In PowerShell, you can convert a string into an array by splitting it based on a specified delimiter using the `-split` operator.
Here's a code snippet to demonstrate this:
$string = "apple,banana,cherry"
$array = $string -split ','
$array
Understanding PowerShell Strings and Arrays
What is a String in PowerShell?
In PowerShell, a string is a sequence of characters treated as a single data type. Strings can include letters, numbers, symbols, and spaces. They are commonly used for text manipulation, command outputs, and even for saving configuration settings. Strings are enclosed in either single quotes (`'`) or double quotes (`"`), with the main difference being that double quotes allow for variable expansion.
What is an Array in PowerShell?
An array in PowerShell is a collection of items that can hold multiple values in a single variable. Arrays allow you to store data elements of various types, including strings, integers, and objects. The primary advantage of using arrays is that they enable you to manage and manipulate related data points efficiently, making your scripts cleaner and easier to maintain.
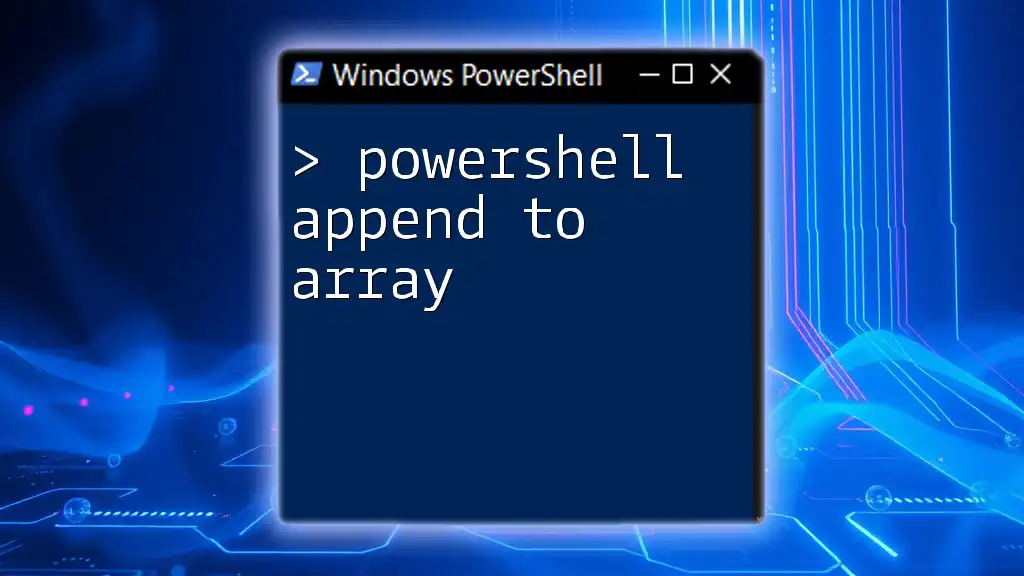
Converting Strings to Arrays in PowerShell
Why Convert a String to an Array?
Converting a string to an array can be beneficial when you want to perform operations on individual elements. For instance, you may need to parse a comma-separated list or manipulate specific words from a sentence. This flexibility allows for better data handling in scripted tasks, especially in automation and report generation.
Basic Conversion Techniques
Using `-split` Operator
One of the most straightforward methods to convert a string to an array is by using the `-split` operator. This operator divides a string into substrings based on a specified delimiter.
Example:
$string = "PowerShell is great"
$array = $string -split " "
$array
In this case, `$array` will contain `("PowerShell", "is", "great")`, breaking the original string at each space.
Using the `.ToCharArray()` Method
Another method to convert a string into an array is by using the `.ToCharArray()` method, which transforms a string into an array of its constituent characters.
Example:
$string = "PowerShell"
$array = $string.ToCharArray()
$array
In this case, `$array` will contain an individual character array `('P', 'o', 'w', 'e', 'r', 'S', 'h', 'e', 'l', 'l')`.
Advanced Conversion Techniques
Specifying Delimiters with `-split`
In some cases, you may need to split a string using multiple delimiters or even complex patterns. The `-split` operator can accept regular expressions to handle this.
Example:
$string = "one,two;three four"
$array = $string -split '[,; ]'
$array
Here, `$array` will contain `("one", "two", "three", "four")`, as it splits the string at commas, semicolons, and spaces.
Using `Select-String` with Pipelines
You can also use `Select-String` along with pipelines to filter and convert strings into an array format effectively. This is particularly useful when processing the output of commands.
Example:
Get-Content "file.txt" | Select-String "pattern" | ForEach-Object { $_.Line } | Out-String -Stream
This command searches through `file.txt`, selecting lines that match the specific pattern and placing them into an array format, allowing for further processing.
