PowerShell's `Recurse` parameter allows you to perform actions on files and directories within a specified path and all its subdirectories.
Here's a code snippet to illustrate how to use the `Recurse` parameter to delete all `.tmp` files in a directory and its subdirectories:
Remove-Item -Path "C:\Path\To\Directory\*.tmp" -Recurse -Force
Understanding Recursion in PowerShell
What is Recursion?
Recursion is a programming technique where a function calls itself to solve a problem. This method allows complex problems to be broken down into simpler sub-problems, making it easier to achieve a solution. In PowerShell, using recursion can simplify tasks involving nested data structures or repetitive processes, enabling streamlined automation.
Key Concepts of Recursion
To grasp the mechanics of recursion, it's essential to understand two fundamental concepts:
-
Base Case: This is a condition that allows the recursive function to terminate. If a recursive function doesn't reach a base case, it can lead to infinite loops or stack overflows.
-
Recursive Case: This condition allows the function to keep calling itself until it reaches the base case. A well-structured recursive function must clearly differentiate between these two cases to function correctly.
Visualizing Recursion: When a function calls itself, it is added to the call stack. If multiple recursive calls are made before reaching a base case, they stack up. Cleaning up this stack happens as the base case gets met, returning control back up the stack until all calls are resolved.
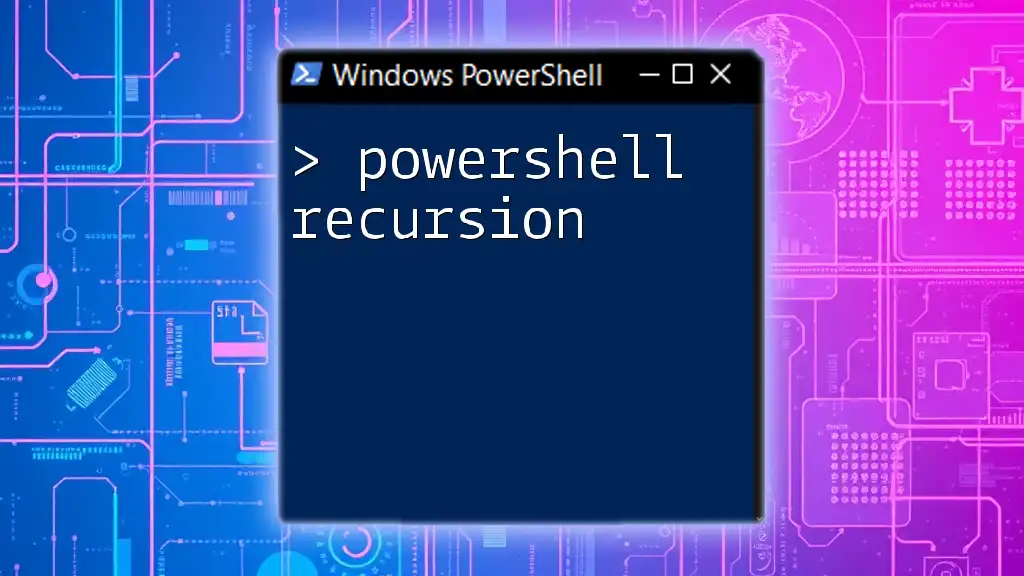
PowerShell and Recursion
Introduction to PowerShell Recurse
In PowerShell, the term recurse often refers to the ability to navigate through directories, process nested data, or iterate over complex structures using recursive logic. Utilizing recursive commands can lead to enhanced efficiency in managing tasks that involve multilevel data.
Use Cases of Recursive PowerShell
PowerShell recursion shines in various areas:
-
File and Folder Management: When dealing with file systems containing many directories, recursion is invaluable. The `Get-ChildItem` command with the `-Recurse` parameter allows deep directory navigation.
-
Data Processing: For processing structured data like JSON or XML, recursion allows easy manipulation of nested elements, making it easier to extract or transform data.
-
Automating Tasks: Recursion can help automate administrative tasks, such as cleaning up nested folders, without the need for complex iterations.
