In PowerShell, string matching can be efficiently performed using the `-match` operator, which allows you to determine if a specified pattern exists within a string.
Here’s a simple code snippet demonstrating this:
$inputString = "Hello, World!"
if ($inputString -match "World") {
Write-Host "Match found!"
} else {
Write-Host "No match."
}
Understanding String Matching in PowerShell
What is String Matching?
String matching refers to the process of searching for specific sequences of characters within strings. In PowerShell, strings are essential elements that represent text data, variables, or even the contents of files. PowerShell's string handling capabilities empower users to perform various operations like searching, replacing, and manipulating strings, making it a powerful ally for system administrators and automation scripts.
Why is String Matching Important?
String matching is invaluable in many scenarios. For example, when analyzing logs, filtering user inputs, or transforming data, it is vital to be able to identify specific strings or patterns. From searching for error messages in large log files to validating user inputs, effective string matching can save time and improve script performance.

PowerShell String Match Techniques
Using the `-match` Operator
The `-match` operator is one of PowerShell’s primary tools for regular expression (regex) string matching. When you use this operator, PowerShell evaluates whether a string contains a specified regex pattern.
Syntax: The basic format looks like this:
$string -match "pattern"
Example: To check if the string "Hello, World" contains the word "World":
$string = "Hello, World"
if($string -match "World") {
"Match found!"
}
This code snippet will output "Match found!" because the string includes the specified pattern. It’s important to note that with `-match`, PowerShell uses case-insensitive regex by default.
Explanation:
Use this operator to perform complex matches, leveraging the full power of regex patterns. For example, `.*` can match any characters, and `^` can denote the start of a string.
The `-like` Operator
The `-like` operator is another way to perform string matching, particularly when wildcard patterns are more appropriate than regex. Unlike `-match`, `-like` employs simple wildcard characters: the asterisk (*) represents any sequence of characters, while the question mark (?) represents a single character.
Example: To check if the string "File_2023.txt" ends with ".txt":
$string = "File_2023.txt"
if($string -like "*.txt") {
"This is a text file."
}
This will successfully output "This is a text file." because the asterisk matches any characters before the ".txt" extension.
Conditions and Use Cases:
Use `-like` when you need straightforward pattern matching where regex complexity is unnecessary. For example, if you're simply checking for file extensions or common prefixes, `-like` is often the more appropriate choice.
Leveraging the `-replace` Operator for String Matching
The `-replace` operator allows you to find a match and replace a string or a regex pattern with another string. This operator is incredibly useful for data cleansing and formatting operations.
Example: Suppose you want to change the word "awesome" to "fantastic" in the following string:
$string = "PowerShell is awesome!"
$string -replace "awesome", "fantastic"
This code turns the original string into "PowerShell is fantastic!" demonstrating how easy it is to alter content dynamically.
Discussion:
Using `-replace` can significantly enhance your data processing capabilities in scenarios like formatting strings, standardizing text, or sanitizing input data.
PowerShell String Methods for Matching
Using `Substring` for Partial Matches
The `Substring` method is a string manipulation technique that allows users to extract a portion of a string. This method can often be used when you only need to check for a specific segment of a string.
Syntax: The following shows how to declare the starting index and length:
$string.Substring(startIndex, length)
Example: Here’s how you can take a substring from "PowerShell Learning":
$string = "PowerShell Learning"
$substring = $string.Substring(0, 6) # "PowerS"
The variable `$substring` will now contain "PowerS".
Applications:
The `Substring` method is handy when you know the precise location of the text you want to check. It can be a useful way to break down strings into more manageable parts for further analysis.
Using the `Contains` Method
The `Contains` method is a straightforward way to check if one string is contained within another without using regex or wildcards.
Example: Here’s how you can utilize it:
$string = "Learning PowerShell"
if($string.Contains("Power")) {
"Found Power in the string!"
}
The output will confirm that the substring "Power" exists inside the string.
