The `Select-Object -First` cmdlet in PowerShell is used to retrieve a specified number of the first objects from a collection, making it ideal for quickly accessing the earliest entries in your data set.
Here’s a code snippet demonstrating its use:
Get-Process | Select-Object -First 5
Understanding the `-First` Parameter
The `-First` parameter of the `Select-Object` cmdlet is specifically designed to limit the number of objects returned from a collection. This is a powerful feature for quickly retrieving a specified number of items, especially when dealing with large datasets or command outputs.
When you want to focus on only the initial entries of a list or output, the `-First` parameter is invaluable. It allows you to streamline your results without the clutter of unnecessary data, commonly useful for initial debugging, reporting, or simply extracting notable entries.
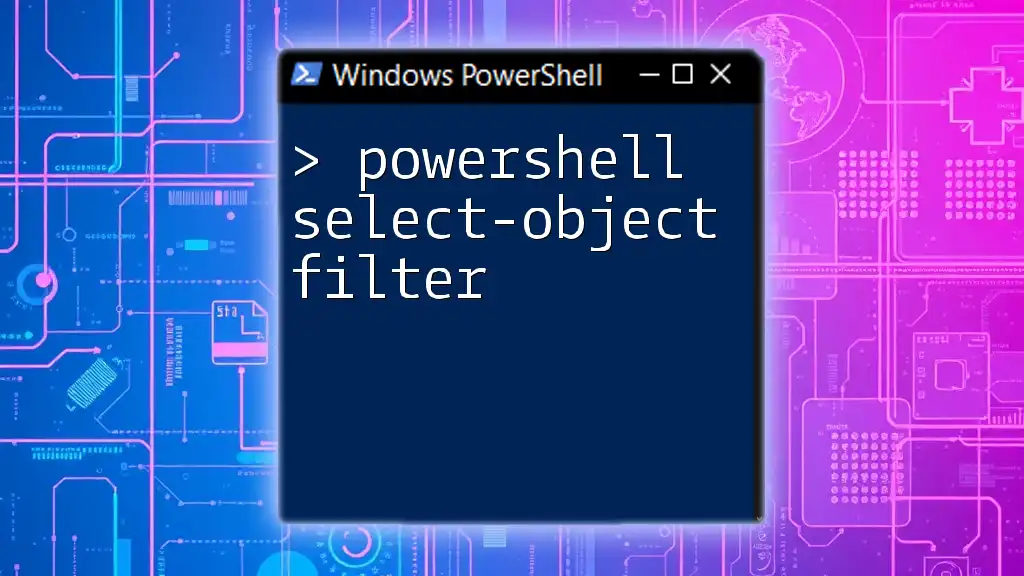
Syntax of `Select-Object -First`
The basic syntax for using `Select-Object -First` is straightforward:
Select-Object -First <number>
Here, `<number>` represents the count of items you wish to retrieve from the input stream. It's important to understand that this command selects the first N items it encounters and is typically followed by a pipeline of data.
