To concatenate multiple files in PowerShell, you can use the `Get-Content` cmdlet to read the files and the `Set-Content` cmdlet to write them into a single output file.
Get-Content file1.txt, file2.txt | Set-Content combined.txt
Preparing for File Concatenation
Choosing Your Files
Before diving into the powerful world of file concatenation with PowerShell, it’s essential to identify the specific files you need to work with. Depending on your requirements, you may choose individual files or entire directories containing multiple files.
Using wildcards can facilitate the selection process, allowing you to concatenate groups of files more efficiently. For instance, if you want to concatenate all text files in a directory, you can use the wildcard `*.txt`.
Organizing your files ahead of time can save you a lot of hassle. Ensure your files are in a well-structured directory to make selection easier.
Setting Up Your PowerShell Environment
To get started with concatenating files, launch PowerShell. You can easily open PowerShell via the Start menu or utilize Windows Terminal or Visual Studio Code. Having your environment set up correctly is crucial for a smooth concatenation process.

Methods to Concatenate Files in PowerShell
Using the `Get-Content` and `Set-Content` Cmdlets
One of the simplest yet effective methods to concatenate files in PowerShell is through the combination of `Get-Content` and `Set-Content`.
The basic syntax for concatenating text files is as follows:
Get-Content -Path 'C:\path\to\source\*.txt' | Set-Content -Path 'C:\path\to\destination\output.txt'
In this command:
- `Get-Content` retrieves the content of all `.txt` files in the specified source path.
- The pipe (`|`) directs the output to `Set-Content`, which writes it into `output.txt` in your destination folder.
This simple approach is suitable for various scenarios, such as merging multiple log files into a single one.
Utilizing the `Add-Content` Cmdlet
The `Add-Content` cmdlet is another powerful tool for concatenating files. It is particularly useful when you want to append content to an existing file, rather than replacing it.
Here’s how you can do this:
Get-Content -Path 'C:\path\to\source\*.csv' | Add-Content -Path 'C:\path\to\destination\combined.csv'
In this instance, `Get-Content` fetches the contents of all CSV files and `Add-Content` appends them to `combined.csv`. Use this method when you want to maintain existing content in your output file while adding more data.
PowerShell Concatenation with `Out-File`
Another effective cmdlet for concatenation is `Out-File`. This cmdlet can handle larger files well and allows you to specify encoding.
For example:
Get-Content -Path 'C:\path\to\source\*.txt' | Out-File -Path 'C:\path\to\destination\merged.txt' -Encoding UTF8
Using `Out-File` gives you control over the file encoding, which is essential when dealing with files that may have different character sets.
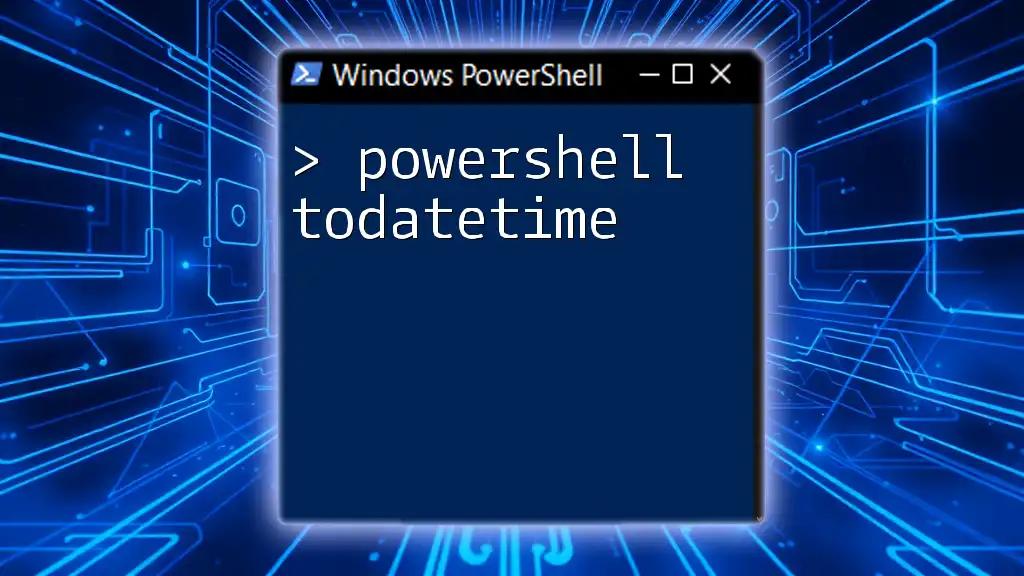
Advanced Concatenation Techniques
Concatenating Specific File Types
PowerShell allows you to use filter parameters to concatenate specific file types. If you only want to include `.txt` files, you can specify that in your command.
