In PowerShell, you can convert an object to a string by using the `Out-String` cmdlet, which takes the object and formats it as a string representation.
$object = Get-Process | Select-Object -First 1
$string = $object | Out-String
Write-Host $string
Understanding PowerShell Objects
What is a PowerShell Object?
PowerShell is fundamentally built around the concept of objects. An object in PowerShell represents a collection of properties and methods that describe a particular entity. For instance, a `Process` object contains properties like `Name`, `Id`, and `CPU`, while a custom object might encompass any type of data defined by the user.
Why Convert Objects to Strings?
There are numerous scenarios in which converting an object to a string is beneficial. These include:
- Logging: When recording information into logs, strings are often preferred for their simplicity and readability.
- Display Output: If you wish to present data to users in a console or graphical interface, string formats allow for better formatting and user comprehension.
Converting objects to strings makes your scripts more versatile and allows for easier integration with various systems that understand string data better than complex objects.
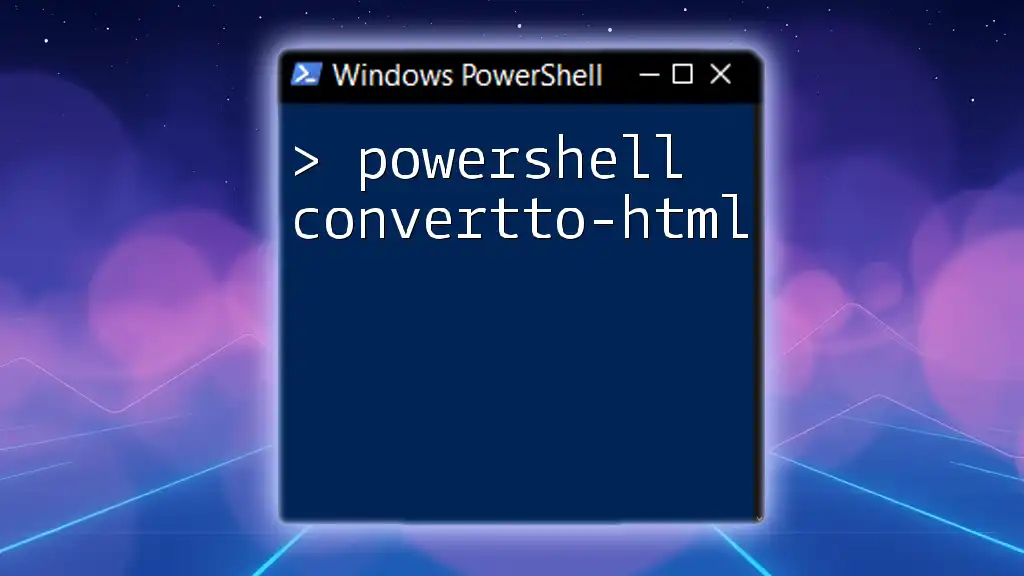
The Basics of Object to String Conversion
Using the `ToString()` Method
PowerShell provides a fundamental method for converting objects to strings—the `ToString()` method. This method is inherited from the .NET Framework and can be applied to most objects.
$obj = Get-Process | Select-Object -First 1
$str = $obj.ToString()
Write-Output $str
In this code, we retrieve the first process from the system, and then convert it to a string using `ToString()`. The output will vary depending on the object type, thus displaying relevant details based on the object’s properties.
Implicit Conversion to String
PowerShell inherently possesses the ability to perform implicit conversions when an object is treated as a string. This occurs in contexts where the object is placed within a string expression, allowing PowerShell to convert it automatically.
$example = Get-Date
"The current date and time is: $example"
In this snippet, the `Get-Date` command retrieves the current date and time. When included in the string output, PowerShell automatically converts the `DateTime` object into a string format, demonstrating convenience and efficiency.
