PowerShell requires a Windows operating environment and administrative privileges to execute scripts and commands effectively, allowing users to automate tasks and manage system configurations.
Write-Host 'Hello, World!'
What is the `#Requires` Directive?
The `#Requires` directive in PowerShell serves a crucial role in scripting, specifically designed to declare script dependencies. By including `#Requires` at the top of your PowerShell script, you outline explicit needs that must be met for the script to execute successfully. This can involve specifying the PowerShell version, required modules, or even essential cmdlets.
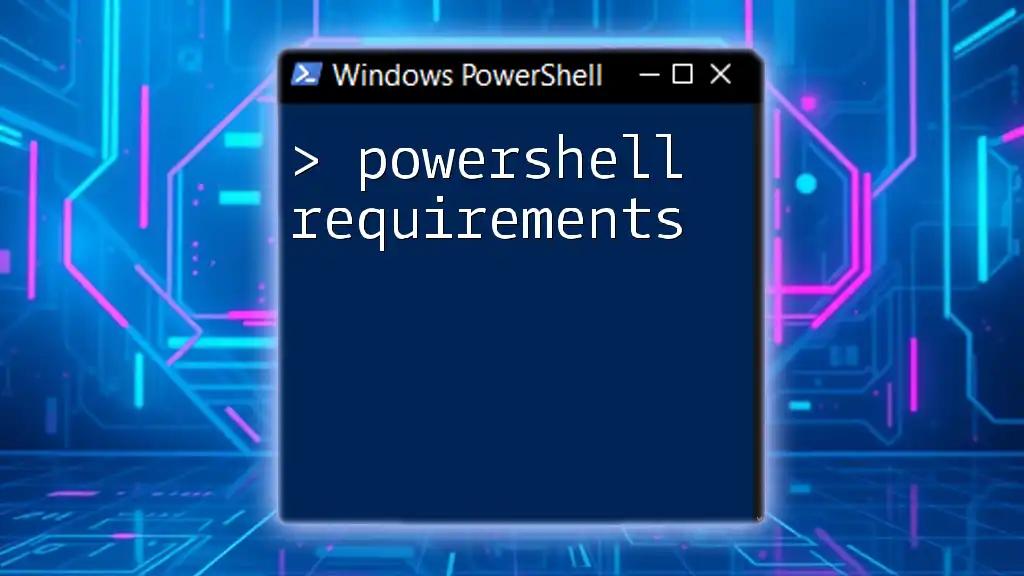
Importance of Using `#Requires`
Ensuring Compatibility
By utilizing the `#Requires` directive, you guarantee that your script runs in a compatible environment. This is particularly vital in scenarios where scripts might work perfectly in one version of PowerShell but fail in another. For example, if you are using a cmdlet that was introduced in PowerShell version 5.1, you can enforce this requirement in your script:
#Requires -Version 5.1
This line ensures that if a user tries to run the script in an earlier version of PowerShell, they will receive an informative error, preventing unexpected behaviors or broken functionality.
Improving Script Reliability
When scripts are designed to run under specific conditions or environments, the risk of runtime errors significantly decreases. The `#Requires` directive acts as a safeguard, allowing you to avoid unintended behaviors that could disrupt the execution of your script. By ensuring that all necessary prerequisites are in place before the script runs, you create a more robust and reliable user experience.

Syntax of the `#Requires` Directive
Basic Syntax
The syntax for the `#Requires` directive is straightforward. Below are common usages:
#Requires -Version 5.0
#Requires -Module SomeRequiredModule
These lines inform the interpreter of the specific requirements that need to be satisfied for the script to run.
Specifying PowerShell Versions
To enforce a specific version of PowerShell, you might use the following command:
#Requires -Version 5.1
By including this line, you ensure that your script will only run in PowerShell 5.1 or a later version, thus maintaining compatibility with features introduced in these versions.
Module Dependencies
To declare dependencies on specific modules, you would utilize:
#Requires -Module Az
This directive checks if the `Az` module is available. If it is not installed, PowerShell will throw an error, preventing the script from executing further and providing clarity on what is missing.
Snapins Requirements
Although less common today, snapins can also be specified similarly, ensuring that the required snapins are loaded before script execution continues.
