PowerShell string interpolation allows you to embed variables directly within double-quoted strings, making it easier to read and write dynamic text output.
$greeting = "Hello"
$name = "World"
Write-Host "$greeting, $name!"
What is String Interpolation?
String interpolation is a mechanism in programming that allows you to embed variable values directly within string literals. Instead of concatenating strings and variables using operators, string interpolation provides a clear and concise syntax for incorporating dynamic values into strings. This approach significantly enhances the readability and maintainability of code.

Why Use String Interpolation in PowerShell?
Utilizing string interpolation in PowerShell offers several advantages:
- Readability: It allows for easy understanding of what the output will be, as variables and expressions are inserted directly into strings.
- Simplicity: String interpolation eliminates the need for cumbersome concatenation operations, making code cleaner and more intuitive.
- Efficiency: It simplifies the syntax needed to create complex strings, reducing the potential for errors.
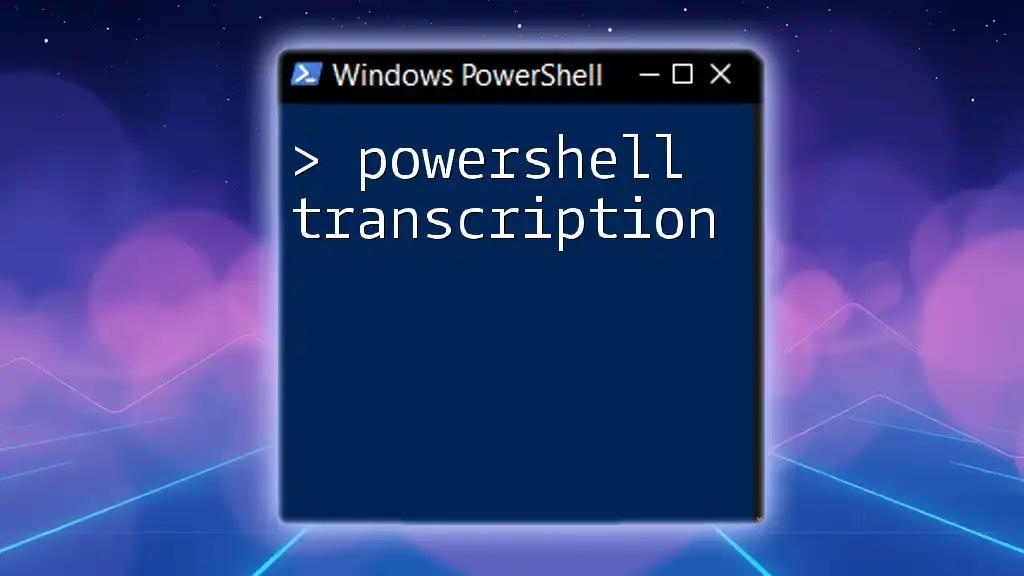
Understanding PowerShell Strings
Types of Strings in PowerShell
In PowerShell, there are two primary types of strings:
- Single-quoted strings: When you use single quotes, PowerShell treats the content as a literal string. It does not evaluate any variables or expressions within the quotes.
- Double-quoted strings: With double quotes, PowerShell will evaluate any variables or expressions. This is where string interpolation comes into play.
Key Differences Between Single and Double Quotes
Understanding when to use single versus double quotes is crucial for effective string interpolation. For instance:
$name = 'World'
"Hello, $name" # Output: Hello, World
'Hello, $name' # Output: Hello, $name
In the above example, the single-quoted string outputs the variable name as plain text, while the double-quoted string outputs its value.
