The `Register-ScheduledTask` cmdlet in PowerShell is used to create a new scheduled task on a computer, allowing users to automate repetitive tasks efficiently.
Here's a code snippet to register a simple scheduled task that runs a PowerShell script:
$action = New-ScheduledTaskAction -Execute 'powershell.exe' -Argument '-File "C:\Scripts\YourScript.ps1"'
$trigger = New-ScheduledTaskTrigger -At 5AM -Daily
Register-ScheduledTask -Action $action -Trigger $trigger -TaskName 'MyDailyTask' -Description 'Runs my PowerShell script daily at 5 AM'
Understanding Scheduled Tasks
What is a Scheduled Task?
A scheduled task is a feature in Windows that allows users toautomatically execute programs or scripts at pre-defined times or events. This capability is essential for automation and helps in managing routine tasks efficiently, freeing up time for more critical activities.
How Scheduled Tasks Work
The Task Scheduler architecture plays a critical role in managing scheduled tasks. It relies on the Task Scheduler Service, which takes care of triggering tasks and executing them according to the predefined schedule.
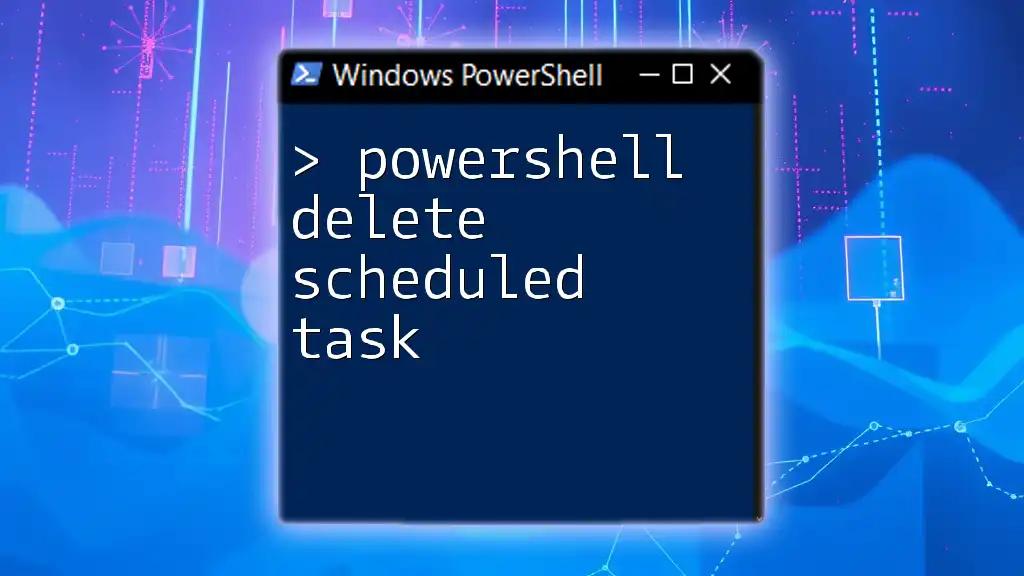
The Basics of `Register-ScheduledTask`
Command Overview
The `Register-ScheduledTask` cmdlet is powerful in PowerShell, enabling you to create new scheduled tasks. The command utilizes various parameters to define important aspects of a scheduled task. Its basic syntax looks as follows:
Register-ScheduledTask -TaskName <String> -Action <Action> -Trigger <Trigger> -Principal <Principal> -Settings <Settings> -Description <String>
Key Parameters Explained
Action Parameter
Define the task’s action—the command or script that will be executed. An action is typically created using New-ScheduledTaskAction.
Example of defining an action:
$action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "-File C:\Scripts\MyScript.ps1"
Trigger Parameter
Triggers initiate the scheduled task at specified intervals. Different types of triggers include time-based executions and event-based triggers.
Example of a time trigger:
$trigger = New-ScheduledTaskTrigger -Daily -At "08:00AM"
Principal Parameter
The principal parameter specifies the user context under which the task runs, ensuring it has the right permissions for execution.
