To change file permissions in PowerShell, you can use the `Set-Acl` cmdlet along with the `Get-Acl` cmdlet to modify access control settings for a specified file or folder.
Here's a code snippet for changing file permissions:
$acl = Get-Acl "C:\Path\To\Your\File.txt"
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule("Username","ReadAndExecute","Allow")
$acl.SetAccessRule($rule)
Set-Acl "C:\Path\To\Your\File.txt" $acl
Understanding Windows File Permissions
File permissions are a critical aspect of Windows security, allowing administrators and users to control access to files and folders. By managing these permissions effectively, you can ensure that only authorized users can read, write, or modify sensitive information.
Common permission types include:
- Read: Users can view the contents of the file or folder.
- Write: Users can modify the contents of the file or folder.
- Modify: Users can read and write, as well as delete the file or folder.
- Full Control: Users have complete control over the file or folder, including the ability to change permissions.
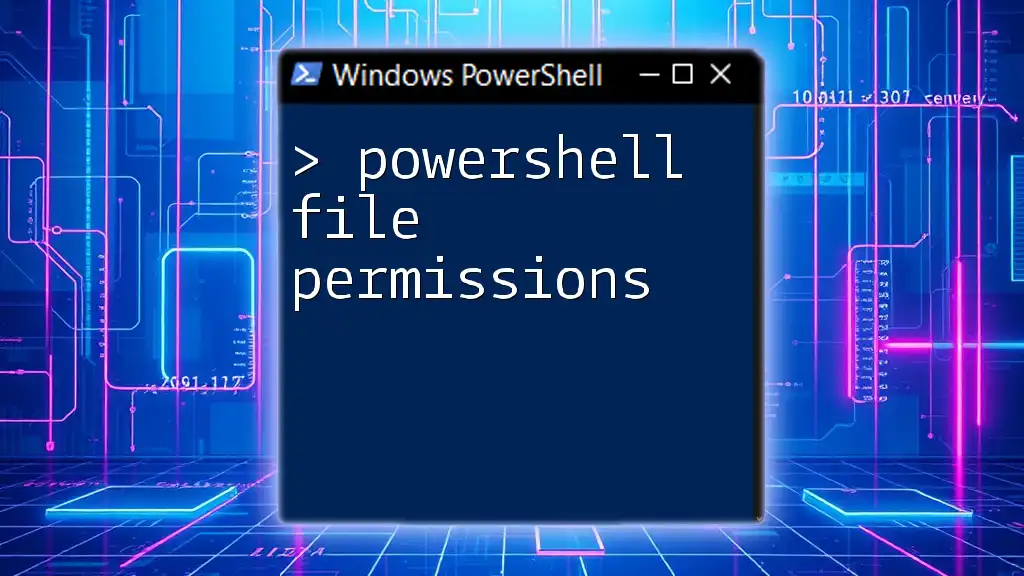
PowerShell Basics for File Permission Management
PowerShell provides powerful cmdlets that enable users to manage file permissions efficiently. The two main cmdlets used in this context are Get-Acl and Set-Acl.
- Get-Acl: Retrieves the access control list (ACL) of a specified file or folder, showing you the current permissions.
- Set-Acl: Modifies the ACL of a file or folder, allowing you to change the permissions as needed.
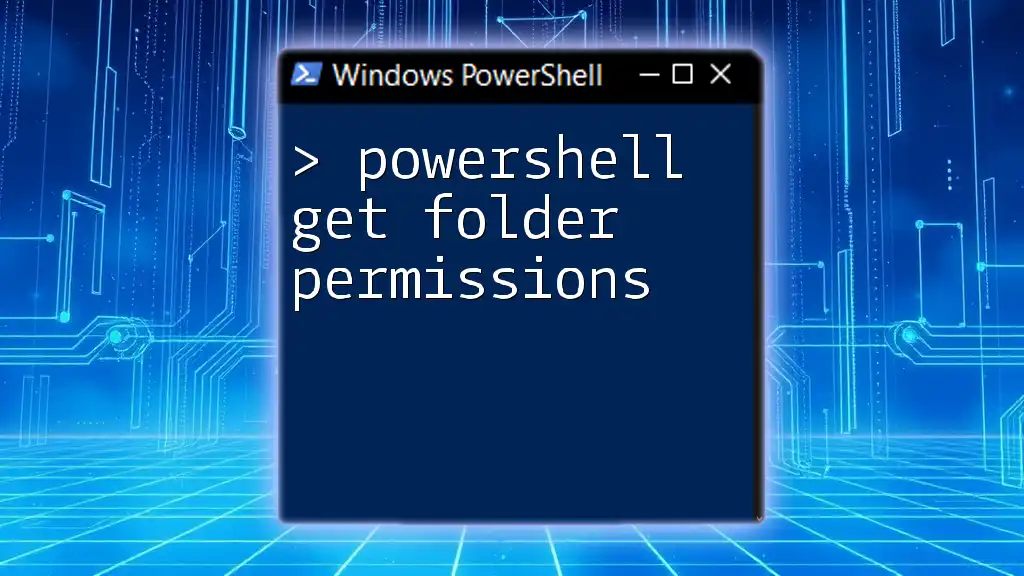
How to Change File Permissions with PowerShell
Using the Get-Acl Cmdlet
To change file permissions using PowerShell, you first need to understand what the current permissions are. This is where the Get-Acl cmdlet comes into play.
