The `$MyInvocation` automatic variable in PowerShell provides information about the current command, including its name, location, and the parameters passed to it, allowing you to gain insight into the execution context.
Here’s a code snippet demonstrating its use:
$scriptName = $MyInvocation.MyCommand.Name
Write-Host "This script is called: $scriptName"
Understanding `MyInvocation`
What is `MyInvocation`?
The `MyInvocation` object in PowerShell is a built-in object that provides information about the command currently being executed. This powerful feature allows you to access contextual details about scripts, functions, and their invocations, which can greatly aid in debugging and logging efforts.
Why Use `MyInvocation`?
Using `MyInvocation` can significantly enhance your scripting capabilities. It allows you to understand the context in which your command was executed. This is especially useful in scenarios such as:
- Logging: Keeping track of what scripts and commands have been executed.
- Debugging: Providing insights into where and how your code is invoked.
- Dynamic Responses: Customizing feedback based on how a script is called, enhancing user interaction.
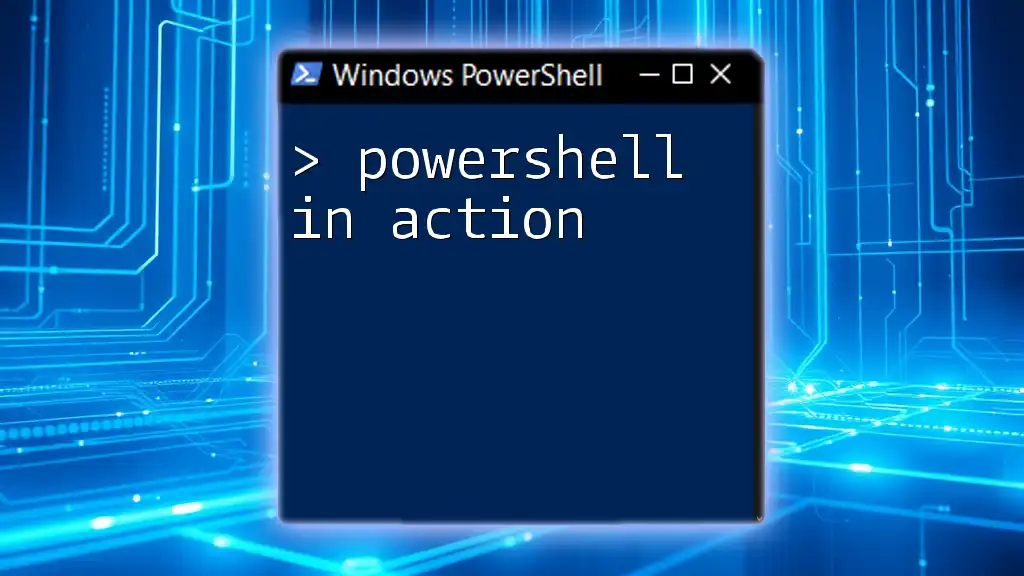
Components of `MyInvocation`
Properties of `MyInvocation`
Each instance of `MyInvocation` contains several properties that offer useful information:
`MyCommand`
The `MyCommand` property represents the command that is currently being executed. It provides detailed information about the command, including its name, path, and parameters.
Example of using this property:
$MyInvocation.MyCommand
This line will return the details of the command currently running, allowing you to inspect and log it.
`InvocationName`
The `InvocationName` property gets the name by which the command was invoked. This is particularly useful for understanding what the user typed or what invoked the script.
Example:
$MyInvocation.InvocationName
This code snippet will return the exact name of the invocation, which can be useful for conditional behaviors or customized output.
`ScriptName`
The `ScriptName` property returns the full path of the script being executed. This information can help you understand the execution context, especially when scripts are called from different directories.
Example:
$MyInvocation.ScriptName
With this, you can easily log or handle operations based on where your script resides.
`Line`
The `Line` property gives the full line of code that the command is executing. This can be useful when troubleshooting or logging the exact input that the script is processing.
