introduction to PSCustomObject
A PSCustomObject is a powerful and versatile feature in PowerShell that allows you to create structured data in a user-friendly manner. It acts as a custom object, allowing you to define properties and their values in a way that is easy to read, modify, and use within your scripts. PSCustomObjects are crucial in enhancing the organization and functionality of your scripts, particularly when dealing with complex data.
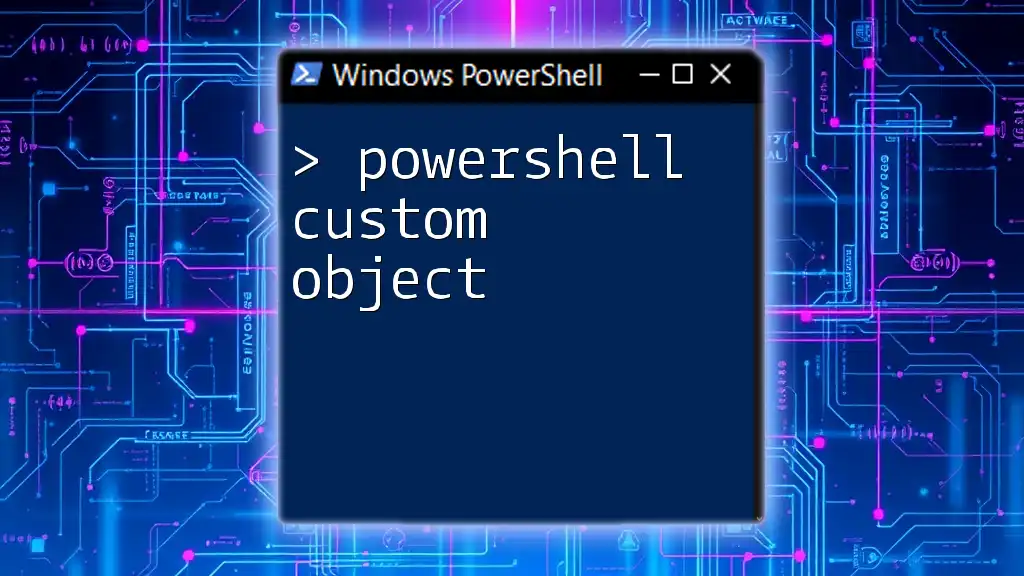
Benefits of Using PSCustomObject
Enhanced Data Structuring
The primary benefit of utilizing a PSCustomObject is its ability to provide structured data. With PSCustomObjects, you can easily create key-value pairs, which helps you to keep your data organized. This structured approach can be immensely beneficial when you have multiple attributes to manage, facilitating data retrieval and manipulation.
Improved Readability and Maintainability
PSCustomObjects enhance the readability of your scripts. When you use custom objects, your intentions are clearer, making it easier for others (or even yourself) to understand the script’s purpose in the future. For example, comparing a statement that uses a PSCustomObject to one that uses arrays or hashtables can clearly illustrate this point:
# Using PSCustomObject
$person = [PSCustomObject]@{
Name = "Alice"
Age = 30
}
# Using Hashtable
$person = @{
Name = "Alice"
Age = 30
}
The PSCustomObject example makes the structure of the data much clearer.
Flexibility in Script Development
Flexibility is another significant advantage of PSCustomObjects. They can be easily modified, enabling quick changes or additions to your data structures without much hassle. This is especially useful in larger scripts and modules where evolving requirements may necessitate updates to your data format.