Example of setting up the principal:
$principal = New-ScheduledTaskPrincipal -UserId "SYSTEM" -LogonType ServiceAccount
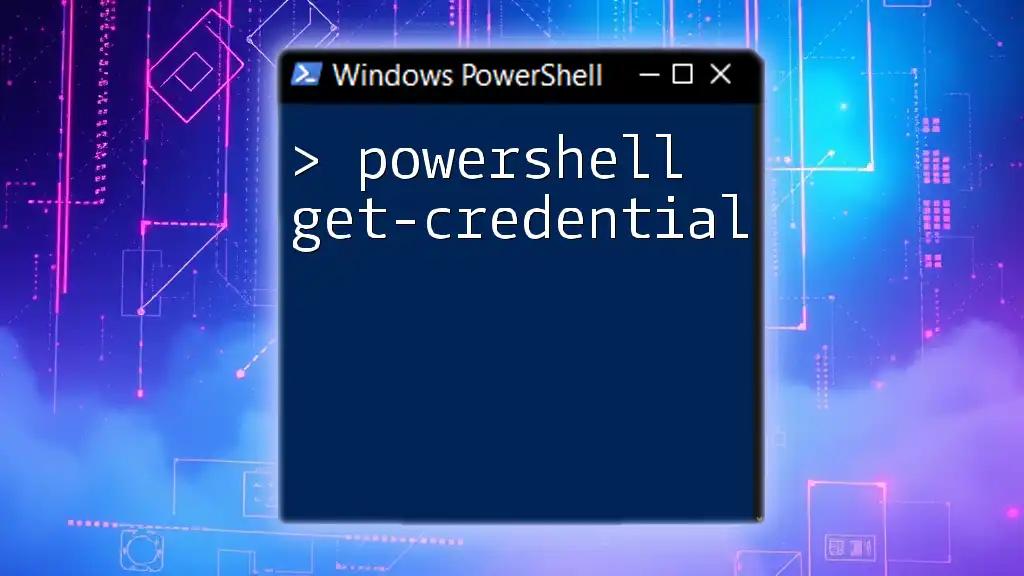
How to Use `Register-ScheduledTask`
Step-by-Step Creation of a Basic Scheduled Task
To create a basic scheduled task, you will need to outline the action, trigger, and principal. Here’s how to do it:
$action = New-ScheduledTaskAction -Execute "PowerShell.exe" -Argument "-File C:\Scripts\MyScript.ps1"
$trigger = New-ScheduledTaskTrigger -Daily -At "08:00AM"
$principal = New-ScheduledTaskPrincipal -UserId "SYSTEM" -LogonType ServiceAccount
Register-ScheduledTask -TaskName "MyDailyScript" -Action $action -Trigger $trigger -Principal $principal
In this snippet:
- $action defines what will run.
- $trigger sets the time the task should execute.
- $principal designates the user context, in this case, running as the SYSTEM account.
Advanced Task Creation
Using Multiple Triggers
You can enhance your scheduled task's functionality by incorporating multiple triggers. This allows for various execution patterns.
Example of setting multiple triggers:
$trigger1 = New-ScheduledTaskTrigger -AtStartup
$trigger2 = New-ScheduledTaskTrigger -Weekly -DaysOfWeek Monday
Register-ScheduledTask -TaskName "CombinedTriggersTask" -Action $action -Principal $principal -Trigger $trigger1, $trigger2
In this example, the task will run at system startup and every Monday.
Scheduling Options
Different frequencies can be quite powerful when creating a scheduled task. Options such as Weekly, Monthly, or specific events like At System Startup provide flexibility.
Example code with various scheduling options:
$trigger1 = New-ScheduledTaskTrigger -AtStartup
$trigger2 = New-ScheduledTaskTrigger -Weekly -DaysOfWeek Monday
Register-ScheduledTask -TaskName "CombinedTriggersTask" -Action $action -Principal $principal -Trigger $trigger1, $trigger2
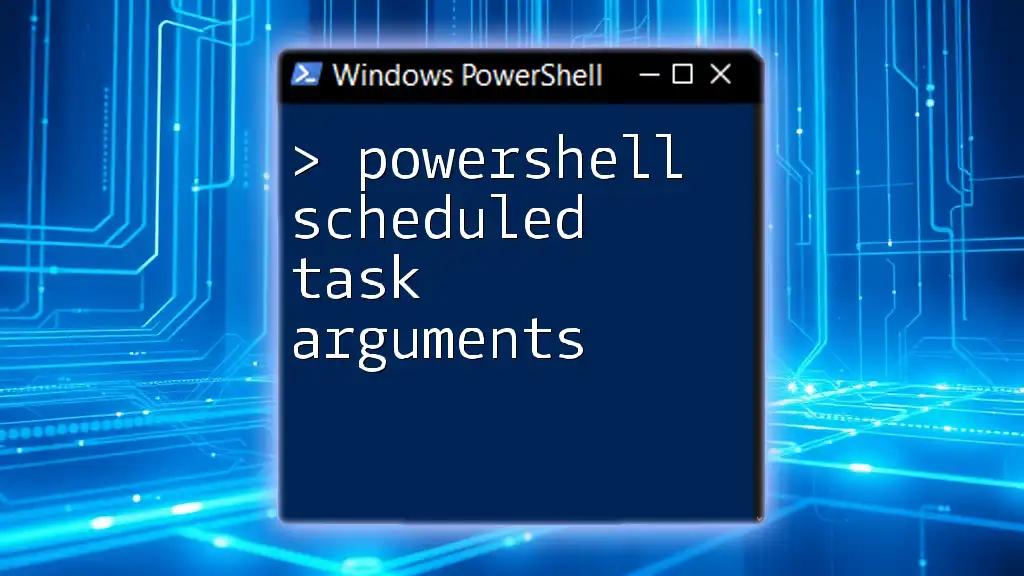
Managing Your Scheduled Tasks
Modifying Existing Tasks
Task modification can be accomplished using the `Set-ScheduledTask` cmdlet. This allows you to adjust triggers and other settings as needed.
