PowerShell debugging involves identifying and fixing errors in your scripts or commands by using various tools and techniques to monitor code execution and variable values for troubleshooting.
# Enable script debugging in PowerShell
$DebugPreference = "Continue"
# Example command to trigger debugging output
Write-Debug "This is a debug message."
Understanding Debugging in PowerShell
What is Debugging?
Debugging is the process of identifying and resolving errors or bugs in programming code. It involves running the code step-by-step, examining values, and understanding the flow of execution to pinpoint where things go wrong. For PowerShell scripting, debugging is crucial to ensure that scripts perform their intended functions without crashing or producing errors.
Why Debugging is Essential for PowerShell Scripts
Debugging serves several vital roles in PowerShell scripting. It helps to:
- Ensure Smooth Operation: Scripts might work on a development machine but fail in production. Effective debugging ensures that scripts perform as expected in different environments.
- Save Time and Reduce Frustration: The quicker you catch errors, the less time you spend troubleshooting. This efficiency promotes a more enjoyable coding experience.
- Improve Code Quality and Reliability: Well-debugged scripts are less likely to fail, leading to higher quality code that can be relied upon in critical systems.
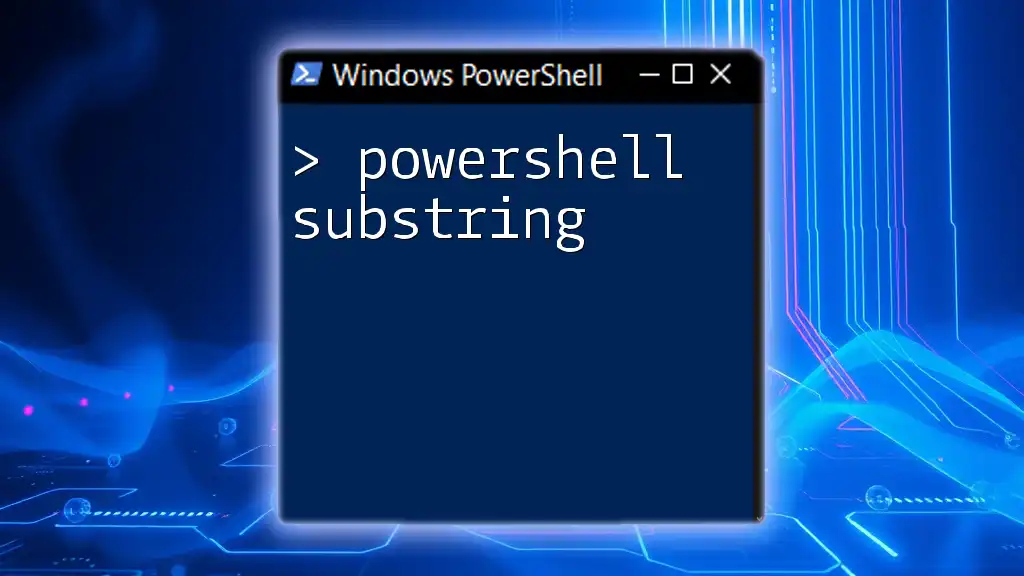
Getting Started with Debugging
Common Types of Errors in PowerShell
Syntax Errors
Syntax errors occur when the code does not conform to the language rules. These mistakes can prevent the script from running entirely. You can spot these errors easily because PowerShell will provide error messages that indicate issues in the script. For example, forgetting a closing parenthesis or using incorrect operators can lead to syntax errors.
Runtime Errors
Runtime errors occur when a script is syntactically correct but fails during execution. For instance, if you attempt to access a variable that hasn't been defined, or if you're trying to divide by zero, PowerShell will throw an error at runtime.
Logic Errors
Logic errors happen when the script runs successfully, but the output is not what was expected. This can often be the hardest type of bug to catch. For example, if your script is supposed to sum two numbers but accidentally multiplies them instead, the script runs without error but produces incorrect results.