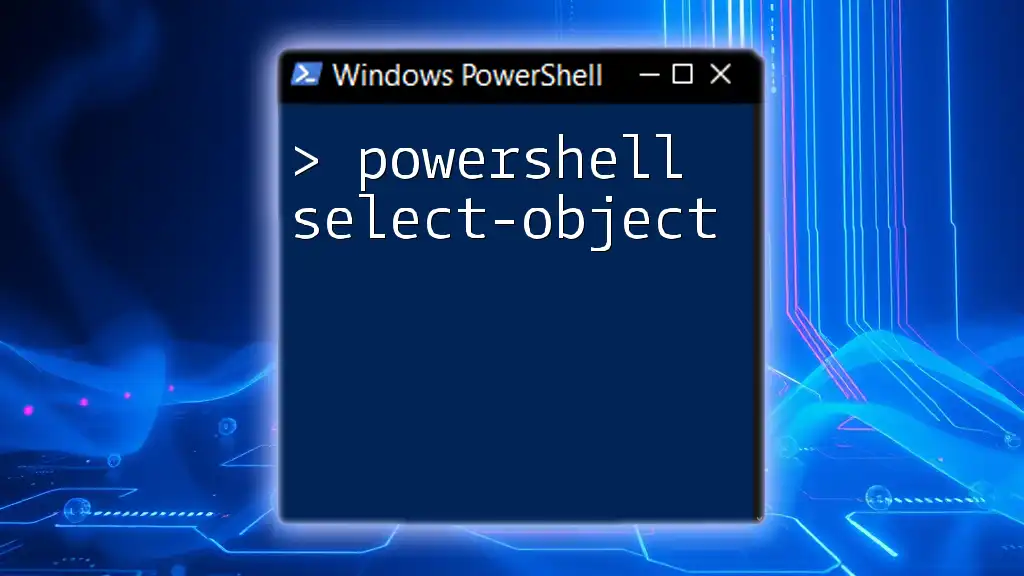
Practical Use Cases
Fetching the First N Items from a List
Suppose you have a simple list of names, and you want to retrieve just the first two. This can be easily accomplished using the following command:
$names = "Alice", "Bob", "Charlie", "David"
$firstTwo = $names | Select-Object -First 2
$firstTwo
The output will display:
Alice
Bob
This illustrates how `Select-Object -First` provides a concise way to access critical information without an overwhelming amount of surrounding context.
Filtering Objects from a Command Output
A more practical scenario involves utilizing `Select-Object -First` in conjunction with existing PowerShell cmdlets. For instance, if you want to see the top five processes running on your machine, you can implement the following command:
Get-Process | Select-Object -First 5
The output will display a list of the first five running processes, including their IDs, handles, and CPU usage, among other details. This immediate filtering is useful for monitoring system performance and allows for rapid analysis without sifting through a long list of processes.
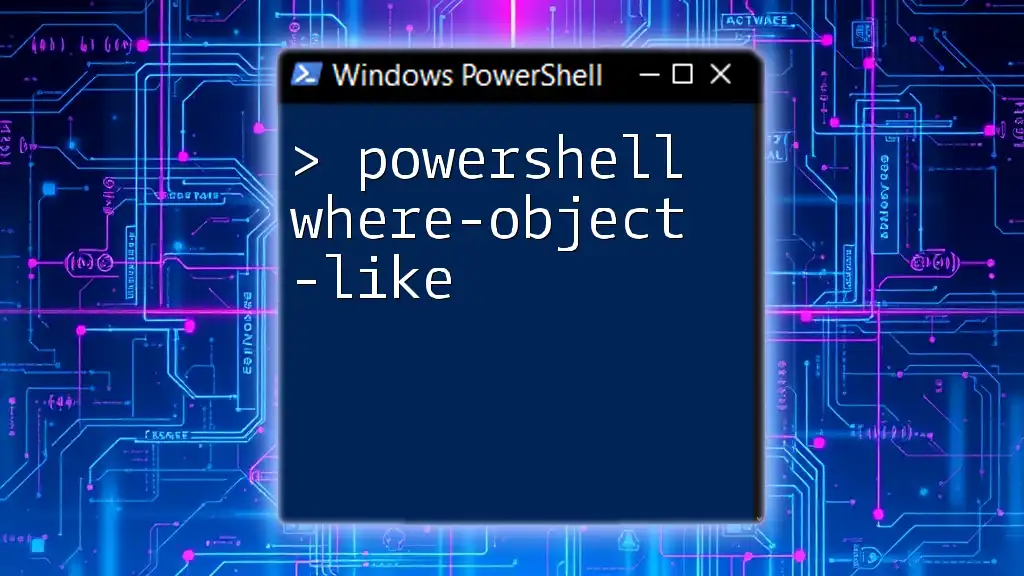
Combining `-First` with Other Parameters
Using `-First` with `-Property`
`Select-Object` also allows you to specify which properties you want to retrieve alongside the number of items you wish to limit. For example, if you're interested in the names and statuses of services, you could run:
Get-Service | Select-Object -First 5 -Property Name, Status
The output will yield:
Name Status
---- ------
Service1 Running
Service2 Stopped
Service3 Running
...