Example code snippet for modifying a task:
$task = Get-ScheduledTask -TaskName "MyDailyScript"
$task.Triggers.StartBoundary = "2024-01-01T08:00:00"
Set-ScheduledTask -InputObject $task
This command retrieves an existing scheduled task and modifies when it starts.
Deleting Scheduled Tasks
If you need to remove a scheduled task, `Unregister-ScheduledTask` provides a simple solution.
Example command to delete a task:
Unregister-ScheduledTask -TaskName "MyDailyScript" -Confirm:$false
Using the `-Confirm:$false` flag avoids a confirmation prompt, making the process quicker.
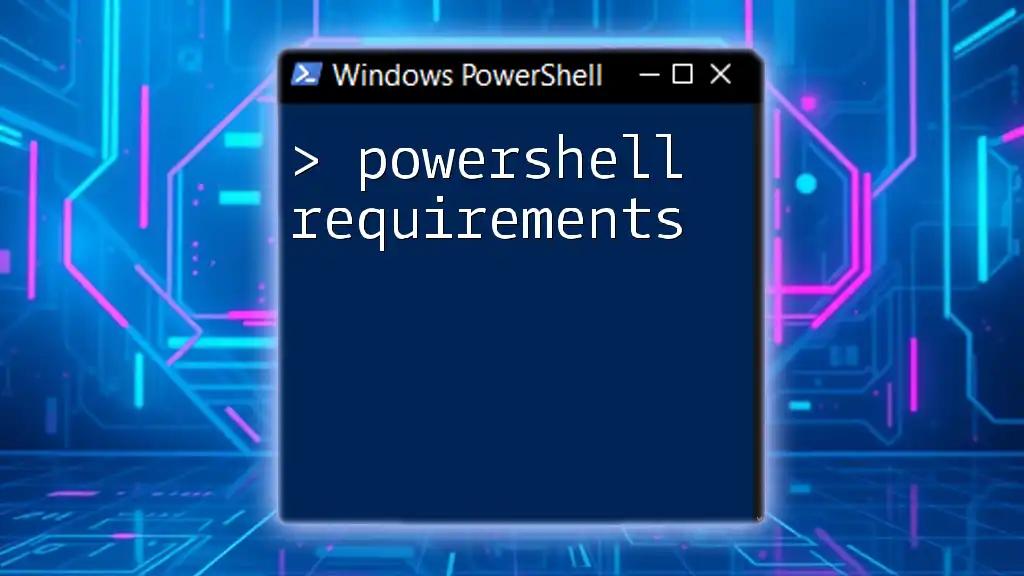
Troubleshooting Common Issues
Error Messages to Watch For
Errors can often surface when tasks do not run as expected or if there’s an issue with permissions. Some common error codes include errors related to access denied or incorrect paths. Understanding these error messages is key to troubleshooting effectively.
Logging and Event Viewer
For deeper insights into task failures, you can check the Task Scheduler logs and the Windows Event Viewer. These logs provide error codes and detailed information about what went wrong during execution, which can be invaluable for diagnosing issues.

Best Practices for Using `Register-ScheduledTask`
When using `Register-ScheduledTask`, keep in mind some best practices to ensure your scheduled tasks run smoothly:
- Naming Conventions: Adopt clear, descriptive naming conventions for easier management and identification.
- Documentation: Maintain documentation for each task to make it easier for others (and yourself) to understand what is scheduled and when.
