To combine PDF files using PowerShell, you can utilize the `PdfSharp` library for a straightforward solution, as shown in the code snippet below:
Add-Type -AssemblyName PdfSharp; $pdfs = @("C:\path\to\file1.pdf", "C:\path\to\file2.pdf"); $output = "C:\path\to\combined.pdf"; $document = New-Object PdfSharp.Pdf.PdfDocument; $pdfs | ForEach-Object { $tempDoc = [PdfSharp.Pdf.PdfReader]::Open($_, [PdfSharp.Pdf.PdfDocumentOpenMode]::Imported); $document.AddPage($tempDoc.Pages[0]) }; $document.Save($output); $document.Close()
Understanding PDF Files
What is a PDF File?
A Portable Document Format (PDF) file is a widely used format that retains the formatting of a document regardless of the software, hardware, or operating system used to view it. PDFs are crucial for disseminating information in a secure and professional manner, and they are utilized in various contexts, such as contracts, reports, and brochures. Combining PDF files can be essential for creating comprehensive documents or compiling reports from multiple sources.
Challenges in Combining PDF Files
Combining PDF files manually can be tedious and often requires specialized software. Many people rely on third-party applications, which may come with licensing fees or dependencies. This is where PowerShell shines as it allows for automation and scripting to handle tasks without needing extra software, making it a flexible solution for managing PDFs.
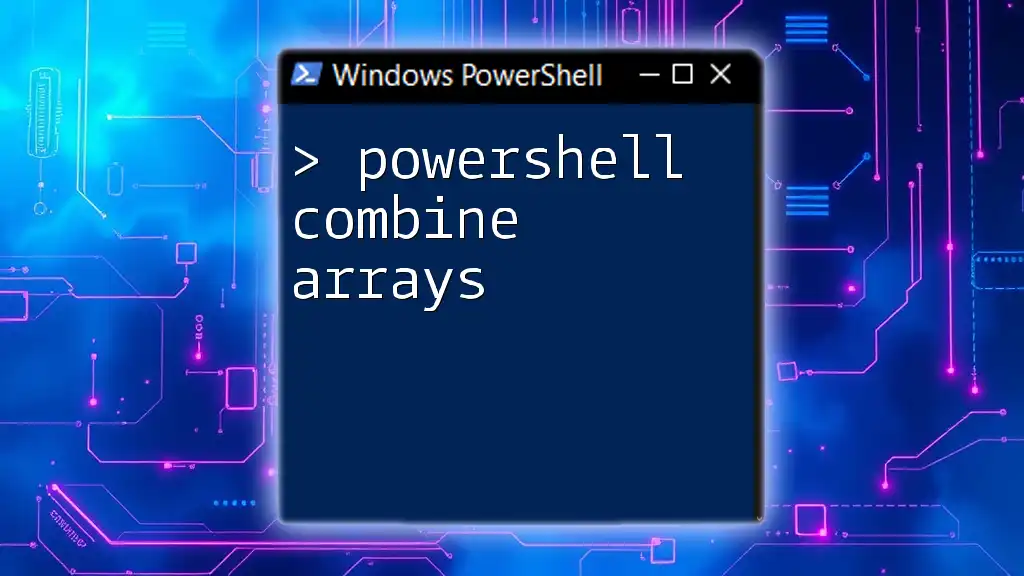
Setting Up Your PowerShell Environment
Installing PowerShell
To start using PowerShell for combining PDF files, ensure you have PowerShell installed on your machine. PowerShell Core is recommended for its improved cross-platform capabilities, while Windows PowerShell is suitable for Windows environments.
Required Libraries for PDF Manipulation
For manipulating PDF files in PowerShell, you will need additional libraries, as PowerShell does not natively support PDF operations. Here are some popular libraries you can use:
- iTextSharp: A powerful library for creating and manipulating PDF files.
- PDFsharp: Allows for the creation and processing of PDF documents.
- PdfSharpCore: A .NET Core version of PDFsharp that you can use with PowerShell Core.
