"PowerShell in action demonstrates the power of automation and scripting by allowing users to execute commands swiftly and efficiently, such as displaying a simple greeting."
Write-Host 'Hello, World!'
Understanding PowerShell
What is PowerShell?
PowerShell is a task automation framework designed for system administrators and power users. It comprises a command-line shell, a scripting language, and a configuration management framework, enabling users to quickly and effectively manage systems and automate mundane tasks. Originally developed by Microsoft, PowerShell has evolved significantly over the years, leading to its cross-platform availability.
Key Features of PowerShell
PowerShell is unique in that it employs cmdlets—specialized classes designed to perform specific tasks. The functionality of cmdlets is abundant, allowing users to perform operations such as file management, user administration, and server configuration. Additionally, PowerShell's pipeline concept allows the output of one cmdlet to be utilized as input for another, facilitating complex command sequences.
One of PowerShell’s standout features is its object-oriented nature. Unlike traditional command-line environments, where output is simply text, PowerShell works with .NET objects. This means that the information returned from a cmdlet can be manipulated naturally, offering high granularity and control.
Why Use PowerShell?
Using PowerShell offers several advantages over conventional command-line interfaces:
- Efficiency: Automate repetitive tasks to save time and minimize errors.
- Flexibility: It’s adaptable across different systems, making it ideal for diverse environments.
- Rich Functionality: The extensive range of cmdlets provides a powerful toolkit for system management.
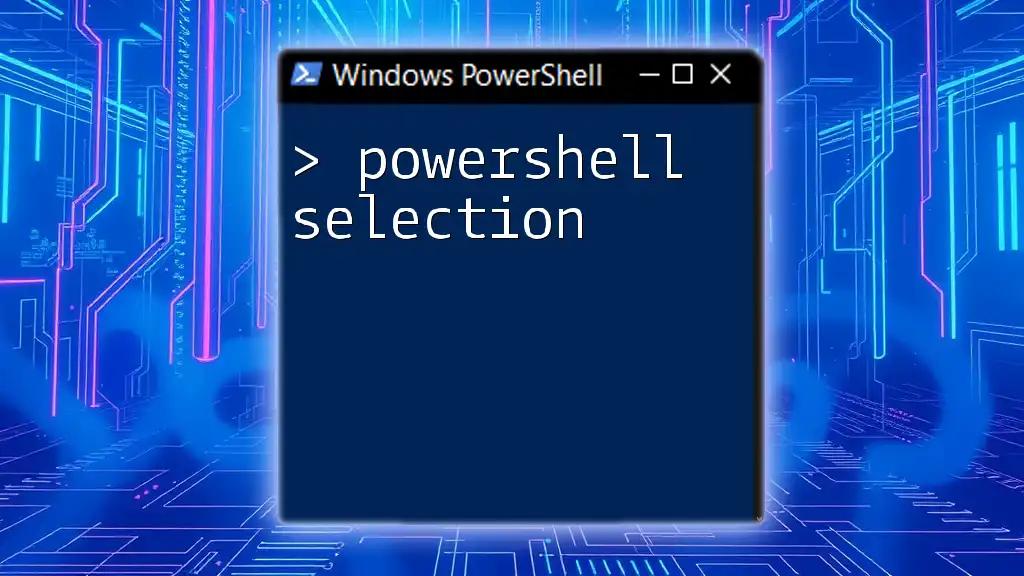
Getting Started with PowerShell
Installing and Setting Up PowerShell
Installing PowerShell is straightforward, with different procedures based on the operating system:
- Windows: PowerShell comes pre-installed with Windows. To access it, search for "PowerShell" in the Start Menu.
- macOS: PowerShell can be installed via Homebrew or by downloading directly from the GitHub repository.
- Linux: Similar to macOS, PowerShell is available through package managers like APT or YUM.
It’s important to understand the difference between Windows PowerShell, which is Windows-only, and PowerShell Core, which is a cross-platform version compatible with macOS and Linux.
Basic PowerShell Commands
Navigation and File Management
Navigating the file system is one of the first skills to master. The `Get-ChildItem` cmdlet can be utilized to list files and directories:
Get-ChildItem -Path C:\Users
This command displays the contents of the "Users" directory, providing an easy reference for file management tasks.