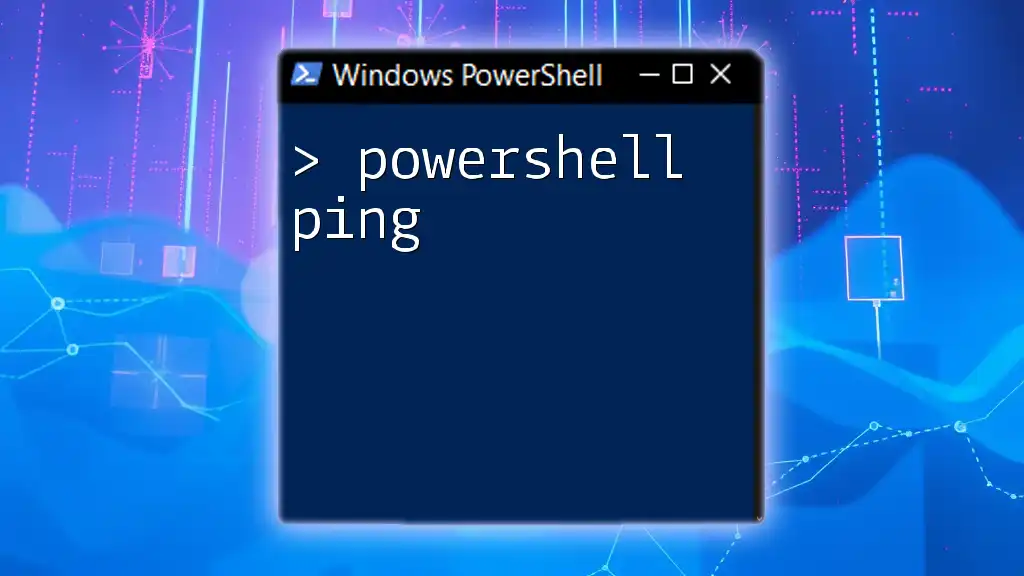
Debugging Techniques
Using Write-Host and Write-Output
One of the simplest ways to debug a PowerShell script is to use `Write-Host` or `Write-Output` to print variable values and program flow. This technique can help you understand how your data is being manipulated at any given time.
Here's an example code snippet that demonstrates this:
$variable = "Debug Test"
Write-Host "Value of variable is: $variable"
Using these commands makes it easy to follow the script's execution and lets you see the values of different variables at crucial points.
Leveraging the PowerShell ISE Debugger
Introduction to PowerShell ISE
The Integrated Scripting Environment (ISE) for PowerShell provides a graphical interface that is particularly helpful for debugging scripts. It offers built-in debugging tools that allow you to view your code in a structured layout.
Step-by-Step Debugging in ISE
One of the powerful features in ISE is that you can set breakpoints, allowing you to pause execution at specific points in your script. This lets you inspect variables and understand the flow of execution better.
To set a breakpoint, you can use the following command:
Set-PSBreakpoint -Script "C:\path\to\your\script.ps1" -Line 10
This command stops execution when it reaches line 10 of your specified script, giving you a chance to analyze the current state of your variables.
Using the `Trace-Command` Cmdlet
The `Trace-Command` cmdlet is another powerful debugging technique in PowerShell. It allows you to trace, or monitor, specific commands and see what's happening during execution.
For example, you can use it like this:
Trace-Command -Name Command -Expression { Get-Process } -PSHost
This command will provide detailed output of what happens when your script runs `Get-Process`. It's particularly useful for understanding complex scripts where multiple functions are involved.
Employing the `Try`, `Catch`, and `Finally` Blocks
What is Exception Handling?
Exception handling is the process of responding to the occurrence of exceptions—unforeseen errors that can crash your script. PowerShell provides `try`, `catch`, and `finally` blocks for this purpose.
Structure of Try-Catch-Finally
Using these blocks allows you to manage errors gracefully rather than letting the entire script fail. Here's an example code snippet:
try {
# Code that may cause an exception
$result = 10 / 0
}
catch {
Write-Host "An error occurred: $_"
}
finally {
Write-Host "This will execute regardless of the outcome"
}
In this example, if you try to divide by zero, the script catches the error and displays a message, while the `finally` block executes regardless of success or failure.