Example:
$MyInvocation.Line
Understanding exactly what line of code is being executed can simplify debugging efforts tremendously.
Other Useful Properties
In addition to the core properties listed above, `MyInvocation` has several other useful properties, including:
- `CommandOrigin`: Indicates whether the command was invoked directly or through a pipeline.
- `PositionMessage`: Provides additional information, such as the position in the call stack.
- `ScriptBlock`: Represents the script block being executed. This is particularly useful in advanced scenarios involving closures or nested functions.
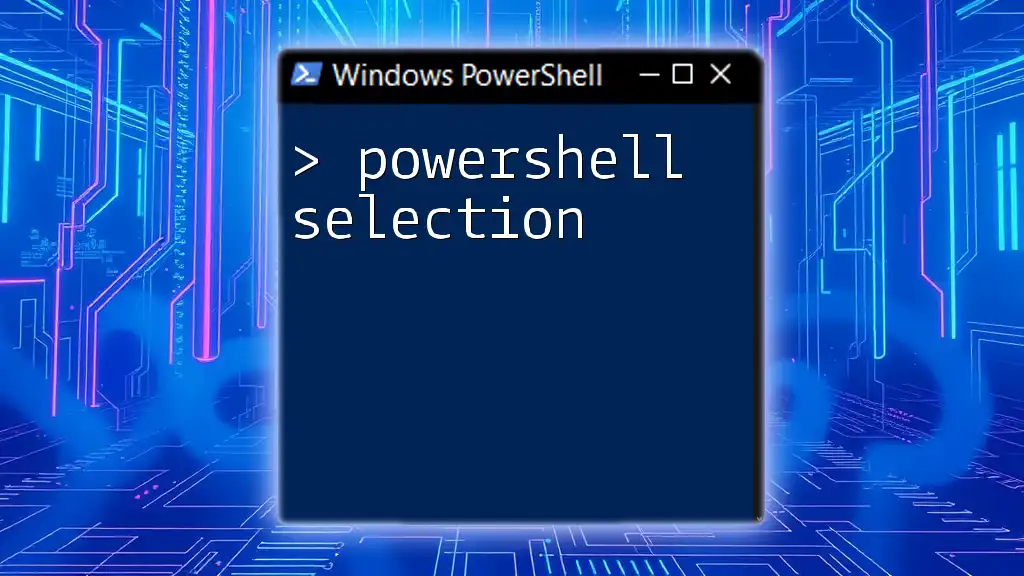
Practical Uses of `MyInvocation`
Accessing Contextual Information
You can use `MyInvocation` to obtain significant contextual data while a script is executing. For instance, here’s a code snippet that utilizes multiple properties of `MyInvocation` to provide a summarized log message:
function Log-Invocation {
Write-Host "Command: $($MyInvocation.MyCommand)"
Write-Host "Invoked as: $($MyInvocation.InvocationName)"
Write-Host "Script Location: $($MyInvocation.ScriptName)"
}
When you invoke this function, it will output a clear message detailing the context of the script execution.
Using `MyInvocation` in Functions
Incorporating `MyInvocation` within functions can enhance your scripts, especially for logging or debugging. Here’s an example that highlights how to log the invocation details whenever a function is called:
function Test-MyInvocation {
Write-Host "This function was called by $($MyInvocation.InvocationName)"
}
When `Test-MyInvocation` is invoked, it will print the invocation name, which can be invaluable for tracking usage in larger scripts.
Handling Nested Functions
When dealing with nested functions, `MyInvocation` behaves contextually. Let’s consider an example:
function OuterFunction {
function InnerFunction {
Write-Host "Inner function called by: $($MyInvocation.InvocationName)"
}
InnerFunction
}
Here, invoking `OuterFunction` will result in `InnerFunction` printing its invocation name, demonstrating how `MyInvocation` can be used in nested scenarios.