Creating and Removing Files
Creating and removing files can be efficiently handled with `New-Item` and `Remove-Item`. Here’s how to create and delete a file:
New-Item -Path "C:\Temp\TestFile.txt" -ItemType "File"
Remove-Item -Path "C:\Temp\TestFile.txt"
The first command generates a new text file called "TestFile.txt", while the second command removes it, showcasing how easily you can manipulate files in PowerShell.
Using Cmdlets Effectively
Understanding Cmdlet Syntax
Cmdlets follow a verb-noun pattern, allowing users to easily deduce what a command does. It's essential to familiarize yourself with common verb choices such as `Get`, `Set`, `New`, and `Remove`, as they are the foundation of the syntax.
Example: Working with PSObjects
PowerShell's ability to work with objects can enhance your scripts significantly. For example, the following command retrieves a list of processes that are using more than 0.5 CPU:
$process = Get-Process | Where-Object { $_.CPU -gt 0.5 }
This example demonstrates how PowerShell can filter objects, making it a powerful tool for performance monitoring.
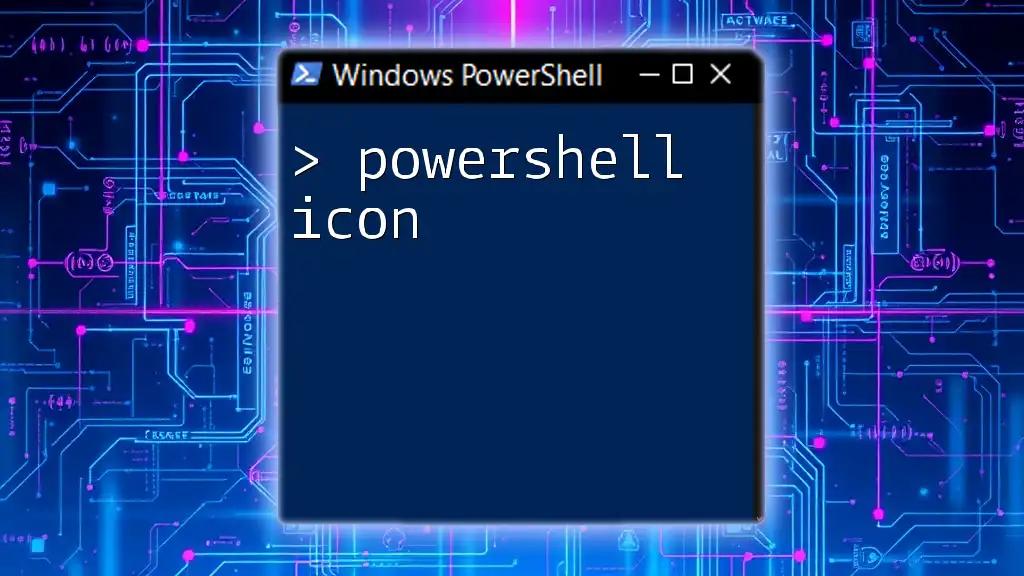
Automating Tasks with PowerShell
Creating Scripts
Writing a script in PowerShell is as simple as creating a plain text file with a `.ps1` extension. Scripts can contain sequences of commands that automate repetitive tasks, allowing for quick execution with improved efficiency.
Scheduling Automation
Task Scheduler Integration
PowerShell scripts can be scheduled through the Windows Task Scheduler, allowing tasks to run automatically at specific intervals. Steps to configure a scheduled task include:
- Open Task Scheduler from the Start Menu.
- Create a new task and set the trigger (daily, weekly, etc.).
- In the Action tab, choose to start a program and enter the path to `powershell.exe`.
- Specify the script's path in the "Add arguments" field.
Example of Creating a Daily Backup Script
A common use case would be creating a script that backs up important files daily. Your script may look something like this:
Copy-Item -Path "C:\Source\*" -Destination "D:\Backup\" -Recurse
Using Loops and Conditionals
PowerShell enables users to implement loops and conditionals to control the flow of scripts. The following example illustrates the use of `ForEach` and `If`:
ForEach ($item in $items) {
if ($item -eq "Target") {
Write-Output "Found Target"
}
}
In this snippet, for each item in `$items`, if the item matches "Target," a message is printed, demonstrating how to exert control over script execution based on dynamic conditions.