- Security Considerations: Be cautious about the user context in which tasks run, especially if they perform actions on sensitive data or critical services.
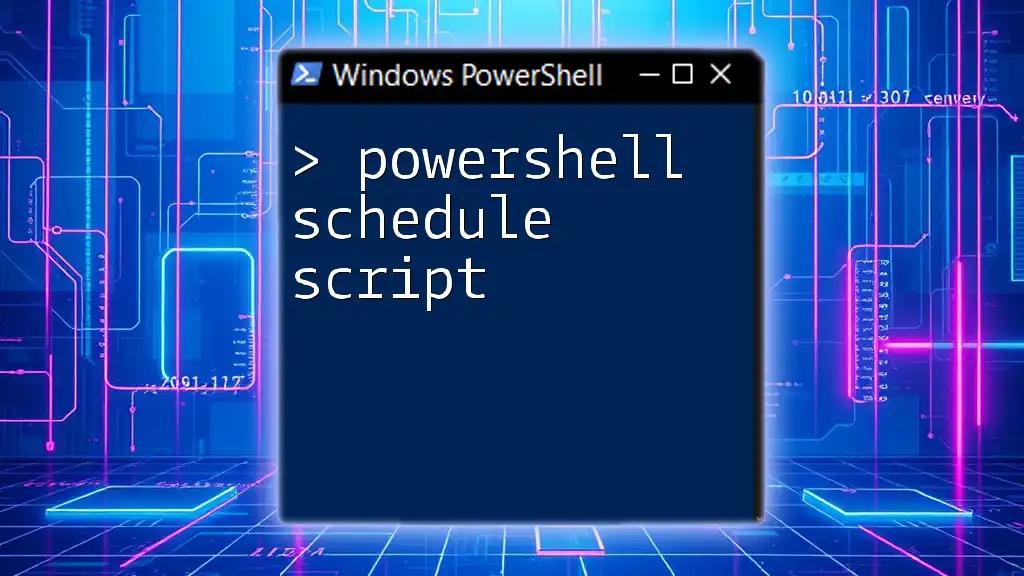
Conclusion
The `powershell register-scheduledtask` cmdlet opens a realm of possibilities for automating your daily tasks in Windows. By employing actions, triggers, and principals effectively, you can optimize your workflow and manage various activities effortlessly. Embrace automation and leverage the power of scheduling, and you will open doors to increased efficiency in your daily operations.
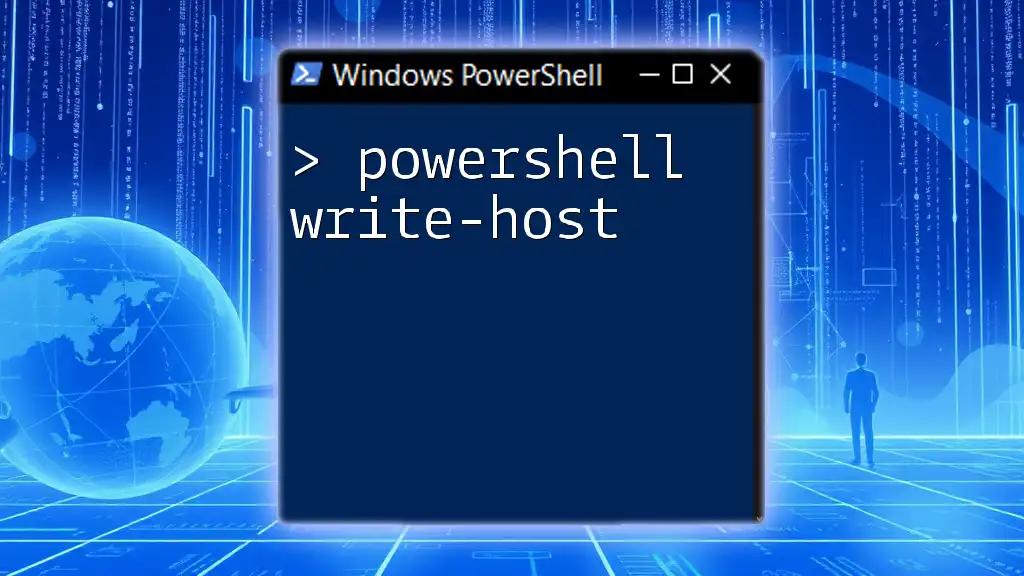
Additional Resources
For further learning, take advantage of the official Microsoft documentation on scheduled tasks, explore PowerShell community forums for real-world applications, and consider investing in books or courses that cover PowerShell automation deeply.