In PowerShell, you can perform a case-insensitive check to see if a string contains a specified substring using the `-like` operator with wildcards or the `-match` operator when using regular expressions.
Here's a simple example using the `-like` operator:
$myString = "Hello, World!"
if ($myString -like "*hello*") {
Write-Host "Substring found (case-insensitive)!"
} else {
Write-Host "Substring not found."
}
And here's an example using the `-match` operator:
$myString = "Hello, World!"
if ($myString -match "hello") {
Write-Host "Substring found (case-insensitive)!"
} else {
Write-Host "Substring not found."
}
Understanding Case Insensitivity in PowerShell
What is Case Insensitivity?
Case insensitivity refers to the characteristic of a programming or scripting language where the distinction between uppercase and lowercase letters is ignored. In practical terms, this means that `PowerShell`, `powershell`, and `POWERSHELL` are treated as identical. Understanding this property is crucial for effective script writing in PowerShell, enhancing user experience by reducing potential errors related to case mismatches.
Benefits of Using Case Insensitive Commands
Using case insensitive commands presents several significant benefits:
-
Ease of use: Beginner scripters often face challenges due to case sensitivity in other languages. PowerShell’s case insensitivity allows users to compose commands without concern for correct casing, which can dramatically reduce mistakes and learning barriers.
-
Flexibility: Users can input commands in whatever case they prefer. This flexibility supports quick typing and individualized coding styles, creating an inclusive environment for diverse skill sets and preferences.
-
Consistency: Working with a case-insensitive language ensures that commands remain uniform across different platforms, which is particularly beneficial for those who work in multi-environment or cross-platform settings.
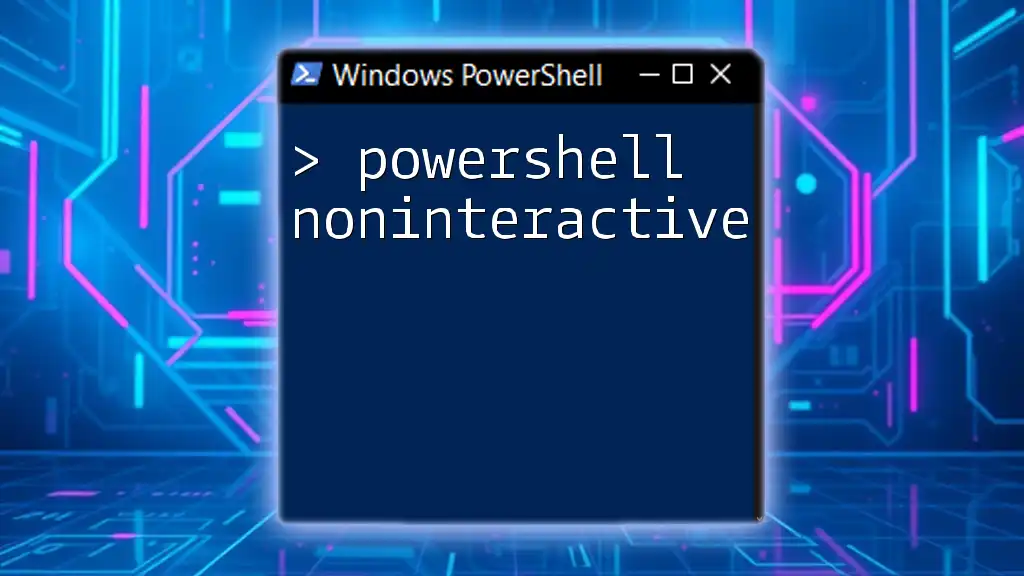
Core PowerShell Commands Related to Case Insensitivity
Commonly Used Cmdlets
PowerShell is rich with cmdlets that exemplify case insensitivity. Regardless of how you type the command, the output remains consistent. For instance:
Get-Process
get-process
GET-PROCESS
All of the above commands will generate a list of currently running processes. This feature not only reinforces user confidence in PowerShell but also aids in maintaining productivity during script development.
Working with Variables
Variable Naming Conventions
PowerShell allows the use of variables irrespective of letter casing, which is pivotal for new users learning scripting basics. For example:
$MyVariable = "Hello"
Write-Output $myvariable
In this case, both `$MyVariable` and `$myvariable` refer to the same entity, containing the string "Hello". This case insensitivity can simplify code since variable names become less rigid.