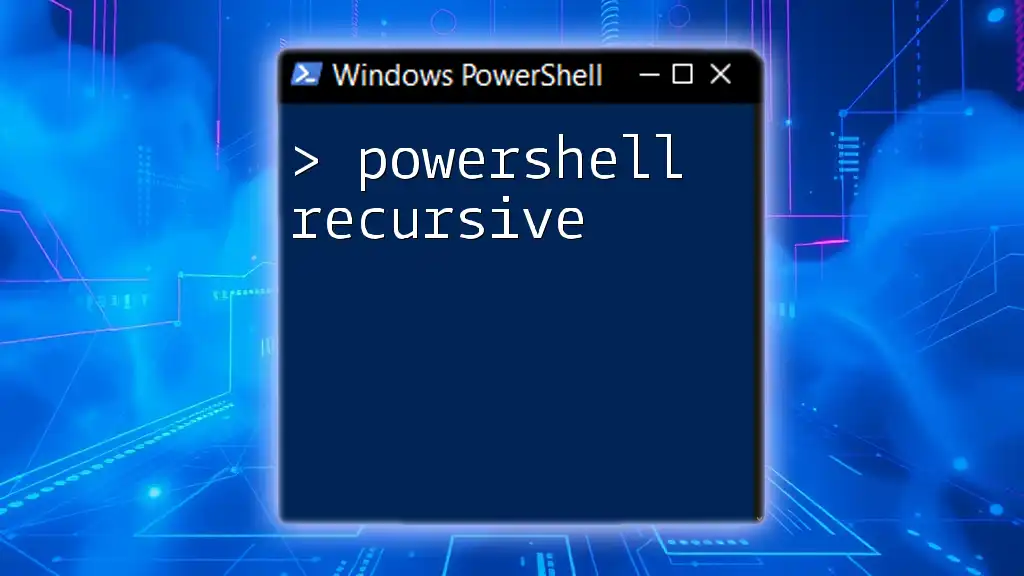
Working with Recursion in PowerShell
Writing a Simple Recursive Function
One of the simplest yet powerful implementations of recursion in PowerShell is calculating the factorial of a number. Here’s an example of how to create a basic recursive function:
function Factorial($number) {
if ($number -le 1) {
return 1
}
return $number * (Factorial($number - 1))
}
In this function:
- It checks if the input number is less than or equal to 1. If so, it returns 1, which serves as the base case.
- Otherwise, it multiplies the number by the result of the function called with the value decreased by one. This creates a chain of calls until the base case is met.
Advanced Recursive Patterns in PowerShell
As problems become more complex, recursion can adapt to handle intricate structures such as trees or graphs. Below is an example demonstrating how recursion can be applied to traverse a nested JSON object:
function Traverse-Json {
param ([hashtable]$jsonObject)
foreach ($key in $jsonObject.Keys) {
if ($jsonObject[$key] -is [hashtable]) {
Traverse-Json $jsonObject[$key]
} else {
Write-Host "$key: $($jsonObject[$key])"
}
}
}
In this function:
- It checks if the value associated with each key is a hashtable. If it is, the function recursively calls itself with that value.
- If the value is not a hashtable, it outputs the key-value pair, effectively navigating through potentially complex nested data.
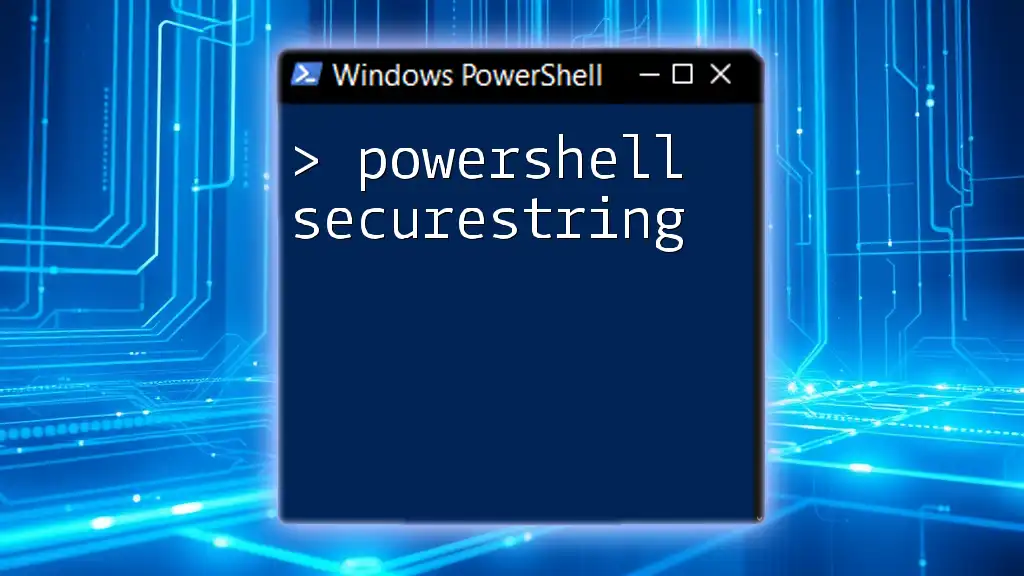
Practical Applications of Recursive PowerShell
Searching for Files Recursively
One of the most common uses of recursion in PowerShell is efficiently searching for files in a directory and its subdirectories. By leveraging the `Get-ChildItem` cmdlet with the `-Recurse` parameter, you can locate files quickly:
Get-ChildItem -Path C:\MyFiles -Recurse -Filter *.txt
This command retrieves all `.txt` files within the specified path, including all nested folders. It simplifies file management significantly.
Managing System Processes Recursively
Another practical application is managing system processes, especially when terminating child processes of a parent process. Here's how you can define a recursive function to kill processes:
function Kill-ChildProcess {
param([int]$PID)
Get-WmiObject Win32_Process -Filter "ParentProcessId = $PID" | ForEach-Object {
Kill-ChildProcess $_.ProcessId
Stop-Process -Id $_.ProcessId -Force
}
}
In this function:
- It queries for all processes with a specific parent ID.