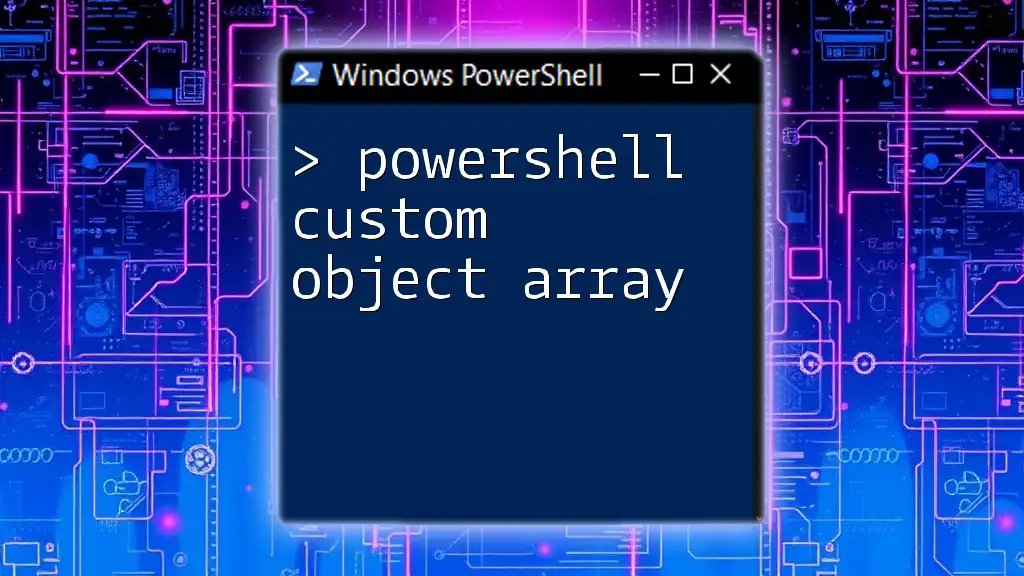
Creating a PSCustomObject
The New-Object Command
Creating a PSCustomObject can be done using the `New-Object` cmdlet. This method provides a straightforward way to instantiate custom objects while specifying properties and their values.
$myObject = New-Object PSObject -Property @{
Name = "John Doe"
Age = 30
Occupation = "Developer"
}
In this example, we created a new PSCustomObject named `$myObject` that contains three properties: `Name`, `Age`, and `Occupation`.
Using the [PSCustomObject] Type Accelerator
A more efficient way to create a PSCustomObject is by using the `[PSCustomObject]` type accelerator. This method is often preferred for its simplicity and directness.
$myCustomObject = [PSCustomObject]@{
Name = "Jane Smith"
Age = 28
Occupation = "Designer"
}
By using the type accelerator, you streamline the object initialization process, making your scripts cleaner and easier to maintain.
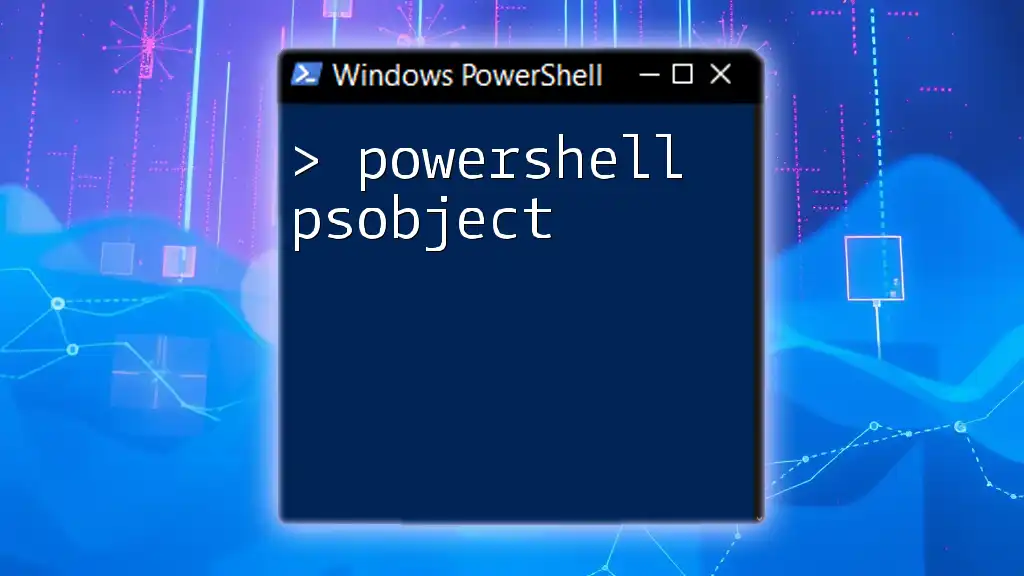
Accessing and Modifying PSCustomObject Properties
Accessing Properties
Once you’ve created a PSCustomObject, accessing its properties is straightforward. You can simply call the property by its name.
$myCustomObject.Name # Outputs "Jane Smith"
This intuitive access method makes working with PSCustomObjects significantly easier compared to traditional data formats.
