The PowerShell ternary operator simplifies conditional logic by allowing you to evaluate a condition and return one of two values based on whether the condition is true or false; here’s a basic example:
$result = $condition ? 'True Value' : 'False Value'
This code assigns 'True Value' to `$result` if `$condition` is true, and 'False Value' otherwise.
What is a Ternary Operator in PowerShell?
The ternary operator is a useful shorthand for conditional expressions in PowerShell. It allows you to evaluate a condition and return one of two values based on whether the condition evaluates to true or false. The syntax for the ternary operator in PowerShell is as follows:
condition ? true_value : false_value
Using this operator can significantly streamline your scripts. For instance, instead of writing multiple lines of code with if-else statements, you can condense it into a single line with the ternary operator. This can enhance not only the efficiency of your scripts but also their readability.
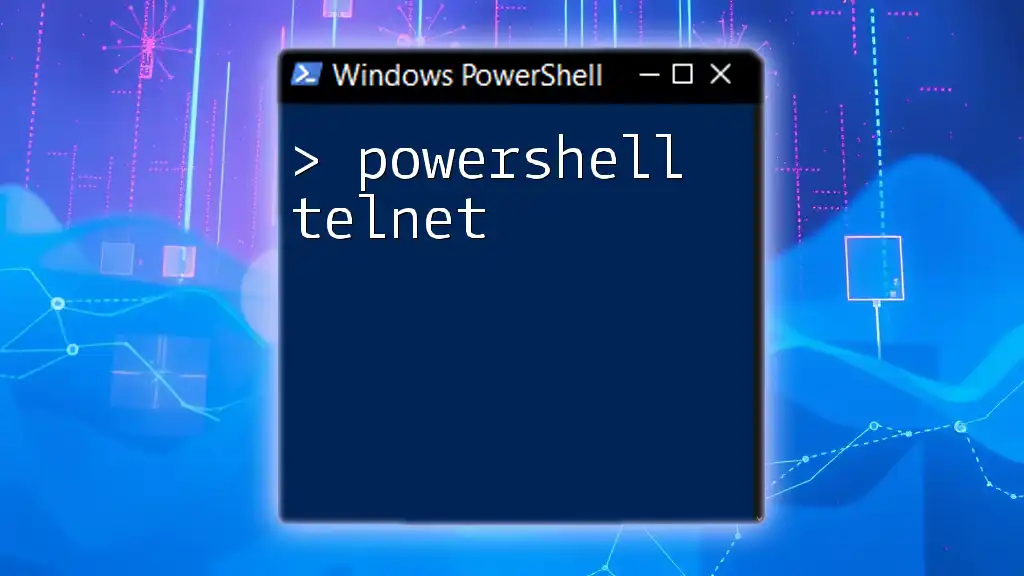
Understanding the Syntax of the Ternary Operator in PowerShell
Basic Structure
Understanding the structure is crucial for implementing the PowerShell ternary operator effectively. The operator requires three major components: a condition to evaluate, a value to return if this condition is true, and another value to return if the condition is false. Here’s a simple example to illustrate this:
$result = ($a -gt $b) ? "A is greater" : "B is greater"
In this code snippet:
- `$a -gt $b` is the condition being checked.
- If `$a` is greater than `$b`, the script returns "A is greater".
- Otherwise, it returns "B is greater".
Condition Evaluation
The ternary operator can evaluate a variety of conditions, leveraging PowerShell's robust comparison and logical operators such as `-eq`, `-lt`, `-gt`, `-and`, and `-or`. This flexibility allows you to create complex evaluations succinctly.
