PowerShell scheduling allows you to automate the execution of scripts at specified times using the Task Scheduler, enabling efficient task management.
Here’s a simple code snippet to create a scheduled task that runs a PowerShell script daily at 7 AM:
$Action = New-ScheduledTaskAction -Execute 'Powershell.exe' -Argument '-File "C:\Path\To\YourScript.ps1"'
$Trigger = New-ScheduledTaskTrigger -Daily -At '7:00AM'
Register-ScheduledTask -Action $Action -Trigger $Trigger -TaskName 'MyPowerShellTask' -User 'SYSTEM' -RunLevel Highest
Understanding PowerShell Scheduling
What is Task Scheduling?
Task scheduling is a critical aspect of automation in the IT environment. It allows scripts and programs to run at predetermined times or intervals without manual intervention. By automating these processes, you can save valuable time, reduce the likelihood of human error, and ensure consistent execution of important administration tasks.
Benefits of Using PowerShell for Scheduling Tasks
Using PowerShell for scheduling offers several key advantages:
- Increased Efficiency: Automating mundane tasks frees up time for more complex issues that require human oversight.
- Reduction of Manual Errors: By eliminating the need for manual executions, the chances of errors, such as running the wrong command or forgetting to execute a task altogether, significantly decrease.
- Seamless Handling of Repetitive Tasks: PowerShell scripts can easily execute repetitive tasks, ensuring they are completed exactly as needed every time.
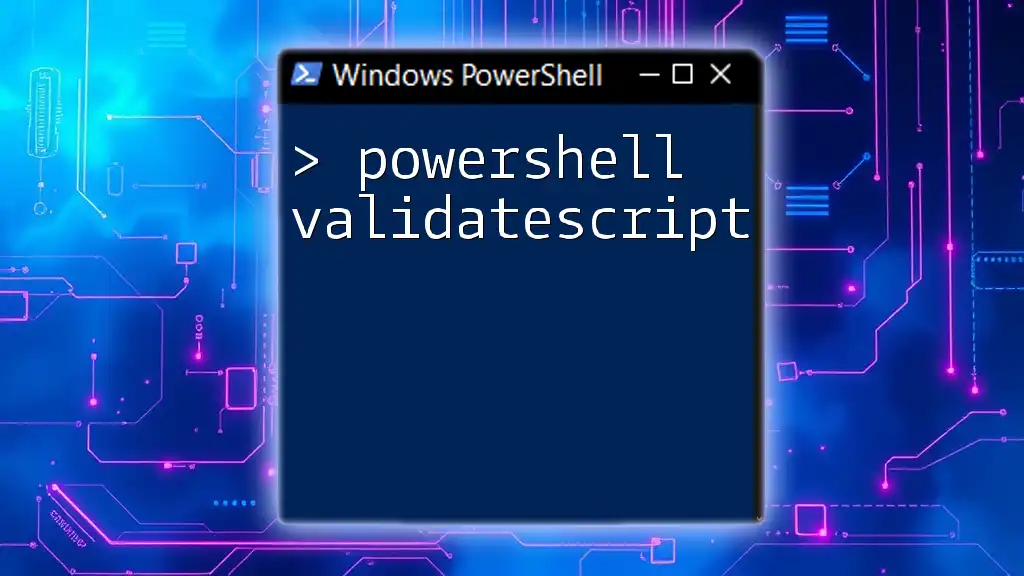
Setting Up Windows Task Scheduler
Accessing Windows Task Scheduler
To get started with PowerShell task scheduling, you first need to access the Windows Task Scheduler. Here are several ways to open the Task Scheduler:
-
Method 1: via Control Panel
- Open Control Panel, go to System and Security, and click on "Administrative Tools." From there, select Task Scheduler.
-
Method 2: via Run Dialog Box
- Press `Win + R`, type `taskschd.msc`, and hit Enter.
-
Method 3: via Windows Search Box
- Type "Task Scheduler" in the search bar and click on the application when it appears.
Creating a Basic Scheduled Task
Once you have the Task Scheduler open, you can create your first scheduled task:
- Click on Create Basic Task located on the right-hand side of the Task Scheduler window.
