In PowerShell, handling errors effectively can be achieved using the `try`, `catch`, and `finally` blocks to gracefully manage exceptions during script execution.
try {
# Code that may throw an error
Get-Content 'nonexistentfile.txt'
} catch {
# Handle the error
Write-Host "An error occurred: $_"
} finally {
# Code that will run regardless of success or error
Write-Host "Execution complete."
}
Understanding PowerShell Errors
What are PowerShell Errors?
In PowerShell, errors are issues that occur during the execution of scripts or commands. They can disrupt the flow of your scripts if not appropriately handled. PowerShell errors can be categorized into two main types: terminating errors and non-terminating errors.
- Terminating Errors: These errors stop the execution of the script immediately. A typical example is trying to access a file that does not exist.
- Non-Terminating Errors: These errors allow the script to continue running after the error occurs. An example would be trying to get an item from a collection that is not found; PowerShell will issue a warning and continue running.
Common Sources of Errors in PowerShell
Understanding where errors originate can help in crafting better error handling. Common sources include:
- Syntax Errors: Mistakes in the command structure or format.
- Runtime Errors: Problems that occur while the script is executing, typically due to bad data or configuration.
- Logical Errors: Flaws in the script's logic that produce incorrect results but don't necessarily stop execution.
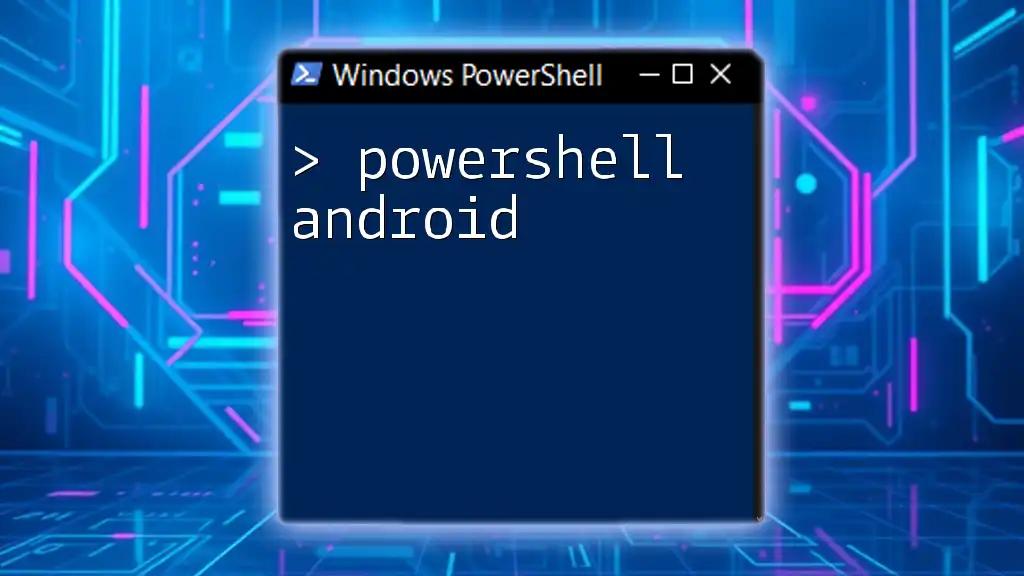
PowerShell Error Handling Techniques
Using `try`, `catch`, and `finally`
One of the most powerful features of PowerShell for error handling is the implementation of `try`, `catch`, and `finally` blocks. These allow you to write scripts that can gracefully recover from errors.
try {
# Code that might throw an error
Get-Item "C:\NonExistentFile.txt"
} catch {
# Code that runs if an error occurs
Write-Host "An error occurred: $_"
} finally {
# Code that runs regardless of the error
Write-Host "Execution completed."
}
In this example:
- The `try` block contains the code that may fail.
- The `catch` block is executed only if an error occurs, where you can handle errors or log them.
- The `finally` block executes regardless of whether an error occurred, making it ideal for cleanup actions like closing files or releasing resources.
Error Variable `$Error`
PowerShell automatically maintains an array of errors, accessible via the `$Error` variable. This variable can store the details of all errors that have occurred in the current session.
Get-Item "C:\NonExistentFile.txt"
if ($Error.Count -gt 0) {
Write-Host "Last error: $($Error[0])"
}
In this snippet, after attempting to get a file that doesn’t exist, we check the `$Error` array to see if there were any errors recorded and print the latest one.
