In PowerShell, you can append an item to an array by using the `+=` operator, which creates a new array with the additional element included.
$array = @(1, 2, 3)
$array += 4
Write-Host $array
Understanding Arrays in PowerShell
What is an Array?
In PowerShell, an array is a versatile data structure that holds a collection of items. Arrays can store a range of data types including strings, numbers, and even objects. One of their key characteristics is that they are zero-indexed, meaning the first item resides at index 0.
To initialize an empty array, use the following syntax:
$array = @()
This creates a new, blank array ready to hold values.
Types of Arrays in PowerShell
PowerShell supports various types of arrays:
- Single-dimensional arrays: These allow you to store a list of items in a single row.
- Multidimensional arrays: These are arrays that can hold data in a tabular format, giving you the ability to store data in multiple dimensions.
Here’s an example of defining both types:
# Single-dimensional array
$singleDimArray = @(1, 2, 3)
# Multidimensional array
$multiDimArray = @(@(1, 2), @(3, 4))
Understanding these types will help you effectively manage and manipulate collections in your scripts.
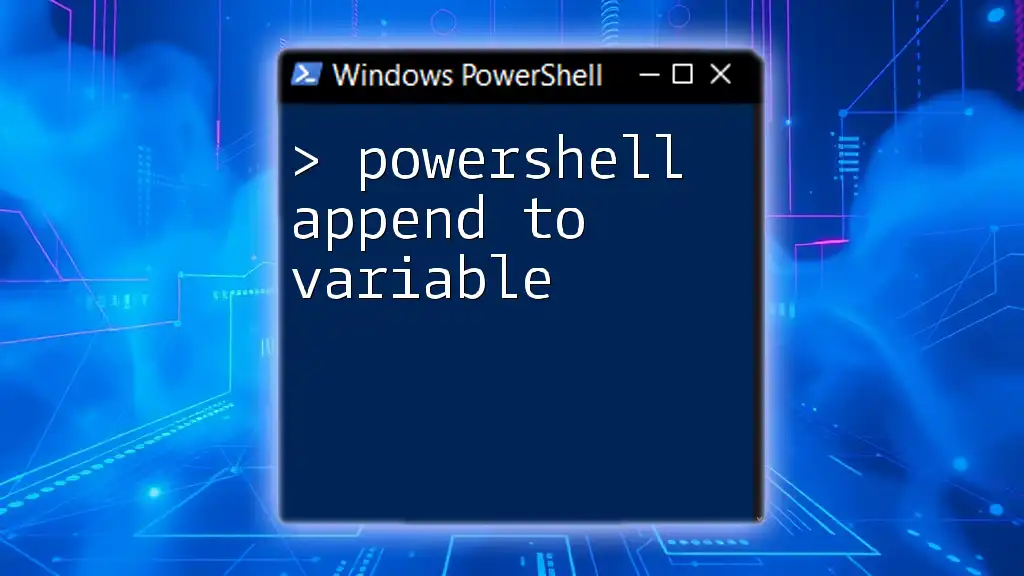
Appending Items to an Array
PowerShell Array Append Basics
Appending items to an array is a common task in PowerShell. There are several methods to achieve this, and each comes with its advantages and disadvantages. Knowing when to use each method is crucial for effective scripting.
Using `+=` to Add Items
One of the simplest ways to append items to an array is by using the `+=` operator. This method allows you to quickly add a new value to an existing array.
Understanding the `+=` Operator
The `+=` operator is intuitive but can be inefficient with large datasets because it creates a new array each time you append an item. Here's a basic example:
$array = @()
$array += 'Item 1'
$array += 'Item 2'
In this example, `Item 1` and `Item 2` are added sequentially to the array. While this method is readable, keep in mind that it may lead to performance limitations if used in tight loops or with large arrays.
Using `ArrayList` for Better Performance
If you expect to modify your array frequently, consider using `ArrayList` instead. This dynamic data structure provides improved performance for scenarios that require the addition of many items.
What is an ArrayList?
An ArrayList can dynamically adjust its size, making it a better alternative when frequently adding elements. It is part of the .NET framework and can be created easily in PowerShell.
Example of Creating and Using an ArrayList
To create an ArrayList, use the following command:
$arrayList = New-Object System.Collections.ArrayList
$arrayList.Add('Item A')
$arrayList.Add('Item B')
In this case, by using the `Add` method, we can efficiently append items without the overhead of creating a new structure each time.
Alternative Methods to Add Items
Using the `Add()` Method with an ArrayList
Utilizing the `Add()` method with an ArrayList is a straightforward way to append new values. For example:
$arrayList.Add('Item C')
This approach allows for efficient addition of new items directly to the ArrayList.
Using a Loop to Add Multiple Items
If you need to append multiple items at once, utilizing a loop is effective:
$itemsToAdd = @('Item D', 'Item E', 'Item F')
foreach ($item in $itemsToAdd) {
$arrayList.Add($item)
}
This method not only keeps your code concise but also enhances readability by clearly showing the intent to add multiple items.