For example:
Get-Content -Path 'C:\path\to\source\*.txt' | Set-Content -Path 'C:\path\to\destination\output.txt'
In this code snippet, only text files are processed, ensuring that your output file contains only the intended data.
Handling Large Files
When concatenating large files, it's vital to optimize performance to prevent memory issues. You can do this by using `-ReadCount` with `Get-Content`, which controls how many lines are read at once.
Example:
Get-Content -Path 'C:\path\to\largefile.log' -ReadCount 1000 | Set-Content -Path 'C:\path\to\destination\output.log'
This approach reads the file in chunks of 1000 lines, minimizing memory overhead while processing large logs.
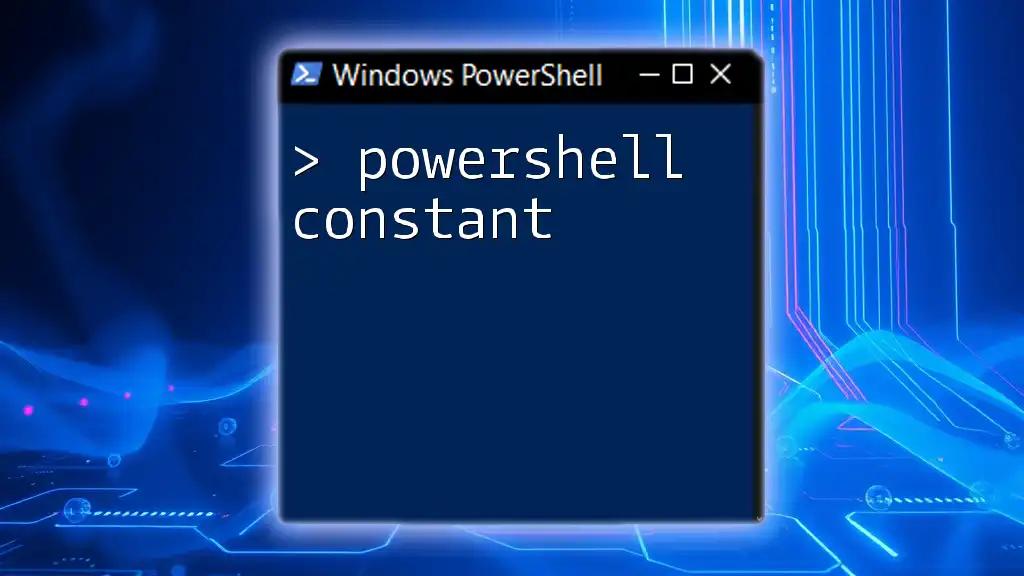
Troubleshooting Common Issues
Error Handling During Concatenation
While working with files, you may encounter errors such as permission issues or the files being locked by another process. It’s essential to handle these scenarios gracefully. You can use `Try-Catch` blocks in PowerShell to manage errors effectively.
Example:
Try {
Get-Content -Path 'C:\path\to\source\*.txt' | Set-Content -Path 'C:\path\to\destination\output.txt'
}
Catch {
Write-Host "An error occurred: $_"
}
Verifying File Integrity
After concatenation, checking the integrity of the merged file is crucial. You can use hash functions to verify that the content matches your expectations.
