PowerShell's `Replace` method allows you to substitute specific characters or strings within a larger string, enhancing text manipulation efficiency.
Here's a code snippet demonstrating how to use the `Replace` method in PowerShell:
$originalString = "Hello, World!"
$modifiedString = $originalString.Replace("World", "PowerShell")
Write-Host $modifiedString # Output: Hello, PowerShell!
Understanding the Replace Method
What is the Replace Method?
The `.Replace` method in PowerShell is an essential function used for manipulating strings. It allows you to search for a specific substring (or character) within a string and replace it with a different substring (or character), making it invaluable for various scripting tasks.
Syntax of the Replace Method
The basic syntax of the `.Replace` method is simple and intuitive:
$string.Replace("oldValue", "newValue")
- `oldValue`: The substring you want to replace within the original string.
- `newValue`: The substring that will replace the `oldValue`.
For example, if you have the string "Goodbye World" and you want to replace "Goodbye" with "Hello," your code would look like this:
$greeting = "Goodbye World"
$newGreeting = $greeting.Replace("Goodbye", "Hello")
In this case, `$newGreeting` will hold the value "Hello World."
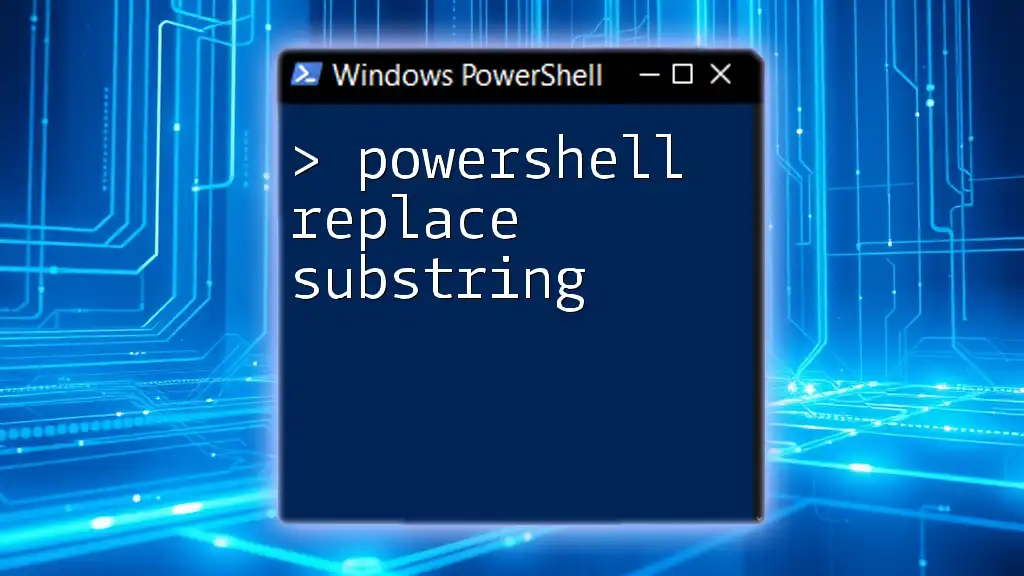
Practical Applications of PowerShell Replace
Replacing Text in Strings
A common use case for the `powershell replace` method is to alter specific text within a string. Here's an example where we replace a substring:
$originalString = "Hello World"
$newString = $originalString.Replace("World", "PowerShell")
The result stored in `$newString` will be "Hello PowerShell." This method is particularly useful for personalizing messages or transforming output strings dynamically.
Replacing Characters
The `.Replace` method can also be employed to replace specific characters. For instance, consider the following example:
$originalString = "Power$hell"
$newString = $originalString.Replace("$", "Shell")
In this code, the character `$` is replaced with the string "Shell," resulting in `$newString` holding the value "PowerShell." It’s critical to bear in mind that if the character being replaced does not exist in the string, the original string remains unchanged without throwing an error.