Modifying Properties
You are also able to modify existing properties or add new ones to a PSCustomObject. Here’s how you can do that:
$myCustomObject.Age = 29 # Modify existing property
$myCustomObject | Add-Member -MemberType NoteProperty -Name Location -Value "New York" # Add new property
If you need to remove a property, you can do so as follows:
$myCustomObject.PSObject.Properties.Remove('Occupation') # Remove property
These operations illustrate the flexibility of PSCustomObjects, making it easy to adapt your data structures as needed.
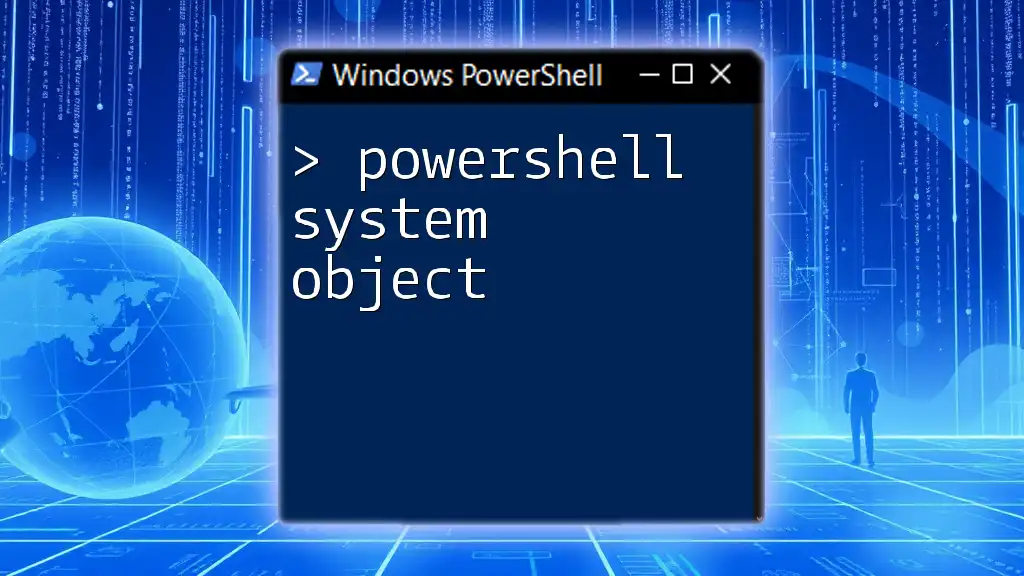
Advanced Usage Scenarios for PSCustomObject
Creating Collections of Custom Objects
You can create arrays of PSCustomObjects, allowing you to group related data together in a structured manner.
$people = @()
$people += [PSCustomObject]@{ Name = "Alice"; Age = 24 }
$people += [PSCustomObject]@{ Name = "Bob"; Age = 31 }
In this example, we have created an array, `$people`, that holds multiple custom objects. This is not only useful for organizing data but also for processing bulk entries.
Using PSCustomObject with Cmdlets
PSCustomObjects can be seamlessly used with PowerShell cmdlets, enhancing their capabilities and the flow of your scripts. For example, you can use `Sort-Object` or `Select-Object` for data manipulation.
$people | Sort-Object Age | Select-Object Name, Age # Sorting and selecting properties
This command sorts the people by age and then selects only their names and ages into the output, demonstrating the synergy between PSCustomObjects and cmdlets.