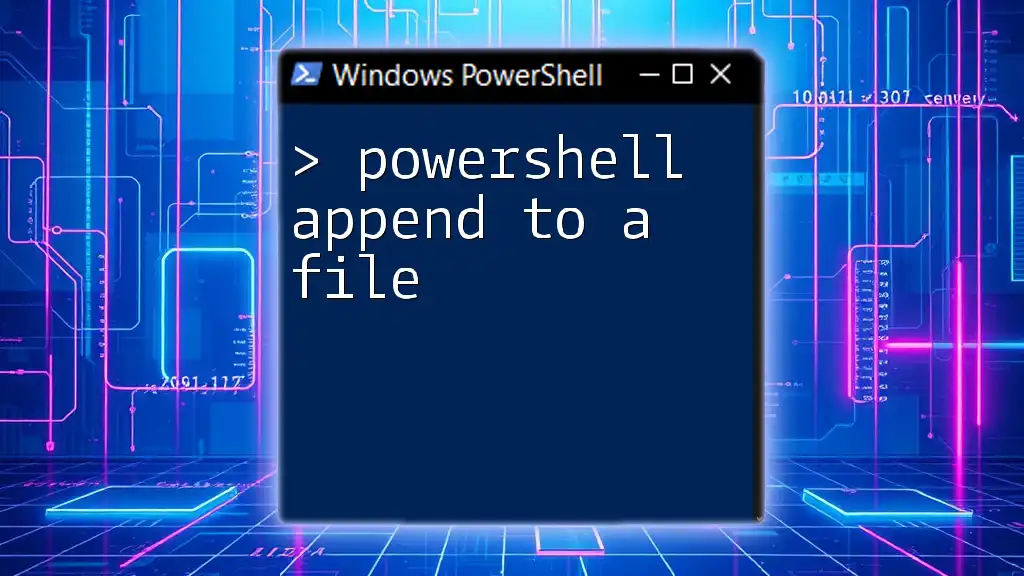
Best Practices for Appending to Arrays in PowerShell
Avoiding Common Caveats
When appending to arrays, particularly with the `+=` operator, ensure you are aware of its potential downsides. If not monitored carefully, it can lead to reduced performance in scripts that involve extensive data manipulation.
Choosing the Right Data Structure
Deciding between an array and an ArrayList requires careful consideration of your script's requirements. Use arrays for small, fixed-size datasets, while `ArrayLists` are ideal for scenarios involving frequent modifications. The following pros and cons can guide your choice:
-
Arrays:
- Pros: Fixed size, easy to work with for small sets of data.
- Cons: Inefficient for dynamic data resizing, especially with the `+=` operator.
-
ArrayLists:
- Pros: Dynamic sizing, better performance for numerous modifications.
- Cons: Slightly more complex syntax and requires understanding the .NET object model.
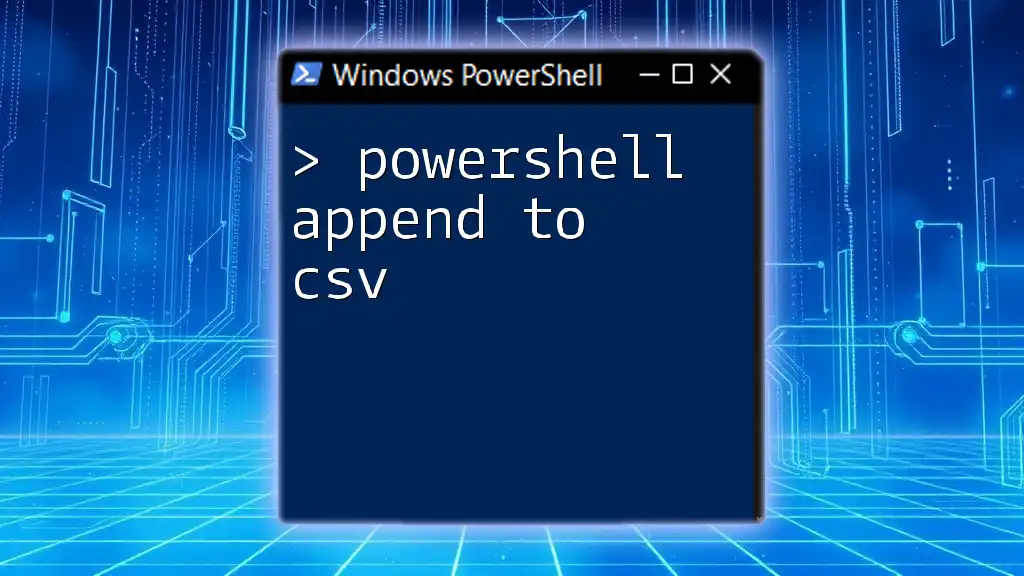
Conclusion
In summary, understanding how to effectively append to arrays in PowerShell is essential for managing collections efficiently. Whether you choose to use the `+=` operator for simple tasks or switch to an ArrayList for enhanced performance, the key is knowing the strengths of each approach. By practicing these methods, you can build powerful scripts that handle data with ease.