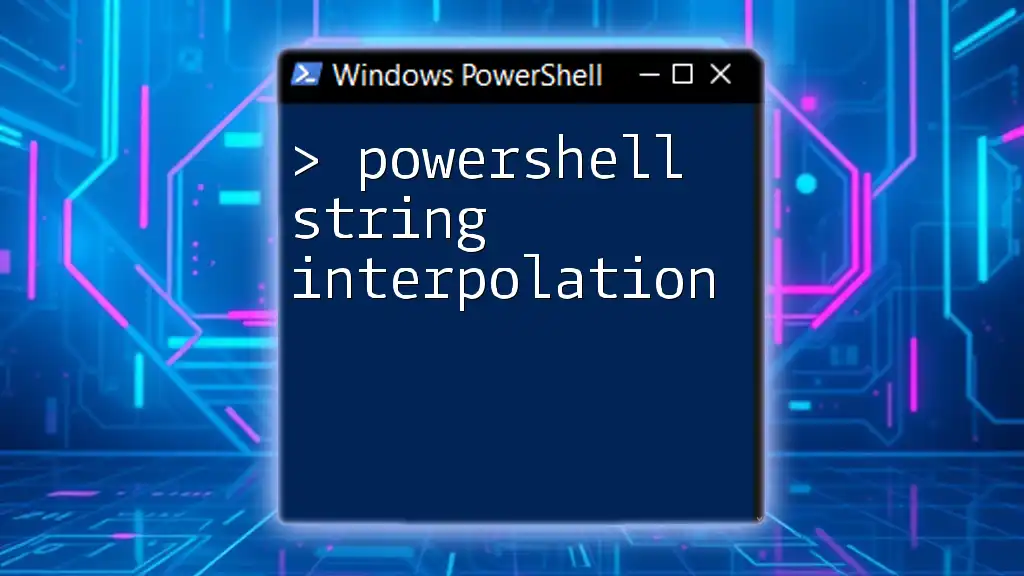
Common Scenarios for String to Array Conversion
Parsing CSV or Delimited Files
An excellent use case for converting strings to arrays is when dealing with CSV files. You can utilize the `Import-Csv` cmdlet to create an array from a specific field within a structured CSV.
Example:
$data = Import-Csv "data.csv"
$firstNames = $data | ForEach-Object { $_.FirstName }
In this instance, `$firstNames` will be an array containing the first names extracted from the CSV data, making it easier to loop through or further manipulate.
Splitting Text from Files
Another common task is to read a text file and convert its contents to an array for processing. PowerShell offers simple methods to achieve this.
Example:
$content = Get-Content "file.txt" -Raw
$lines = $content -split "`r?`n"
This splits the content of the file into an array of lines, allowing you to handle each line individually as needed.
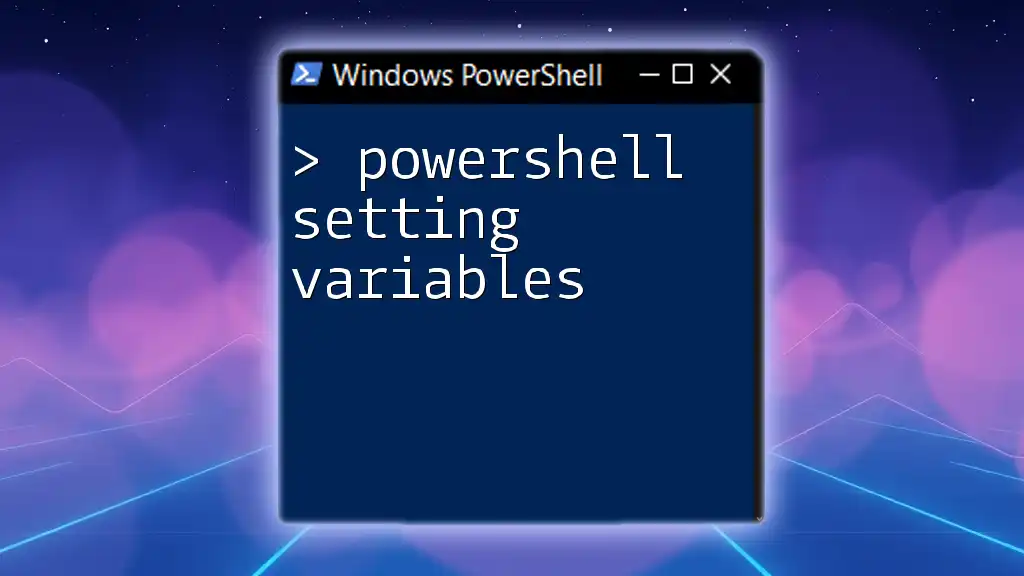
Handling Special Cases
Dealing with Null or Empty Strings
When working with null or empty strings, special care is needed, as they may lead to unexpected results during conversion.
Example:
$string = ""
$array = $string -split " "
if ($array.Count -eq 0) {
Write-Host "The array is empty."
}
Here, the condition checks if the array is empty and can be a useful practice to avoid errors in your scripts when dealing with uncertain data.
Converting Complex Strings
Sometimes, your strings may contain structured data, such as JSON. In these scenarios, you can parse the JSON and extract the relevant parts into an array.