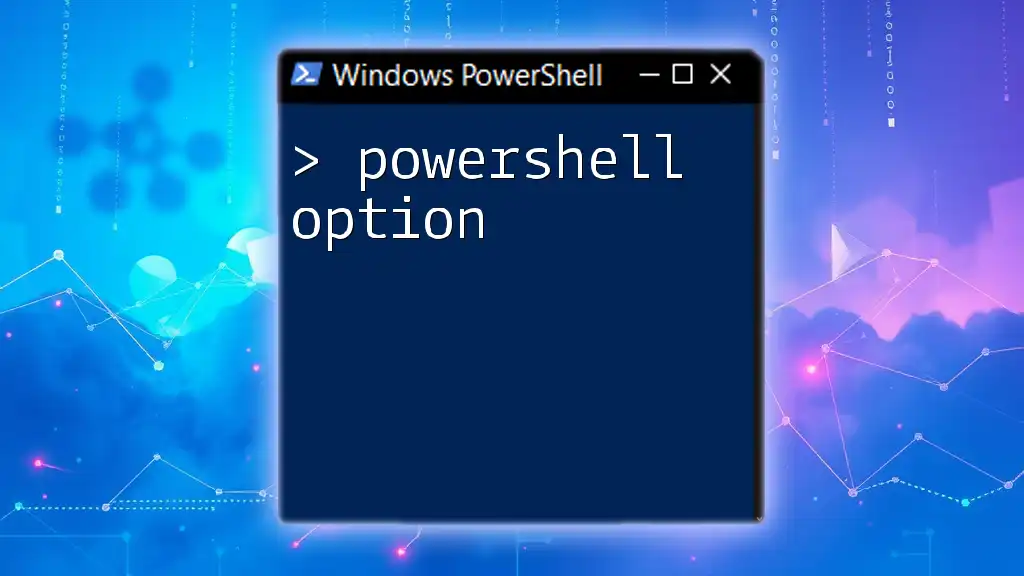
Advanced Techniques with `MyInvocation`
Customizing Output with MyInvocation
You can customize output messages or error handling based on the properties of `MyInvocation`. Here’s an example function that formats its messages according to how it was called:
function Custom-Message {
Param([string]$Message)
Write-Output "$($MyInvocation.InvocationName): $Message"
}
This function will prepend the invocation name to any output message, making it easy to trace where each message originated.
Using `MyInvocation` for Script Validation
Additionally, you can utilize `MyInvocation` to validate whether a script is being executed from a specified location. Here’s a simple example:
if ($MyInvocation.ScriptName -ne "C:\Path\To\YourScript.ps1") {
throw "Script must be run from the specified path!"
}
This snippet ensures that your script operates only when invoked from its designated directory, which can help maintain organization and prevent errors.
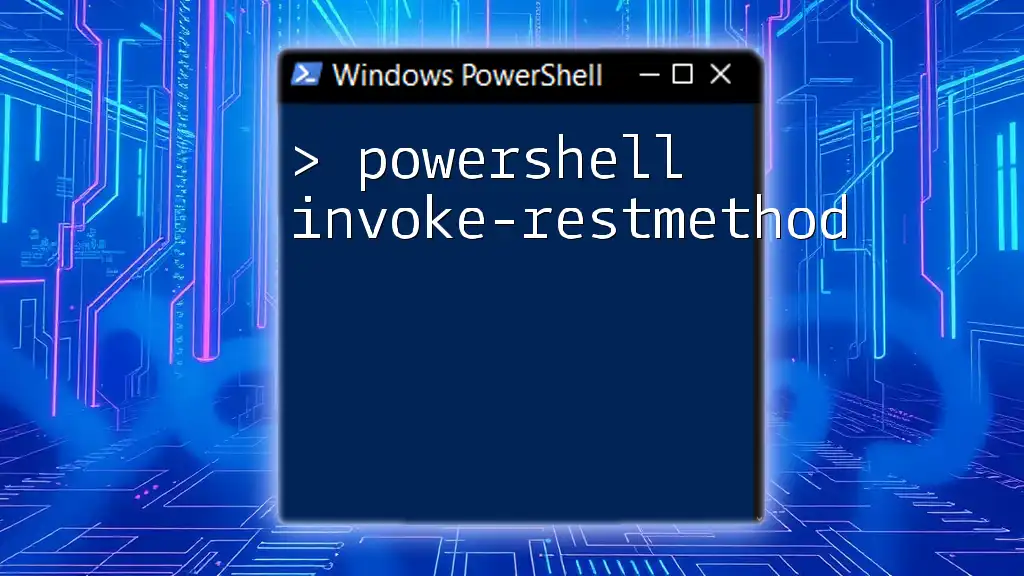
Common Mistakes and Troubleshooting
When utilizing `MyInvocation`, there are common pitfalls that users may encounter:
- Confusing `InvocationName` with `ScriptName`: Remember that `InvocationName` reflects how the command was called, while `ScriptName` indicates the script path.