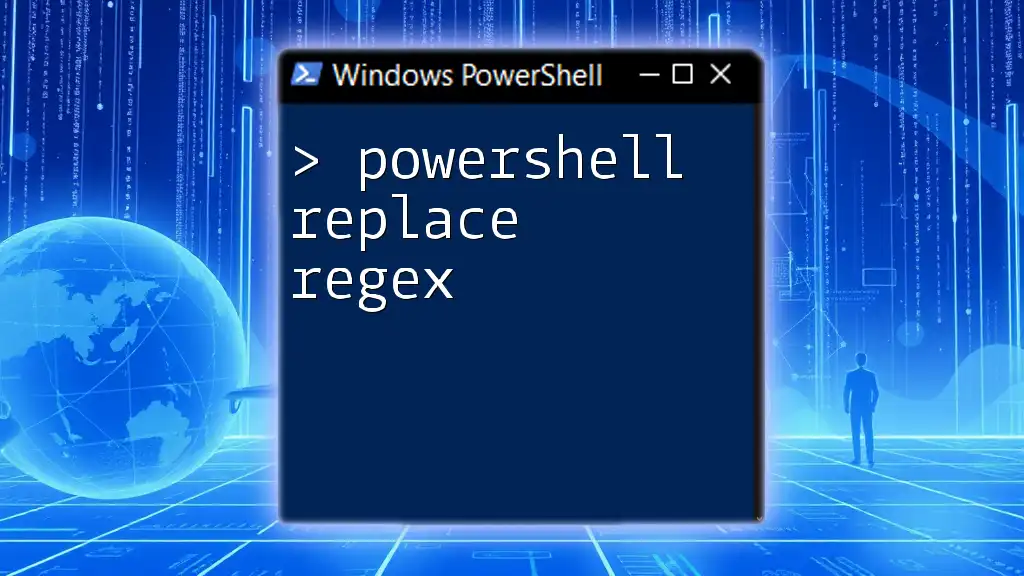
Advanced Usage of PowerShell Replace
Using Replace with Variables
Variables can add vital flexibility to your scripts, allowing dynamic replacements. Here’s how to implement it:
$old = "foo"
$new = "bar"
$string = "foo is not the same as foo"
$result = $string.Replace($old, $new)
In this scenario, every instance of "foo" in `$string` is replaced with "bar," so `$result` will be "bar is not the same as bar." Utilizing variables not only enhances readability but also simplifies future modifications to your script.
Replacing with Regular Expressions
For cases where you need more advanced pattern matching, PowerShell provides the `-replace` operator which allows the use of regular expressions. Regular expressions provide robust capability to find and replace complex patterns in text.
Here’s an example:
$string = "The quick brown fox jumps over the lazy dog."
$string -replace 'fox', 'cat'
Here, "fox" is replaced with "cat." The beauty of `-replace` lies in its ability to handle regex patterns, making it particularly powerful for tasks requiring intricate text manipulations.
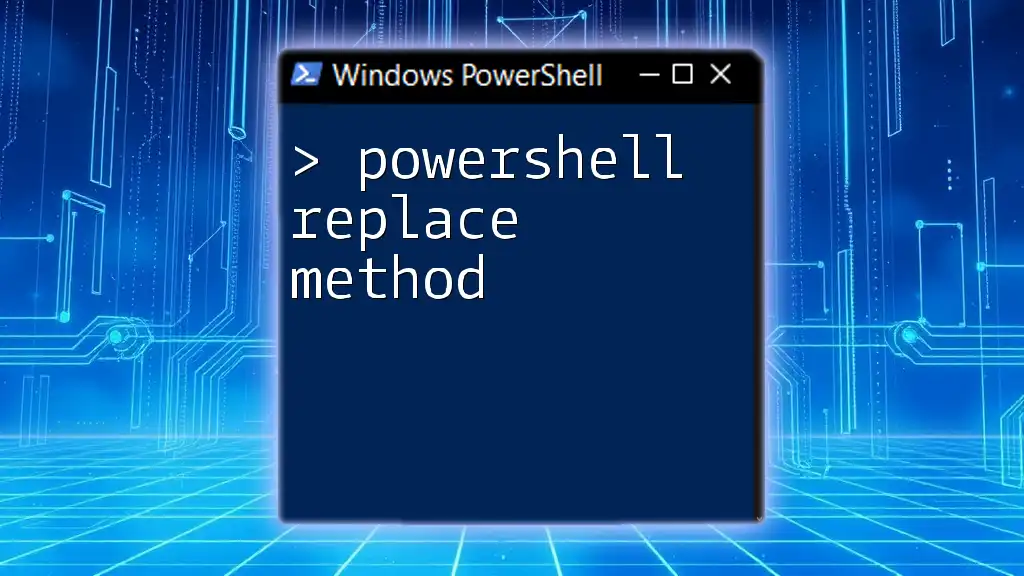
Common Errors and Troubleshooting
Understanding Errors in Replace Operations
Using the `.Replace` method may sometimes lead to unexpected behavior if certain factors are overlooked. For instance, keep in mind that the method is case-sensitive. Therefore, if you try to replace "hello" when the string says "Hello," nothing will happen.
Troubleshooting Common Issues
If you encounter issues where `.Replace` does not appear to work as intended, here are some steps to troubleshoot:
- Check for Case Sensitivity: Ensure that you're matching the exact casing.