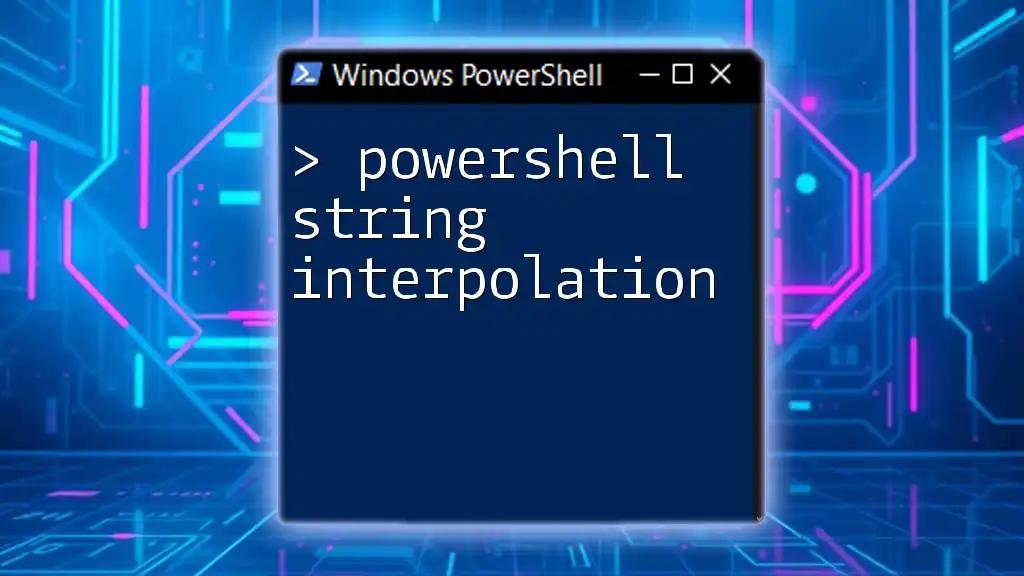
Regular Expressions in PowerShell String Matching
Introduction to Regular Expressions
Regular expressions, or regex, are sequences of characters defining search patterns. They are highly versatile and can be applied in string matching tasks, enabling complex matching behavior far beyond simple substring checks.
Implementing RegEx in PowerShell
To leverage regex in PowerShell, you often employ `-match`, as shown earlier. Here’s a basic example of email validation:
$string = "Email: example@example.com"
if ($string -match "\w+@\w+\.\w+") {
"Valid email format."
}
This piece of code checks if the string matches the common pattern of an email address, reinforcing the utility of regex in PowerShell.
Capturing Groups and Match Results
Capturing groups in regex allow you to isolate specific portions of a matched string. This is useful for extracting data from strings.
Example: Consider extracting an area code from a phone number:
$string = "My phone number is 123-456-7890"
if ($string -match "(\d{3})-(\d{3})-(\d{4})") {
$areaCode = $matches[1]
"Area code is $areaCode."
}
In this example, the area code "123" is captured and stored in the `$areaCode` variable for further use.
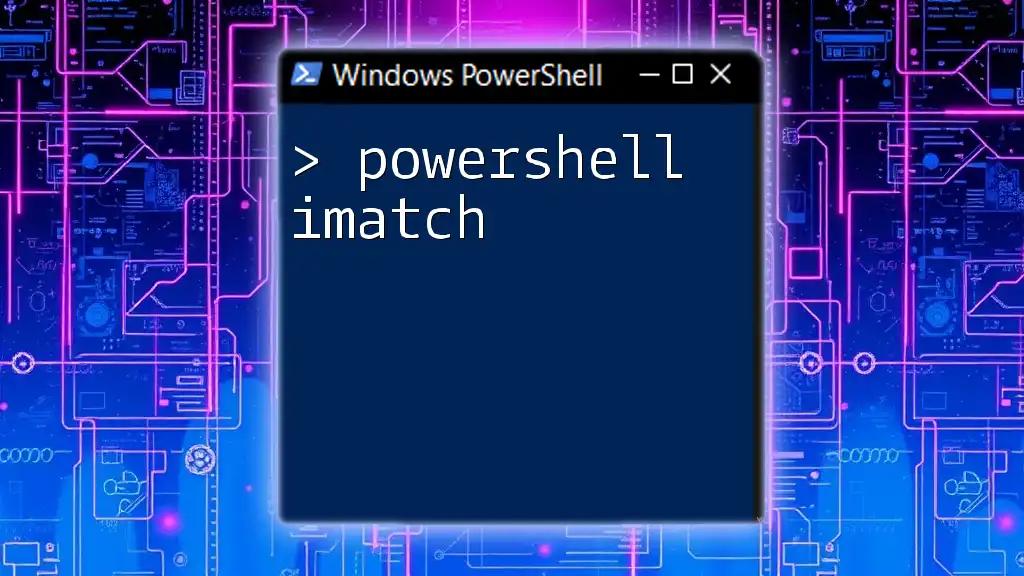
Performance Considerations
Efficiency of String Matching Operations
Understanding performance is crucial when implementing string matching, especially in scripts that handle large volumes of data. String length and complexity can affect the execution time of operations.
Best Practices: To optimize string matching:
- Use `-like` for simple patterns and `-match` for complex regex.