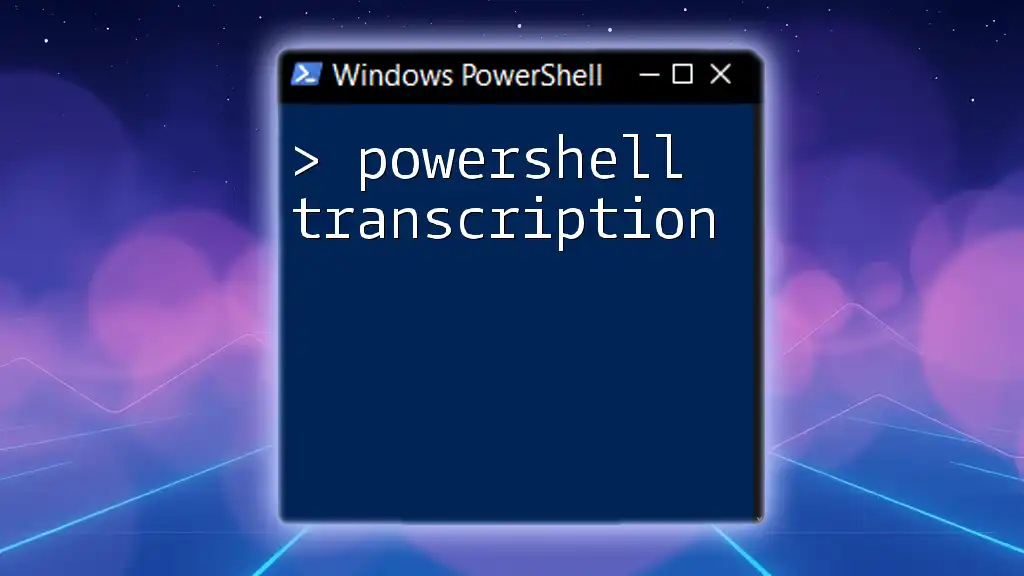
Practical Examples of Using the Ternary Operator
Example 1: Simple Comparison
Let’s look at a straightforward example using the PowerShell ternary operator:
$num = 10
$result = ($num -gt 5) ? "Greater than 5" : "5 or less"
Write-Output $result
In this code:
- `$num` is assigned the value 10.
- The condition checks if `$num` is greater than 5. Since it is true, the output will be "Greater than 5".
Example 2: Nested Ternary Operators
You can also nest ternary operators for more complex evaluations. Here’s an example:
$value = 10
$result = ($value -gt 10) ? "Greater than 10" : ($value -eq 10 ? "Equals 10" : "Less than 10")
Write-Output $result
In this scenario:
- If `$value` is greater than 10, it returns "Greater than 10".
- If `$value` equals 10, it returns "Equals 10".
- If neither condition applies, it will state "Less than 10".
Example 3: Ternary Operator in Function
You can encapsulate the ternary operator within functions to improve code modularity and reusability. Here’s how you can construct a simple function:
function Check-Positive {
param ($number)
return ($number -ge 0) ? "Positive" : "Negative"
}
This function checks whether a given number is positive or negative, returning the appropriate string based on the evaluation of the condition passed into the `param`.
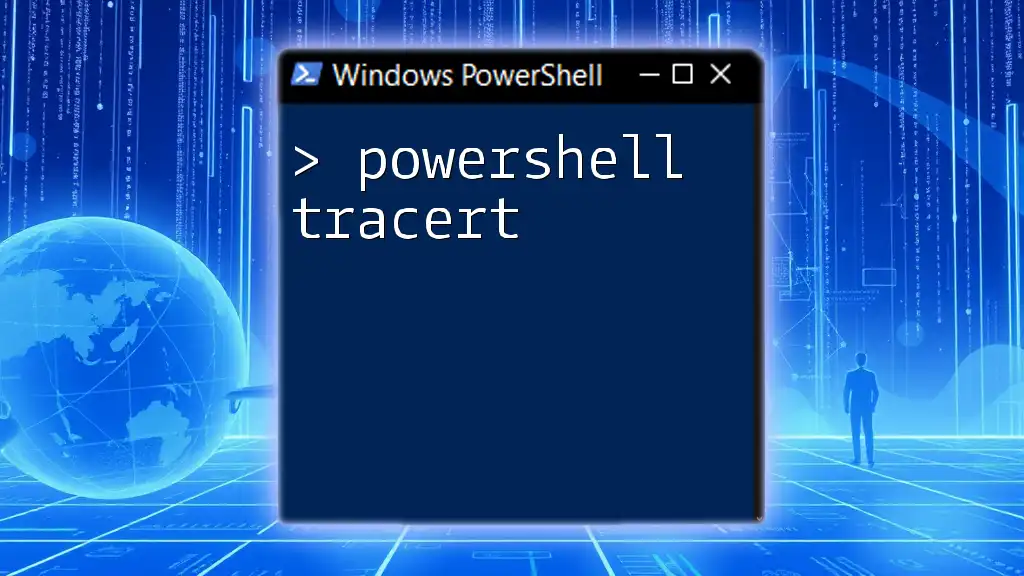
Advantages of Using the Ternary Operator in PowerShell
Conciseness and Clarity
Utilizing the ternary operator leads to more concise code. Instead of writing lengthy if-else statements, a single line can achieve the same result. This promotes clarity within your scripts, making them easier to read and understand at a glance.
For example, compare these two snippets of code:
Using if-else:
if ($age -ge 18) {
Write-Output "Adult"
} else {
Write-Output "Minor"
}
Using the ternary operator:
$result = ($age -ge 18) ? "Adult" : "Minor"
Write-Output $result
The second example is noticeably cleaner and more succinct.
