A PowerShell enumerator is a type of collection that allows you to iterate over a set of items, providing methods to access each element in a sequential manner.
Here's a simple code snippet demonstrating how to use a `foreach` loop to enumerate through an array of numbers in PowerShell:
$numbers = 1..5
foreach ($number in $numbers) {
Write-Host "Number: $number"
}
Understanding PowerShell Enumeration
What is Enumeration?
Enumeration is a fundamental concept in programming that refers to the process of collecting a number of items or values. In PowerShell, enumeration allows users to iterate through collections of objects, whether they are files, processes, or services. It helps automate tasks by enabling scripts to dynamically respond to the current state of the system or environment.
Significance of Enumerating Objects in PowerShell
Enumerators play a crucial role in enhancing productivity and efficiency when managing systems. By utilizing enumerators, PowerShell users can easily manipulate large datasets, filter data according to specific criteria, and generate actionable insights from those datasets.
For example, an IT professional may need to audit which services are currently running on a server. Using PowerShell enumeration, they can harness the power of scripts to streamline this task, saving countless hours over manual processing.

Common Use Cases for PowerShell Enumeration
Enumerating Files and Directories
One of the most common uses of enumeration in PowerShell is traversing the file system. The `Get-ChildItem` cmdlet is perfect for retrieving files and directories recursively. It provides a structured view of files, allowing users to apply filters and extract useful information.
Example Code Snippet:
Get-ChildItem -Path "C:\MyFolder" -Recurse
In this command, PowerShell lists all files and directories under “C:\MyFolder” and its subfolders. The output includes various properties such as name, length, and last modified date, allowing for easy assessment of file structures.
Enumerating Processes
Another powerful utility of the PowerShell enumerator is in managing processes. The `Get-Process` cmdlet retrieves a list of all processes running on the system.
Example Code Snippet:
Get-Process | Select-Object Name, Id, CPU
This snippet provides a concise listing of processes, highlighting the name, process ID, and CPU usage. This data is invaluable when monitoring system resources and troubleshooting performance-related issues.
Enumerating Services
Services are essential components of Windows operating systems. To effectively manage these services, PowerShell offers the `Get-Service` cmdlet, which enumerates services currently running on the system.
Example Code Snippet:
Get-Service | Where-Object { $_.Status -eq 'Running' }
In this example, only running services are displayed. This kind of focused enumeration is especially beneficial for system administrators looking to quickly assess the operational status of services.
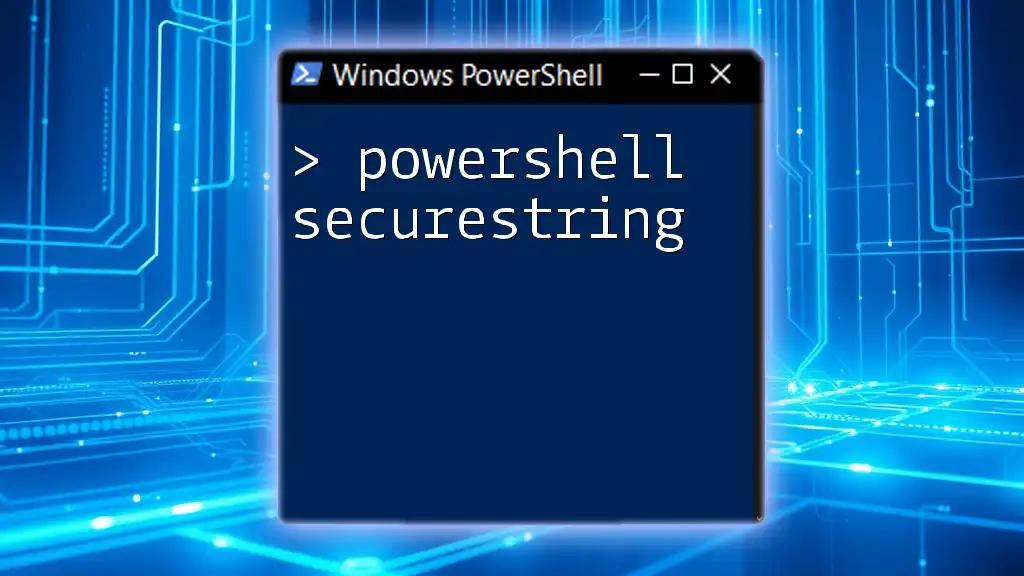
Creating Custom Enumerators in PowerShell
The Basics of Custom Enumerators
A custom enumerator allows developers to define how collections of data should be traversed and managed. This approach is particularly useful when working with complex data structures or when the built-in enumerators do not meet specific needs.