- For each child process, it first calls itself recursively to terminate any further child processes before finally stopping the process.
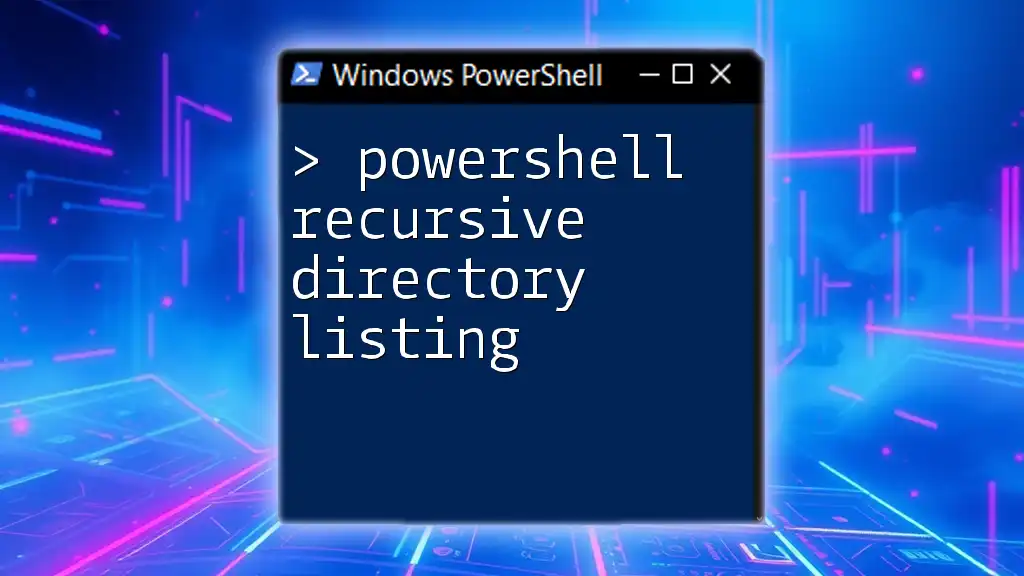
Best Practices for Recursive Scripts
Optimizing Memory Usage
To avoid stack overflow, especially in deep recursion cases, it's crucial to structure your recursive functions carefully. Here are a few strategies:
- Consider using iterative solutions when possible if the data structure is very deep.
- Optimize by implementing tail recursion, where the recursive call is the final operation.
Debugging Recursive Functions
Debugging can be challenging with recursion due to multiple layers of calls. Here are some tips:
- Utilize `Write-Debug` to monitor the flow of your recursive calls.
- Implement `Try-Catch` blocks to capture and handle potential errors effectively.
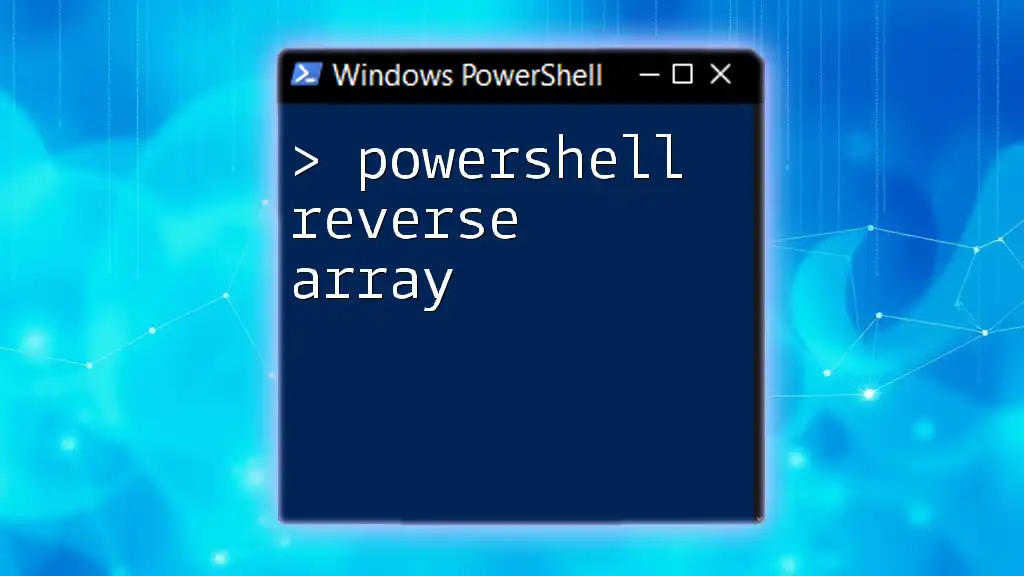
Conclusion
Recursion is a powerful concept in PowerShell that, when mastered, can lead to more efficient and elegant scripting solutions. By understanding when to use recursion and following best practices, you can tackle complex tasks with finesse. As you explore more advanced concepts, consider delving into community resources to expand your skills further. Embrace recursion and watch your PowerShell scripting capabilities grow!