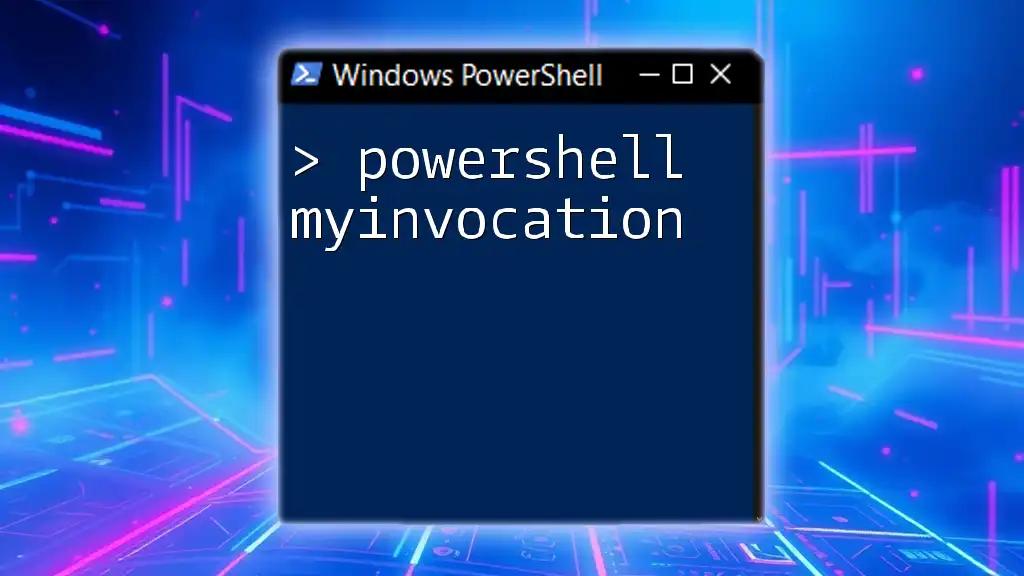
How String Interpolation Works in PowerShell
Basic Syntax of String Interpolation
In PowerShell, string interpolation starts with the dollar sign (`$`). When inside a double-quoted string, a variable prefixed with `$` will be replaced with its current value.
Using Variables in String Interpolation
Embedding variables into strings is straightforward with string interpolation. For example:
$firstName = "Jane"
$lastName = "Doe"
"My name is $firstName $lastName" # Output: My name is Jane Doe
In this example, both `$firstName` and `$lastName` are seamlessly included in the output string.
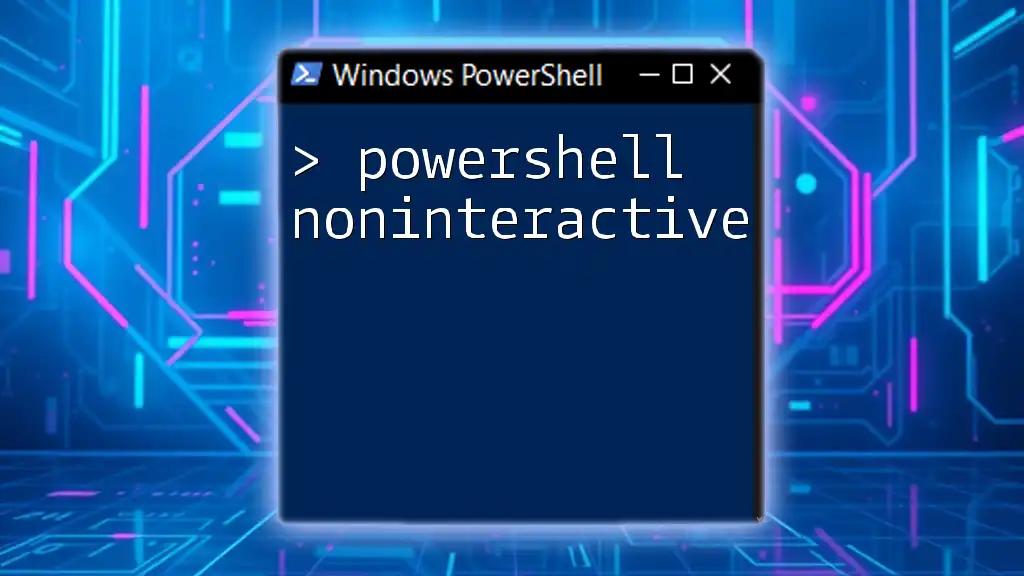
Advanced String Interpolation Techniques
Working with Expressions
PowerShell also allows you to include expressions in string interpolation. You can calculate or manipulate variables within the output string using `$()` syntax. Consider this example:
$x = 10
"The sum of 5 and $x is $(5 + $x)" # Output: The sum of 5 and 10 is 15
Here, `(5 + $x)` is evaluated, and the result is included within the string.
Using Arrays within Interpolated Strings
String interpolation can also handle arrays elegantly. For example:
$colors = @("Red", "Green", "Blue")
"The first color is $($colors[0])" # Output: The first color is Red
In this case, the expression `$($colors[0])` retrieves the first element from the array and seamlessly fits it into the string.