For instance, to retrieve the permissions for a specific file, you can use the following command:
Get-Acl "C:\Path\To\File.txt"
This command will display the current access rights, helping you determine what changes need to be made.
Using the Set-Acl Cmdlet
Once you know the current permissions, you can change them using the Set-Acl cmdlet. Here’s a step-by-step process to modify file permissions:
-
Retrieve the current access control list: You first retrieve the ACL for the file:
$acl = Get-Acl "C:\Path\To\File.txt"
-
Define the new permission rule: You create a new access rule. For example, to grant a user named "Username" full control, you would set it up like this:
$permission = "DOMAIN\Username","FullControl","Allow" $accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule $permission
-
Apply the new access rule to the ACL: You add the access rule to the existing ACL:
$acl.SetAccessRule($accessRule)
-
Update the file’s ACL: Finally, write the modified ACL back to the file:
Set-Acl "C:\Path\To\File.txt" $acl
By following these steps, you will successfully change file permissions using PowerShell.
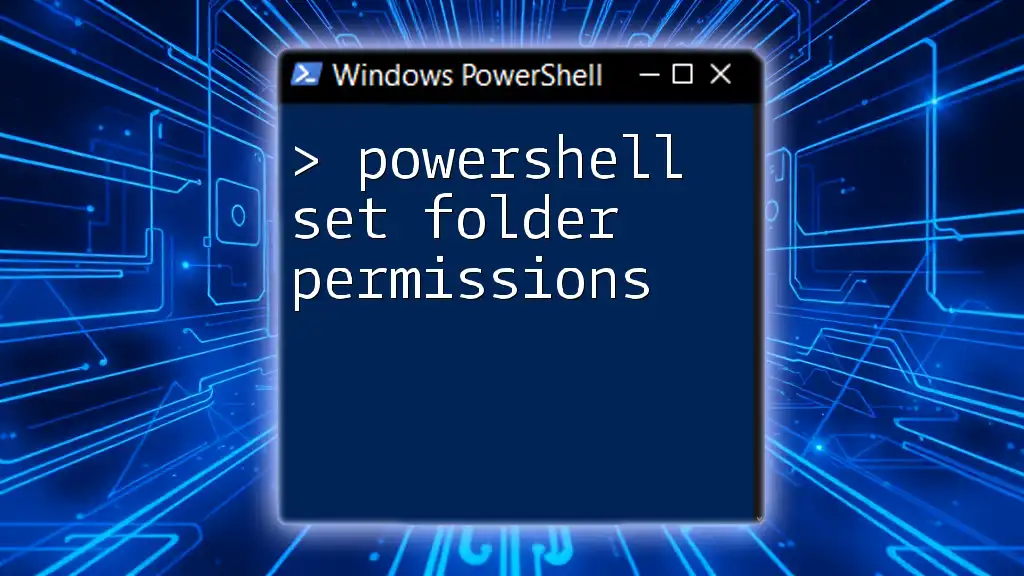
Changing Folder Permissions with PowerShell
PowerShell Set Permissions on Folder
Changing permissions on folders follows a similar process to modifying file permissions. The main difference is that you can apply permissions at a folder level, affecting all files and subfolders within it.
To set permissions on a folder, follow these steps:
-
Get the current ACL: Retrieve the ACL for the folder:
$acl = Get-Acl "C:\Path\To\Folder"
-
Define the new permission rule: For example, to grant a user named "Username" modify permissions on the folder, use:
$permission = "DOMAIN\Username","Modify","Allow" $accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule $permission
-
Apply the access rule to the folder’s ACL: Add the new rule:
$acl.SetAccessRule($accessRule)
-
Update the folder's ACL: Apply the changes:
Set-Acl "C:\Path\To\Folder" $acl
Modify Folder Permissions PowerShell
It's important to note that changing permissions on a parent folder can also affect its contents. If you want to apply the same permission adjustments recursively to all subfolders and files, you can use a loop. Here’s how:
Get-ChildItem "C:\Path\To\Folder" -Recurse | ForEach-Object {
$acl = Get-Acl $_.FullName
$acl.SetAccessRule($accessRule)
Set-Acl $_.FullName $acl
}
This command iterates through each item in the specified folder and applies the new access rights.
