In PowerShell, the `Substring` method allows you to extract a portion of a string by specifying the starting index and the length, enabling efficient string manipulation.
Here’s a code snippet that demonstrates its use:
$originalString = "Hello, World!"
$subString = $originalString.Substring(7, 5)
Write-Host $subString # Output: World
What is a Substring?
A substring is a contiguous sequence of characters within a string. In programming, the concept of a substring is essential for extracting and manipulating portions of data. Whether you're dealing with log files, user input, or configuration settings, being able to work with substrings can help you streamline your scripts and process data more efficiently.
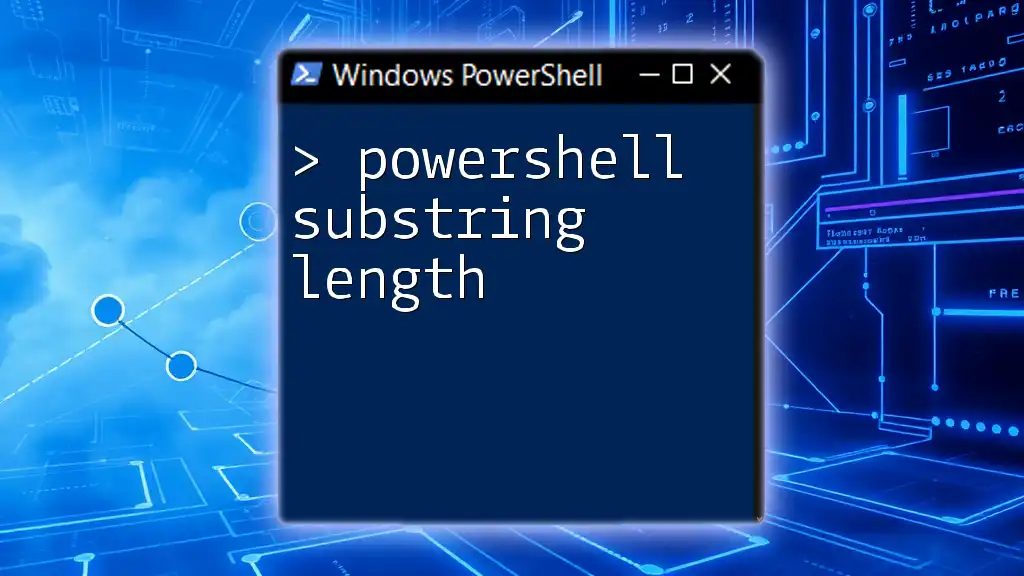
Overview of PowerShell Strings
In PowerShell, strings are sequences of characters that can be used for various purposes, such as representing text, parsing data, and more. Strings are handled as object types, meaning they come with a rich set of methods, including the ability to extract substrings. Understanding how PowerShell handles strings will set you up for success as you learn to use the `Substring()` method effectively.
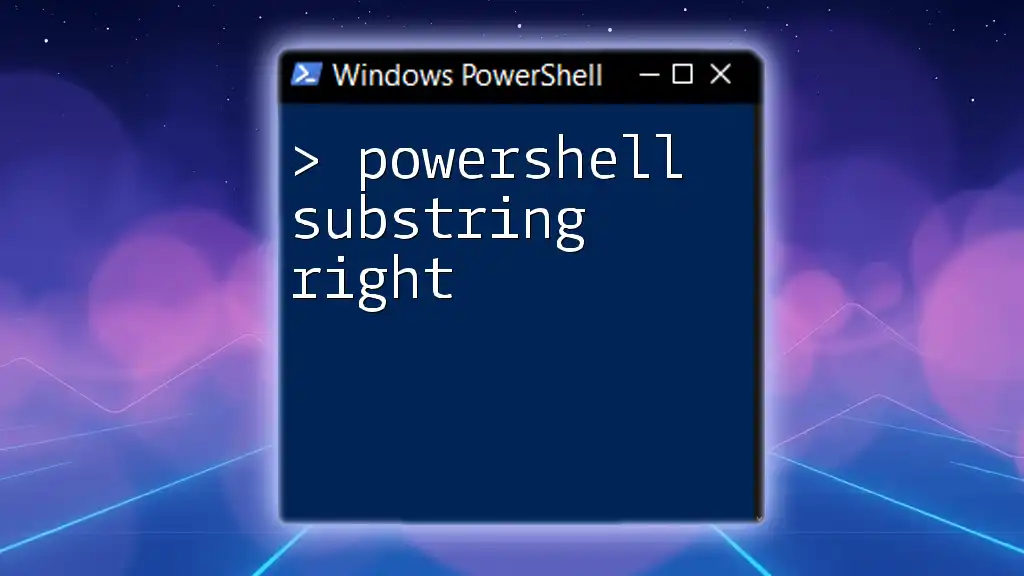
Understanding the `Substring()` Method
Syntax of the `Substring()` Method
The `Substring()` method in PowerShell has a straightforward syntax:
$substring = $string.Substring(startIndex, length)
- startIndex: This parameter specifies the zero-based starting position of the substring.