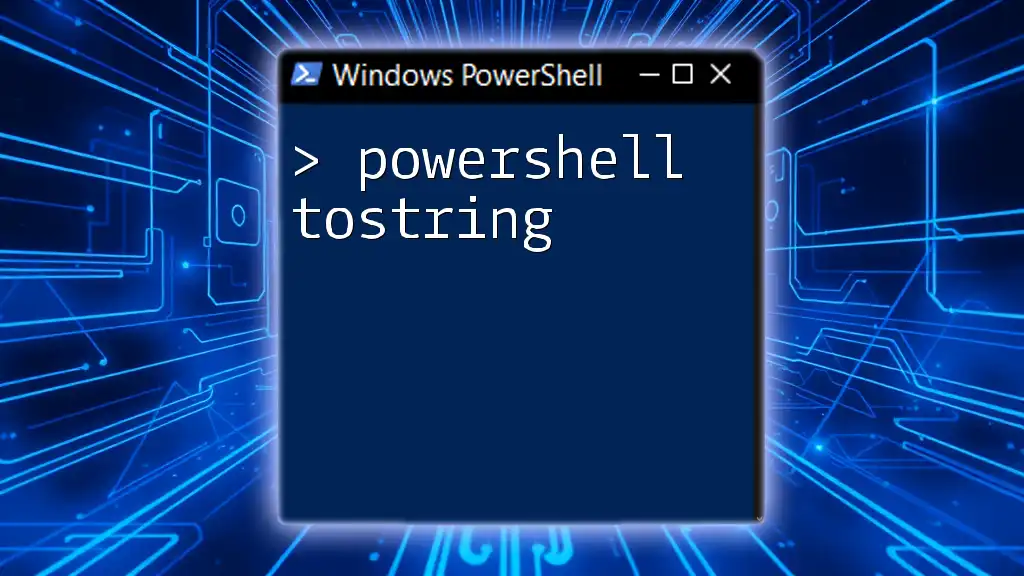
Advanced Debugging Techniques
Using Debugging Tools and Modules
There are several debugging tools and modules that can enhance your PowerShell debugging experience. For instance, PSScriptAnalyzer analyzes your script for potential issues before you even run it, pointing out areas that need improvement. Using such tools can save you significant debugging time in the long run.
Profiling Your Scripts
Profiling your scripts helps you understand performance issues by examining how long different parts of scripts take to execute and whether they consume excessive resources. You can use the `Measure-Command` cmdlet for this purpose.
Here’s how you can measure the execution time of a command:
$executionTime = Measure-Command { Get-Process }
Write-Host "Execution Time: $executionTime"
This command helps you identify areas in your script that may need optimization by providing insights into execution speed.
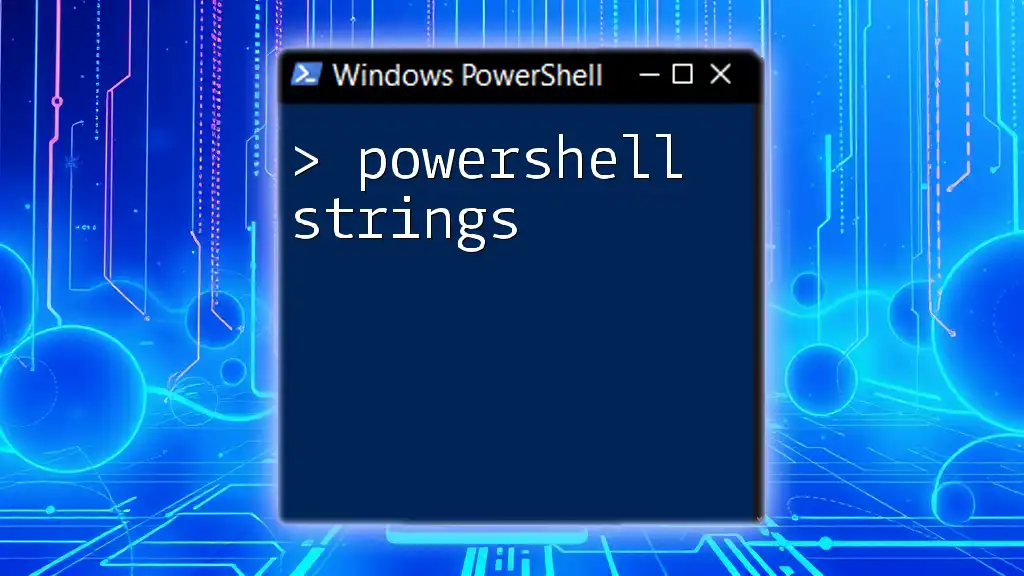
Tips for Effective Debugging
Developing Debugging Habits
One of the best strategies you can adopt for effective debugging is to cultivate good habits while coding. Always write test scripts with debugging in mind. This might include:
- Breaking complex scripts into smaller, manageable functions.
- Adding comments to clarify the purpose of different sections of the code.
Logging for Debugging
Logging is a critical tool for debugging because it allows you to capture the state of your application during execution. This can help immensely when you’re trying to identify what went wrong after an error has occurred.
$logFile = "C:\path\to\log.txt"
Add-Content -Path $logFile -Value "Script started at $(Get-Date)"
In this example, you're logging the start time of a script. You can log key variables and checkpoints throughout your code to identify issues when revisiting a failed script run.