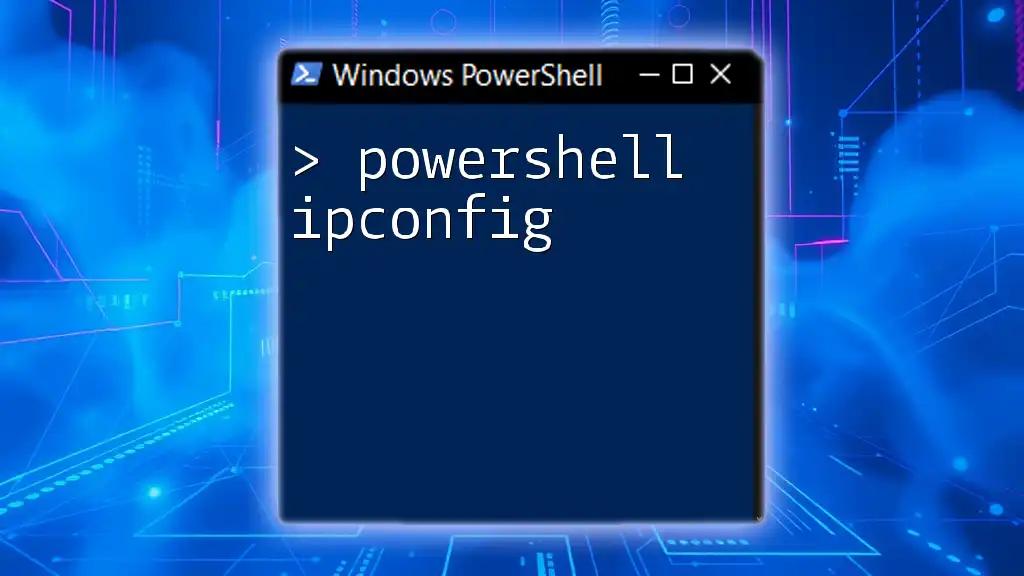
Advanced PowerShell Techniques
Working with Modules
Modules enhance PowerShell's functionality by grouping related cmdlets. To utilize them, simply import the module with the `Import-Module` command:
Import-Module Az
This command imports the Azure PowerShell module, allowing you to manage Azure resources effectively.
Creating Functions
Functions can streamline frequently used code in PowerShell. Defining a function is simple and aids in maintaining clean scripts. Here’s a basic function to get file details:
function Get-FileDetails {
param([string]$path)
Get-Item $path | Select-Object Name, Length, CreationTime
}
This function accepts a file path and outputs its name, size, and creation time.
Error Handling and Debugging
Error handling is key to creating resilient scripts. PowerShell provides `Try`, `Catch`, and `Finally` blocks for structured error management. For example:
Try {
Get-Item "C:\NonExistentFile.txt"
}
Catch {
Write-Host "An error occurred:" $_.Exception.Message
}
In this example, if the file doesn’t exist, an error message is displayed instead of halting script execution.
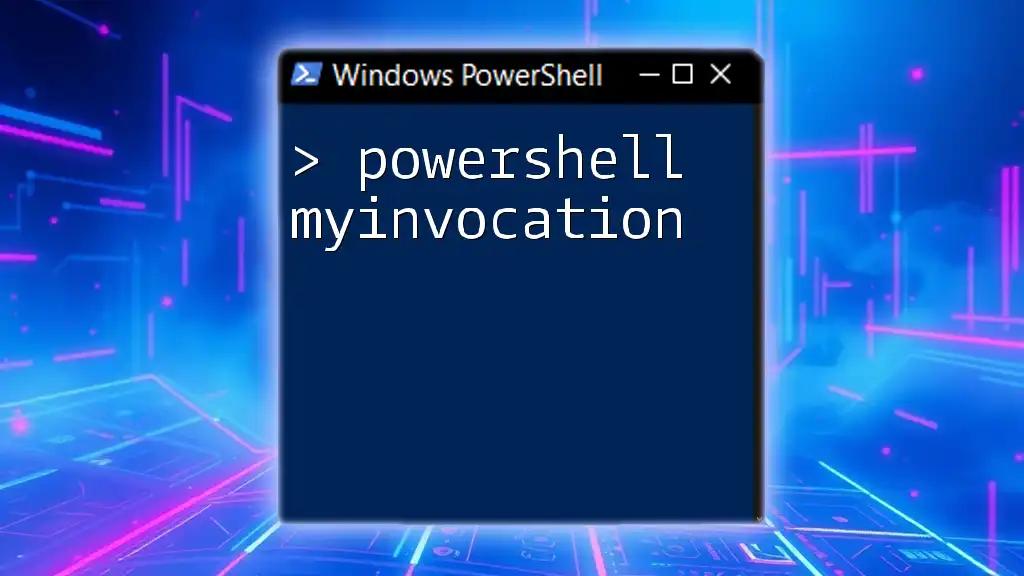
Real-World Applications of PowerShell
System Administration Tasks
PowerShell shines in its ability to streamline system administration tasks. Common applications include:
- User Management: Create, delete, and modify user accounts efficiently.