- length: This optional parameter defines the number of characters to extract. If omitted, the substring will extend from the starting index to the end of the string.
How the `Substring()` Method Works
The `Substring()` method extracts a specified number of characters from the original string, beginning at a designated starting index. Here's a basic example to illustrate this point:
$exampleString = "Hello, World!"
$subString = $exampleString.Substring(7, 5) # Outputs 'World'
In this example, the substring starts from the 8th character (at index 7) and extracts the next 5 characters, resulting in the word 'World'.
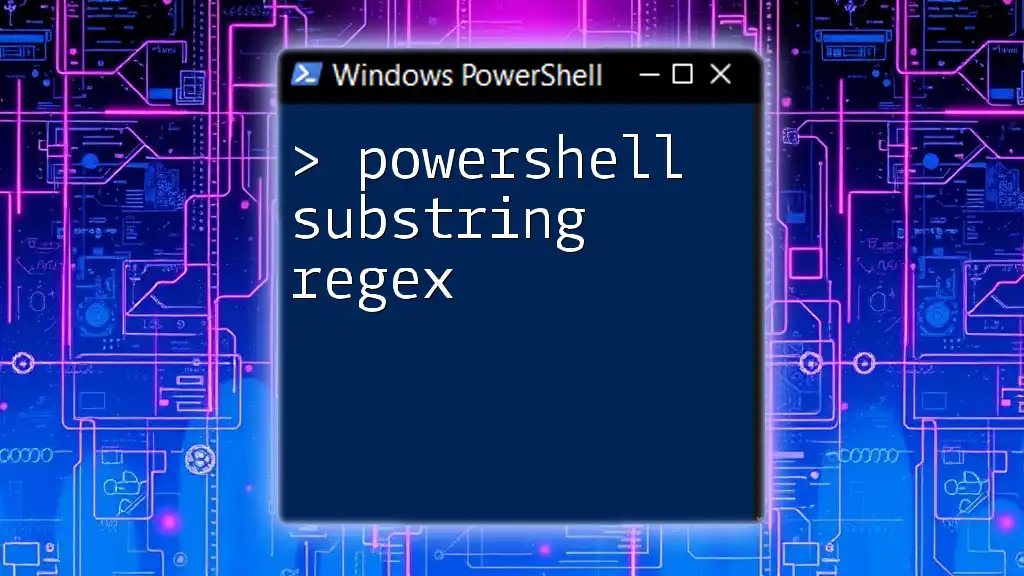
Using PowerShell Substring
Basic Examples of PowerShell Substring
Utilizing the `Substring()` method is easy and can be done with simple examples.
For instance, consider the string "PowerShell Scripting":
$string = "PowerShell Scripting"
$result = $string.Substring(0, 10) # Outputs 'PowerShell'
Here, we've extracted the first 10 characters from the string, resulting in 'PowerShell'.
Another example demonstrates extracting a different section of the string:
$dynamicIndex = 8
$result = $string.Substring($dynamicIndex, 9) # Outputs 'Scripting'
In this case, we dynamically specify the starting index to extract 'Scripting'.