Installing PDF Libraries
Installing iTextSharp
To install iTextSharp via NuGet, use the following command in PowerShell:
Install-Package itext7
This command will download and install the library, making it available for use in your scripts.
Installing PdfSharpCore
If you prefer PdfSharpCore, execute this command:
Install-Package PdfSharpCore
With either of these libraries, you can effectively manipulate PDF files in PowerShell.
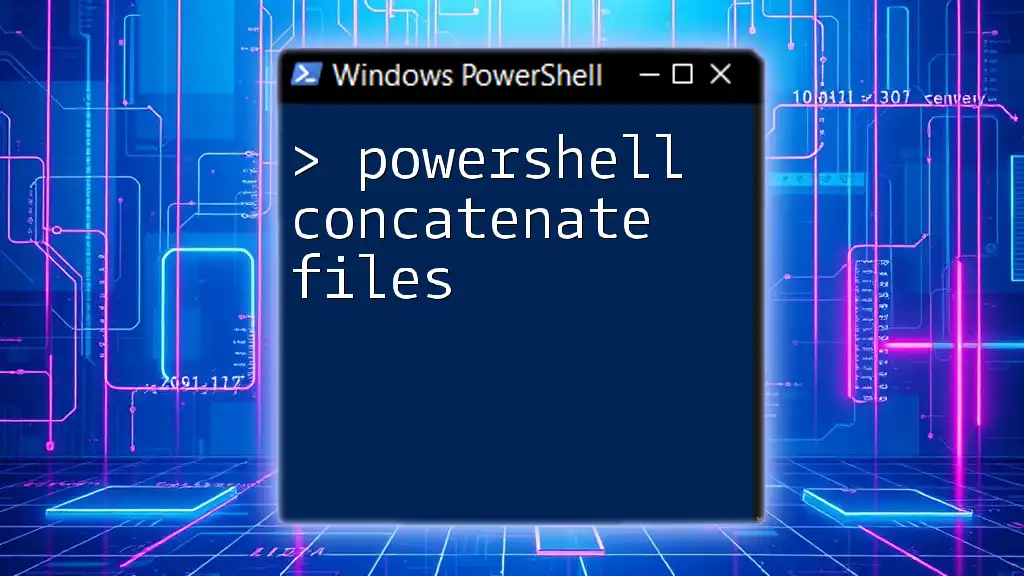
Creating a PowerShell Script to Combine PDF Files
Writing the Script
Now that your environment is set up, let’s write a script to combine PDF files. The following are the essential components that make up the script:
- Add-Type: To include the required DLLs.
- Function Definition: A function that takes in the PDF files you wish to combine and an output path.
- Adding Pages: Logic that iteratively adds pages from each PDF to a new document.
Sample Code Snippet
Here's a sample PowerShell script to help you combine PDF files:
Add-Type -Path "path\to\PdfSharp.dll"
function Combine-PDFs {
param (
[string[]]$inputFiles,
[string]$outputFile
)
$document = New-Object PdfSharp.Pdf.PdfDocument
foreach ($file in $inputFiles) {
$tempDoc = [PdfSharp.Pdf.IO.PdfReader]::Open($file, [PdfSharp.Pdf.IO.PdfDocumentOpenMode]::Import)
foreach ($page in $tempDoc.Pages) {
$document.AddPage($page)
}
}
$document.Save($outputFile)
$document.Close()
}
In this script, the Combine-PDFs function takes an array of input PDF file paths and an output path for the combined PDF. It opens each PDF and adds its pages to a new document, which is finally saved.
Explanation of the Script
- Add-Type: This line is crucial for importing the PDFSharp assembly so you can access its functionalities.
- Combine-PDFs Function: The parameters $inputFiles and $outputFile allow for flexibility in selecting which PDFs to combine and where to save the final document.
- Importing and Merging PDF Pages: The script uses a loop to process each file, reading its pages and appending them to the new document. The use of `[PdfSharp.Pdf.IO.PdfReader]::Open` allows you to open and process existing PDF files.