Readability
While the ternary operator improves conciseness, it is also essential for readability. Properly using the ternary operator can enhance script readability, but overuse or improper nesting can lead to confusion. Always balance the use of this operator with clear conditional logic to ensure that anyone reading the code can easily grasp its function.
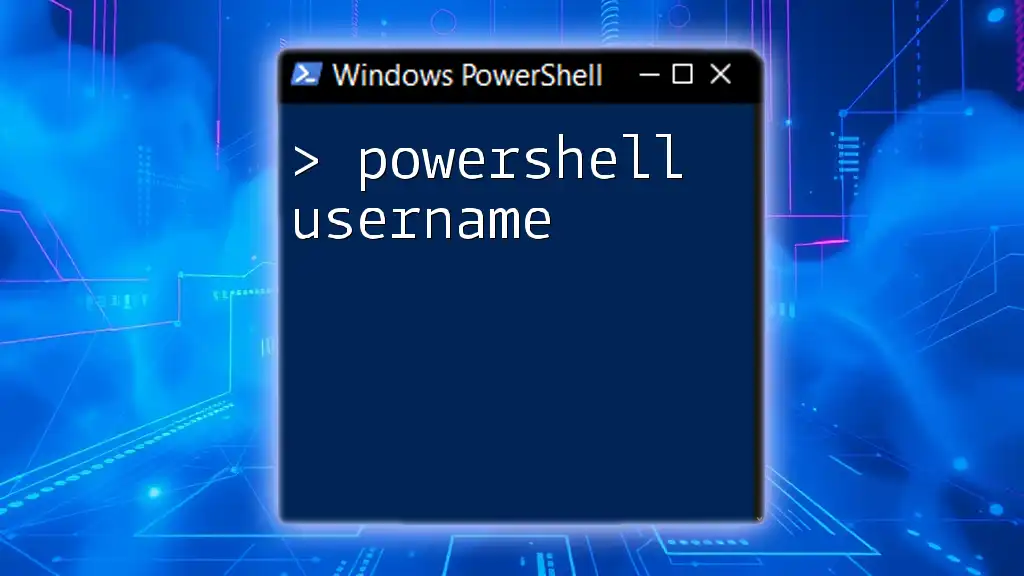
Common Pitfalls and Best Practices
What to Avoid
Avoid excessive nesting of ternary operators as it can lead to convoluted and difficult-to-read code. Each nested layer can reduce clarity and make debugging more challenging.
Best Practices
- When to Use: Use the ternary operator for simple conditions or where brevity improves clarity.
- When to Avoid: Reserve traditional if-else constructs for more complex logic to maintain readability and comprehension.
- Combine Conditions Wisely: Use logical operators for combining conditions without cluttering the code.
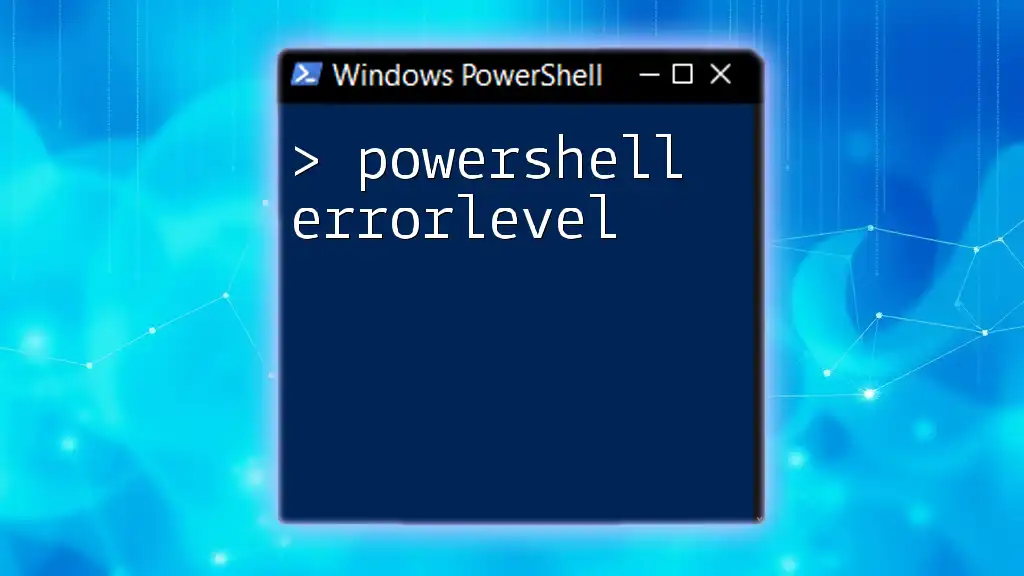
Conclusion
The PowerShell ternary operator is a powerful tool that can help streamline your scripts and enhance readability. With its concise syntax, it allows you to evaluate conditions efficiently and return values based on those evaluations. By practicing its usage in various scenarios, you'll gain confidence and improve your coding fluency in PowerShell. Use the examples and guidelines provided in this guide to master the innovative potential of the ternary operator, integrating it seamlessly into your scripting repertoire.