Advanced Techniques with PowerShell Substrings
Nested Substrings
Advanced techniques can further enhance your scripting capabilities. For example, you can use `Substring()` within another `Substring()`, giving you even more flexibility:
$string = "PowerShell Scripting"
$nestedResult = $string.Substring(0, $string.Substring(0, 10).Length) # Outputs 'PowerShell'
This code first extracts the length of the 'PowerShell' substring and then uses that length to extract it again, demonstrating how to manipulate substrings in a nested fashion.
Using Substrings in String Manipulation
PowerShell substrings are also valuable for data transformation and manipulation tasks. For example, if you receive a string formatted as a date, extracting specific portions can be quite helpful:
$inputString = "04-15-2023"
$month = $inputString.Substring(0, 2) # Outputs '04'
In this instance, we extract the month from a date string, which can be critical for various operations like logging or notifications.
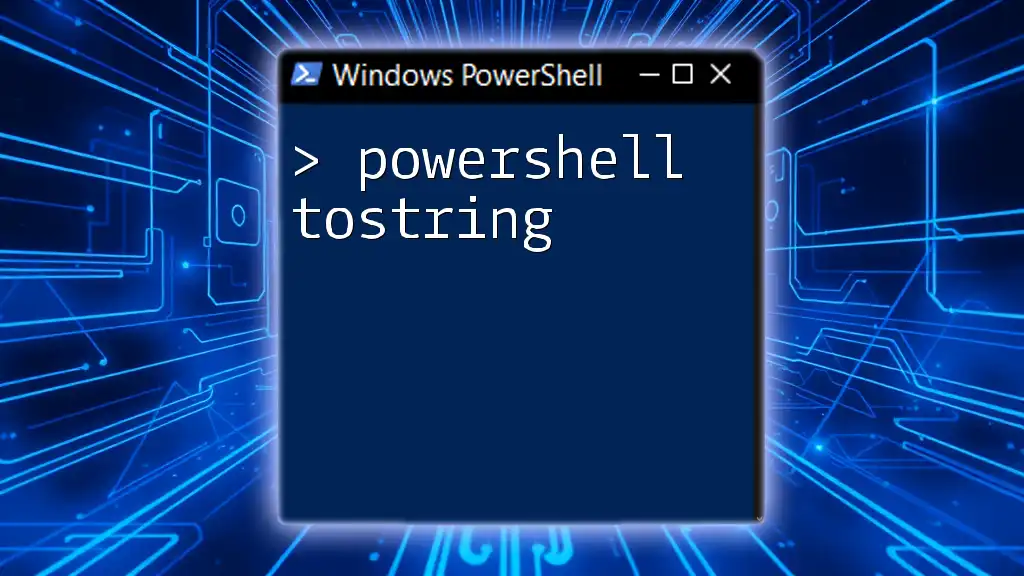
Practical Applications of Substring in PowerShell
Parsing Data
Substrings can be particularly useful when parsing data. For example, if you are working with a CSV string:
$data = "Name, Age, Location"
$firstPart = $data.Substring(0, 4) # Outputs 'Name'
In this case, you can extract the name header from a comma-separated string easily, making data parsing more efficient.
String Formatting
Substrings also prove beneficial in formatting outputs for more complex scripts. For instance, consider this situation:
$fullName = "John Doe"
$firstName = $fullName.Substring(0, 4) # Outputs 'John'
Here, we extract part of a full name, allowing you to format or display data in your preferred manner.