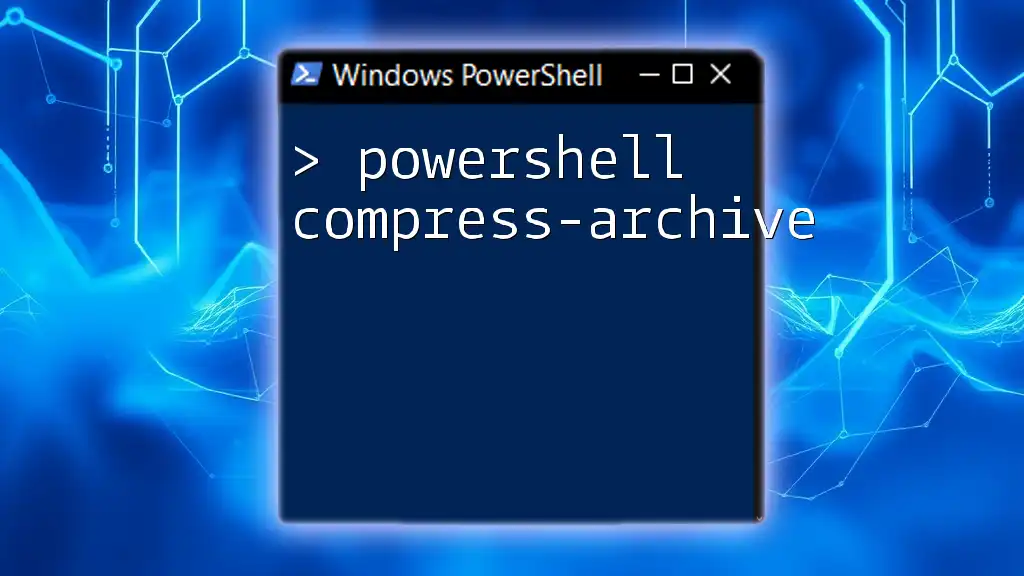
Running the Script
How to Execute the Script
To run the script and combine your PDF files, simply execute the function from the PowerShell console with the following command:
Combine-PDFs -inputFiles "C:\path\to\file1.pdf", "C:\path\to\file2.pdf" -outputFile "C:\output\combined.pdf"
This command will take `file1.pdf` and `file2.pdf` and create a new PDF at the designated output path called `combined.pdf`.
Handling Errors
You may encounter common errors while executing the script, such as file paths not found or issues with file permissions. Implementing basic error handling can save time and frustration. To do this, consider using try-catch blocks within the script:
try {
# Your combine PDF logic here
} catch {
Write-Host "An error occurred: $_"
}
This will print a more user-friendly error message while debugging.
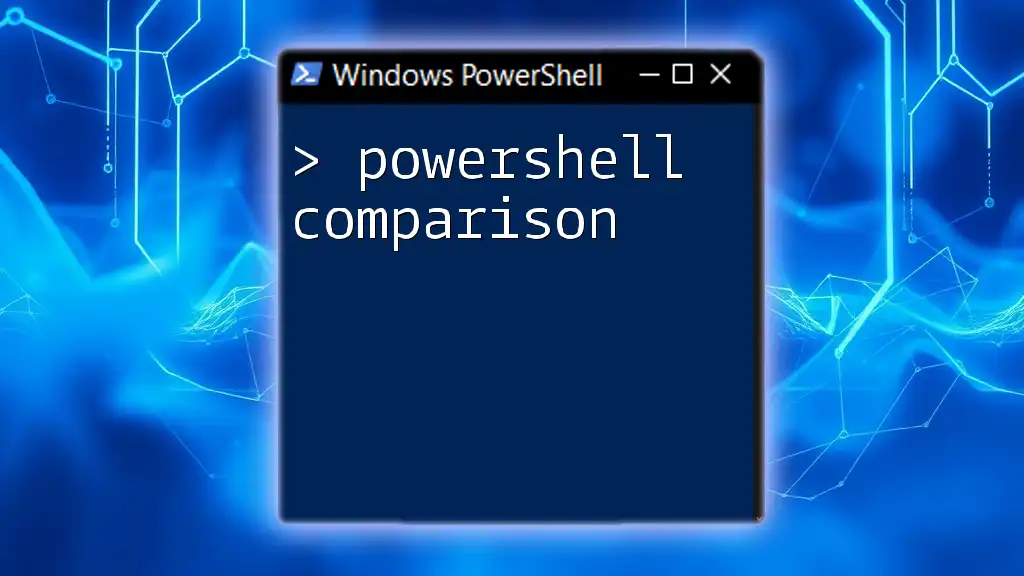
Best Practices for Working with PDF Files in PowerShell
Organizing Input Files
Before running your script, ensure your input files are well organized. Maintain a clear folder structure and consistent naming conventions to avoid confusion and errors during the merging process. This can save significant time and reduce the chances of mistakes.