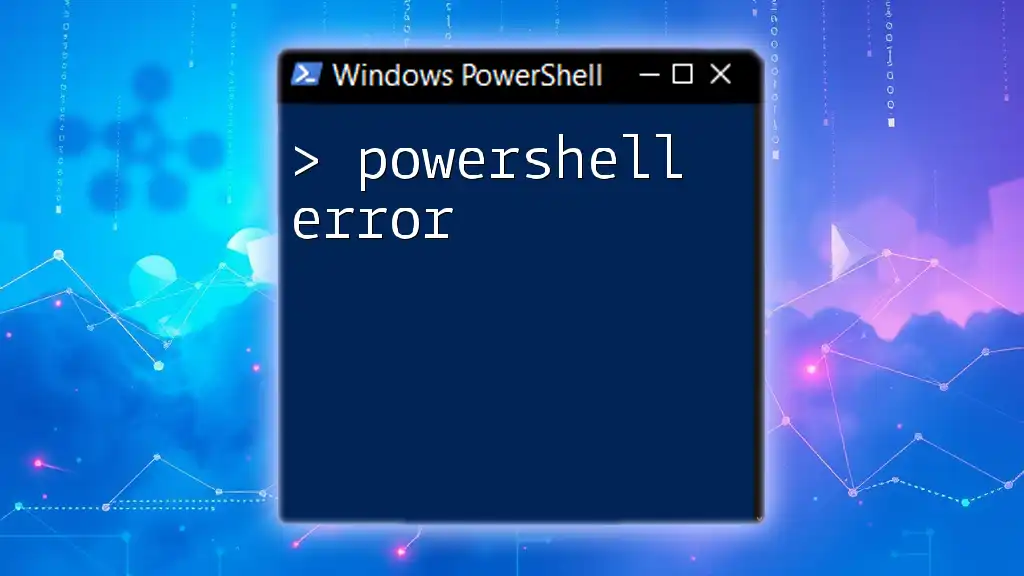
Additional Resources
For further exploration of the PowerShell ternary operator and other essential commands, consider checking out the official PowerShell documentation and community forums. These resources will provide you with a deeper understanding and additional insights into effectively leveraging PowerShell's capabilities.
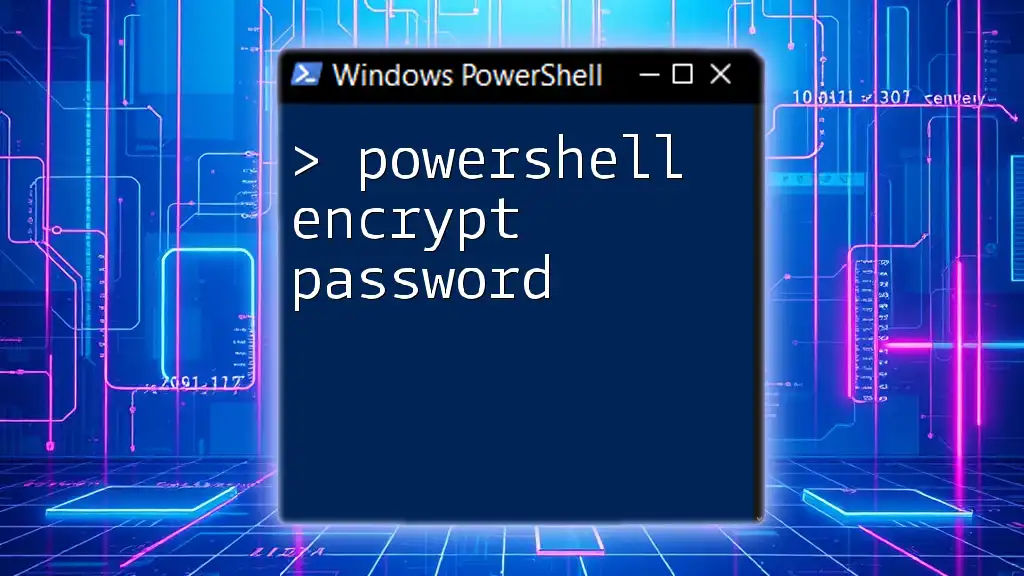
Call to Action
Don’t stop here! Explore more posts on PowerShell commands and consider signing up for a newsletter or course on advanced PowerShell scripting. Your journey to mastering PowerShell starts now!