- Overlooking context in nested functions: Ensure you're aware of the current scope and how `MyInvocation` will reflect that.
Tips for Troubleshooting
- Use simple output statements to check the values of `MyInvocation` properties during debugging sessions.
- Create test cases where you intentionally invoke functions in different ways to see how `MyInvocation` responds.
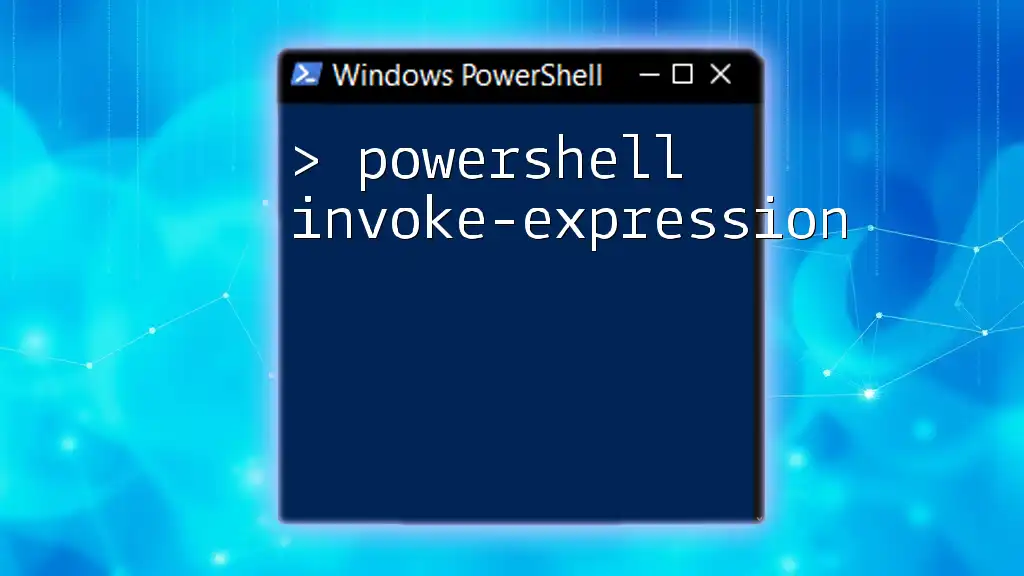
Conclusion
In summary, the `MyInvocation` object in PowerShell is an invaluable resource for any developer. Understanding its properties and how to effectively use them empowers you to write more robust scripts. Whether you are logging context, debugging dynamically, or validating script behavior, `MyInvocation` adds a layer of contextual awareness to your PowerShell commands.
Remember to explore and experiment with `MyInvocation` as you enhance your PowerShell skills. Its capabilities can dramatically improve the quality and maintainability of your scripts.
