To convert a string to an integer in PowerShell, you can simply cast the string into an integer type using `[int]`.
$intValue = [int]"123"
Write-Host $intValue # Outputs: 123
Understanding Data Types in PowerShell
What are Strings and Integers?
In PowerShell, strings are sequences of characters that can represent text, whereas integers (or int) are numerical values without any fractional components. Understanding these basic data types is essential because they dictate how data is manipulated within your scripts.
For instance, a string like `"123"` is treated as text, even though it represents a numeric value. When performing calculations or comparisons, this distinction can lead to errors if not handled correctly.
Why Convert Strings to Integers?
The need to convert strings to integers arises in various situations, particularly when you are dealing with user input, reading data from files, or interfacing with databases. Converting to integers allows you to perform mathematical operations or other comparisons in your PowerShell scripts.
In your scripts, you might encounter scenarios where:
- You need to perform calculations (e.g., adding, subtracting values).
- You want to compare numeric values derived from user input or external sources.
Understanding how to convert strings to integers seamlessly can save a lot of debugging time and enhance script efficiency.

PowerShell Convert String to Int Techniques
Using `[int]` to Cast a String
One of the most straightforward methods to convert a string to an integer in PowerShell is by using the casting operator `[int]`. This method is simple and easy to remember:
$stringValue = "123"
$intValue = [int]$stringValue
In this example, the value `"123"` is converted to the integer `123`. If the string contains purely numeric characters, the conversion will succeed without issues.
Using the `ConvertTo-Int` Cmdlet
PowerShell allows the creation of custom functions, which can encapsulate repetitive tasks. Here’s an example of a custom function, `ConvertTo-Int`, that can be reused throughout your scripts:
Function ConvertTo-Int {
param (
[string]$inputString
)
return [int]$inputString
}
To use this function, simply pass a string representing a number, and it will return the integer equivalent. This modular approach enhances code reusability and maintainability.
Employing `System.Int32::Parse()`
Another powerful method for converting strings to integers in PowerShell is to use the `.NET` class method `System.Int32::Parse()`. This method explicitly indicates that you are working with integer types, which can improve readability in your scripts:
$stringValue = "456"
$intValue = [System.Int32]::Parse($stringValue)
This technique can also be beneficial when converting formatted numbers, providing additional granularity and control.
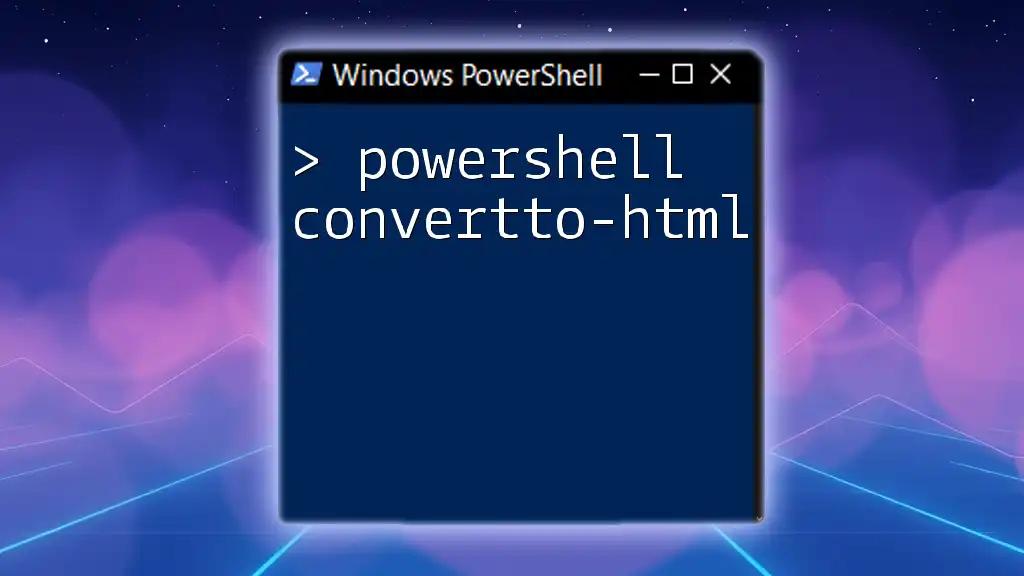
Handling Conversion Errors
Common Errors in String-to-Int Conversion
When converting strings to integers, common errors can occur, particularly with non-numeric inputs. For example, attempting to convert the string "abc" will yield an error because it cannot be interpreted as an integer.
Implementing Try-Catch for Error Handling
To handle potential errors gracefully, you can implement a `Try-Catch` block. This allows you to manage exceptions and provide meaningful feedback when conversion fails:
$stringValue = "abc"
try {
$intValue = [int]$stringValue
} catch {
Write-Host "Conversion failed: $_"
}
In this example, if the conversion fails, the error message is captured and printed, allowing the script to continue running without crashing.

Best Practices for Data Conversion
Validating Input Strings
Before attempting to convert a string to an integer, it is essential to validate the input. Ensuring that the string is not null or empty, and that it consists solely of numeric characters can prevent errors:
if (-not [string]::IsNullOrWhiteSpace($stringValue) -and $stringValue -match '^\d+$') {
$intValue = [int]$stringValue
} else {
Write-Host "Input is not a valid number."
