Certainly! The `Where-Object` cmdlet in PowerShell allows you to filter objects based on multiple conditions using logical operators, enabling you to refine your data selections efficiently.
Here’s a code snippet demonstrating how to use `Where-Object` with multiple conditions:
Get-Process | Where-Object { $_.CPU -gt 100 -and $_.WorkingSet -lt 1MB }
This example retrieves processes that use more than 100 CPU seconds and less than 1 MB of memory.
Understanding the Basics of Where-Object
What Does Where-Object Do?
The `Where-Object` cmdlet in PowerShell is a potent tool that allows users to filter objects from a collection based on specified criteria. Filtering is crucial for working efficiently with commands that return a vast amount of information. By utilizing `Where-Object`, users can narrow down results to only the relevant items that meet their needs.
Basic Syntax of Where-Object
The basic syntax of `Where-Object` is straightforward:
Where-Object { <condition> }
In this structure, the condition defined within the braces is evaluated for each object in the pipeline. If the condition evaluates to `True`, that object is passed along in the results.
For instance, to get all processes running on your machine, you could use:
Get-Process | Where-Object { $_.Status -eq 'Running' }
In this example, `$_` represents the current object in the pipeline.

How to Use Where-Object with Multiple Conditions
Combining Conditions with `-and`
One of the primary ways to filter using multiple conditions is by utilizing the `-and` operator. This operator allows you to specify that all of the conditions must be true for an object to be selected.
For example, to filter processes that are actively running and utilizing more than 50 CPU cycles, you can do the following:
Get-Process | Where-Object { $_.CPU -gt 50 -and $_.Status -eq 'Running' }
In this snippet, the command retrieves all processes from `Get-Process`, and then `Where-Object` filters them by checking both the CPU usage and the running status.
Breaking Down the Example
- Get-Process: Retrieves a list of all processes currently running on the system.
- Where-Object: Evaluates the conditions for each process.
- Conditions: Both conditions must be `True` for the process to be included in the output.
Utilizing `-or` for Flexible Conditions
The `-or` operator is incredibly useful for situations where you want to include objects that meet at least one of several conditions. For instance, if you wanted to retrieve services that are either running or stopped, you could write:
Get-Service | Where-Object { $_.Status -eq 'Running' -or $_.Status -eq 'Stopped' }
Analyzing the Output
In this command:
- It fetches all services from `Get-Service`.
- Filters them based on whether they are either "Running" or "Stopped". This command can be particularly advantageous in diagnostics when you want to identify the status of services.

Advanced Filtering with Where-Object
Using Nested Conditions
Complex filtering scenarios may require combining both `-and` and `-or` operators. This can be achieved through nested conditions.
For instance, if you need to filter processes that are either using more than 50 CPU cycles while running or are named `svchost`, the command would look like this:
Get-Process | Where-Object { ($_.CPU -gt 50 -and $_.Status -eq 'Running') -or $_.Name -like 'svchost*' }
Importance of Parentheses
Notice the use of parentheses; they dictate the order of evaluation. In this case, the conditions inside the parentheses must be checked first, allowing for clear and logical filtering.
Filtering with Properties
In many cases, you will filter not just on values but also on dates or other properties. A practical example would be fetching error logs from the Application event log generated within the last week:
Get-EventLog -LogName Application | Where-Object { $_.EntryType -eq 'Error' -and $_.TimeGenerated -gt (Get-Date).AddDays(-7) }
Explanation of the Results
This command retrieves the event log entries and filters them based on two properties: `EntryType` and `TimeGenerated`. This is particularly useful for system administrators looking to identify recent errors for troubleshooting.

Performance Considerations
Impact of Multiple Conditions on Performance
Using multiple conditions can impact performance, especially when dealing with large datasets. Logical evaluations will increase the processing time required for executing commands. Always consider the efficiency of your conditions.
Best Practices for Optimizing Where-Object Commands
To optimize, try to:
- Limit the data fetched: If possible, retrieve only the necessary data.
- Use direct filtering: Employ filters in commands that directly return results to minimize the number of objects passed to `Where-Object`.
Alternatives to Where-Object for Complex Queries
For highly complex queries, you might consider using `ForEach-Object` or leveraging filtering methods available in other cmdlets. For instance, using `Select-Object` can often achieve simpler outcomes without involving `Where-Object`.

Practical Use Cases
Real-World Examples of Where-Object with Multiple Conditions
Scenario 1: Filtering System Logs for Errors
A commonly faced issue in system management is identifying errors. Use the following code to retrieve relevant information:
Get-EventLog -LogName System | Where-Object { $_.EntryType -eq 'Error' -and $_.TimeGenerated -gt (Get-Date).AddDays(-30) }
Scenario 2: Managing Services on a Server
To manage your services, you can filter to show only those services that are either stopped or automatically running:
Get-Service | Where-Object { $_.Status -eq 'Stopped' -or $_.StartType -eq 'Automatic' }
Scenario 3: Monitoring Process Utilization
Monitoring high CPU usage processes can help alleviate performance issues on a server:
Get-Process | Where-Object { $_.CPU -gt 75 -and $_.Status -eq 'Running' }

Conclusion
Utilizing `Where-Object` with multiple conditions is a powerful method for refining your data retrieval efforts in PowerShell. By mastering this capability, you’ll enhance your scripting efficiency and improve your system management tasks. Remember to practice these commands and adapt them to your workflow. Keep exploring and expanding your PowerShell knowledge for even greater success in your automation tasks!
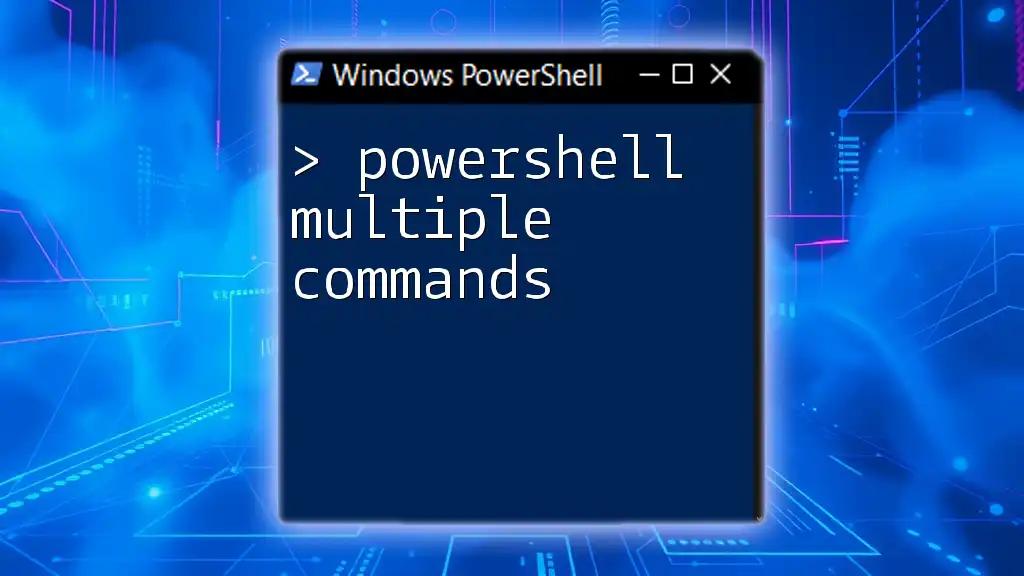
Additional Resources
For more extensive learning, consider referring to the official Microsoft PowerShell documentation, engaging with community-driven forums, or exploring specialized books and online courses dedicated to PowerShell.