In PowerShell, you can find a substring within a string using the `-like` operator or the `.Contains()` method, which allows for efficient searching and comparison.
Here's a code snippet demonstrating both approaches:
# Using -like operator
$string = "Hello, World!"
if ($string -like "*World*") {
Write-Host "Substring found using -like!"
}
# Using .Contains() method
if ($string.Contains("World")) {
Write-Host "Substring found using .Contains()!"
}
Understanding Strings in PowerShell
What is a String?
In programming, a string is a sequence of characters used to represent text. In PowerShell, strings are pivotal for processing and manipulating textual data. You can create strings in PowerShell using either single quotes (`'`) or double quotes (`"`). While single quotes treat everything literally, double quotes allow for variable interpolation and escape sequences.
Creating and Manipulating Strings
Creating a string in PowerShell is straightforward. For example:
$string1 = "Hello, World!"
You can easily manipulate strings using concatenation and interpolation. To concatenate two strings, simply use the `+` operator:
$string2 = $string1 + " How are you?"
For interpolation, you can include variables directly in double-quoted strings:
$name = "Alice"
greeting = "Hello, $name!"
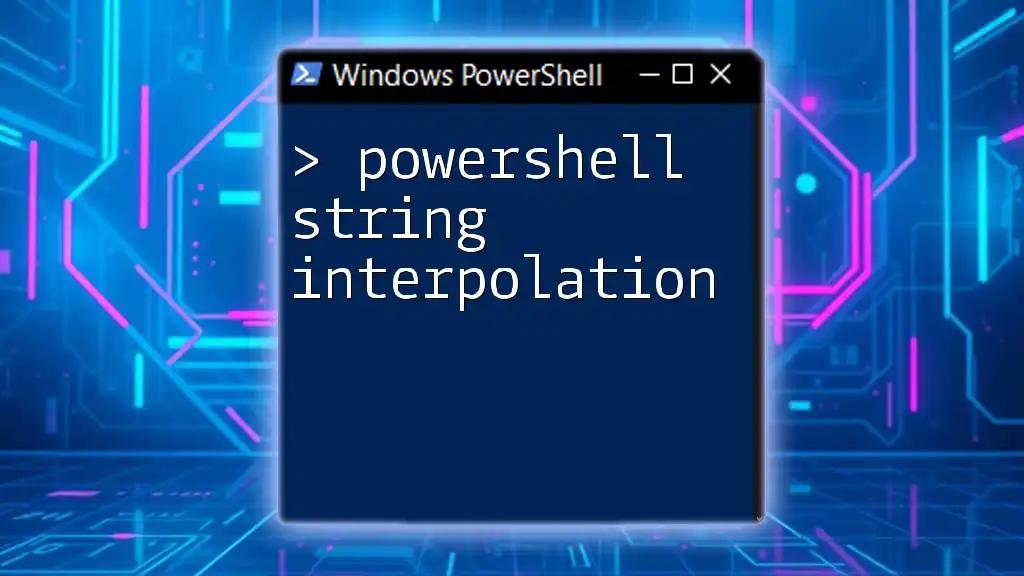
The Need for String Search
Real-Life Applications of String Searching
Searching through strings is essential in numerous real-life applications. For example, system administrators rely on string searches for log analysis, where they sift through logs for specific keywords. Similarly, data scientists may use string searches for data validation to ensure integrity within datasets.
Common Scenarios for Using String Find
Here are a few common scenarios where string find operations are useful:
- Extracting specific data from text files during data processing.
- Searching configurations to validate settings.
- Monitoring system logs to identify warnings and errors.
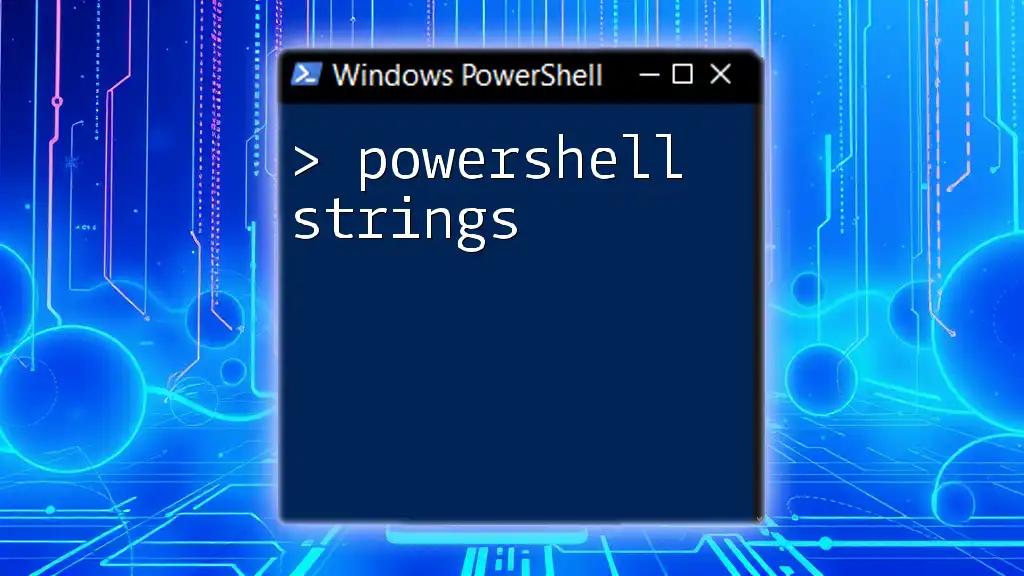
Using Find Methods in PowerShell
Introduction to Finding Strings
PowerShell provides various methods for finding strings, each suitable for specific use cases. Understanding these methods will enhance your string manipulation skills and enable you to perform targeted searches effectively.
Using the `-like` Operator
The `-like` operator is a simple way to perform string comparisons with wildcard characters. It is particularly useful for checking if a string contains a specific pattern.
Here's an example of using the `-like` operator:
if ($string -like "*World*") { "Match found!" }
In this example, the script checks if `$string` contains the substring "World". Wildcards (`*`) denote that there can be any characters before or after the specified string.
A practical application of `-like` is filtering content from a file:
Get-Content "file.txt" | Where-Object { $_ -like "*Error*" }
This script retrieves lines from "file.txt" that contain the word "Error".
The `Contains()` Method
The `Contains()` method of string objects allows you to determine whether a specific substring exists within a string.
$string = "PowerShell is great!"
$found = $string.Contains("great")
In this code, `$found` will be `$true` if "great" is found in `$string`. This method is efficient for searching and works well when you need a straightforward yes/no answer.
The `IndexOf()` Method
If you need to find the position of a substring, `IndexOf()` is your go-to method. It returns the index of the first occurrence, or `-1` if not found.
$index = $string.IndexOf("is")
if ($index -ne -1) { "Found at index $index" }
This snippet checks where "is" occurs in `$string`, indicating that it has been located if the returned index is not `-1`. This method is helpful for string manipulation tasks where knowing the position is paramount.
The `-match` Operator
The `-match` operator enables powerful regular expression searches. This operator returns a Boolean indicating whether a pattern is found within the string.
Here’s an example:
if ("Test123" -match "\d+") { "Number found" }
In this case, the regex pattern `\d+` successfully matches the digits in Test123, and the message "Number found" will be displayed. Use this operator when you require more complex pattern matching, such as validating email formats or extracting formatted data.