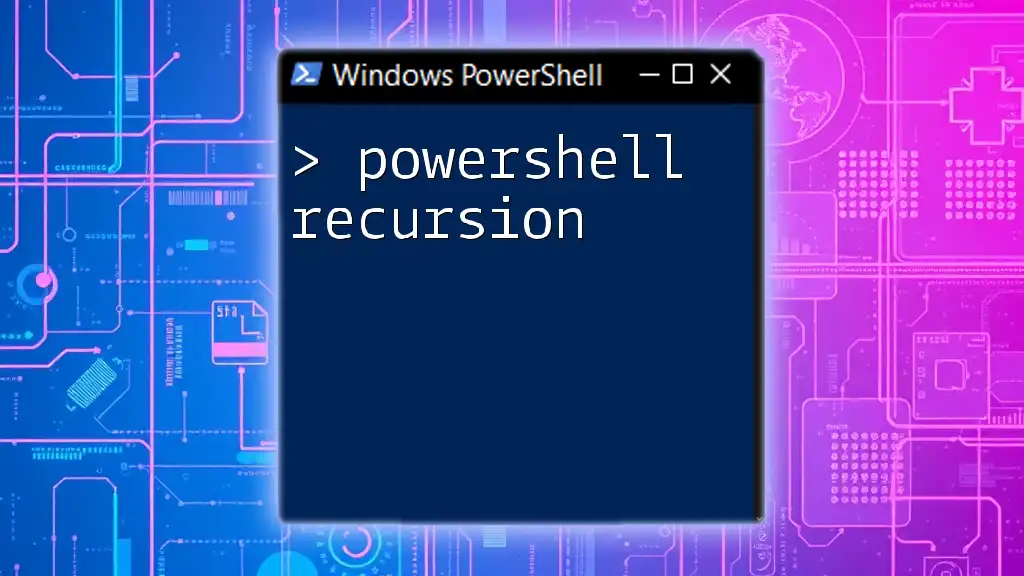
Conclusion
This comprehensive guide to PowerShell debugging provides you with essential techniques and practices that you can utilize to debug your scripts effectively. By understanding different types of errors, employing debugging techniques, and adopting best practices, you'll significantly enhance your PowerShell scripting capabilities. Make debugging a regular part of your development process to ensure that your scripts run smoothly and efficiently. Happy scripting, and don’t hesitate to explore more resources to boost your PowerShell skills!
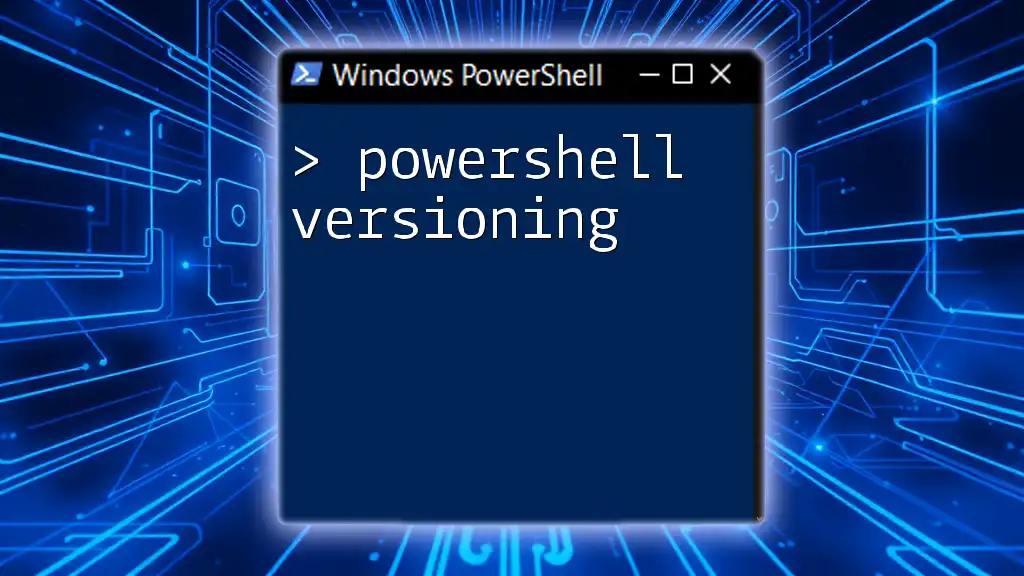
Additional Resources
For further reading and practice, consider checking the official PowerShell documentation, exploring recommended books, and participating in community forums. Engaging with others who share your interest in PowerShell can provide invaluable support as you continue to refine your debugging skills.