A PowerShell dynamic array is a flexible collection that can automatically adjust its size to accommodate new elements, allowing for efficient data management during script execution.
Here’s a simple code snippet demonstrating how to create and add elements to a dynamic array:
# Initialize a dynamic array
$dynamicArray = @()
# Add elements to the dynamic array
$dynamicArray += "First Item"
$dynamicArray += "Second Item"
$dynamicArray += "Third Item"
# Display the contents of the dynamic array
$dynamicArray
Understanding Arrays in PowerShell
What are Arrays?
Arrays are fundamental data structures in programming used to store multiple values in a single variable. In PowerShell, arrays can hold a collection of items, which can be of similar or varied data types. A significant distinction between arrays is that static arrays have a fixed size, while dynamic arrays can grow and shrink in size based on the elements they contain.
Importance of Dynamic Arrays
Dynamic arrays offer flexibility not found in static arrays. When scripting with PowerShell, utilizing dynamic arrays allows you to:
- Easily add or remove items at runtime based on conditions or inputs.
- Handle various data types, from strings and integers to objects.
- Implement operations that require a changing dataset, making your scripts more robust and adaptable.

Creating Dynamic Arrays in PowerShell
Initializing Dynamic Arrays
Initializing a dynamic array in PowerShell is simple. You can create an empty array using the `@()` syntax:
$dynamicArray = @()
This creates an empty dynamic array ready to store your data.
Adding Elements to Dynamic Arrays
Adding elements to a dynamic array is straightforward. You can use the `+=` operator, which allows you to append new items:
$dynamicArray += "First Element"
$dynamicArray += "Second Element"
With each addition, PowerShell automatically handles resizing the array to accommodate the new element.
Handling Different Data Types
One of the powerful features of dynamic arrays is their ability to store mixed data types. You can easily include numbers, strings, booleans, or even objects in a single array:
$mixedArray = @("String", 123, $true)
This flexibility makes dynamic arrays particularly useful for scripts that require varied data inputs.

Modifying Dynamic Arrays
Removing Elements from Dynamic Arrays
To remove elements from a dynamic array, there are multiple approaches. One common method is to use the `Where-Object` cmdlet to filter out unwanted items:
$dynamicArray = $dynamicArray | Where-Object { $_ -ne "First Element" }
This command effectively removes the specified element by reassigning the filtered array back to `$dynamicArray`.
Updating Elements in Dynamic Arrays
Updating existing elements within a dynamic array is straightforward. You can simply assign a new value to a specific index:
$dynamicArray[0] = "Updated Element"
This allows you to maintain dynamic arrays that reflect the most current data needed for your tasks.

Accessing Elements in Dynamic Arrays
Index-Based Access
Accessing elements in a dynamic array is done using their index, which is zero-based. For example, to retrieve the first element:
$firstElement = $dynamicArray[0]
This indexing method allows for quick access to any item in your dynamic array.
Looping Through Dynamic Arrays
You can loop through the elements of a dynamic array using a `foreach` loop. This is particularly useful for processing or displaying each element:
foreach ($element in $dynamicArray) {
Write-Host $element
}
Employing loops effectively allows you to handle each element according to your needs.
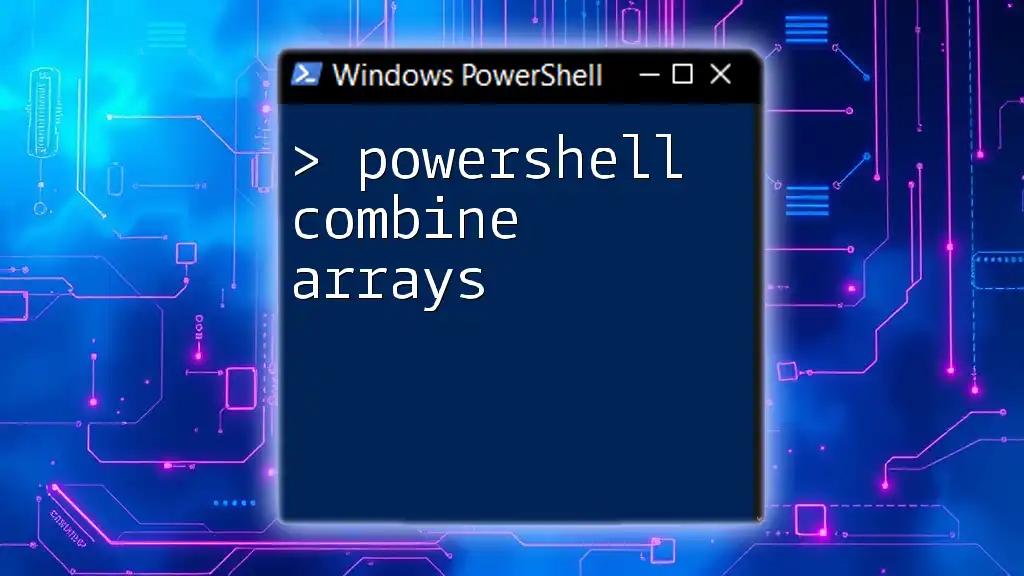
Advanced Techniques with Dynamic Arrays
Filtering Dynamic Arrays
PowerShell provides powerful filtering capabilities through cmdlets like `Where-Object`. You can create filtered versions of your dynamic arrays based on certain conditions:
$filteredArray = $dynamicArray | Where-Object { $_ -like "*Element*" }
In this example, the filtered array will only contain elements that match the specified condition.
