In PowerShell, converting text files to sentence case means modifying the text so that only the first letter of each sentence is capitalized while the rest are in lowercase; here's a code snippet to demonstrate this process.
(Get-Content 'input.txt') -replace '(^\s*|\.\s+)([a-z])', { $matches[0] + $matches[2].ToUpper() } | Set-Content 'output.txt'
Understanding Sentence Case
Definition of Sentence Case
Sentence case is a text formatting style that involves capitalizing only the first letter of each sentence while leaving the rest of the text in lowercase. This contrasts with other text styles, such as Title Case, where the first letter of every major word is capitalized.
For example, in sentence case, "this is an example." appears as it is, whereas in Title Case, it would be "This Is An Example." Sentence case is particularly useful for improving the readability of documents and creating a more professional appearance in text outputs.
Use Cases for Sentence Case
Recognizing when to use sentence case can significantly enhance the clarity of your texts. Here are a few notable scenarios:
- Documentation: When writing user manuals or technical documentation, employing sentence case makes instructions clearer.
- Coding Comments: Comments written in sentence case can make it easier for others (or yourself in the future) to understand the logic behind code.
- Email Templates: Creating templates for professional communication in sentence case ensures a formal and respectful tone.
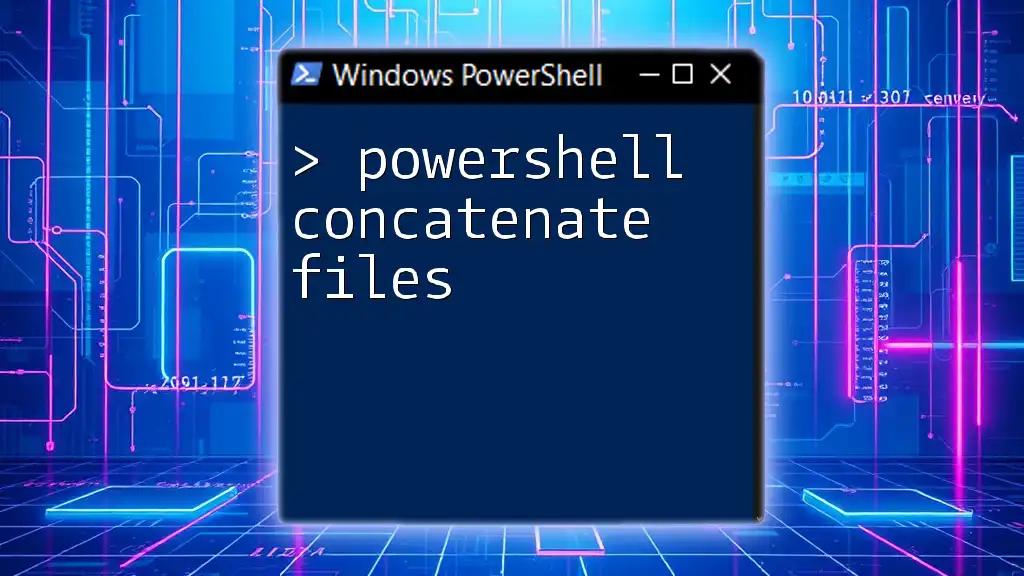
Working with Files in PowerShell
Overview of File Manipulation
PowerShell provides a robust environment for file manipulation. Handling files usually involves four primary tasks: reading file contents, modifying them, renaming files, and managing file metadata. Understanding these tasks is crucial for effective scripting.
Accessing Files with PowerShell
Getting Started with File Paths
File paths can either be absolute or relative. Absolute paths indicate the full directory from the root level, while relative paths reference locations based on the current directory. Properly navigating file paths is essential for accurately accessing files in PowerShell.
For instance, to list all the files in a directory, you can use the `Get-ChildItem` cmdlet:
Get-ChildItem "C:\ExampleFolder"
Opening Files
Once you’ve identified your files, you can read their contents using `Get-Content`. This cmdlet allows you to quickly retrieve text from files:
Get-Content "C:\ExampleFolder\example.txt"
This command returns the entire content of `example.txt`, enabling you to review the text before making any changes.