- Provide a descriptive name and an optional description for your task to help you identify its purpose.
- Decide when you want the task to start. You can set triggers for daily, weekly, or monthly executions according to your needs.
Configuring Actions for Your Task
To run a PowerShell script with your scheduled task, you need to set the action that will execute your script.
Running a PowerShell Script
-
Choose the Start a Program action.
-
For program/script, you will enter the path to PowerShell. Typically, this is located at `C:\Windows\System32\WindowsPowerShell\v1.0\powershell.exe`.
-
In the Add arguments (optional) field, you need to specify how to execute your script. The command below will run your PowerShell script and avoid any execution policy blocks:
-ExecutionPolicy Bypass -File "C:\path\to\script.ps1"
-
Make sure to replace `C:\path\to\script.ps1` with the actual path of your PowerShell script.
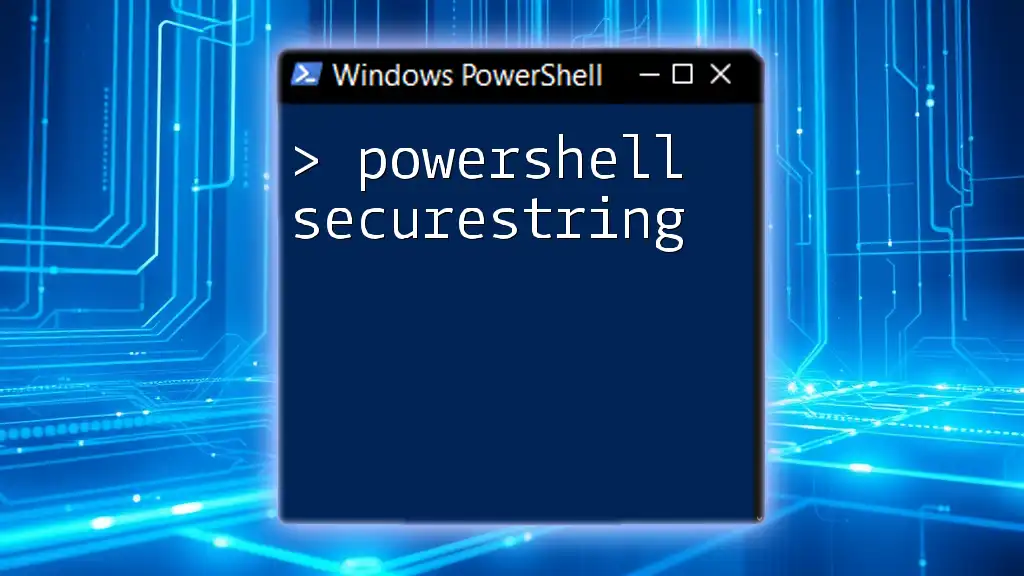
Writing Your PowerShell Script
Crafting a Basic PowerShell Script
A basic PowerShell script can be incredibly straightforward yet powerful. Below is an example of a simple script that retrieves and saves a list of currently running processes to a text file:
Get-Process | Out-File "C:\path\to\processes.txt"
Explanation of the Code
- `Get-Process`: This cmdlet retrieves all processes running on your local machine.
- `|`: The pipe operator is used to send the output of one cmdlet to another.
- `Out-File`: This cmdlet is used to send output to a file, allowing you to save the running processes in a `.txt` format.
Testing Your PowerShell Script
Before scheduling your script, it’s essential to test it manually:
- Open PowerShell as an Administrator.
- Navigate to the directory where your script is located.
- Run your script by typing `.\script.ps1`.
This will ensure that your script works as intended. If errors appear, you’ll be able to address them before automation kicks in. Common issues include permissions or syntax errors, so debug any problems thoroughly.