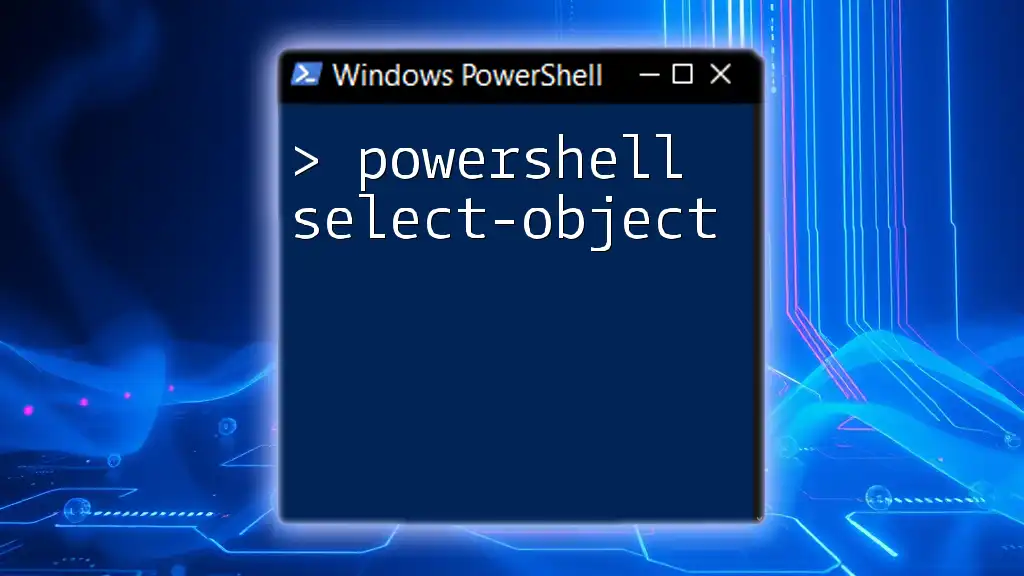
Best Practices When Working with PSCustomObject
Naming Conventions
When working with PSCustomObjects, it's important to maintain consistent naming conventions. Choose descriptive names for your properties that clearly represent the data they hold, making your script more readable and maintainable.
Documentation and Commenting
Good scripting practice involves thorough commenting and documentation. Clearly explain the purpose of your PSCustomObjects, especially when dealing with complex scripts or multiple objects. This will aid future developers (or your future self) in understanding the script’s logic and data structure.
Avoiding Common Pitfalls
Be aware of common mistakes when working with PSCustomObjects. For example, avoid overwriting properties inadvertently or failing to check if properties exist before accessing them. This practice can save time and prevent errors in your scripts.
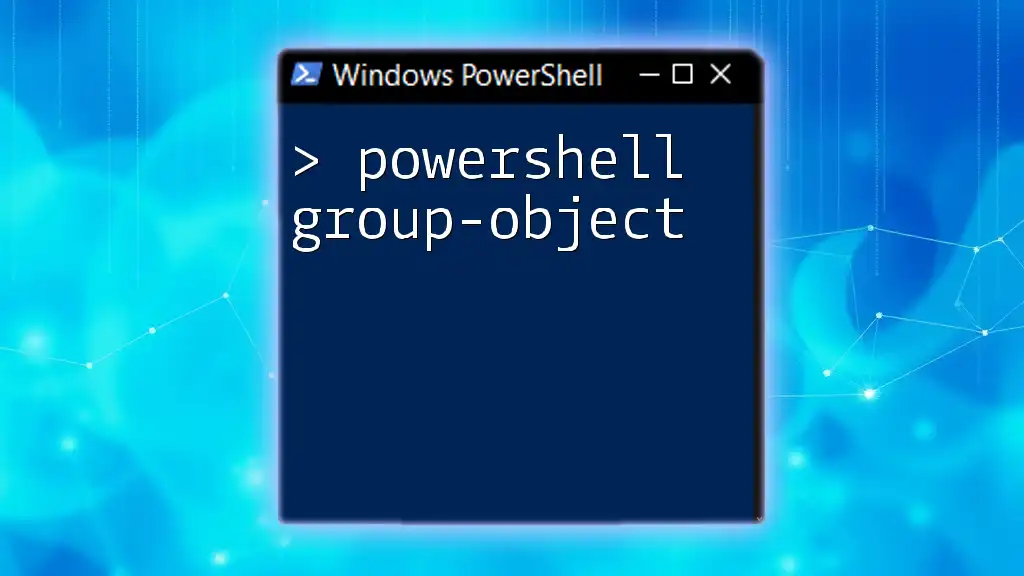
Conclusion
In summary, PSCustomObjects are an invaluable tool in PowerShell scripting, enhancing data structuring, improving readability and maintainability, and offering flexibility in script development. Practice creating and modifying PSCustomObjects to become proficient in their use. The possibilities they offer will empower you to write cleaner, more efficient PowerShell scripts.
