In PowerShell, you can convert a string to a date using the `Get-Date` cmdlet along with a string formatted as a date, as shown in the following code snippet:
$dateString = "2023-10-01"
$convertedDate = Get-Date $dateString
Write-Host $convertedDate
Understanding Date and Time Formats in PowerShell
ISO 8601 Format
PowerShell recognizes the ISO 8601 date format, which is crucial for ensuring consistency across different systems and applications. This format looks like this: `YYYY-MM-DDTHH:MM:SSZ`. It is both human-readable and machine-friendly, making it the preferred format for data interchange.
Other Common Formats
In addition to ISO 8601, there are various other date formats like MM/DD/YYYY and DD-MM-YYYY. Understanding these formats is vital before performing conversions, as PowerShell's interpretation of a date string can change based on its structure. Knowing how different formats are processed under the hood will help you avoid common pitfalls when using PowerShell commands.
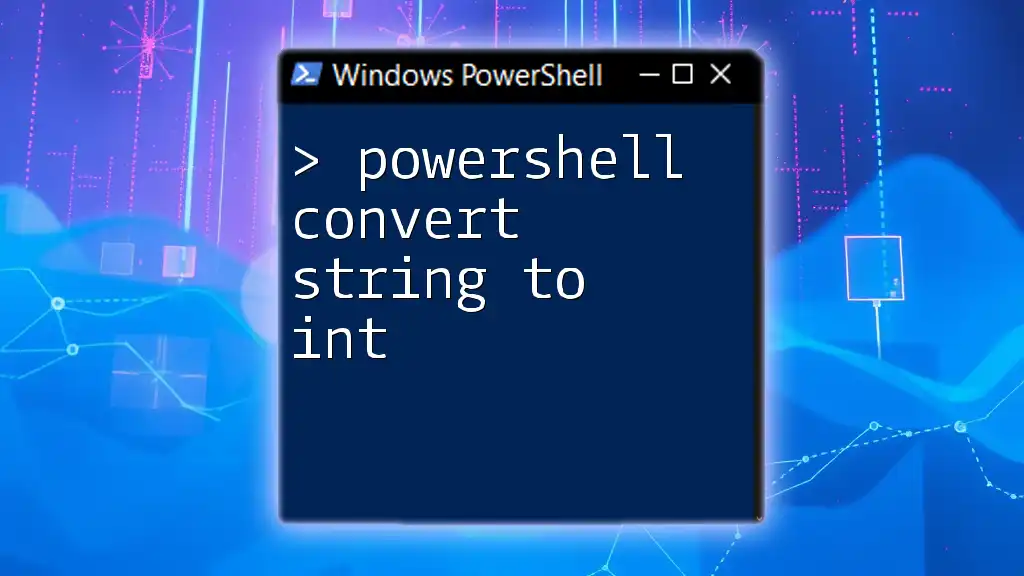
PowerShell String to Date Conversion
PowerShell Convert String to Date Command Overview
The primary cmdlet used for converting strings to dates in PowerShell is `Get-Date`. This command can interpret various string formats and convert them into a `<DateTime>` object. Mastering this command is essential for manipulating dates effectively in your scripts.
Basic Syntax of Convert String to Date
The syntax for the conversion command is straightforward:
$date = Get-Date "YourDateString"
This command takes a date string as input and returns a date object, enabling you to perform additional operations or comparisons.