Example:
$jsonString = '{"name": "John", "skills": ["PowerShell", "C#"]}'
$array = ((ConvertFrom-Json $jsonString).skills)
In this case, `$array` will contain the skills as an array `("PowerShell", "C#")`, offering a seamless way to handle structured data formats.
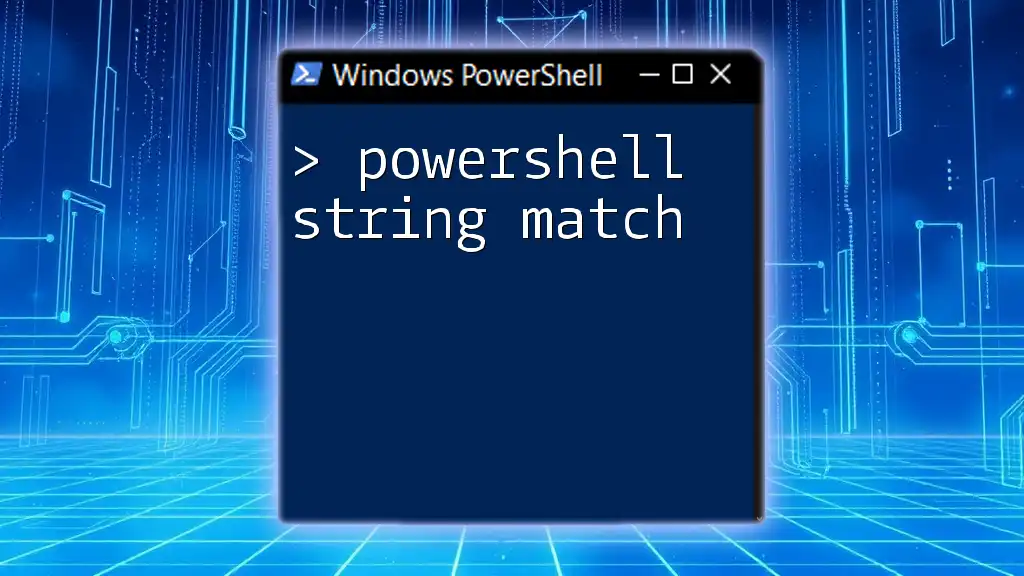
Best Practices
When to Use Arrays
Arrays are particularly useful when dealing with collections of data elements that require manipulation or processing. However, it's important to consider performance implications when working with large datasets, as excessive use of arrays may lead to memory consumption issues.
Readability and Maintenance
Maintaining readability and organization in your scripts is vital. Always comment your code, especially when converting strings to arrays, to ensure that your intentions are clear, not only for yourself but also for others who might read your scripts in the future.
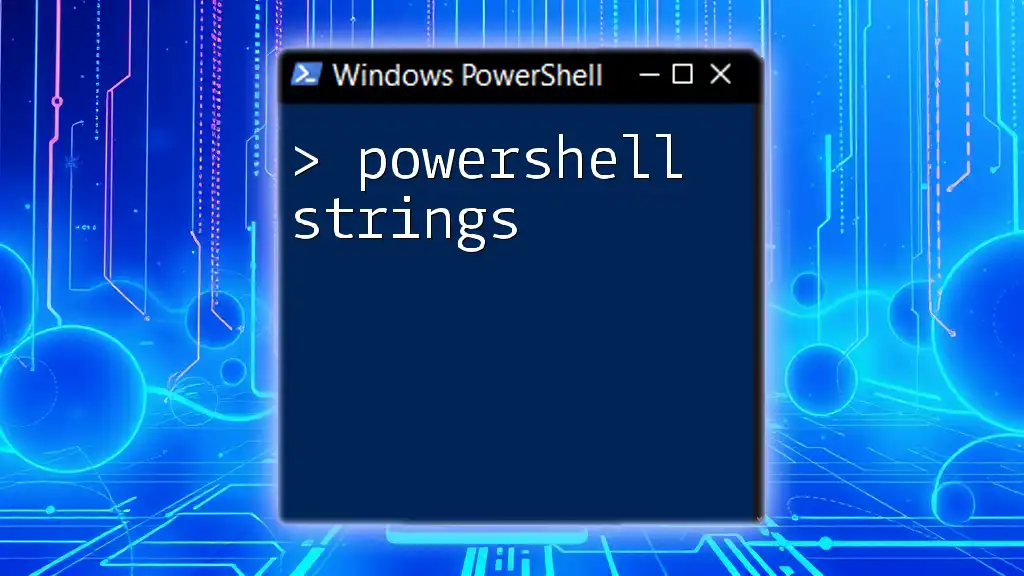
Conclusion
Summary of Key Points
Understanding how to convert a PowerShell string to an array using different methods, such as the `-split` operator and `.ToCharArray()` method, greatly enhances your ability to manipulate data. Efficiently utilizing arrays allows you to improve both the effectiveness of your scripts and the clarity of your code.
Additional Resources
For further reading and skill enhancement, consider exploring online courses, tutorials, and books that focus on PowerShell scripting and data manipulation techniques.