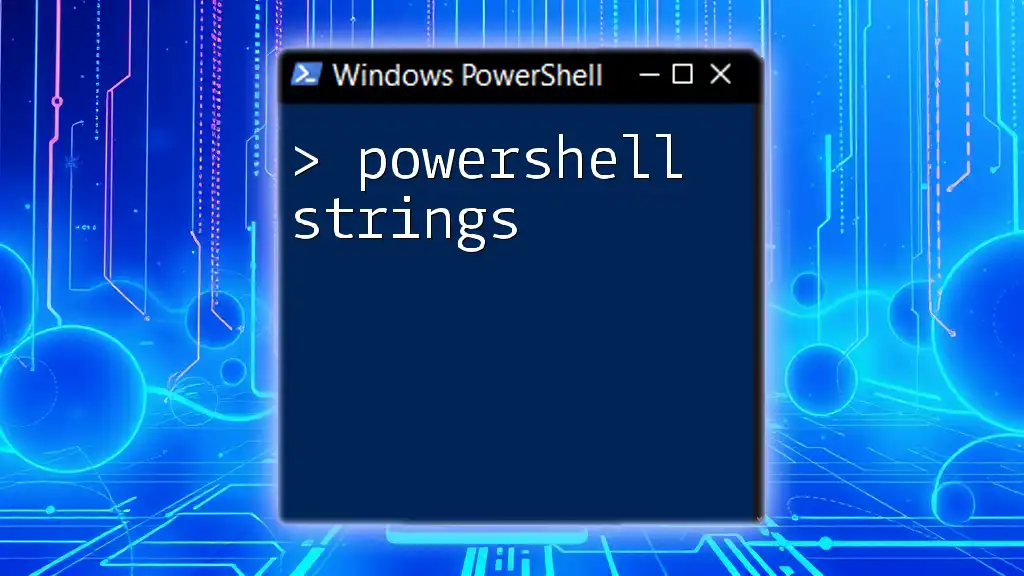
Common Errors and Troubleshooting
Handling IndexOutOfRange Exceptions
One common pitfall when using `Substring()` is not properly understanding the string's length, which can lead to IndexOutOfRange exceptions. Always ensure your starting index is valid:
try {
$errorExample = $exampleString.Substring(15) # Could throw an error
} catch {
Write-Host "Error: " $_
}
In this example, we catch the error and display a message if the starting index is out of bounds.
Validating Index Inputs
To avoid errors, validating indices before executing a `Substring()` operation is a good practice. You can achieve this with a simple check:
if ($startIndex -lt $exampleString.Length) {
$subString = $exampleString.Substring($startIndex, $length)
} else {
Write-Host "Invalid start index."
}
By verifying that your starting index is less than the length of the string, you can prevent runtime errors and make your scripts more robust.
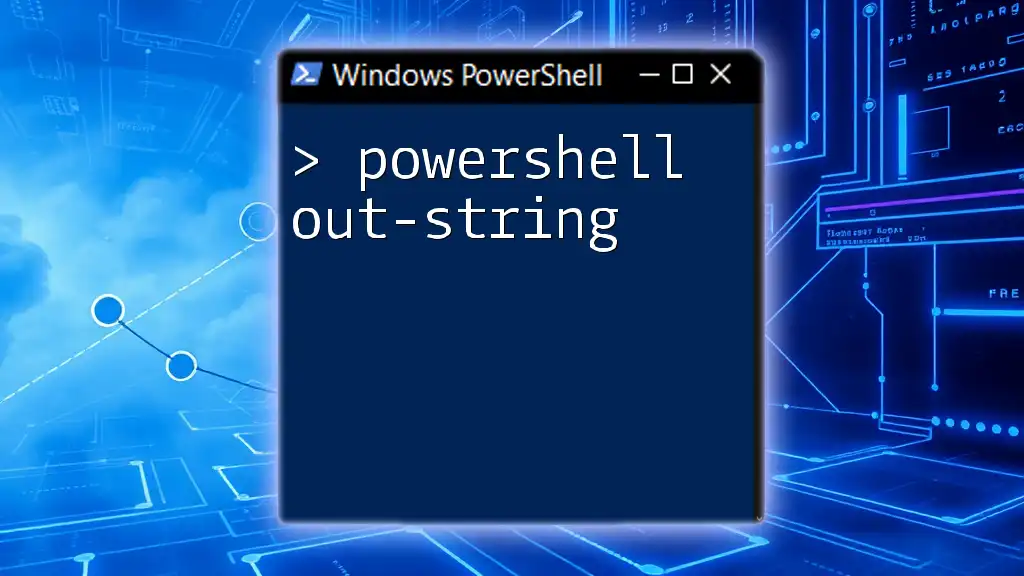
Conclusion
In summary, understanding PowerShell substrings and effectively using the `Substring()` method can significantly enhance data manipulation in your scripts. The ability to extract specific parts of strings opens up various possibilities for parsing, formatting, and handling data.
Feel free to continue experimenting with the `Substring()` method and let your creativity guide your scripting endeavors. As you become more comfortable with this powerful tool, you'll find numerous applications for it in your PowerShell projects.