- System Reporting: Generate detailed reports on system settings and user activity.
Data Manipulation and Reporting
PowerShell excels at data manipulation. Through commands like `Import-Csv`, users can easily import data from external sources and process it:
Import-Csv -Path "data.csv" | Export-Csv -Path "output.csv" -NoTypeInformation
This command reads a CSV file and subsequently exports the data to a new CSV file, streamlining data workflows.
Integrating with Other Technologies
PowerShell's versatility extends to integrating with external systems, such as REST APIs. This capability allows users to fetch or send data dynamically:
$response = Invoke-RestMethod -Uri "https://api.example.com/data" -Method Get
By leveraging this functionality, PowerShell can bridge the gap between local and cloud services.
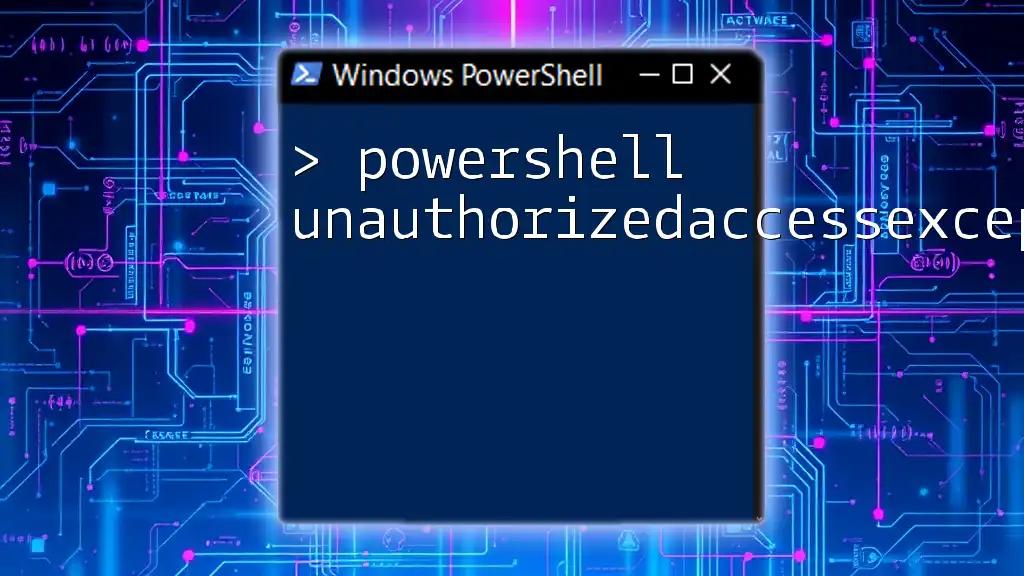
Conclusion
In summary, PowerShell in action reveals a powerful utility for automating tasks and managing systems. With its array of cmdlets, scripting capabilities, and integration options, it stands as an essential tool for system administrators and power users alike. As you delve deeper into PowerShell, you'll uncover endless possibilities for increasing efficiency and streamlining operations.

Additional Resources
For further learning, consider exploring the official PowerShell documentation, engaging with PowerShell forums, or diving into recommended books and online courses designed to enhance your understanding and skills.

Call to Action
To fully harness the power of PowerShell, connect with us for resources, training, and personalized guidance. Together, we can elevate your command-line skills and automate tasks like a pro!