The `if -contains` statement in PowerShell checks if a specified collection contains a particular value, allowing for condition-based execution within scripts.
Here's a code snippet demonstrating its usage:
$fruits = @('apple', 'banana', 'cherry')
if ($fruits -contains 'banana') {
Write-Host 'Banana is in the list!'
}
Understanding the Basics of PowerShell `if` Statements
What is an `if` Statement?
The `if` statement in PowerShell is a fundamental control structure that allows you to execute a block of code based on whether a specified condition is true or false. It is essential for creating dynamic scripts that respond to various circumstances.
The basic syntax of an `if` statement looks like this:
if (condition) {
# code to execute if condition is true
}
Structure of an `if` Statement
A typical `if` statement consists of three main parts:
- The Condition: This is the expression that PowerShell evaluates. If it evaluates to true, the code within the curly braces executes.
- The Curly Braces: These denote the block of code that will execute if the condition is met.
- The Code: This is where you place the actions you want to occur when the condition is true.

Introducing the `-contains` Operator
What is the `-contains` Operator?
The `-contains` operator is used to check whether a collection (like an array) contains a specified item. It returns `True` if the item is found in the collection and `False` otherwise.
This operator is particularly useful when you want to verify if a particular value exists in a list. It's important to note that `-contains` performs a value check, meaning it checks membership rather than equality. Therefore, it is crucial not to confuse it with the `-eq` operator, which checks for equality between two values.
Syntax and Format
The syntax for using the `-contains` operator is straightforward:
$collection -contains $item
For example, to check whether the number 3 is present in an array of numbers:
$numbers = 1, 2, 3, 4, 5
$result = $numbers -contains 3 # This will return True
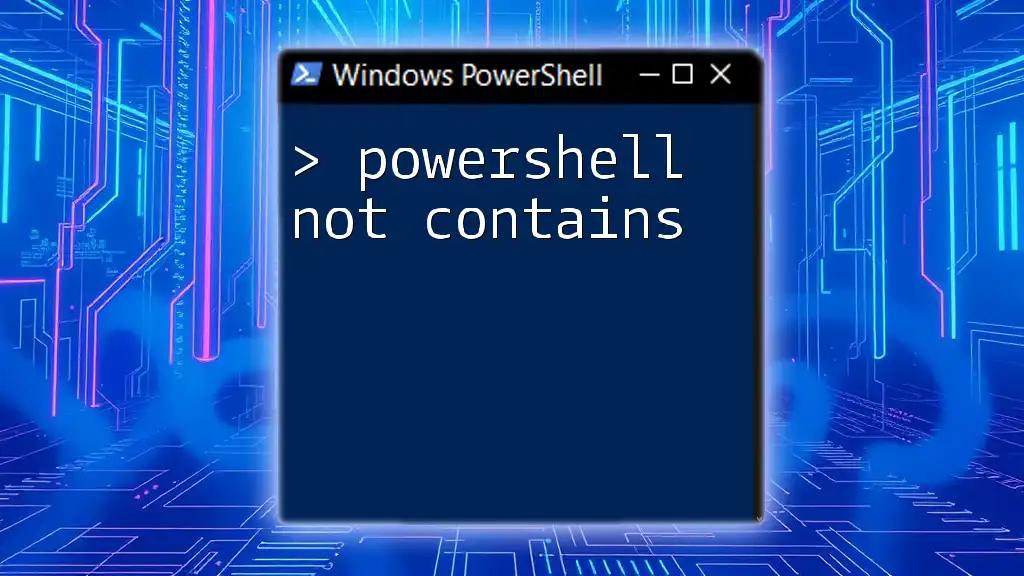
Using `if -contains` in PowerShell
Basic Example of `if -contains`
Let’s consider a simple example to see how `if -contains` works. Imagine you have an array of fruit, and you want to check for the presence of "apple":
$fruits = "banana", "apple", "orange"
if ($fruits -contains "apple") {
Write-Host "Apple is in the list!"