This demonstrates how you can target specific attributes while still applying the limit on results.
Combining `-First` with Sorting
Another powerful feature of PowerShell is the ability to sort data before applying `Select-Object -First`. For instance, if you want to find the processes using the most CPU, the following command will sort the processes by CPU usage in descending order and then select the top three:
Get-Process | Sort-Object CPU -Descending | Select-Object -First 3
This capability allows you to gain insights into system resources effectively and efficiently, providing a refined list crucial for performance tuning and monitoring.
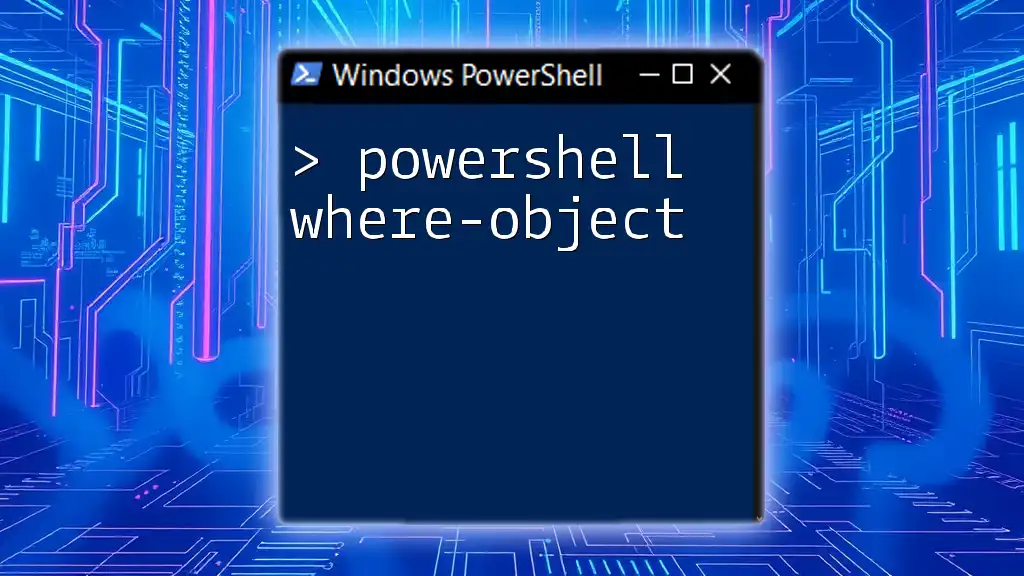
Common Mistakes and Troubleshooting
New users often misunderstand how the `-First` parameter operates, typically assuming it would return the first N results regardless of any sorting or filtering previously applied. It’s crucial to remember that without prior command modifications, `Select-Object -First` simply returns the first N items from the unfiltered output.
To avoid confusion, ensure that the data you're piping into `Select-Object` is relevant to your needs.
Here are some tips for effective use:
- Always sort data first if order matters.
- Specify properties when required to make outputs clear and useful.
- Test commands with smaller datasets before scaling up.