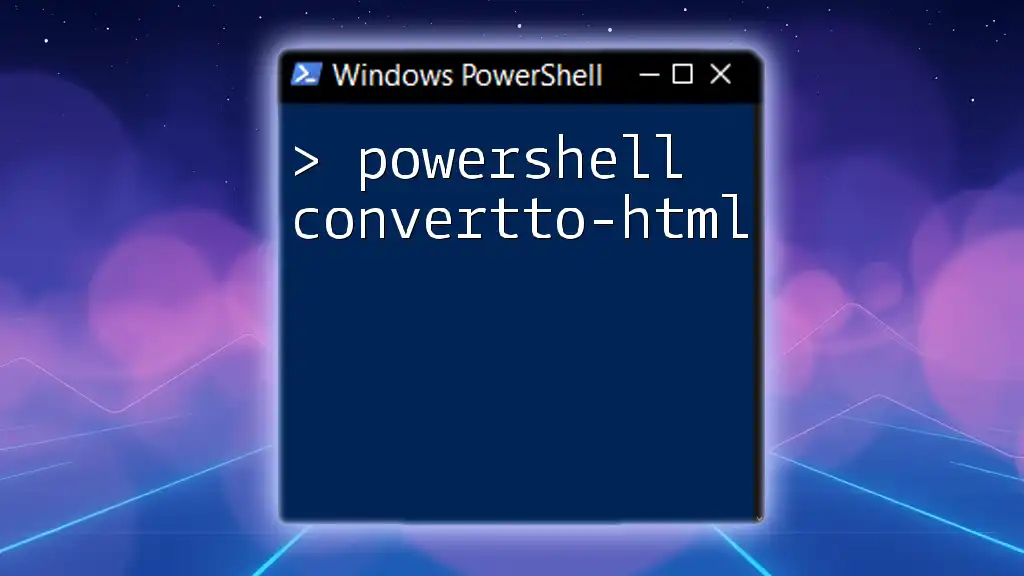
Using Get-Date for String to Date Conversion
Converting a Simple Date String
To demonstrate how to convert a string representing a date, consider the following example:
$dateString = "October 15, 2023"
$date = Get-Date $dateString
In this example, PowerShell identifies "October 15, 2023" as a valid date format and converts it to a date object. Understanding how PowerShell interprets different string formats will enhance your ability to handle user input and data from various sources accurately.
Handling Different Date Formats
MM/DD/YYYY Format
Another prevalent format is MM/DD/YYYY. When you want to convert this format, you can use:
$dateString = "10/15/2023"
$date = Get-Date $dateString
PowerShell recognizes this string as October 15, 2023 without any ambiguity. Such conversions can be especially useful when processing input from users or external systems where date formats can vary.
DD-MM-YYYY Format
You may also encounter dates in the DD-MM-YYYY format. To convert a date in this format, you would use:
$dateString = "15-10-2023"
$date = Get-Date $dateString
It is important to know when to specify the format explicitly, especially if the string can be misinterpreted. This helps avoid unexpected behavior in your scripts and ensures that your date values are accurate.
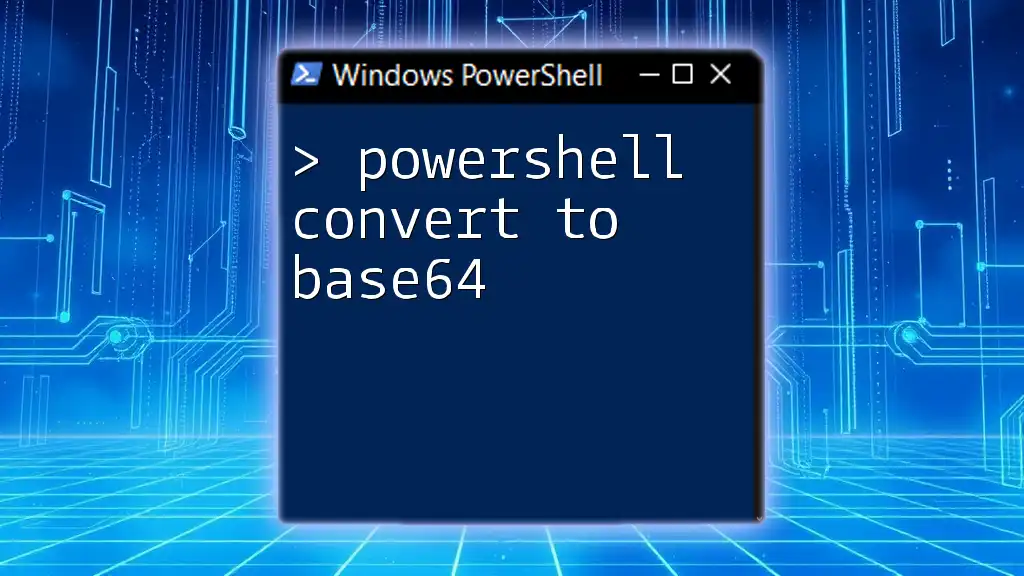
Advanced Conversions
PowerShell Convert String to DateTime
In PowerShell, `DateTime` is a more precise representation of date and time. To convert a string to a `DateTime` object, you can use a similar command:
$dateTimeString = "2023-10-15 14:30:00"
$dateTime = Get-Date $dateTimeString
This command converts the string into a `DateTime` object, allowing for enhanced functionality, such as time zone handling and more granular date operations.