} else {
Write-Host "Apple is not in the list!"
}
In this code, if "apple" is in the `$fruits` array, PowerShell will display `Apple is in the list!`. Otherwise, it will indicate that "apple" is not present.
Practical Applications of `if -contains`
Checking User Input
A common use of `if -contains` is to validate user input. Suppose you want to create a script that only accepts specific commands. You can implement it as follows:
$validCommands = "start", "stop", "restart"
$userInput = Read-Host "Enter a command"
if ($validCommands -contains $userInput) {
Write-Host "Valid command entered: $userInput"
} else {
Write-Host "Invalid command. Please try again."
}
Here, if the user enters a valid command, the script acknowledges it; otherwise, it prompts for a valid command again.
Filtering Data from a List
The `if -contains` operator is also valuable when creating filtered lists based on predetermined criteria, such as filtering a list of user roles:
$roles = "admin", "editor", "viewer"
$checkingRole = "editor"
if ($roles -contains $checkingRole) {
Write-Host "$checkingRole has access to the system."
} else {
Write-Host "$checkingRole does not have access."
}
In this example, if `$checkingRole` matches any value in `$roles`, it prints the appropriate message.

Advanced Usage of `if -contains`
Using `if -contains` within Functions
The power of PowerShell is greatly enhanced by the use of functions. Implementing `if -contains` within a function can help modularize your scripts. Below is an example:
function Check-Access {
param (
[string]$role
)
$allowedRoles = "admin", "editor"
if ($allowedRoles -contains $role) {
Write-Host "$role has access rights."
} else {
Write-Host "$role does not have access rights."
}
}
Check-Access -role "editor" # Call the function with a role
In this function, we can easily check if a role has access, making it reusable for various inputs.
Combining `if -contains` with Other Operators
You can enrich your control structures by combining `if -contains` with other logical operators like `-and` or `-or`. For instance, imagine needing to check if a role is valid and if a user is active:
$activeUsers = "user1", "user2", "user3"
$validRoles = "admin", "editor"
$currentUser = "user2"
$currentRole = "editor"
if ($activeUsers -contains $currentUser -and $validRoles -contains $currentRole) {
Write-Host "$currentUser is active and has the role $currentRole."
} else {
Write-Host "Access Denied."
}
This snippet will only grant access if both conditions about the user and role are true.

Common Errors and Troubleshooting
Common Mistakes with `-contains`
One frequent mistake when using the `-contains` operator is to misuse collections. Remember that `-contains` only evaluates arrays or collections. If you try to use it directly on strings or unsupported data types, you might not get the expected result. For example:
"apple" -contains "a" # This will not work as expected.
Debugging Techniques
When your `if -contains` conditions don't function as intended, consider using `Write-Host` or `Write-Output` to help debug your code. This can help you track variable values and control flow within your script.
For example, insert debugging statements:
Write-Host "Checking if $item is contained..."