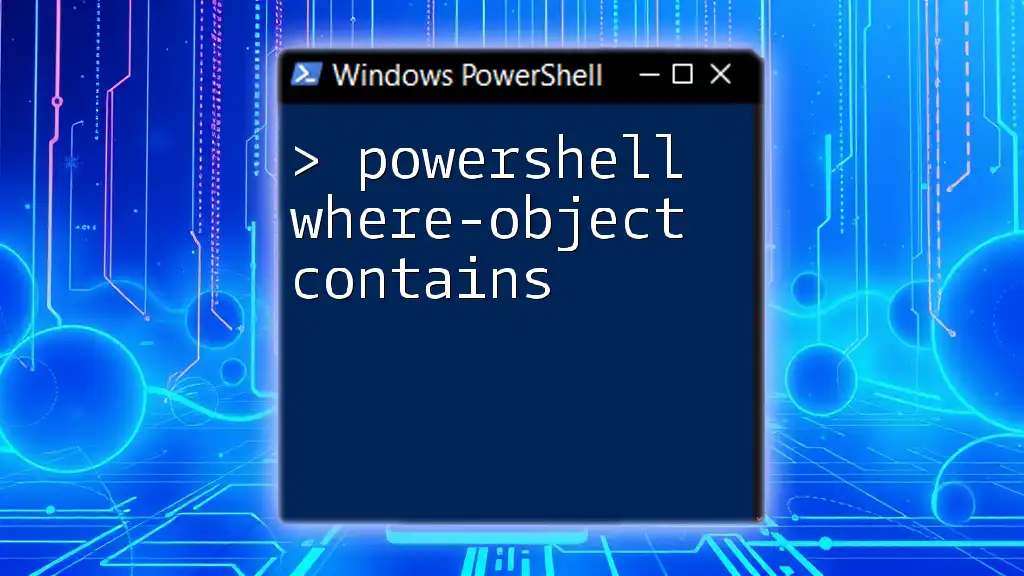
Advanced Techniques for Object to String Conversion
Using `ConvertTo-Json`
In situations involving complex data structures, the `ConvertTo-Json` cmdlet becomes invaluable, allowing for the representation of objects in JSON format. This format is widely accepted in various applications and services.
$processes = Get-Process | Select-Object -First 5
$jsonString = $processes | ConvertTo-Json
Write-Output $jsonString
The command retrieves the first five processes running on the machine and converts them to a JSON string. This is particularly useful for APIs and applications requiring structured data formats.
Formatting with `Out-String`
Another powerful cmdlet for converting objects to strings is `Out-String`. This cmdlet is especially beneficial when you wish to create a more human-readable format of the output.
$process = Get-Process | Select-Object -First 1
$formattedString = $process | Out-String
Write-Output $formattedString
Here, `Out-String` takes the `Process` object and formats it into a readable string. By using this cmdlet, you can gain more control over how the output appears, enabling clearer communication of information to users.
Using String Interpolation
String interpolation in PowerShell allows you to craft strings that embed expressions directly. This is particularly useful for incorporating object properties seamlessly.
$user = New-Object PSObject -Property @{ Name = "John"; Age = 30 }
"User Info: Name: $($user.Name), Age: $($user.Age)"
In this example, we create a custom object `user` and use string interpolation to display its properties. The use of `$()` around `$user.Name` and `$user.Age` is essential to ensure that the properties are evaluated correctly within the string.
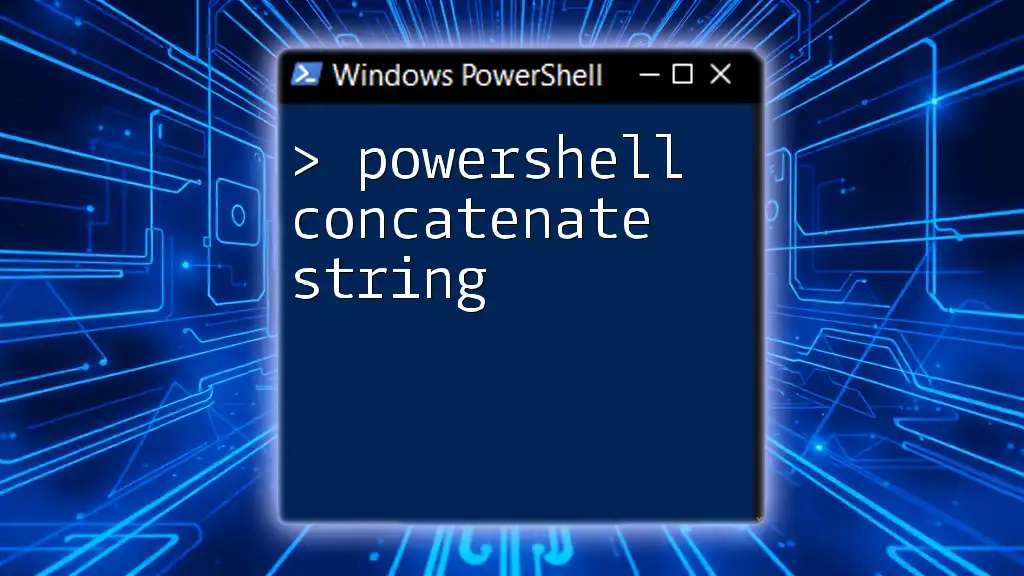
Handling Custom Objects
Creating Custom Objects in PowerShell
PowerShell allows for the creation of custom objects using the `New-Object` cmdlet or its simpler alternative, `PSCustomObject`. Custom objects enable the grouping of related data into a single entity.