- Minimize the use of regex where simpler alternatives exist.
- When iterating through large datasets, consider structuring your script to limit the number of string operations performed within loops.
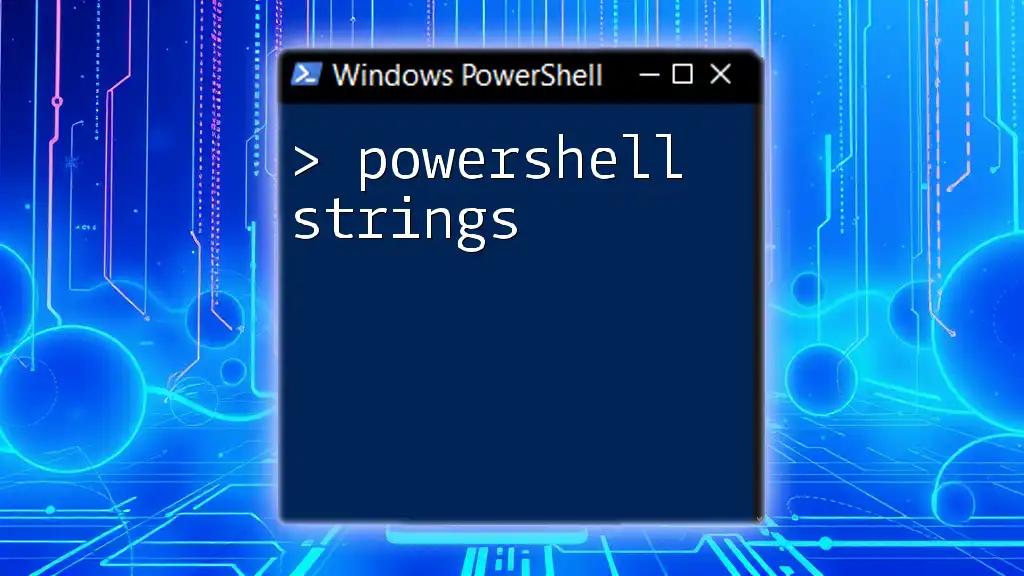
Conclusion
This guide has unpacked various methods of performing string matching within PowerShell, highlighting the differences, strengths, and applications of each. Mastering these techniques will enhance your scripting capabilities, allowing for sophisticated data manipulations and improved automation.
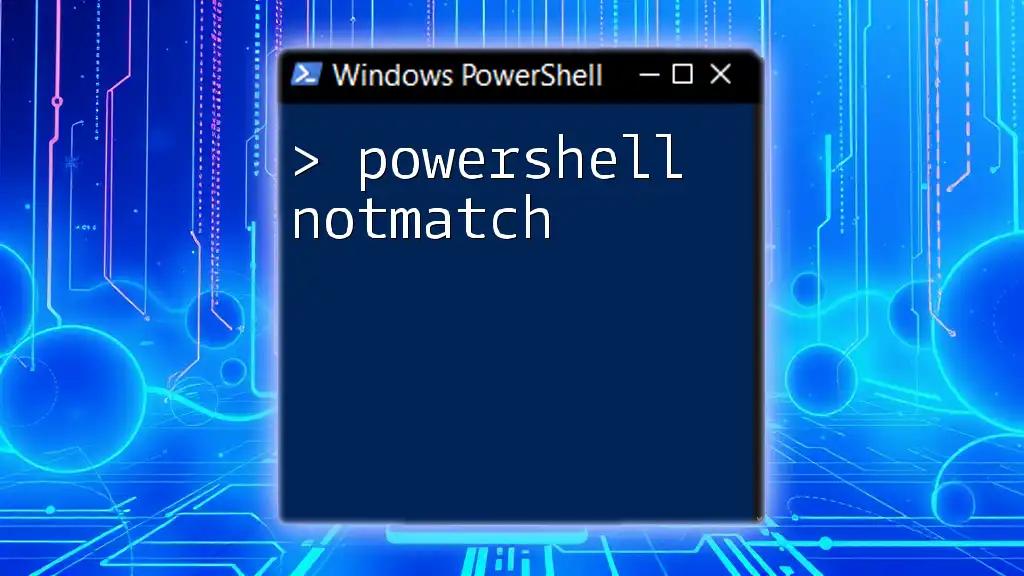
Call to Action
To further enhance your PowerShell proficiency, consider enrolling in advanced courses focused on string manipulation and pattern matching. By practicing these techniques, you will find yourself better equipped to handle various scripting challenges that require powerful string processing capabilities.