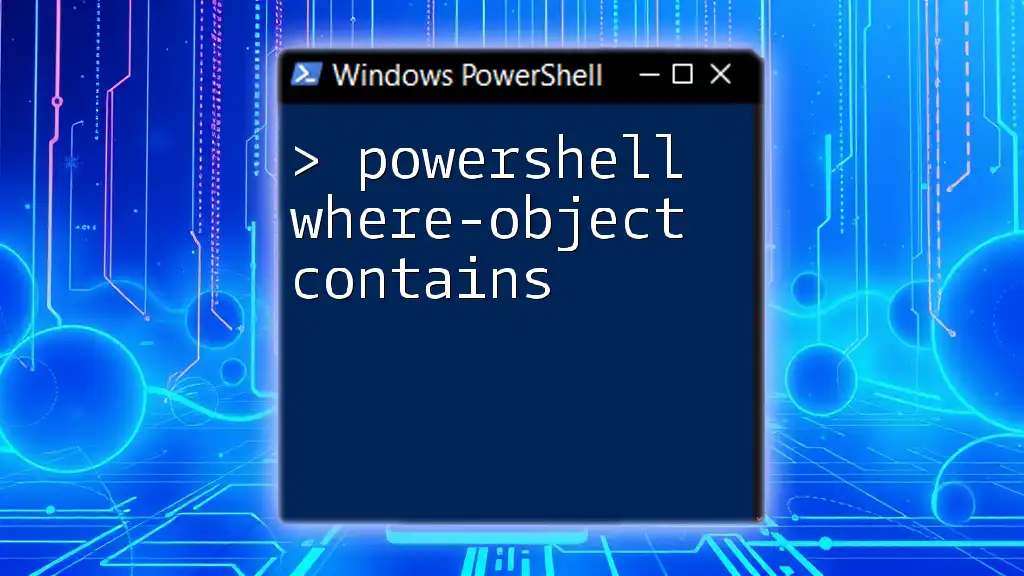
Performance Considerations
When working with large collections, using `Select-Object -First` can greatly enhance performance by minimizing the volume of data passed through subsequent pipeline commands. This strategy not only conserves resources but also speeds up execution, which is especially important during automated tasks or scripts running frequently.
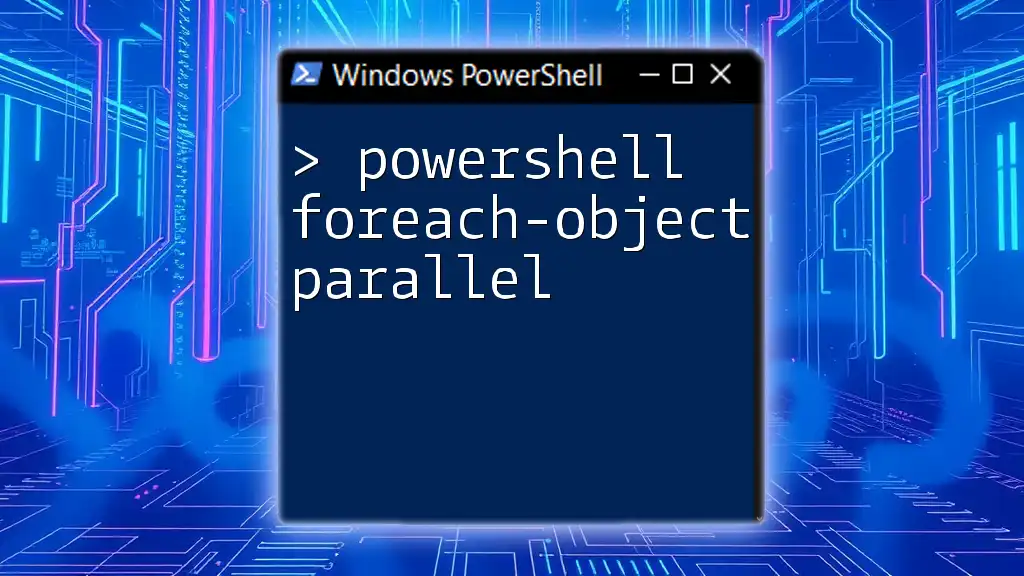
Conclusion
In summary, the `Select-Object -First` parameter is an essential aspect of PowerShell that allows users to extract concise portions of data effortlessly. By understanding its syntax and exploring its various applications, you can significantly enhance your PowerShell scripting capabilities.
Don't hesitate to experiment with `Select-Object -First` in your daily scripting tasks, and you'll soon find it an indispensable tool in your PowerShell toolbox.