}
By incorporating validation checks, you significantly increase the robustness of your scripts, ensuring that only valid data is processed.
Performance Considerations
Frequent string-to-integer conversions in larger scripts can impact performance. It is advisable to minimize conversions by validating user input once and then storing it in integer variables for further calculations.
In high-performance scenarios, consider structuring your code to handle data efficiently,choosing appropriate data types when declaring variables, as this can lead to improved script performance and reduced overhead.
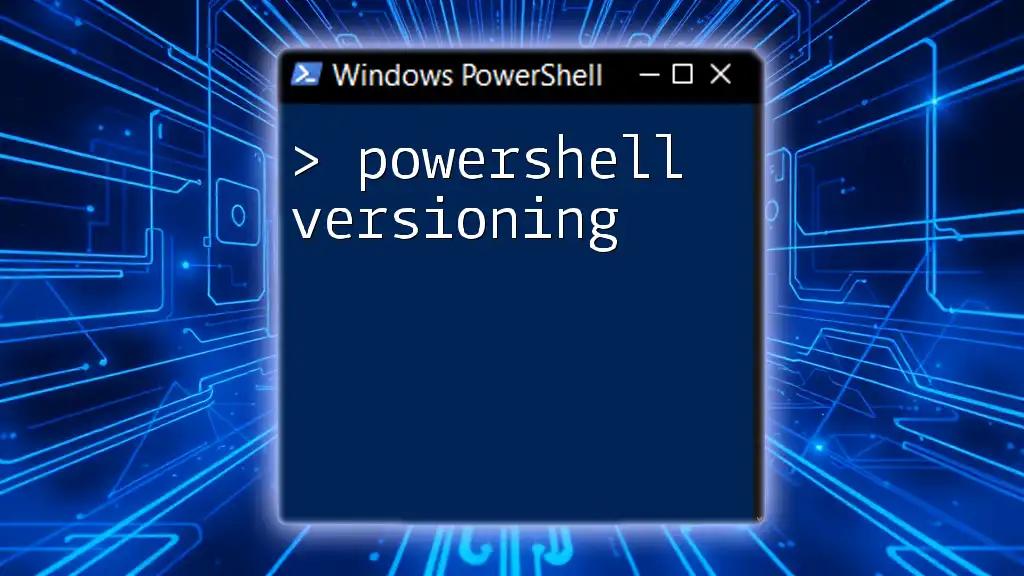
Conclusion
Understanding how to effectively convert strings to integers in PowerShell is a fundamental skill for any scripter or developer. By mastering techniques such as casting, utilizing .NET methods, and implementing proper error handling and validation, you can write scripts that are both efficient and resilient.
As you practice converting strings to integers in your scripts, you'll find that this knowledge enhances your ability to manipulate data and develop more complex automation tasks. Embrace the power of type conversion in PowerShell, and you'll unlock a new level of script efficiency and effectiveness.
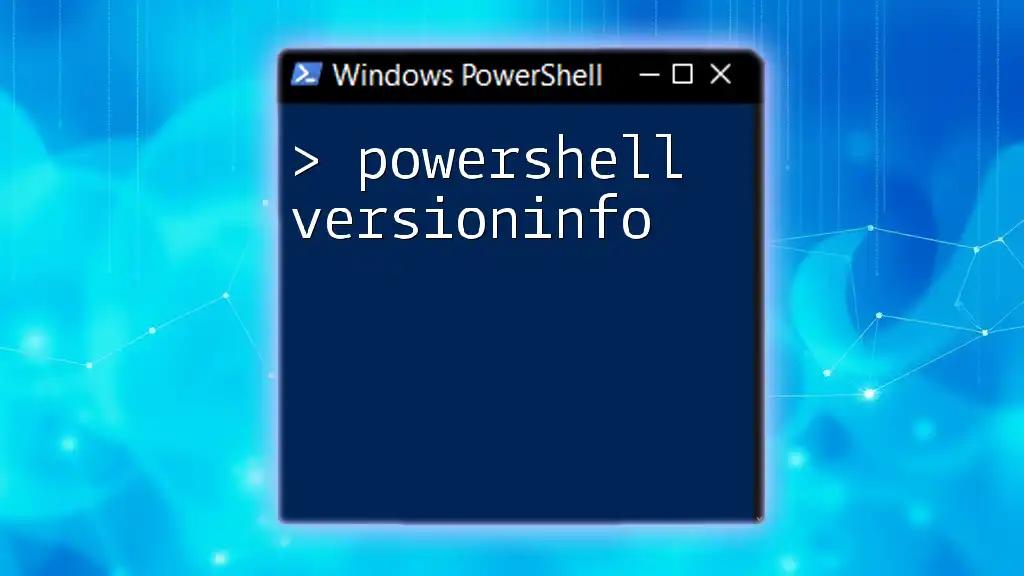
Additional Resources
For further exploration of PowerShell data types and conversions, consider checking out official documentation, forums, and additional tutorials. Engaging with the PowerShell community will also provide insights into practical applications and advanced techniques related to data manipulation in your scripts.