Automating the Process
You can easily automate the script using Windows Task Scheduler, which can run your PowerShell script on a regular schedule. This is particularly useful if you regularly combine several PDF files, streamlining your workflow significantly.
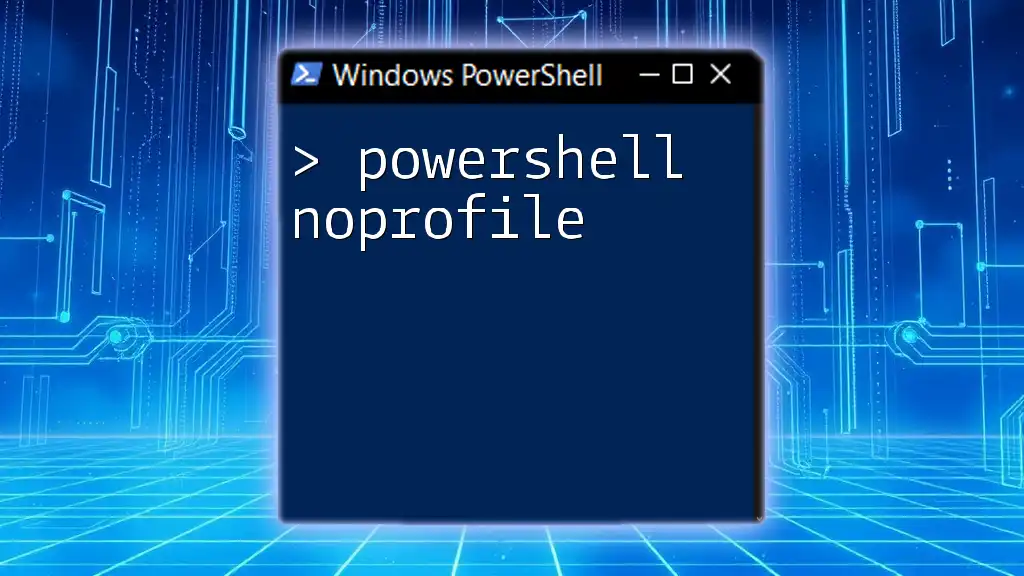
Conclusion
In this guide, we explored how to effectively use PowerShell to combine PDF files. We outlined the setup process, discussed necessary libraries, and provided a detailed script to achieve your goal. PowerShell is a powerful tool that can automate and simplify many tasks, including PDF manipulation.
Now that you have the knowledge, feel free to experiment with variations of the script and integrate more complex functionalities as you become more comfortable with PowerShell. With the growing adoption of PowerShell, you will find yourself equipped to handle a variety of automation tasks seamlessly.