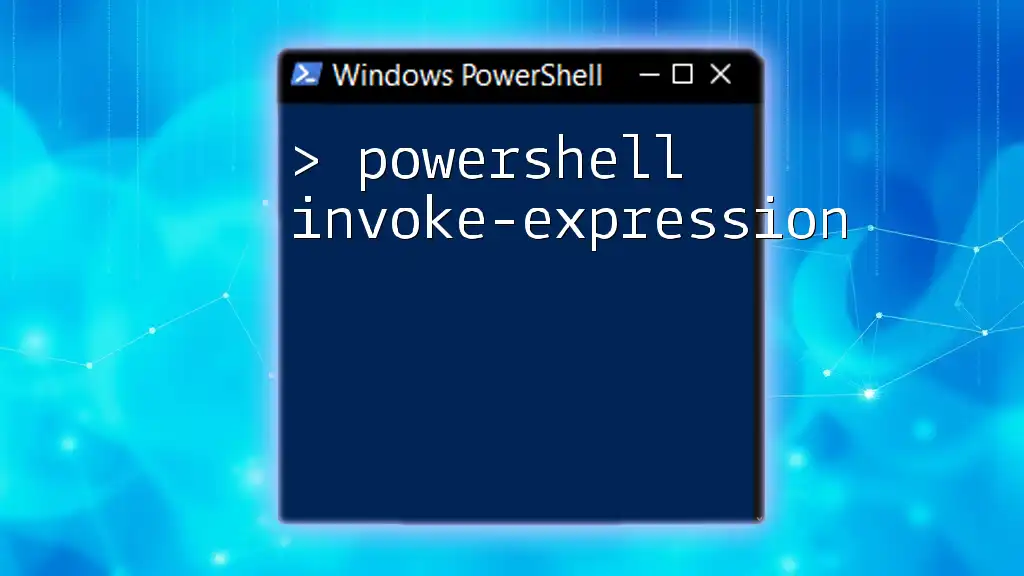
Advanced Techniques for Changing Permissions
Using PowerShell to Change Permissions on Multiple Files
If you need to change permissions for multiple files without iterating individually through each file, you can leverage the Get-ChildItem cmdlet with filters. For example, if you want to change permissions for all `.txt` files:
Get-ChildItem "C:\Path\To\Folder" -Filter *.txt | ForEach-Object {
$acl = Get-Acl $_.FullName
$acl.SetAccessRule($accessRule)
Set-Acl $_.FullName $acl
}
This method simplifies the permission adjustment process for multiple files.
Auditing File Permissions
It's crucial to monitor and verify permissions before and after changes. This helps avoid unexpected access issues. You can quickly audit permissions with the following command:
Get-Acl "C:\Path\To\File.txt" | Format-List
This command outputs the ACL in a readable format, showing all current permissions applied to the file.
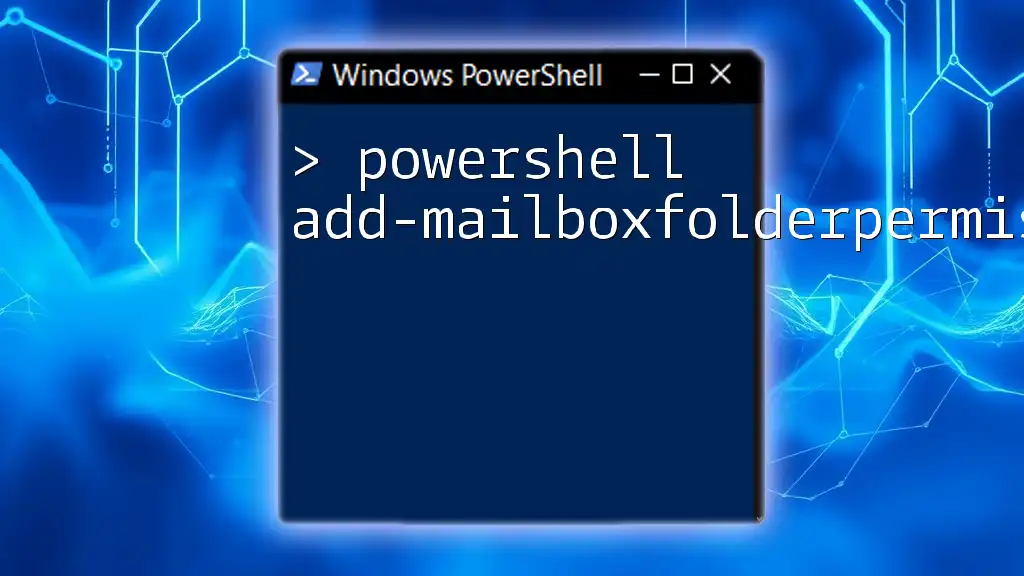
Troubleshooting Common Issues
While using PowerShell to change file permissions is straightforward, you may encounter some common issues.