Sorting Dynamic Arrays
Sorting elements within a dynamic array can be achieved with the `Sort-Object` cmdlet. This is essential when you need your data organized in a specific order:
$sortedArray = $dynamicArray | Sort-Object
Using Dynamic Arrays with Objects
Dynamic arrays become exceptionally powerful when working with objects. You can create arrays that hold custom objects, enhancing the richness of your data structures:
$people = @()
$people += [PSCustomObject]@{Name="Alice"; Age=30}
$people += [PSCustomObject]@{Name="Bob"; Age=25}
This capability allows for structured data management, particularly useful in real-world scripting scenarios like managing user information or system configurations.
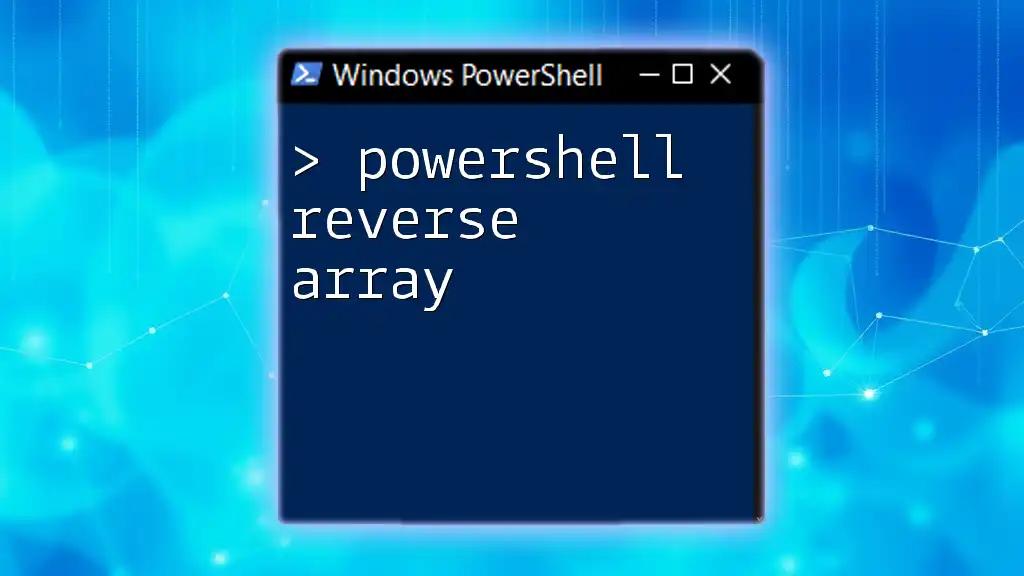
Best Practices for Using Dynamic Arrays
Performance Considerations
While dynamic arrays provide great flexibility, it's important to be aware of performance implications. Frequent resizing due to extensive addition or removal of elements can affect performance. When possible, initialize your array with an estimated size or batch add elements.
When to Use Dynamic Arrays
Dynamic arrays are ideal when dealing with datasets where the size is uncertain. Use dynamic arrays when:
- You expect to add and remove data frequently.
- You need to manage collections of varying data types or structures.
- The order and organization of data are vital to your scripting tasks.