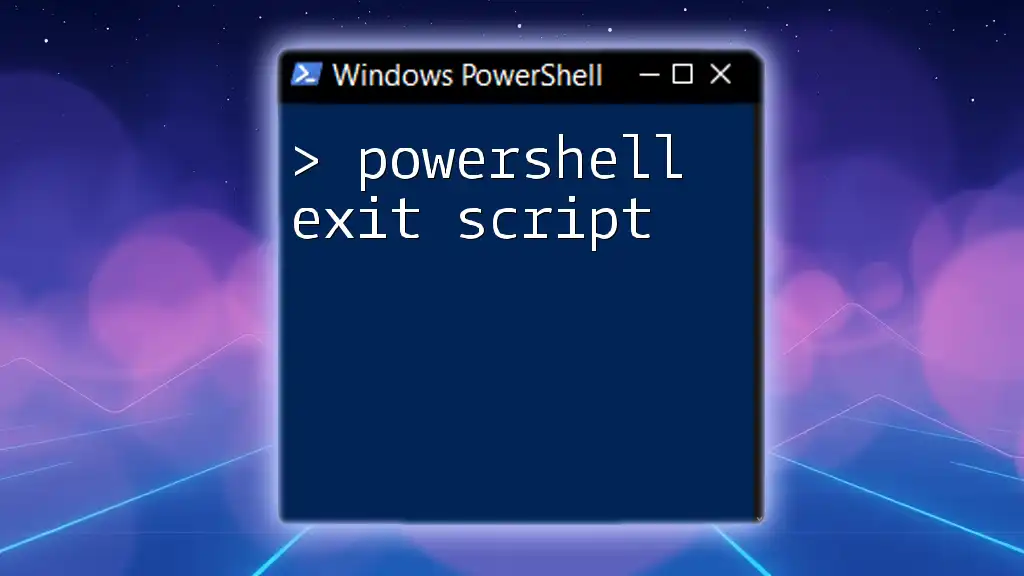
Advanced Scheduling Options
Configuring Triggers and Conditions
To enhance your scheduled task, consider various triggering options available:
- Logon Triggers: Execute a task when a specific user logs on.
- Event Triggers: Run a task in response to specific events in the event log.
Understanding these advanced triggers helps tailor tasks according to operational needs, making them even more efficient.
Configuring Task Settings
In Task Scheduler, you can configure numerous task properties:
- Run only when user is logged on: This option is useful when the task does not need to interact with the desktop.
- Run with highest privileges: If your script needs administrative permissions, ensure to check this setting.
Managing your task settings effectively is vital to ensuring optimized task performance.
Handling Script Output: Email Notifications
To keep track of your script's execution status, you may want to send notifications when your script completes or encounters an error. Below is an example of how to send an email notification:
$smtpServer = "smtp.yourserver.com"
$message = "Script executed successfully!"
Send-MailMessage -From "you@domain.com" -To "recipient@domain.com" -Subject "PowerShell Script Status" -Body $message -SmtpServer $smtpServer
Explanation of Code
- This script snippet sends an email using a specified SMTP server. Make sure to replace the placeholders with actual email addresses and your SMTP server details.
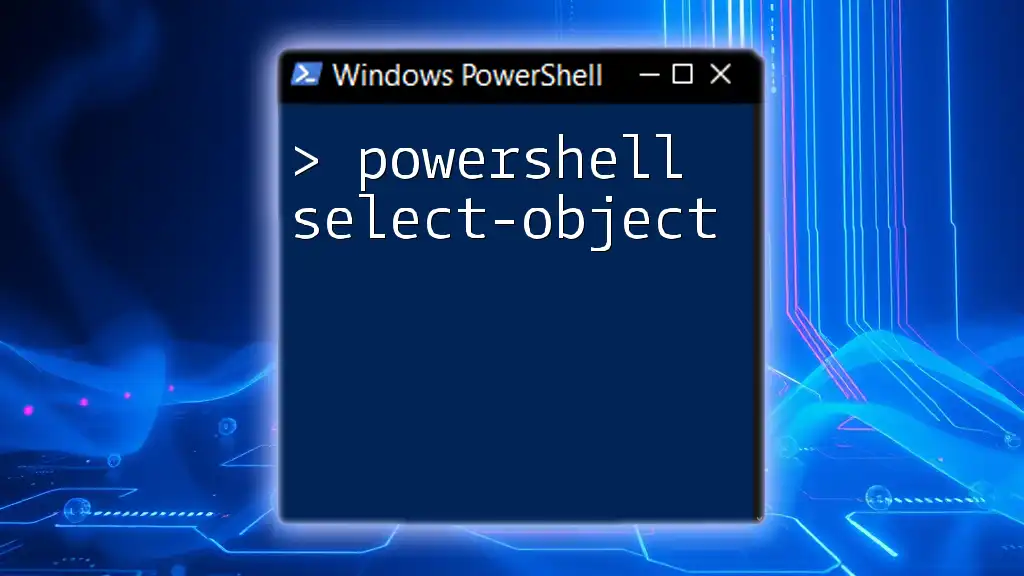
Monitoring and Managing Scheduled Tasks
Viewing Scheduled Tasks
To keep track of your scheduled tasks, frequently visit Task Scheduler to check the status of running and completed tasks. Task Scheduler provides a clear overview of when tasks are scheduled, their last run times, and whether they succeeded or failed.