Special Characters and Escaping in PowerShell
Dealing with Special Characters
When working with string interpolation, be aware that certain characters may conflict with the intended output. For example, the dollar sign (`$`) is reserved for variables. To include it literally in an interpolated string, you need to escape it.
How to Escape Special Characters
To escape special characters like `$`, use the backtick character (`` ` ``):
"Dollars: `$125" # Output: Dollars: $125
This technique allows you to present strings accurately without misinterpretation by PowerShell.
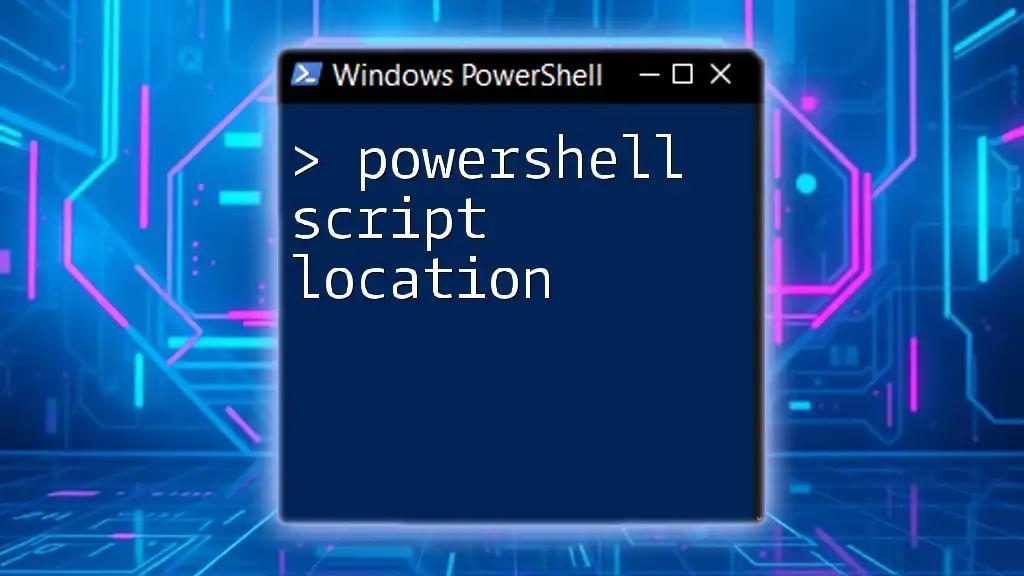
Practical Use Cases for String Interpolation
Creating User-Friendly Messages
String interpolation is often employed to generate clear and informative messages for users. For instance, if you want to inform a user about their account status:
$accountStatus = "active"
"Your account is currently $accountStatus." # Output: Your account is currently active.
In this code snippet, the status message becomes dynamic and informative.
Logging and Debugging Output
Another significant advantage of string interpolation is its utility in logging. Clarity in logs can immensely help during debugging processes. For example:
$logLevel = "INFO"
"[$logLevel] Script executed successfully."
By embedding the log level directly within the output message, it enhances the format and improves promptness in troubleshooting.
Building Command-Line Outputs
Finally, string interpolation shines in scripts that utilize command-line outputs like `Write-Host`. Here’s an example showing how many files are in a directory:
$fileCount = 10
Write-Host "There are $fileCount files in the directory." # Output: There are 10 files in the directory.
This illustrates how string interpolation can dynamically present a message based on computed values.