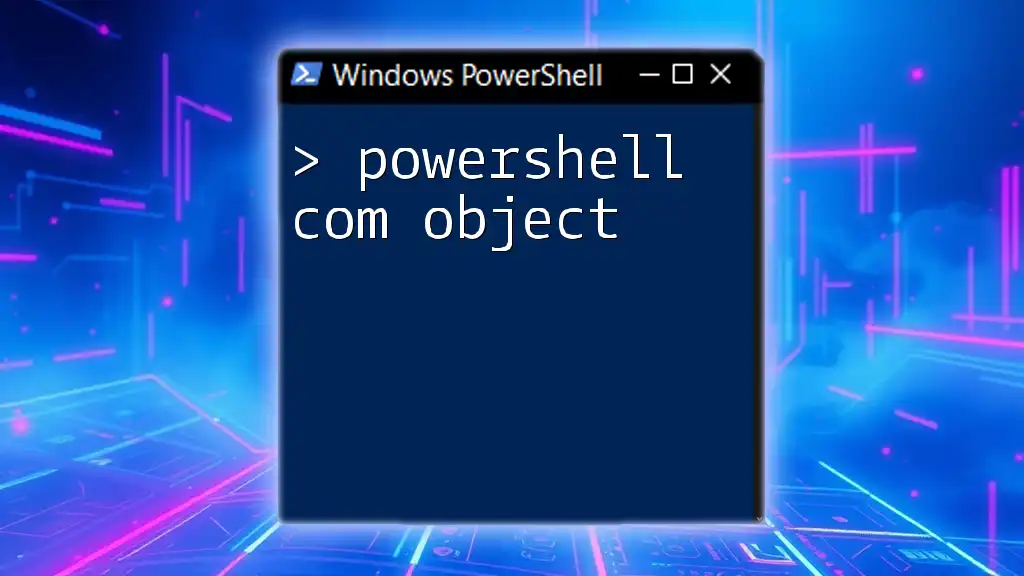
Additional Resources
To further your learning, consider exploring official PowerShell documentation, community tutorials, and online forums dedicated to PowerShell scripting and its capabilities. Engaging with the community can provide insight and practical knowledge for using PSCustomObject effectively.
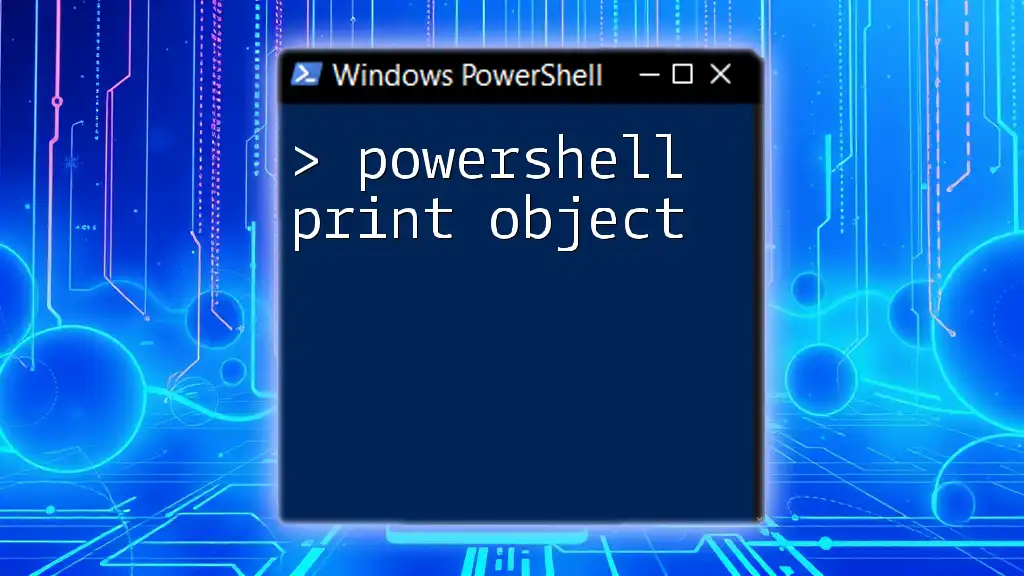
Call to Action
Stay tuned for more concise and actionable PowerShell tutorials designed to enhance your scripting skills! Sign up for our updates to continue your journey in mastering PowerShell commands.