Modifying and Deleting Scheduled Tasks
Over time, your automation needs may change. To modify or delete a scheduled task:
- Right-click on the task in Task Scheduler and select either Properties to edit or Delete to remove.
Maintaining an organized list of tasks helps ensure that outdated or unnecessary automations do not clutter your task manager.
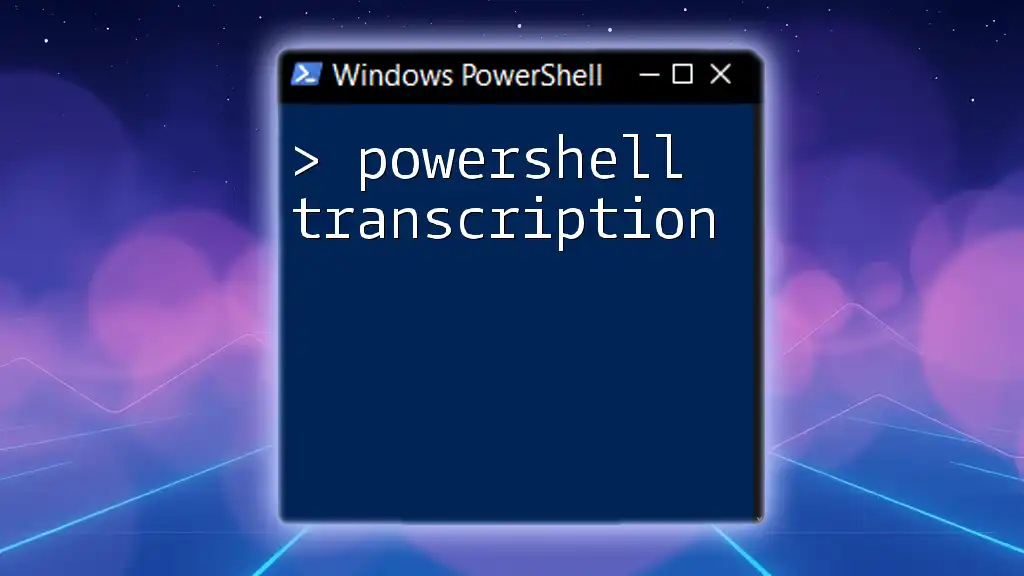
Troubleshooting Common Issues
Common Problems and Their Solutions
Some of the most common issues when scheduling PowerShell scripts include:
- Script not running: Verify the trigger settings and confirm that the path to PowerShell and your script are correct.
- Access permissions: Ensure that the task is configured with an account that has adequate permissions to run the script.
- Execution policy issues: If errors arise regarding policy restrictions, consider modifying the `ExecutionPolicy` as shown previously.
Logging and Monitoring Task Execution
You can enable logging for your scheduled tasks, providing an audit trail for every execution. In the task’s properties, you can set options to create logs which can be helpful for troubleshooting.