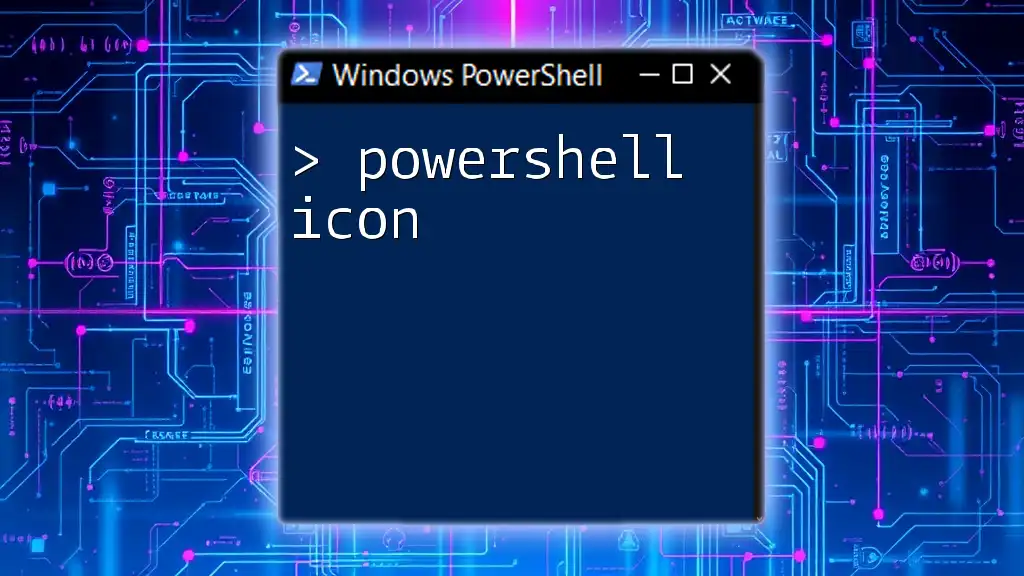
Additional Resources
For further exploration, check out the official Microsoft documentation and consider engaging in dedicated PowerShell tutorials or courses that dive deeper into this and other advanced topics.
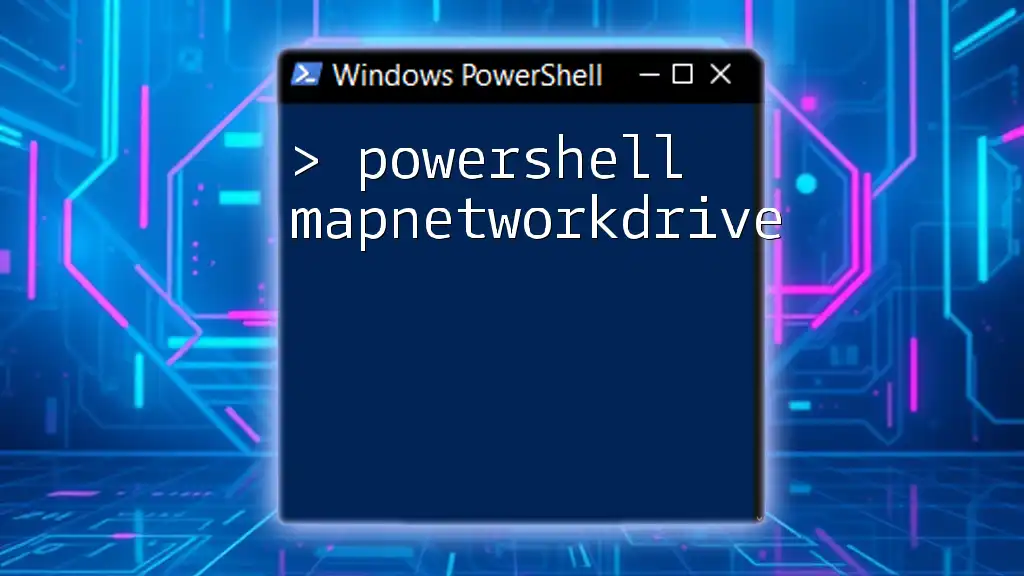
FAQs About MyInvocation
-
What does `MyInvocation` do?
- `MyInvocation` provides information about the command that is currently being executed in PowerShell.
-
Can I use `MyInvocation` in classes?
- Yes, `MyInvocation` can be accessed in any script or function, including those encapsulated in classes.
-
What are the performance implications of using `MyInvocation`?
- The overhead of using `MyInvocation` is minimal and generally negligible in most applications.