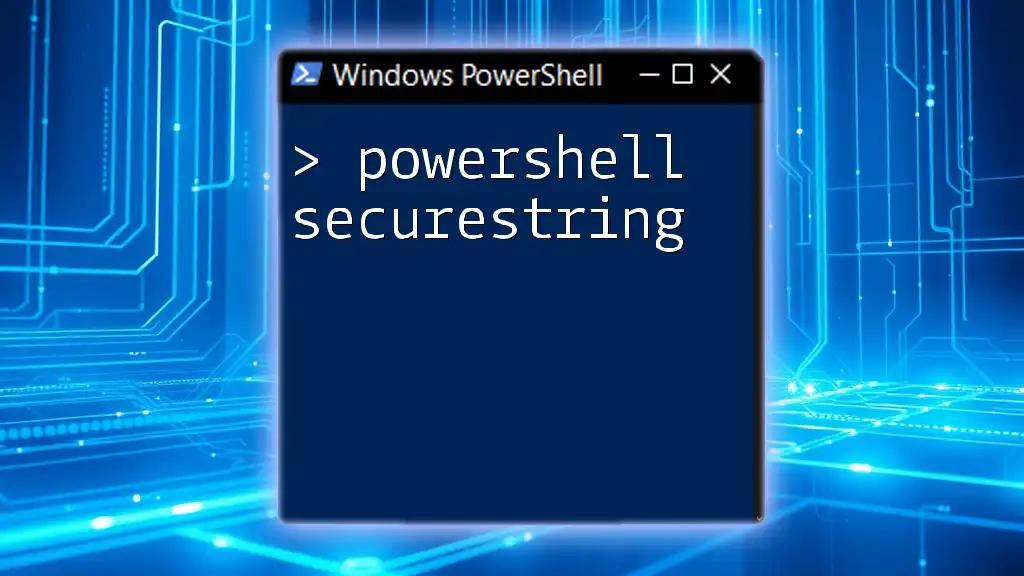
Using `#Requires` with Other Parameters
Required Cmdlets or Functions
The `#Requires` directive also allows for prerequisites regarding specific cmdlets. For instance, if your script requires a particular cmdlet to function properly, you can declare this:
#Requires -Cmdlet Get-Item
Error Handling
Proper error handling can enhance the user experience. If the requirements are not met, you can include logical checks in your script to provide informative feedback. Here’s an example:
if (-not (Get-Module -ListAvailable -Name SomeRequiredModule)) {
throw "Required module 'SomeRequiredModule' is not installed."
}
This piece of code ensures that users receive a clear message regarding the missing module rather than encountering ambiguous runtime errors.
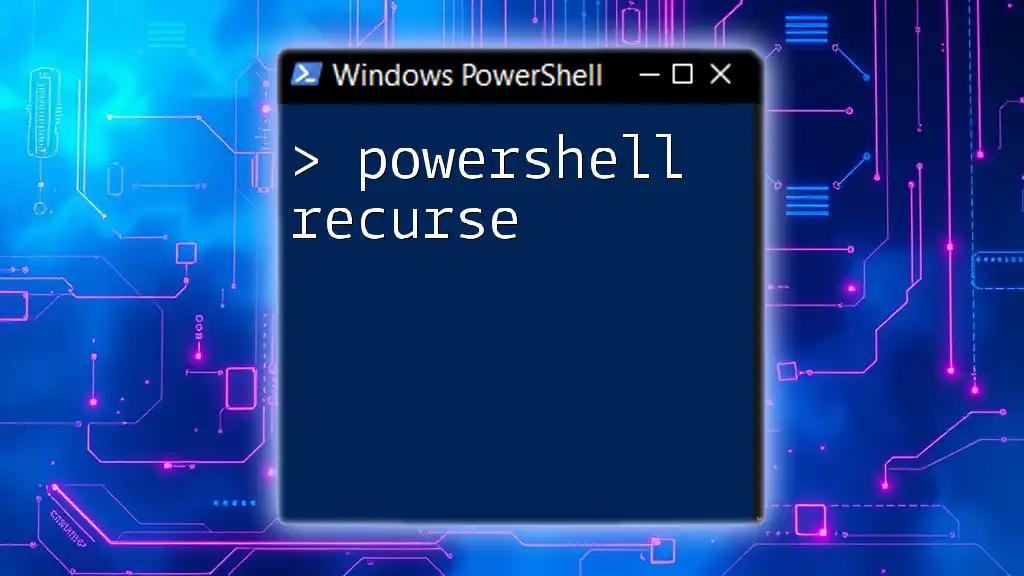
Practical Examples of `#Requires` in Action
Example 1: Simple Script with Version Check
Consider a simple script that requires a specific PowerShell version:
#Requires -Version 7.0
Write-Host "This script runs only on PowerShell 7.0 or higher"
The directive at the top establishes the minimum version requirement, and the script itself only proceeds if this condition is satisfied.
Example 2: Script with Module Dependency
Here’s another script that leverages a specific module necessary for its operations:
#Requires -Module AzureAD
# Script content using AzureAD cmdlets
If the `AzureAD` module is not available, the script will halt execution so that the user can install the missing module.
Example 3: Multi-requirements Script
In a more complex scenario, suppose you have a script that requires both a certain version of PowerShell and specific cmdlets:
#Requires -Version 5.0 -Module SqlServer -Cmdlet Get-SqlInstance
This directive ensures that all specified conditions must be satisfied before any part of the script is executed.