Using Culture-Specific Formats
Handling different cultural settings can introduce additional complexities into date parsing. For instance, users from different regions may have their date settings. You could use the following code snippet to convert a culture-specific format:
$dateString = "15/10/2023"
$date = [datetime]::ParseExact($dateString, "dd/MM/yyyy", $null)
Here, `ParseExact` is particularly useful for situations where date input formats are ambiguous. By providing the exact format that matches your string, you ensure the conversion is executed correctly, thus minimizing errors.
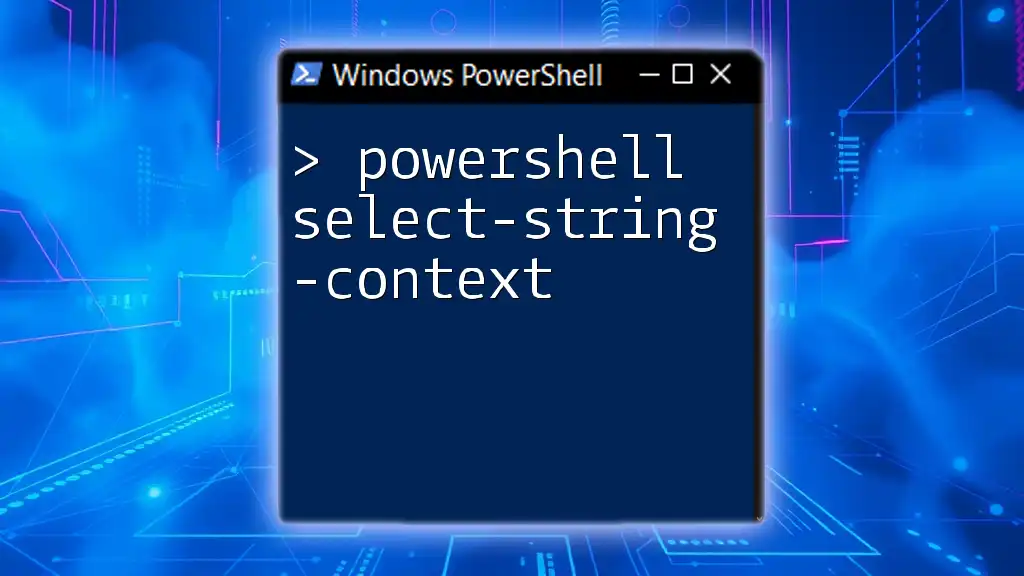
Error Handling in Date Conversions
Common Errors
While converting string dates, you may encounter common errors, primarily due to invalid formats or misinterpretation by PowerShell. Examples include errors like "String was not recognized as a valid DateTime."
Error Handling Techniques
To effectively handle errors in date conversions, implement `Try` and `Catch` blocks, ensuring that your scripts can recover gracefully from exceptions:
try {
$date = Get-Date "invalid date string"
} catch {
Write-Host "Error: $_"
}
Employing these error handling techniques not only improves the reliability of your scripts but also enhances the user's experience by providing clear feedback on input issues.