Step-by-Step Guide to Creating a Custom Enumerator
To create a custom enumerator in PowerShell, one must define a class that implements the `IEnumerator` interface. The class manages the current position within the collection and provides mechanisms to navigate through it.
Example Code Snippet:
class MyEnumerator {
[int]$CurrentIndex = -1
[array]$Items
MyEnumerator([array]$items) {
$this.Items = $items
}
[bool] MoveNext() {
$this.CurrentIndex++
return $this.CurrentIndex -lt $this.Items.Count
}
[object] Current {
return $this.Items[$this.CurrentIndex]
}
}
In this code, `MyEnumerator` is a simple implementation of an enumerator. It holds an array of items and includes methods to move through the items and return the current item. This flexibility allows developers to tailor the iteration process to their specific needs.

Advanced Enumeration Techniques
Using PowerShell's `ForEach-Object`
PowerShell's `ForEach-Object` cmdlet enhances enumeration by allowing users to process items as they pass through a pipeline. This technique is invaluable for applying operations to every element in a collection.
Example Code Snippet:
Get-Process | ForEach-Object { $_.Name }
In this example, each running process's name is printed. The use of `ForEach-Object` significantly simplifies manipulation of data and can be customized further with additional logic within the script block.
Creating Pipelines for Efficient Enumeration
PowerShell pipelines are a powerful feature that permits the chaining of cmdlets. By sending the output of one cmdlet as input to another, users can create sophisticated enumeration processes.
Example Code Snippet:
Get-Service | Where-Object { $_.Status -eq 'Stopped' } | Sort-Object DisplayName
In this snippet, services that are stopped are filtered and sorted by their display names in a single, concise statement. Pipelines reduce the need for intermediate variables and enhance code readability.
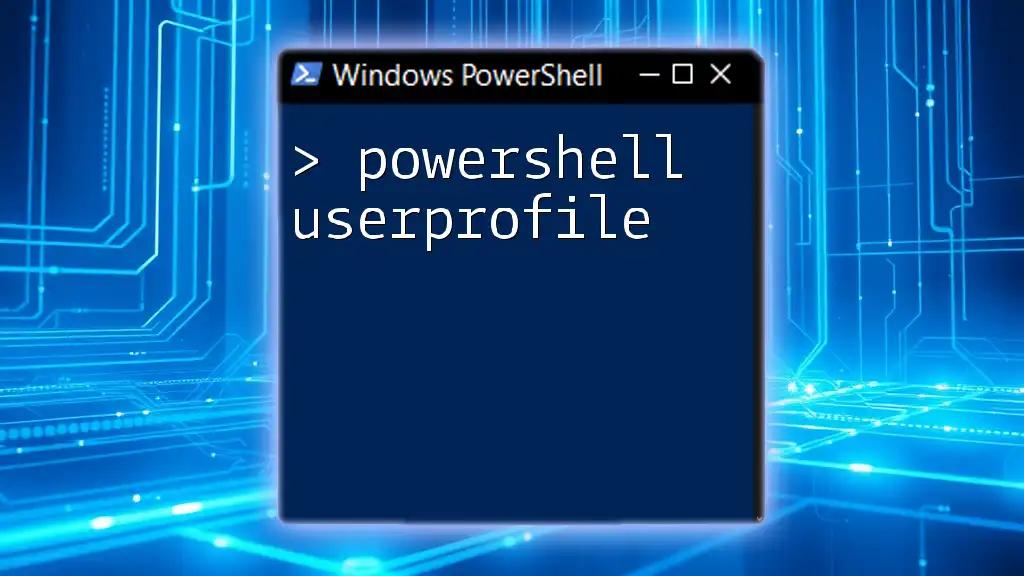
Best Practices for PowerShell Enumeration
Performance Considerations
To optimize performance during enumeration, consider the scope of data you are querying. Always filter results as early as possible to limit the amount of data being processed. This can drastically reduce execution time, especially when working with large datasets.
Handling Errors and Exceptions in Enumeration
Error management is essential for creating robust PowerShell scripts. Common pitfalls include attempting to enumerate non-existent objects or running into permission issues. Using `Try-Catch` blocks allows for graceful error handling.
Example Code Snippet:
Try {
Get-ChildItem -Path "C:\NonExistentFolder"
} Catch {
Write-Host "An error occurred: $_"
}
In this example, if the specified folder does not exist, the script captures the exception and outputs a friendly error message, preventing abrupt script termination and improving the user experience.