- Leading/Trailing Spaces: Inspect your original string for any unintentional spaces.
- String Immutability: Remember, strings in PowerShell are immutable. The `.Replace` method does not modify the original string but returns a new one.
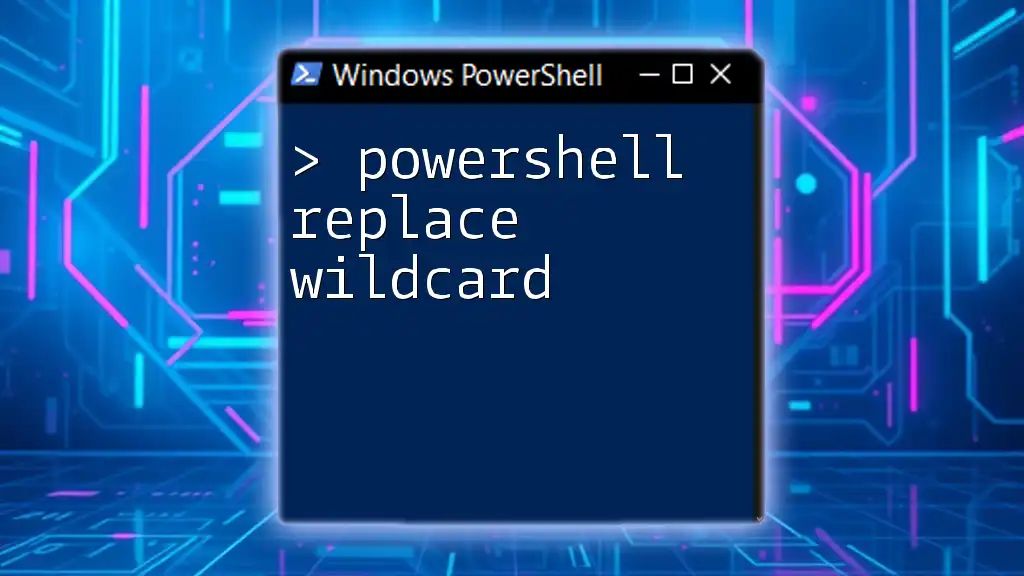
Best Practices for Using Replace in PowerShell
Tips for Efficient String Replacement
To optimize your string replacement operations, consider these practices:
- Utilize immutable strings wisely since they can enhance performance, especially in large scripts where many replacements may be necessary.
- If your replacements are done in a loop, structure them efficiently to minimize performance hits.
Performance Considerations
While `.Replace` is efficient for straightforward replacements, for larger datasets or more complicated patterns, consider using bulk replacements or even leveraging regular expressions for increased performance and control.
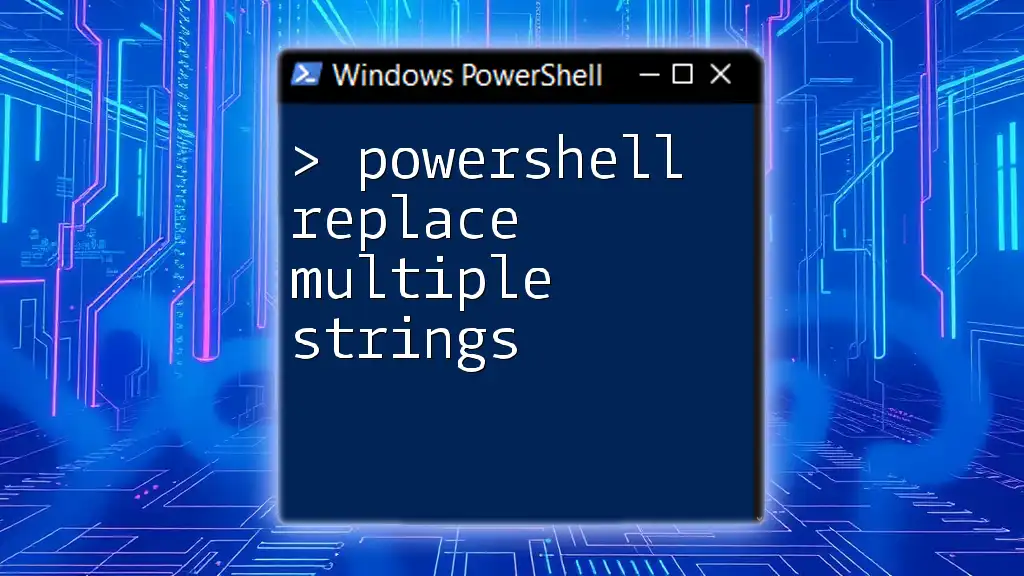
Conclusion
In summary, PowerShell replace capabilities are a fundamental part of scripting that enables dynamic string manipulation. Understanding the nuances of the `.Replace` method and the `-replace` operator will facilitate efficient string handling in your PowerShell scripts.