- Permission Denied: Ensure you have administrative privileges to modify file or folder permissions.
- Errors with Set-Acl: Make sure the ACL object is correctly structured and includes the necessary access rules before applying them.
Take your time to troubleshoot and verify that all commands are executed correctly.
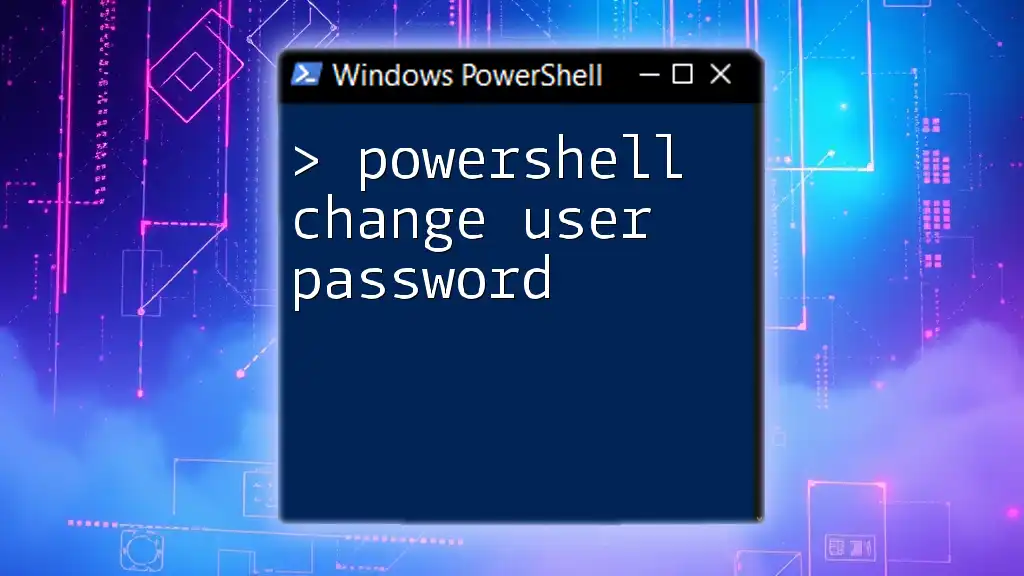
Conclusion
In summary, managing file permissions in PowerShell provides an efficient way to secure your files and folders. With the capabilities of PowerShell at your disposal, you can make precise changes to permissions with just a few commands. By understanding and utilizing the Get-Acl and Set-Acl cmdlets, you can streamline your file management tasks and enhance your overall control over Windows file security. Practice the commands provided, and soon you'll be able to navigate file permissions like a seasoned pro.
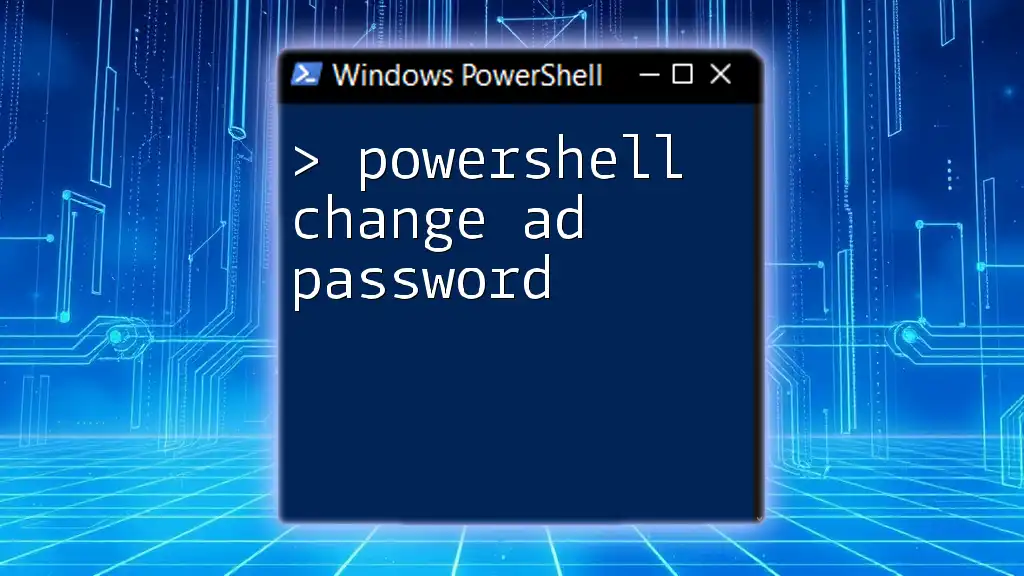
Additional Resources
For further reading and to deepen your understanding of PowerShell, consider checking out the official PowerShell documentation and resources related to Windows file permissions. The more you learn, the more effectively you can harness the power of PowerShell in your workflows.