Transforming Text to Sentence Case
The Power of Regular Expressions
What are Regular Expressions?
Regular Expressions (regex) are powerful text processing tools that enable you to search, match, and manipulate strings. They can identify patterns in text, making them particularly useful for formatting tasks.
Crafting a Sentence Case Function
To efficiently convert text to sentence case, you can create a PowerShell function. This function takes an input string and modifies it to follow sentence case rules:
function ConvertTo-SentenceCase {
param (
[string]$inputString
)
return -join ((Get-Content $inputString) -replace '(?<=[.!?])\s*(\w)', { $_.Value.ToUpper() }) -replace '^\w', { $_.Value.ToUpper() })
}
Explanation:
- The function reads the specified file, replaces all instances of the first character after sentence-ending punctuation (like '.', '?', or '!') with an uppercase letter, and ensures the very first letter of the text is capitalized as well.
Applying Sentence Case to File Contents
Searching and Replacing Text
After implementing your conversion function, you'll want to use it to update file contents automatically. You can perform a search and replace operation with the following code snippet:
$content = Get-Content "C:\ExampleFolder\example.txt"
$content = $content -replace 'text to be replaced', 'new text'
Set-Content -Path "C:\ExampleFolder\example.txt" -Value $content
This command retrieves content from `example.txt`, replaces specified text, and saves the changes back to the file.
Saving Changes Back to File
Ensure you save the modified content back using `Set-Content`. Before overwriting files, it’s always wise to create a backup to prevent losing original data.

Practical Applications of Sentence Case in File Management
Automating Text Formatting in Batch Files
PowerShell’s capabilities allow you to automate formatting across multiple files. The following script can apply sentence case conversion to all `.txt` files in a directory:
Get-ChildItem "*.txt" | ForEach-Object {
$content = Get-Content $_.FullName
$newContent = ConvertTo-SentenceCase $content
Set-Content -Path $_.FullName -Value $newContent
}
This loop goes through all text files, converts their content to sentence case, and saves the updated content back to each file.
Structuring Output for Reports and Documentation
When generating reports or documentation, presenting data in sentence case promotes better readability. For instance, a script that creates a summary report can format text to make headings and descriptions clearer, improving your audience's understanding and engagement.

Conclusion
Utilizing PowerShell sentence case files can simplify and enhance your workflow, especially when handling large volumes of text. Whether you’re working on project documentation, automating reports, or just tidying up comments in scripts, the ability to format text efficiently is invaluable.
Additional Resources
To deepen your knowledge and skills in PowerShell:
- Explore the official PowerShell documentation
- Participate in community forums
- Read books dedicated to PowerShell scripting
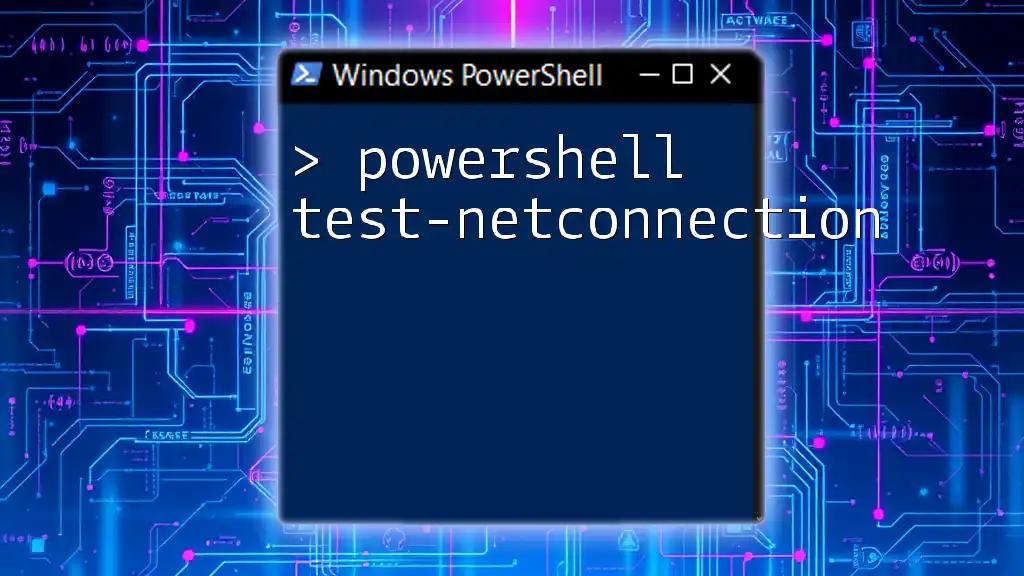
Call to Action
We invite you to share your experiences with sentence case in PowerShell! Feel free to leave comments or questions to engage further with the community. Your insights and inquiries can help others on their journey to mastering PowerShell scripting.