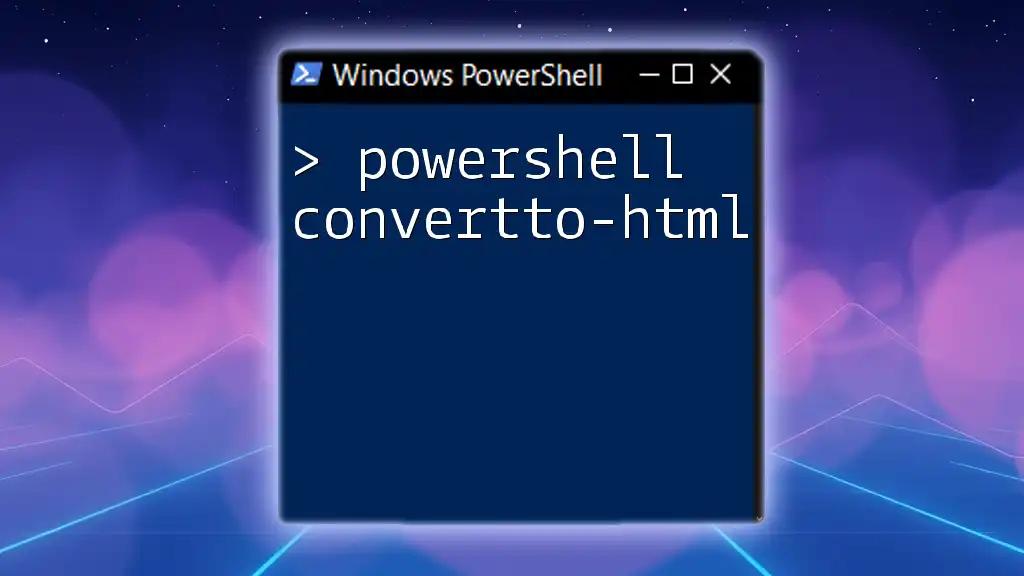
Conclusion
PowerShell enumerators are a powerful instrument in the toolkit of anyone working with automation and system management. By mastering the skills of enumeration, users can significantly enhance their efficiency and effectiveness in various tasks. From enumerating files and services to creating custom enumerators, the possibilities are vast. Embracing these techniques leads to a deeper understanding of PowerShell's capabilities and equips professionals and enthusiasts alike with the tools necessary to conquer complex challenges.
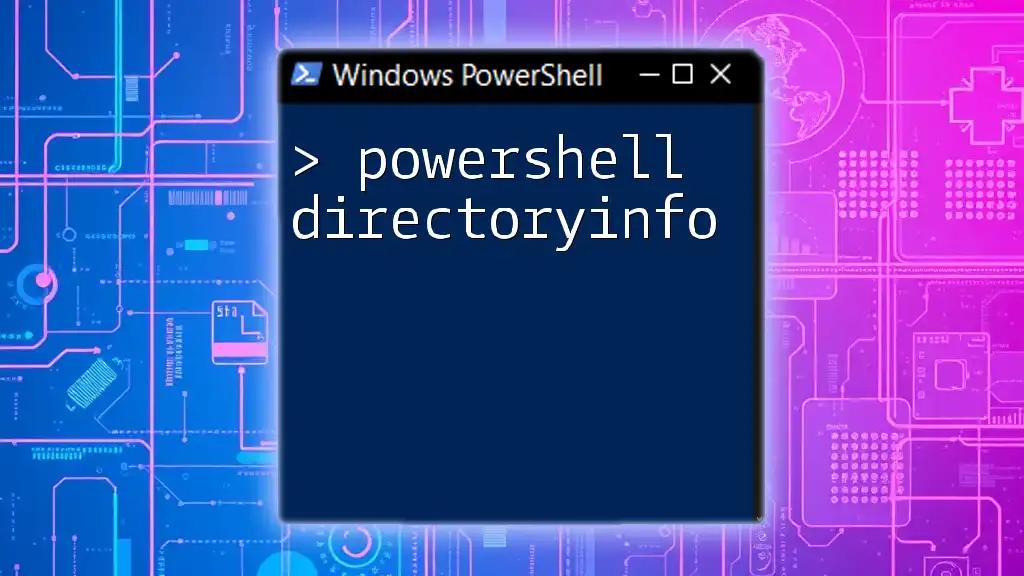
Additional Resources for Learning PowerShell
For those eager to expand beyond this guide, various resources are available for furthering your knowledge of PowerShell. Recommended books, online tutorials, and community forums can provide a wealth of information for continuous learning and mastery of PowerShell. Whether you're a beginner or looking to deepen your expertise, the journey is just beginning.