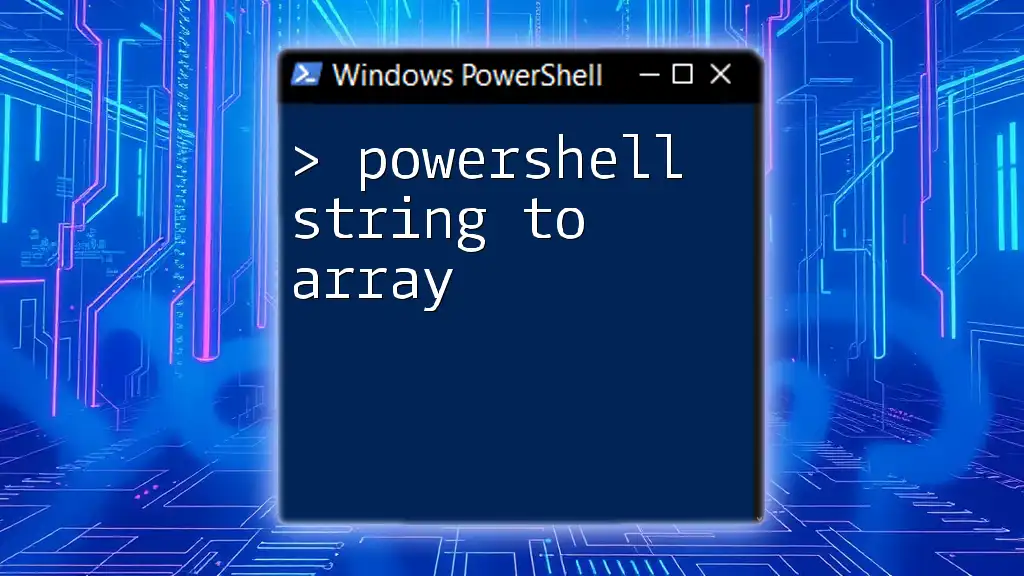
Further Resources
To deepen your understanding, consult the official PowerShell documentation and consider exploring online tutorials for practical exercises on array manipulation.
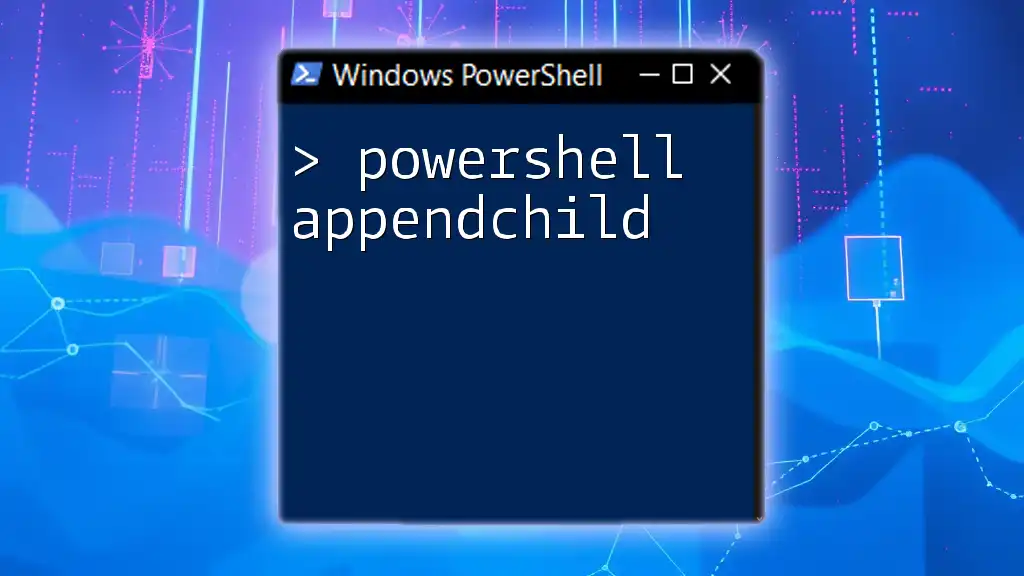
FAQs
Can I use `+=` to append to a multi-dimensional array?
Yes, you can use the `+=` operator to add elements to a multi-dimensional array, but keep in mind that it may not be the most efficient method for larger datasets.
Is there a limit to the size of an array in PowerShell?
While PowerShell does not impose strict limitations on array sizes, performance can be impacted as data volume increases, especially with methods like `+=`.
How do I remove an item from an array?
To remove an item from an array, you typically create a new array that omits the unwanted item or use filtering techniques with `Where-Object`. Consistency and clarity in your coding practices will aid in effective data management.