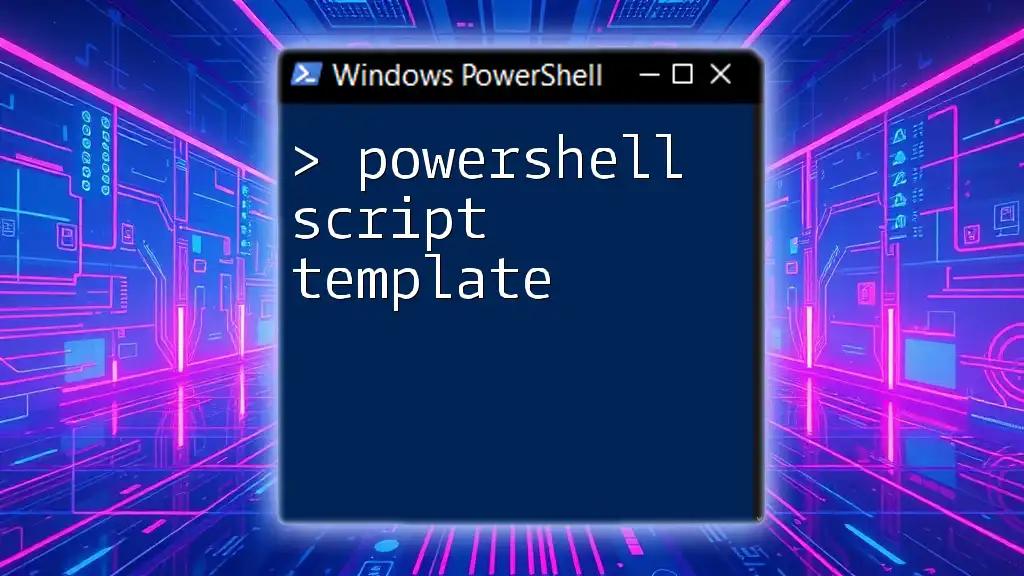
Conclusion
In summary, PowerShell string interpolation provides a powerful and efficient way to create dynamic strings that enhance code clarity. From working with simple variables to incorporating complex expressions and arrays, understanding and utilizing string interpolation can greatly improve your scripting skills.
For further mastery, practice using string interpolation in real scripts and explore the official PowerShell documentation for more advanced techniques and features. Engaging with practical exercises can reinforce your understanding and proficiency.
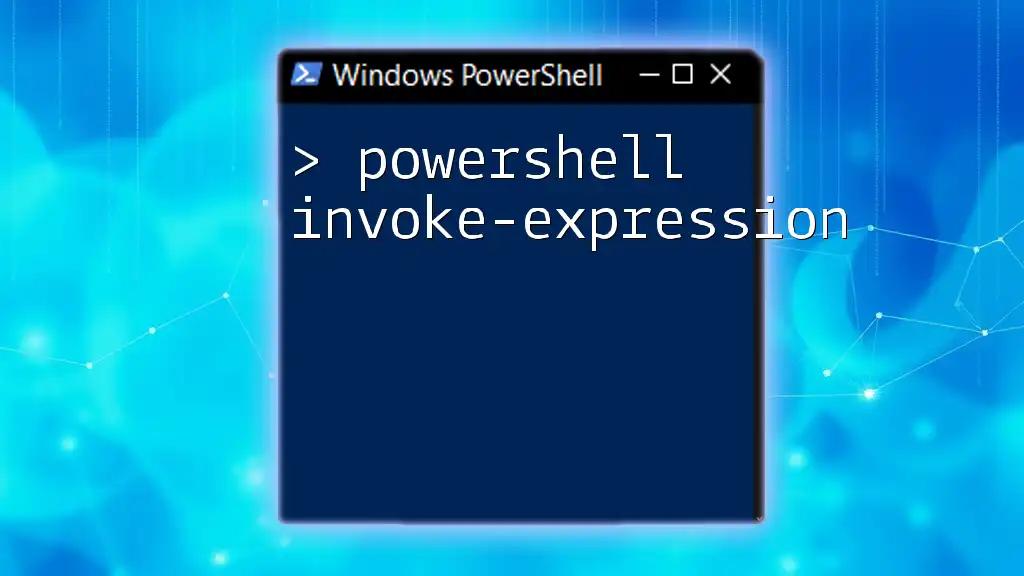
Frequently Asked Questions (FAQs)
What is the difference between string interpolation and string concatenation?
String interpolation allows for the inclusion of variables and expressions directly within a string using a simple syntax. In contrast, string concatenation requires separate operators (like `+`) to join strings and variables, which can be more cumbersome and less readable.
Can string interpolation be used in function parameters?
Yes, string interpolation can be used in function parameters. When passing strings that include variables into functions, enclosing the string in double quotes allows for variable evaluation.
What are some common errors when using string interpolation?
Common errors include:
- Forgetting to use double quotes, which will not evaluate variables.
- Not escaping special characters, resulting in unexpected outputs.
- Misplacing the `$(...)` syntax when trying to include expressions.
By avoiding these pitfalls, you can make full use of string interpolation's capabilities in PowerShell.