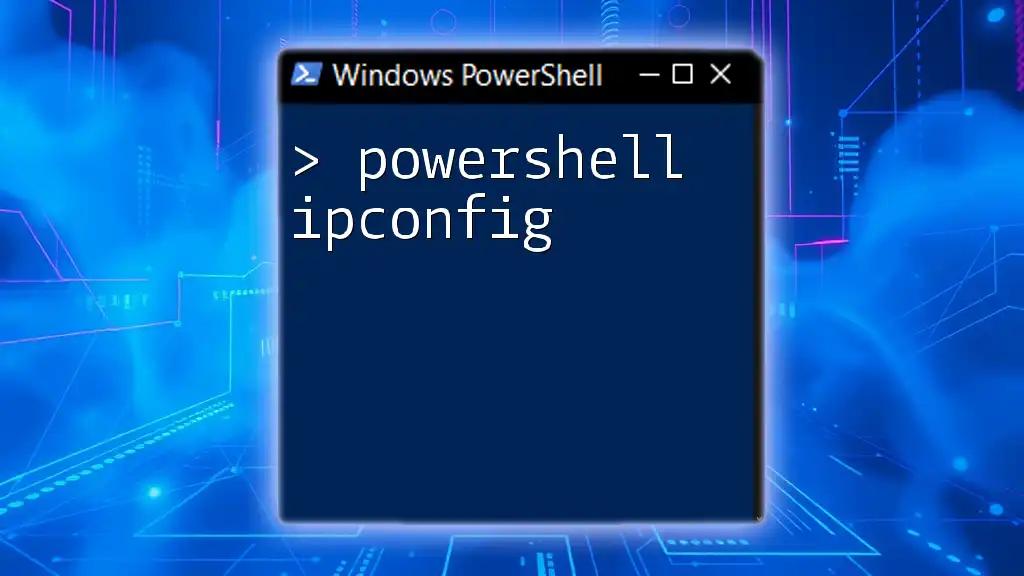
Best Practices for Using `if -contains`
Writing Clear and Concise Scripts
When writing scripts, prioritizing readability and maintainability is crucial. Use meaningful variable names and place comments throughout your code to clarify intentions and logic.
Performance Considerations
While using `-contains` is generally efficient, be cautious with very large datasets. If performance becomes an issue, consider using other data structures or filtering techniques better suited for larger collections.
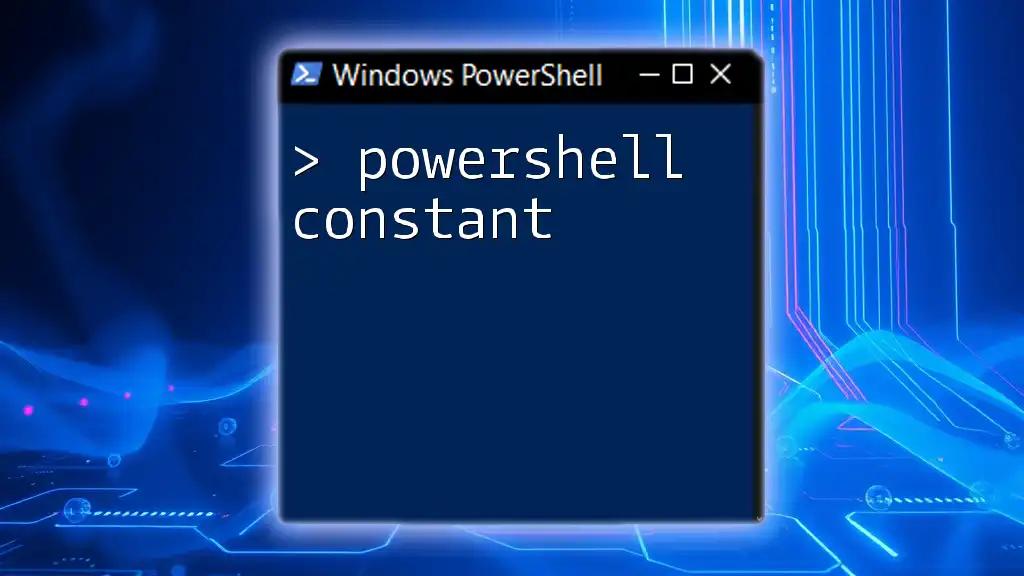
Conclusion
The PowerShell `if -contains` operator is a powerful tool for scripts that require checks on the presence of elements in collections. By mastering its use, along with the `if` statement, you can write more dynamic and effective scripts. As you practice implementing these techniques, you’ll find new and creative ways to enhance your PowerShell scripting abilities.