$customObj = [PSCustomObject]@{ Name = "Alice"; Age = 25 }
In this code snippet, a custom object is created with properties `Name` and `Age`, demonstrating how you can encapsulate multiple pieces of information about a single entity.
Converting Custom Objects to String
When it comes to converting custom objects to a string format, the same techniques apply. However, to enhance readability, converting to JSON or using string representations might often be the preferred methods.
$customObjString = $customObj | ConvertTo-Json
Write-Output $customObjString
This example illustrates using `ConvertTo-Json` to transform our custom object into a JSON string, allowing for easier sharing and storage of data in a universally recognized format.
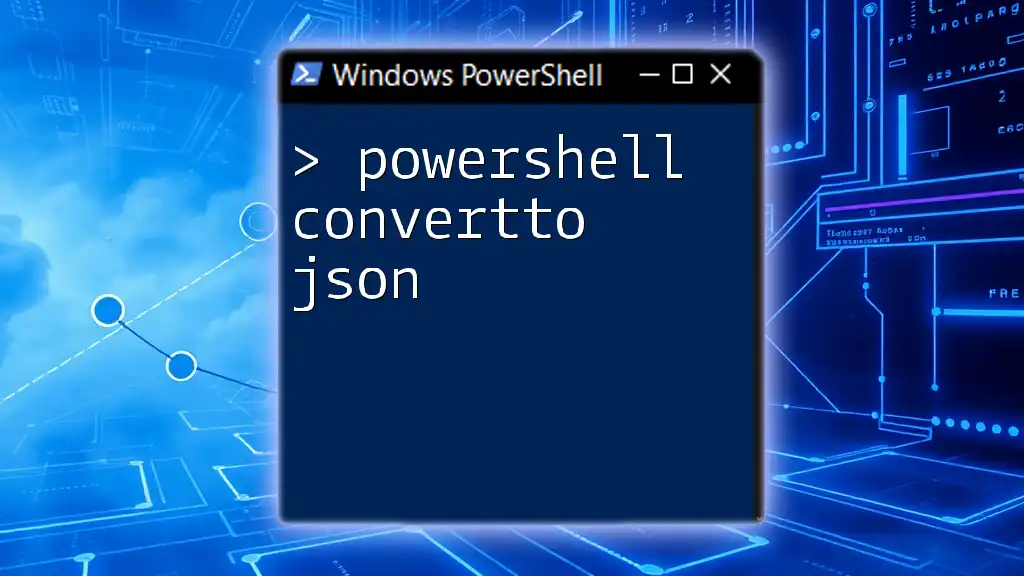
Conclusion
Converting objects to strings in PowerShell is not just a technical necessity but also a practical skill for effective scripting and automation. Through methods like `ToString()`, `ConvertTo-Json`, `Out-String`, and string interpolation, users can tailor their outputs based on specific needs.
Understanding the nuances of object types and their string representations will enhance your scripting capabilities greatly. Remember, the key to maximizing PowerShell's efficiency lies in leveraging the robust features designed for object manipulation, enabling more effective commands and better results.
Take time to experiment with these techniques and find the ones that work best for your scenarios. The world of PowerShell is rich with possibilities, and mastering these skills will elevate both your technical prowess and productivity.