PowerShell strings are sequences of characters that can be used to store, manipulate, and display text data within scripts and commands.
Write-Host 'Hello, World!'
What are Strings?
In the context of PowerShell, strings are sequences of characters used to represent text. They form the backbone of most programming and scripting tasks, as they are essential for handling data, messages, and user input. Understanding PowerShell strings is crucial for effective scripting, automation tasks, and data manipulation.
Creating Strings in PowerShell
The creation of strings in PowerShell can be accomplished through two primary methods: using single-quoted and double-quoted strings.
Single-quoted Strings
A single-quoted string is treated literally in PowerShell. This means that variables inside the string are not expanded or interpreted—they appear as plain text.
For example:
$greeting = 'Hello, World!'
Write-Host $greeting
In this case, the output will be: `Hello, World!`.
If you need to include a single quote within the string itself, you can use an escape character (`'`) in front of it:
$quote = 'It''s a beautiful day!'
Write-Host $quote
Double-quoted Strings
Double-quoted strings allow for variable expansion and interpretation of escape sequences, making them more flexible.
For example:
$name = 'Alice'
$greeting = "Hello, $name!"
Write-Host $greeting
Here, the output will be: `Hello, Alice!`.
Additionally, to include special characters (like newlines or tabs), you can do so within double quotes:
$multiline = "Line 1`nLine 2"
Write-Host $multiline
Concatenating Strings
Combining strings (or concatenation) can be done in several ways.
-
Using the `+` operator is straightforward:
$string1 = "Hello" $string2 = "World" $greeting = $string1 + ", " + $string2 + "!" Write-Host $greeting
-
Alternatively, you can use the `-join` operator to join arrays of strings:
$words = ("Hello", "World") $joinedString = $words -join " " Write-Host $joinedString
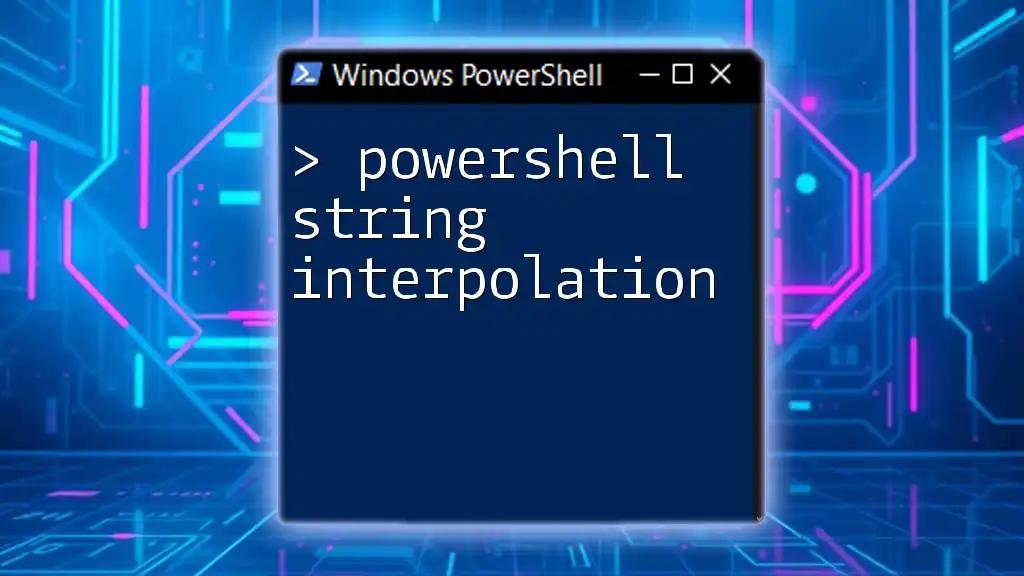
Working with PowerShell String Functions
Overview of String Functions in PowerShell
PowerShell comes with a variety of built-in functions to manipulate strings efficiently, enhancing your ability to perform data processing tasks.
Common PowerShell String Functions
Length
To retrieve the length of a string, you can simply access the `Length` property:
$string = "PowerShell"
$length = $string.Length
Write-Host "Length: $length"
This will display: `Length: 10`.
Substring
The `Substring` method allows you to extract parts of a string based on specified starting positions and lengths:
$string = "PowerShell is great!"
$subString = $string.Substring(0, 6)
Write-Host $subString
Output: `Power`.
Replace
To replace a specific substring within a string, use the `Replace` method:
$string = "Hello, World!"
$newString = $string.Replace("World", "PowerShell")
Write-Host $newString
This gives the output: `Hello, PowerShell!`.
Split
The `Split` method separates a string into an array based on a delimiter:
$string = "PowerShell is awesome"
$array = $string.Split(" ")
Write-Host $array[1] # Outputs: is
Advanced String Manipulations
Trimming Strings
To remove unwanted characters from the start and end of a string, use the `Trim` method:
$string = " PowerShell "
$trimmed = $string.Trim()
Write-Host "Trimmed: '$trimmed'"
Output: `Trimmed: 'PowerShell'`.
Changing Case
You can easily convert strings to upper or lower case with methods like `ToUpper` and `ToLower`:
$string = "PowerShell"
$upper = $string.ToUpper()
$lower = $string.ToLower()
Write-Host "Upper: $upper, Lower: $lower"
This will display: `Upper: POWERSHELL, Lower: powershell`.

Format Strings in PowerShell
String Interpolation
String interpolation in PowerShell allows for dynamic variable inclusion within strings:
$name = "Alice"
$greeting = "Hello, $($name)!"
Write-Host $greeting
Output: `Hello, Alice!`.
Using the `-f` Operator
The `-f` operator allows for more complex string formatting:
$name = "Bob"
$formattedString = "Hello, {0}!" -f $name
Write-Host $formattedString
This results in: `Hello, Bob!`.

Regular Expressions with Strings in PowerShell
Introduction to Regular Expressions
Regular expressions (regex) are patterns used to match character combinations in strings. They are powerful tools for searching, validating, and replacing strings.
Using Regex in PowerShell
In PowerShell, regex can be applied easily to search for matches:
$string = "The quick brown fox"
if ($string -match "quick") {
Write-Host "Match found!"
}
Extracting Data with Regex
You can extract specific data using regex with the `Select-String` command:
$text = "My email is example@mail.com"
if ($text -match "(\w+@\w+\.\w+)") {
Write-Host "Email found: $($matches[0])"
}
This will output the detected email address.

Common Use Cases for PowerShell Strings
Data Validation
Strings play a vital role in validating user inputs and formatting data correctly. For instance, checking if a string represents a valid email format can be accomplished conveniently with regex.
File Manipulation
Dynamic string creation is essential when dealing with file names and paths. You can construct file names based on user input or other parameters seamlessly with strings.
Scripting Best Practices
Efficient string handling in scripts improves readability and maintainability. Utilizing built-in string functions can help minimize errors and enhance the functionality of your PowerShell scripts.

Conclusion
In summary, mastering PowerShell strings is fundamental to becoming proficient in scripting and automation. This guide has covered the creation, manipulation, and formatting of strings, along with providing insights into string functions and the use of regex. Practice these concepts regularly to enhance your PowerShell skills and efficiency!

Additional Resources
For further exploration and deeper understanding, consider referring to the official PowerShell documentation and interactive learning platforms that focus on string manipulation. Happy scripting!