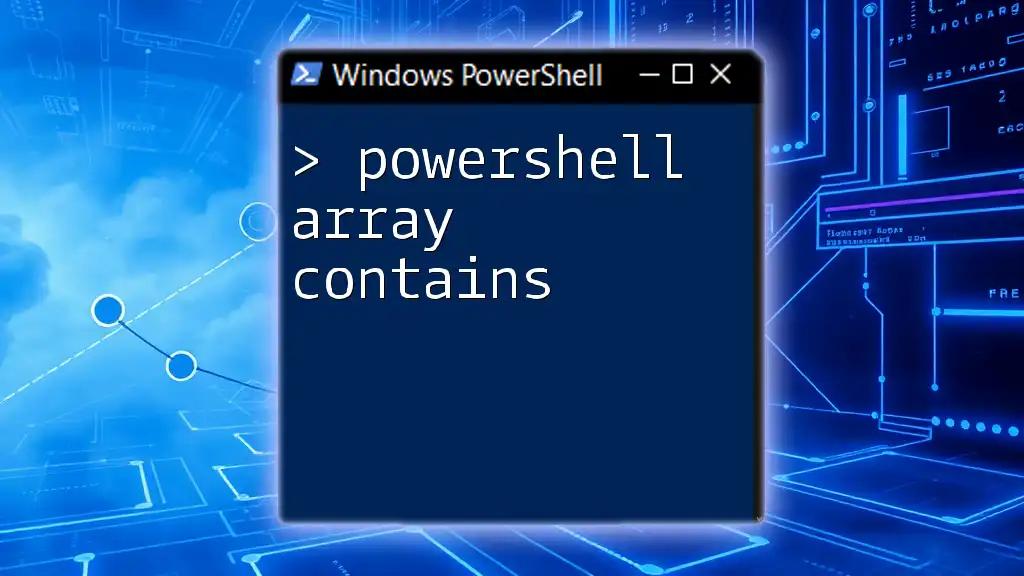
Additional Resources
For further learning, refer to PowerShell documentation, explore recommended books, and engage with community forums. These resources can greatly enhance your understanding and command of PowerShell.
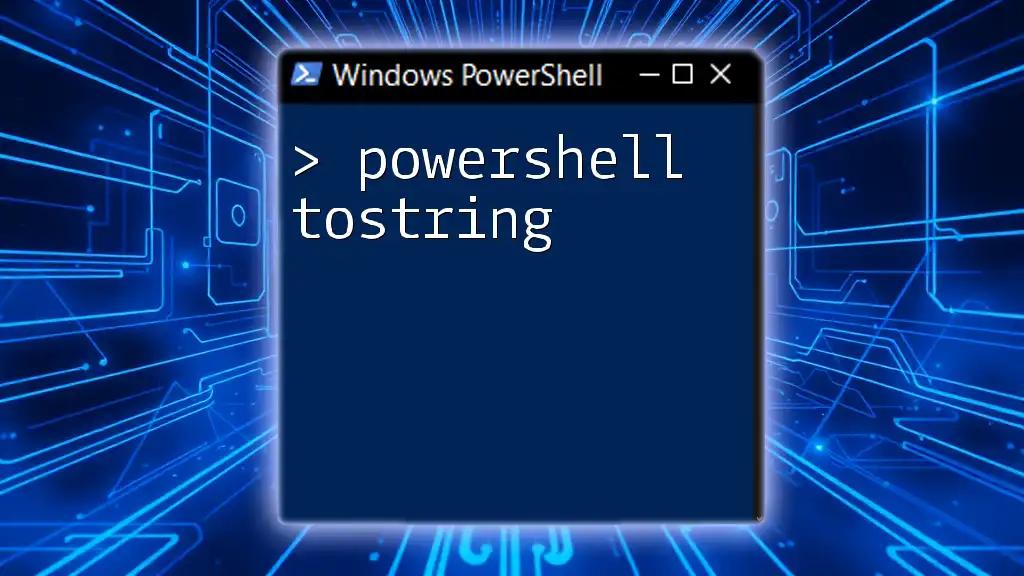
Call to Action
Share your experiences with the `if -contains` operator or ask any questions you may have. If you're interested in learning more about PowerShell commands, consider checking out our services designed to help you master PowerShell quickly and effectively.