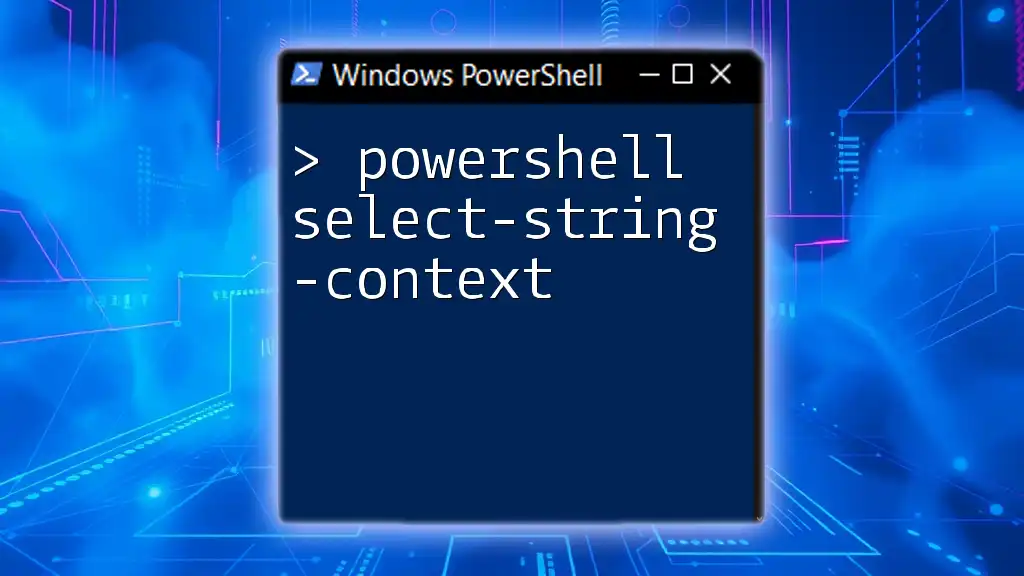
Additional Resources
For further reading, you may want to check the official Microsoft documentation on `Select-Object` and explore other PowerShell topics that aid in your journey to mastery.
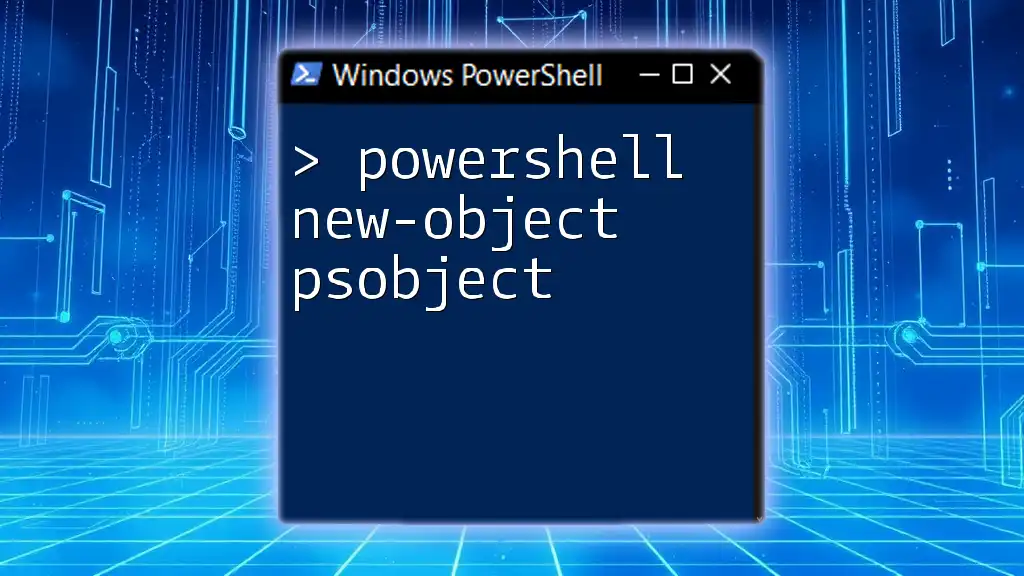
Call to Action
Stay tuned for more PowerShell tips and tricks. Subscribe to receive updates and boost your command-line skills!