By practicing these methods and adhering to best practices, you'll enhance your scripting efficiency significantly, leading to more effective automation solutions.
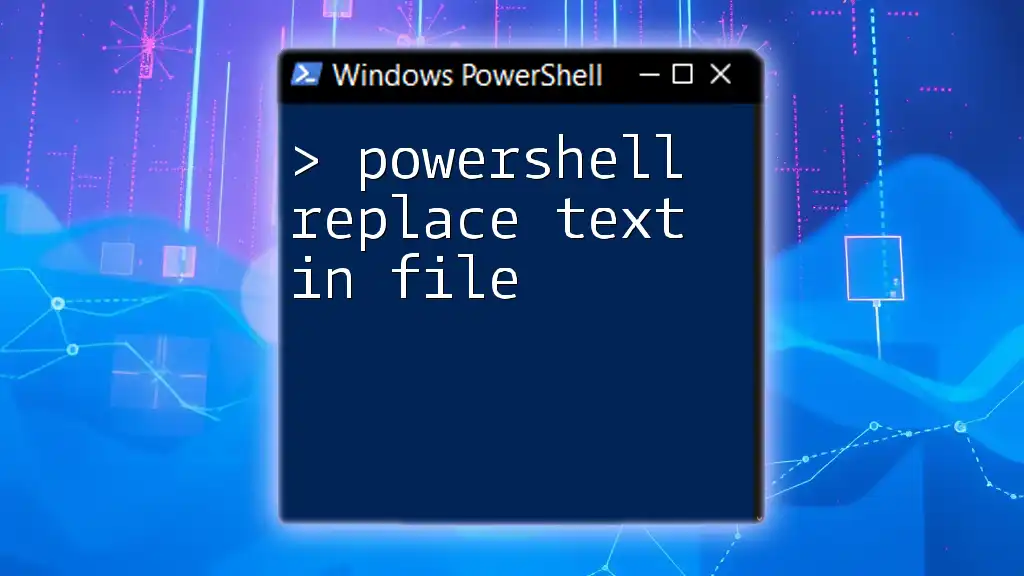
Further Reading and Resources
To continue your learning journey on string manipulation in PowerShell, visit the official PowerShell documentation. Exploring online platforms that offer tutorials or courses can also significantly enhance your understanding of scripting in PowerShell.
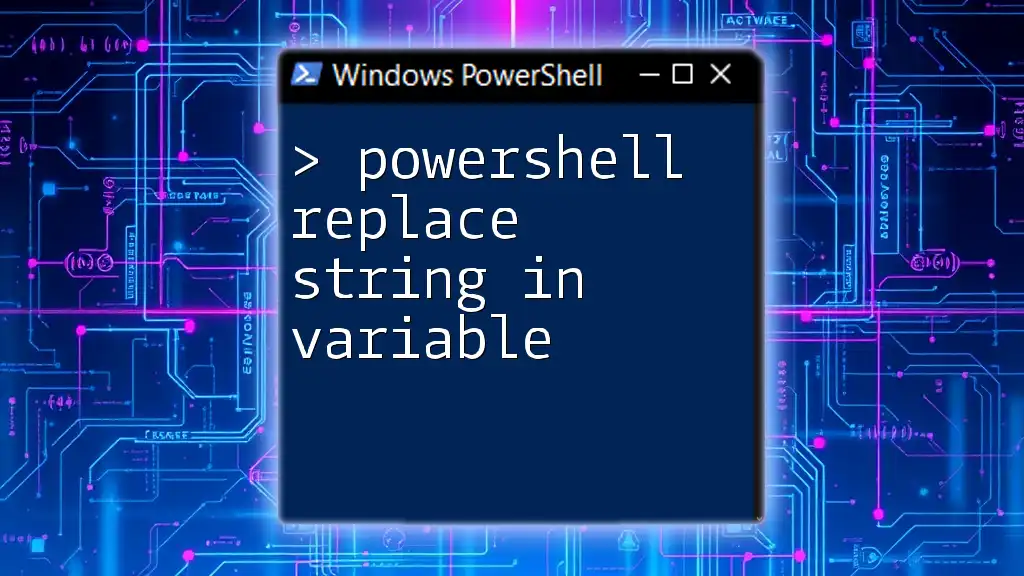
Call to Action
What experiences do you have with string manipulation in PowerShell? Share your insights or questions about using the `powershell replace` method to foster a collaborative learning environment. Don't forget to subscribe for more useful tips and tricks related to PowerShell!