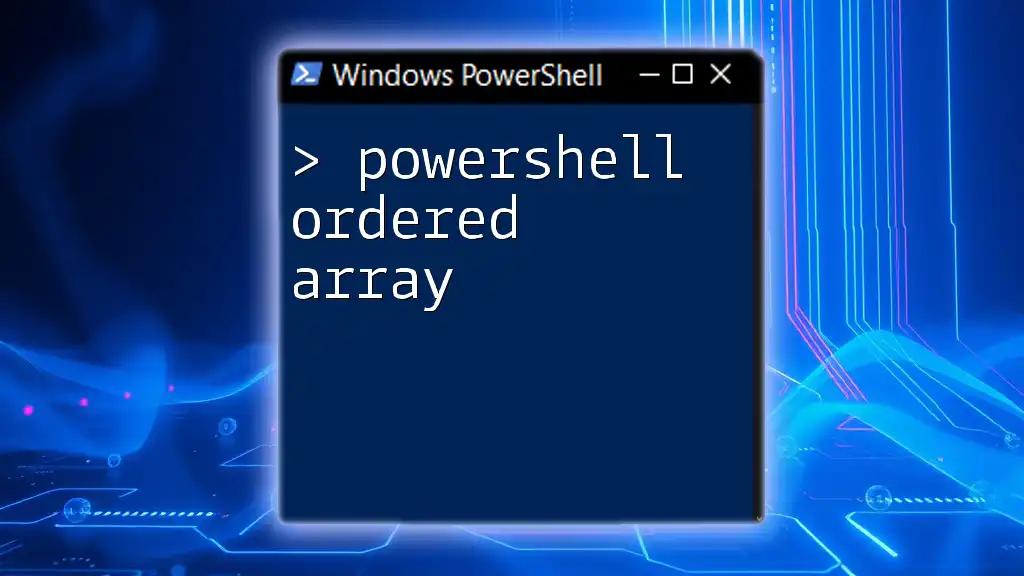
Common Errors and Troubleshooting
Common Issues with Dynamic Arrays
Misunderstandings when using dynamic arrays often arise from index misuse, such as trying to access an index that doesn't exist or misconceptions about how to remove items.
Debugging Tips
When troubleshooting dynamic array issues, consider using `Write-Host` to output the current state of your array after operations. This can help track changes and diagnose problems efficiently.
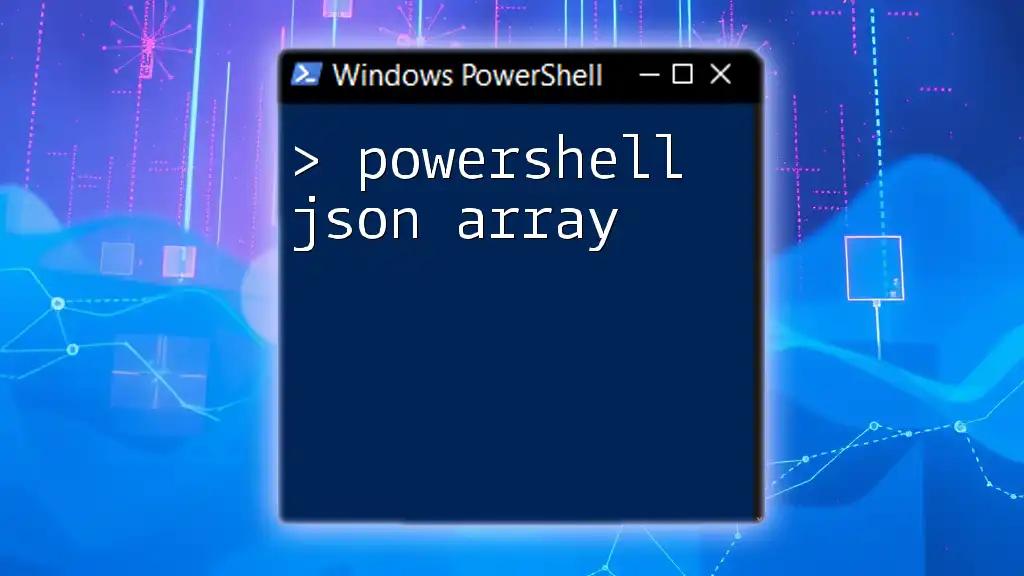
Conclusion
In this guide, we've explored the PowerShell dynamic array, understanding its significance, creation, modification, and advanced techniques. Dynamic arrays empower your scripting capabilities, enabling you to write more flexible and powerful scripts.
Explore using dynamic arrays in your scripts, and embrace the versatility they offer in your PowerShell journey. Remember, practice is key to mastering these concepts, so experiment with dynamic arrays in various contexts to deepen your understanding.