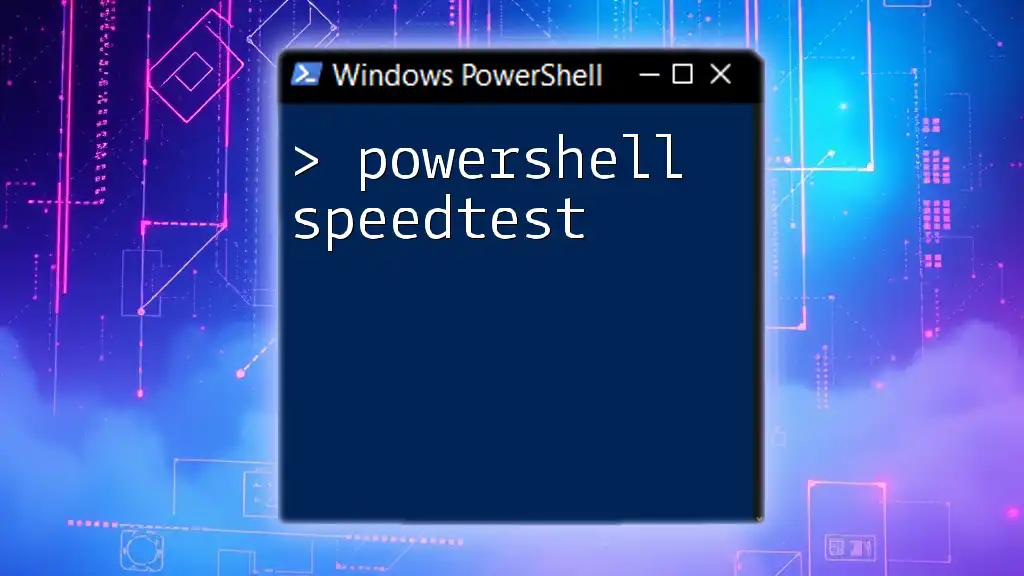
Conclusion
Scheduling PowerShell scripts using Windows Task Scheduler is a powerful method to automate repetitive tasks and improve productivity. With a few simple steps, you can set up your scripts to run as needed, freeing yourself from the tedium of manual execution. By leveraging the capabilities of PowerShell and Task Scheduler, you can create a streamlined workflow that enhances efficiency and reduces errors in your daily operations.
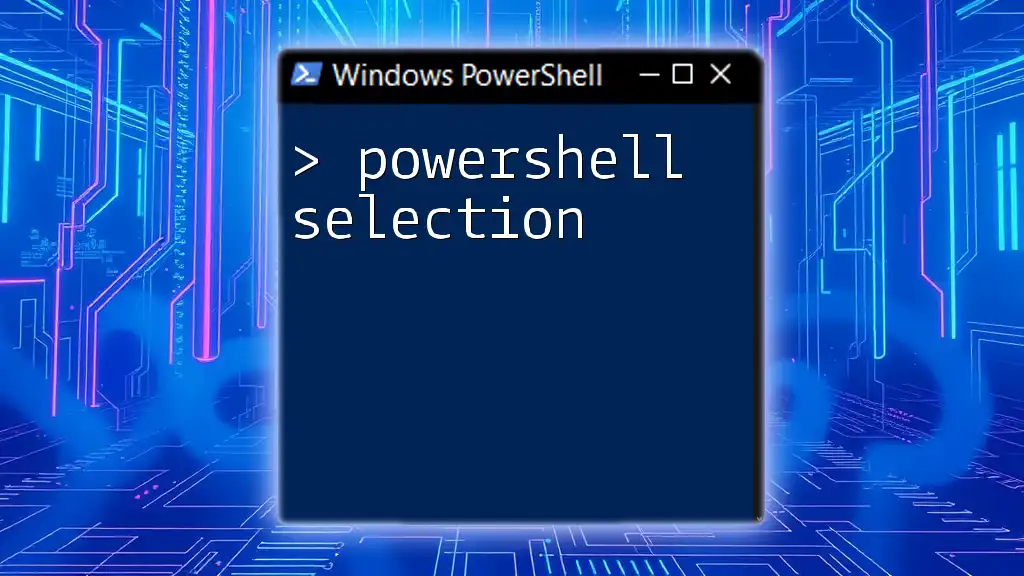
Call to Action
Don’t hesitate to try scheduling your first PowerShell script today! Explore the powerful possibilities that automation brings, and if you wish to delve deeper into PowerShell scripting, consider joining our courses and tutorials for comprehensive learning.