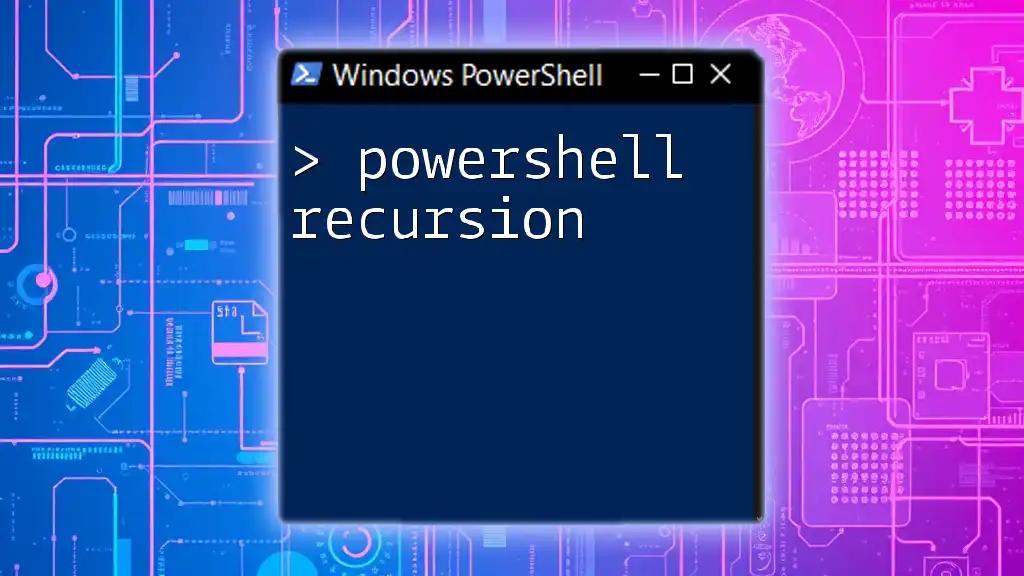
Best Practices for Using `#Requires`
Documentation
Always document why each `#Requires` directive is included in your script. Clear comments can help others (and your future self) understand the rationale behind certain requirements:
#Requires -Version 5.1 # This script uses cmdlets available only in this version
Testing and Validation
Testing scripts under various environments is crucial. Ideally, you should run your scripts in environments that mimic your users' systems. It helps ensure that every dependency is accurately declared and facilitated.

Conclusion
Using the `#Requires` directive in PowerShell scripts is not just a good practice but an essential step towards ensuring reliability and clarity. By explicitly defining requirements, you safeguard your scripts against compatibility issues and runtime errors. As you develop more complex scripts, integrate the `#Requires` directive responsibly, making your tools more robust for yourself and others who may run your code.
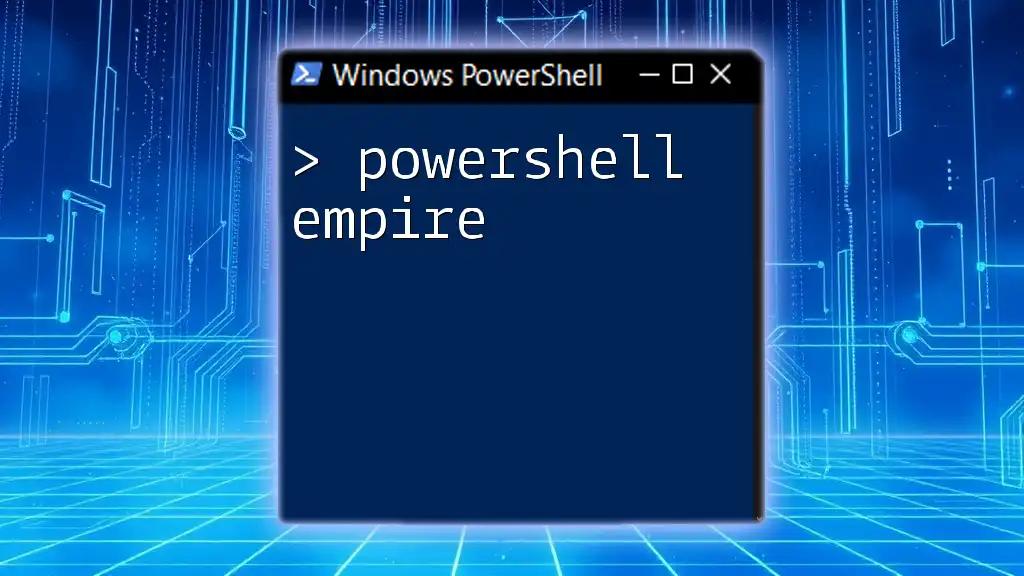
Call to Action
Explore the `#Requires` directive in your own PowerShell scripts today! Share your experiences in the comments section below and let us know how this directive has helped you enhance your scripting practices.