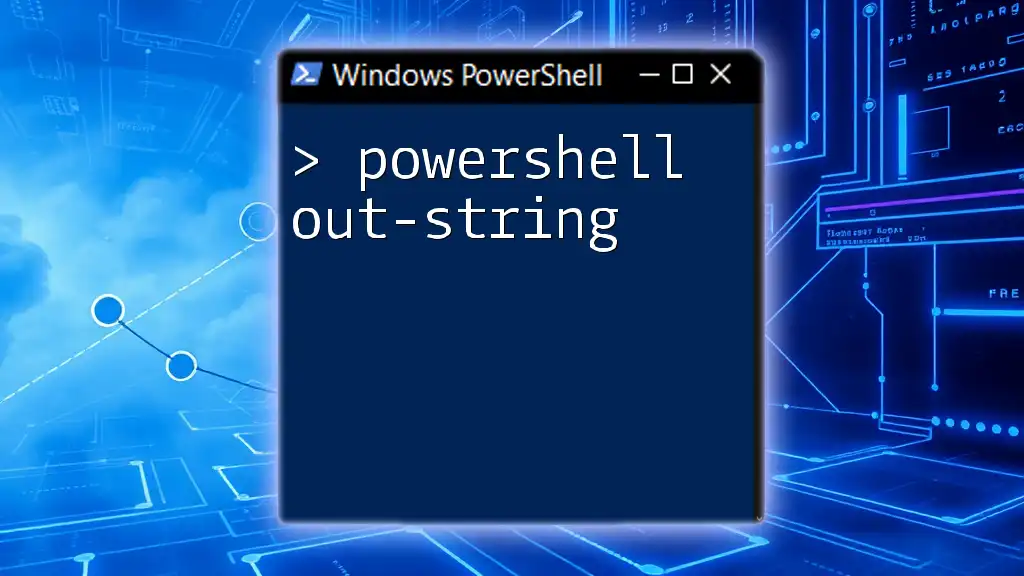
Conclusion
Mastering the techniques for string to date conversions in PowerShell is an invaluable skill for any scripting enthusiast. Knowing how to manipulate and handle dates is critical for a wide range of applications, from logging events to data analysis. By understanding various date formats, using the `Get-Date` command effectively, and implementing strong error handling, you will significantly enhance your PowerShell scripting capabilities. Practice with different date formats and scenarios to deepen your expertise and confidence in using this powerful command.
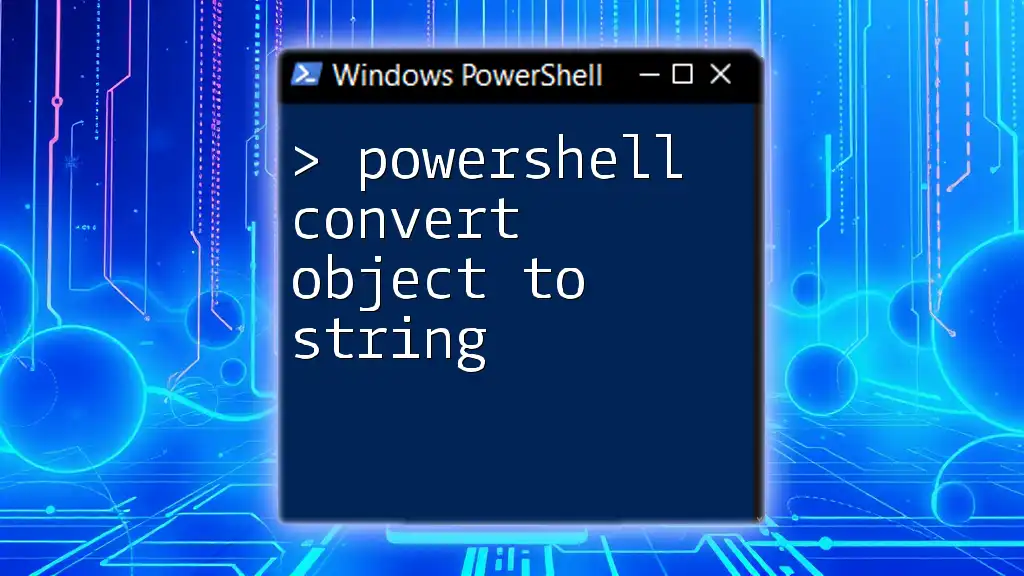
Additional Resources
For further reading, consider exploring additional online resources dedicated to PowerShell's date and time commands. Engaging with PowerShell forums and communities can also provide valuable insights and tips from experienced users, helping you to grow in your scripting journey.