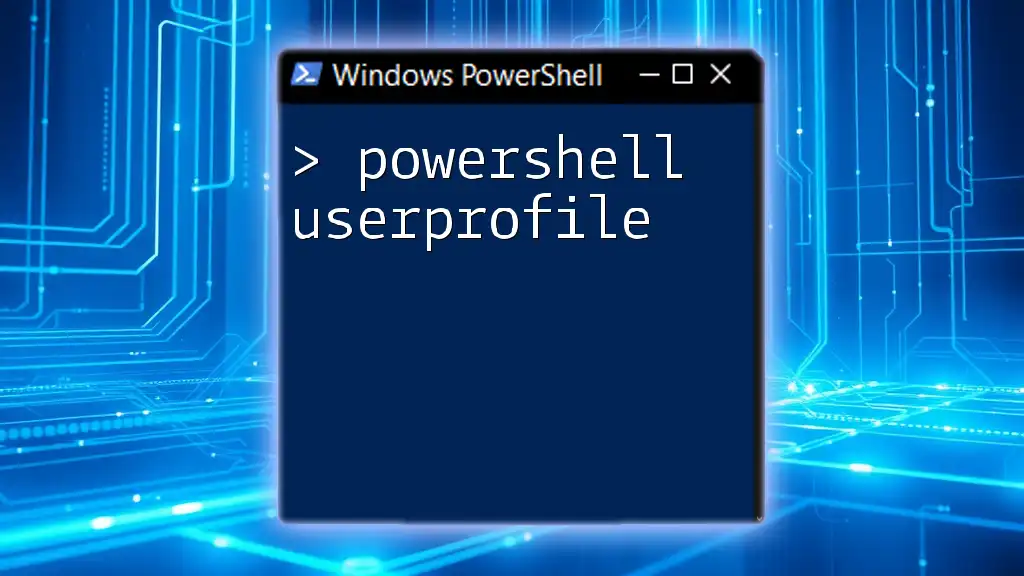
Frequently Asked Questions (FAQ)
Can I combine other file types besides PDFs?
While the method discussed here focuses on PDFs, PowerShell can manipulate other file formats using different libraries. However, combining differing file formats into a single PDF may require conversion before using the same method.
Do I need administrative privileges to use these commands?
Generally, administrative privileges are not required to run the script unless you're accessing restricted directories or modifying system files.
Where can I find additional resources for learning PowerShell?
There are numerous resources available, including the [official Microsoft PowerShell documentation](https://docs.microsoft.com/powershell/) and community forums where you can find tips, scripts, and problem-solving advice.
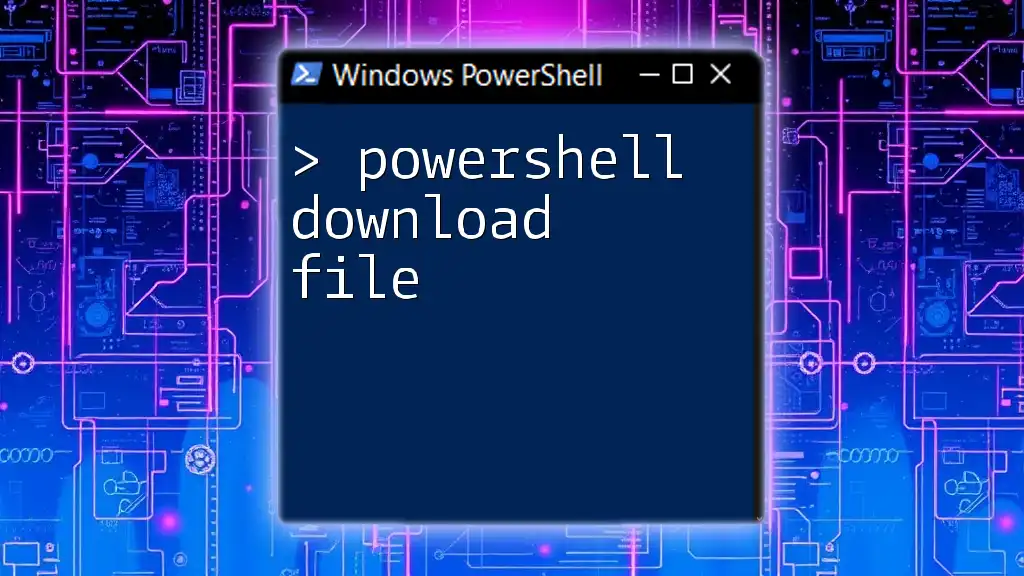
Additional Resources
To continue your journey into PowerShell, explore the libraries discussed earlier, look for online tutorials, and join PowerShell communities for deeper insights and shared knowledge.