Example:
Get-FileHash -Path 'C:\path\to\destination\output.txt' -Algorithm SHA256
This command generates a hash for the output file, giving you a way to verify the file’s integrity against your source files.
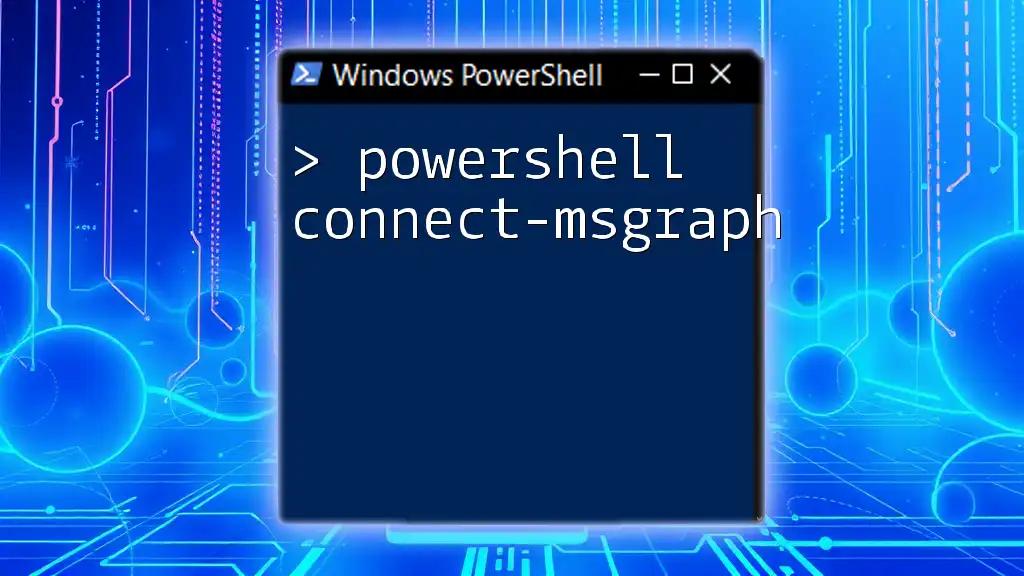
Additional Tips and Best Practices
Keeping Your Scripts Organized
Organizing your PowerShell scripts with comments and clear formatting is essential for readability and maintenance.
Consider creating reusable functions for commonly used commands. Here’s an example of how to define a function for concatenation:
Function Concatenate-Files {
param (
[string]$sourcePath,
[string]$destinationPath
)
Get-Content -Path "$sourcePath\*" | Set-Content -Path $destinationPath
}
Optimizing for Future Use
To streamline repetitive tasks, consider scheduling your concatenation tasks using Task Scheduler in Windows. This feature allows you to automate file concatenation during off-peak hours, ensuring resource optimization.