Using Variables in Scripts
Let's consider a practical example in which variables play a fundamental role.
$Username = "Alice"
Write-Host "Current user is: $username"
Here, whether we reference `$Username` or `$username`, the output will be consistent: "Current user is: Alice". This allows for easier readability and flexibility in writing scripts.
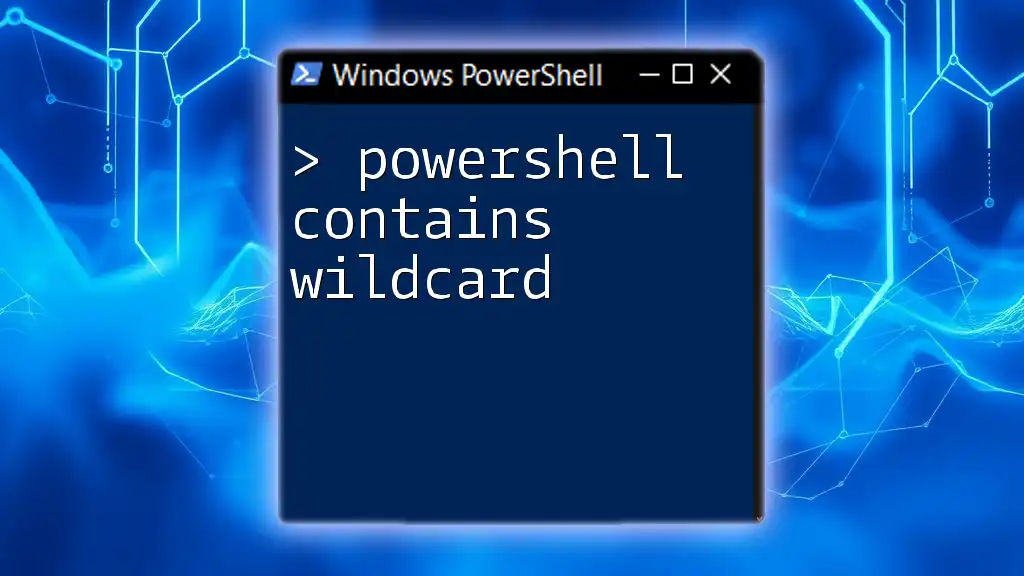
Case Insensitivity in String Comparisons
String Comparison Operators
PowerShell provides various operators for string comparison, and they operate in a case-insensitive manner by default. For instance:
'hello' -eq 'HELLO' # Returns True
This equality operator confirms that regardless of the casing, both strings are considered equal. This characteristic avoids pitfalls in conditions where input might vary in case.
Utilizing the `-like` and `-match` Operators
The `-like` and `-match` operators are notorious for their flexibility in handling string patterns. Take a look at this example:
'PowerShell' -like '*powershell*' # Returns True
Here, the `-like` operator shows its versatility, as it successfully and seamlessly matches different cases. Similarly, when using regular expressions:
'PowerShell is fun' -match 'powershell' # Returns True
These comparisons illustrate the simplicity and efficiency with which PowerShell facilitates string operations.
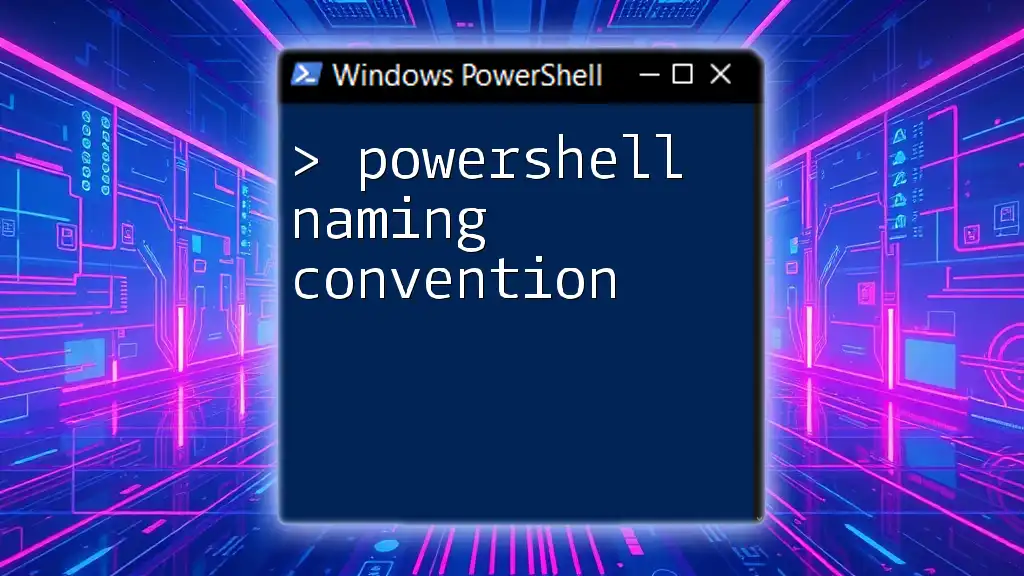
Filtering and Searching
Case Insensitive Filters
In PowerShell, filtering commands also follow case insensitivity. For instance, if you want to retrieve services based on part of their display name, Cap case isn’t a concern:
Get-Service | Where-Object {$_.DisplayName -like "*wIn*"}
This command will return services that contain "Win" in any letter combination, demonstrating how filtering works effectively without needing to worry about the case.