Practical Examples
Example 1: Searching Text Files
Imagine you need to find specific phrases in text files. You can create a simple command to do this:
Get-Content "example.txt" | Where-Object { $_ -match "error" }
This retrieves lines from "example.txt" that contain the text "error," which is crucial for troubleshooting.
Example 2: Filtering System Logs
If you want to filter event logs for critical errors, you can use:
Get-EventLog Application | Where-Object { $_.Message -like "*Critical*" }
This command scans the Application log for any entries marked as "Critical," allowing you to quickly identify severe issues.
Example 3: Dynamic Searches
You can also craft dynamic search functionality. For instance, the following function allows users to search for a string in a specified file:
Function Search-String {
param (
[string]$SearchTerm,
[string]$Path
)
Get-Content $Path | Where-Object { $_ -contains $SearchTerm }
}
This function can be called with different search terms and file paths, making it versatile for various applications.

Performance Considerations
Understanding Performance Impact
When executing string find operations, it’s vital to consider performance, especially with large datasets. Certain methods, like using `-match`, can be slower than `-like` due to regex processing overhead.
Tools to Analyze Performance
To evaluate the performance of your string operations, consider using profiling tools such as the `Measure-Command` cmdlet, which helps determine execution time, allowing you to optimize where necessary.

Conclusion
In this guide, we’ve covered the essentials of using PowerShell string find commands, exploring various methods including `-like`, `Contains()`, `IndexOf()`, and `-match`. These tools empower you to efficiently search and manipulate strings—vital skills in any PowerShell user's toolkit. Practicing these techniques will enhance your ability to handle string searches in real-world scenarios.

FAQ
What is the difference between `-like` and `-match`?
While `-like` uses wildcard characters for comparisons, `-match` employs regular expressions for more complex pattern matching. Choose `-like` for simple substring searches and `-match` for powerful regex needs.
Can I search for multiple strings at once?
You can indeed search for multiple strings simultaneously by utilizing arrays and combining them with loops or conditional statements.
How can I perform a case-insensitive search for strings?
For case-insensitive searches, you can use the `-ieq` operator for equality or the `-ilike` operator, which is a case-insensitive version of `-like`:
if ($string -ilike "*world*") { "Match found!" }
By implementing these techniques and understanding the various methods available, you can confidently navigate the world of string manipulation in PowerShell.