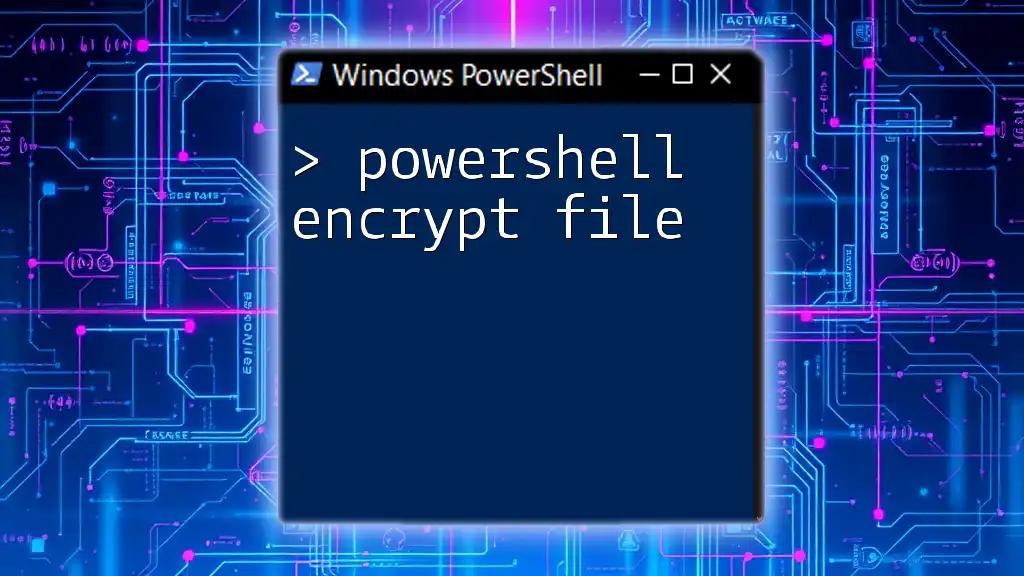
Conclusion
Mastering how to concatenate files in PowerShell opens up a realm of possibilities for managing and organizing your data. By leveraging cmdlets like `Get-Content`, `Set-Content`, `Add-Content`, and `Out-File`, you can perform efficient file operations, saving time and effort.
Don’t hesitate to experiment with your own files to see how PowerShell can transform your file management processes. For further learning, explore online resources and communities that focus on PowerShell, keeping your skills up to date.
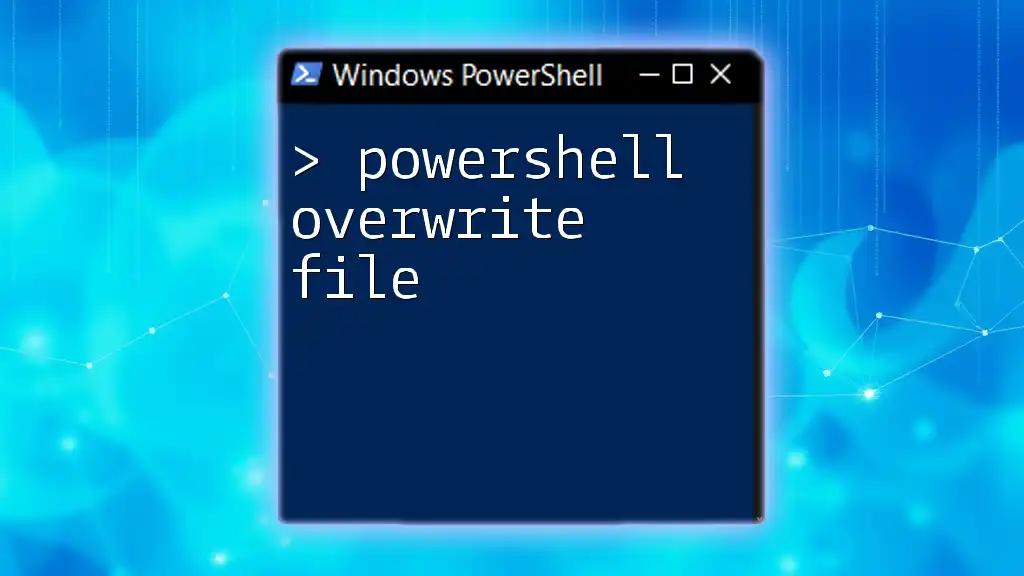
FAQs
How do I concatenate files in PowerShell?
You can concatenate files using the `Get-Content` cmdlet in combination with `Set-Content` or `Add-Content` to either overwrite or append file content respectively.
Can I concatenate files of different types?
Yes, PowerShell can concatenate files of different types, but be mindful of the format and compatibility of the data being merged.
What if my files are too large?
For large files, utilize the `-ReadCount` parameter when using `Get-Content` to manage memory usage effectively and improve performance during the concatenation process.