Using Regular Expressions
Regular expressions (regex) can be powerful tools for searching through string data. PowerShell handles regular expressions in a case-insensitive way by default:
'PowerShell is fun' -match 'powershell' # Returns True
In this example, the regex engine allows for elaborate search patterns without case affecting the outcome, offering further flexibility for users looking for specific data.
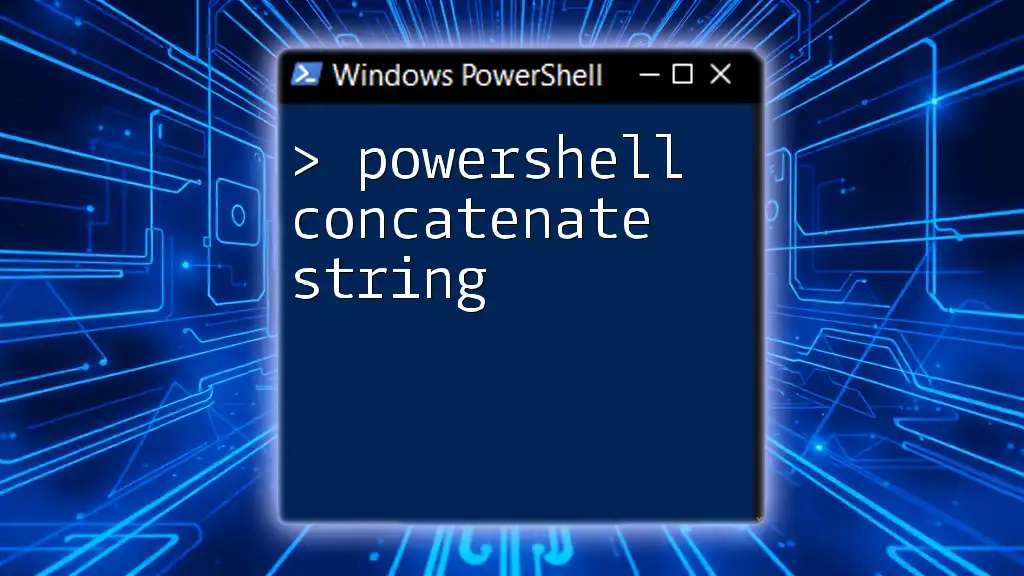
Best Practices for Case Insensitivity in PowerShell
Consistency in Script Writing
While PowerShell’s leniency with case can enhance usability, maintaining consistency in scripting is still an important practice. Being mindful of casing in command and variable names throughout your scripts can support readability and help other users (or future you) understand the code without confusion.
Reading and Commenting on Code
Given that PowerShell contains case insensitive features, having appropriate comments is essential. A consistent commenting style can alleviate confusion caused by varied case usage. For example:
# Using lower case to reference the variable, which holds the username
Write-Host "User: $username"
Adding comments can provide clarity and context for other users, especially in collaborative environments.
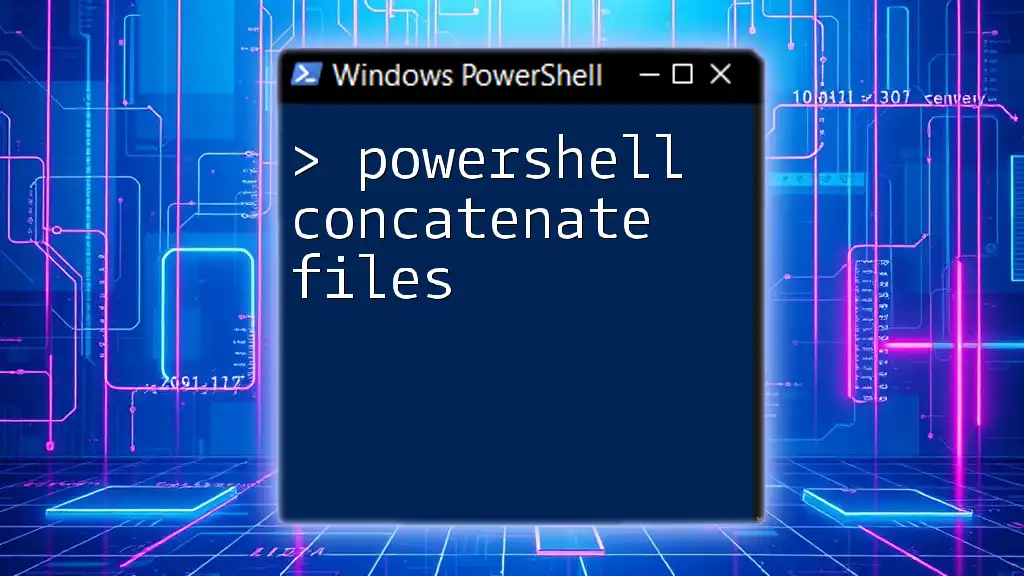
Conclusion
In summary, understanding that PowerShell contains case insensitive properties can significantly enhance user experience and improve coding efficiency. By grasping the nuances of case insensitivity, users can leverage the strengths of PowerShell confidently, making scripting less error-prone and more productive. Continuous practice and exploration of PowerShell's capabilities further empower developers to write efficient and readable scripts.
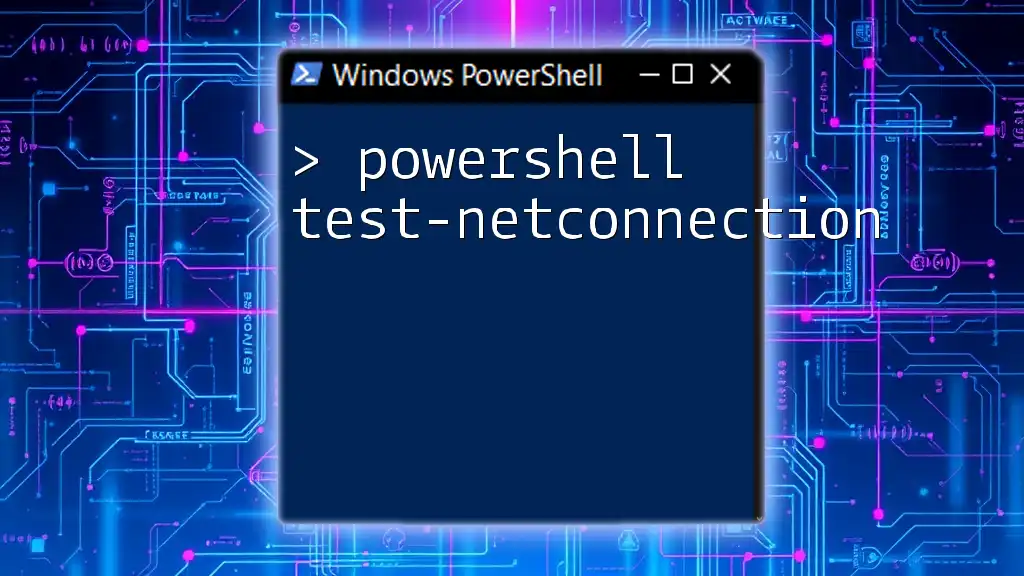
Additional Resources
To deepen your knowledge of PowerShell's case insensitivity and capabilities, consider visiting the official Microsoft documentation and exploring recommended books and online courses tailored for PowerShell learners. Engaging with communities can also provide valuable insights and support as you navigate your PowerShell journey.