Using Error Action Preference
The `$ErrorActionPreference` variable can change how PowerShell handles errors globally for all commands running in the session. This variable has several settings:
- Continue: The default behavior; non-terminating errors will be shown, but execution continues.
- Stop: Non-terminating errors are treated as terminating errors.
- SilentlyContinue: Errors are ignored, and no messages are shown.
- Inquire: Prompts the user for input when an error occurs.
$ErrorActionPreference = "Stop"
try {
Get-Item "C:\NonExistentFile.txt"
} catch {
Write-Host "Handled an error due to ErrorActionPreference."
}
Setting `$ErrorActionPreference` to "Stop" allows developers to manage errors more effectively and perform specific error handling actions in a `catch` block.
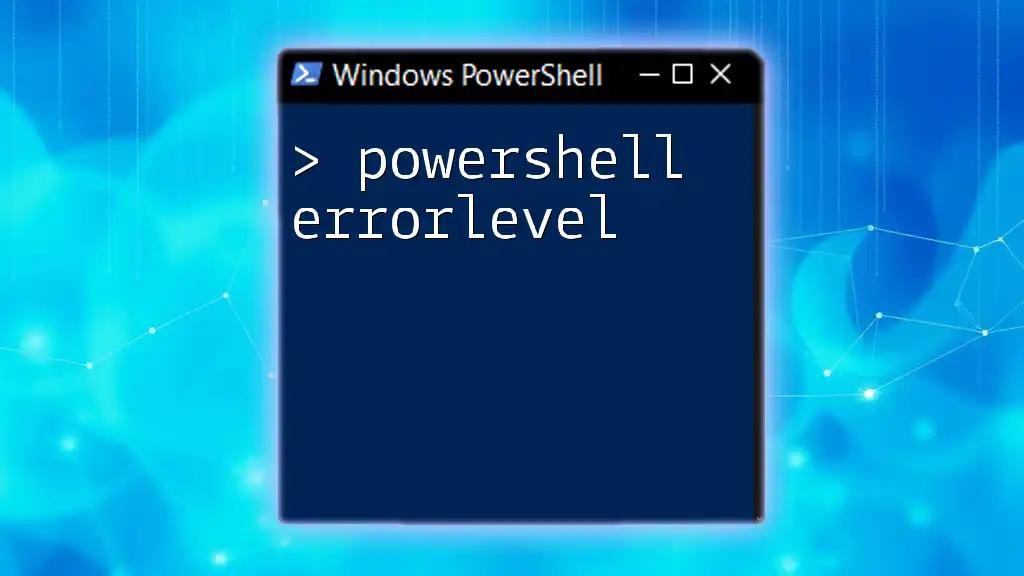
Debugging PowerShell Scripts
Utilizing the `-ErrorAction` Parameter
Many PowerShell cmdlets accept an `-ErrorAction` parameter, allowing you to specify how to handle errors at the command level.
Get-Item "C:\NonExistentFile.txt" -ErrorAction Stop
In this example, the command will treat any non-terminating error as a terminating error, which will then invoke the `catch` block if surrounded by `try`/`catch`.
Enable Debugging with `Set-PSDebug`
Using `Set-PSDebug`, you can enable debugging options to trace the execution of your scripts, making it easier to identify where an error occurs.
Set-PSDebug -Trace 1
This command outputs each line of your script as it runs, providing visibility into the script's execution flow, which can help pinpoint errors as they occur.

Managing and Logging Errors
Creating Custom Error Messages
Providing user-friendly error messages can significantly enhance user experience and troubleshooting. Custom messages clarify issues, making it easier to understand what went wrong.
try {
Get-Item "C:\NonExistentFile.txt"
} catch {
throw "Custom error: File not found at: C:\NonExistentFile.txt"
}
In this case, a more informative error message is thrown, improving clarity regarding the error’s nature.
Logging Errors to a File
Capturing error details in a log file is a best practice for long-running scripts or scheduled tasks. This approach enables debugging without requiring immediate interaction from users.
try {
Get-Item "C:\NonExistentFile.txt"
} catch {
$_ | Out-File "C:\ErrorLog.txt" -Append
}
Using this method, any encountered errors are appended to a specified log file, allowing for review and analysis at a later time.
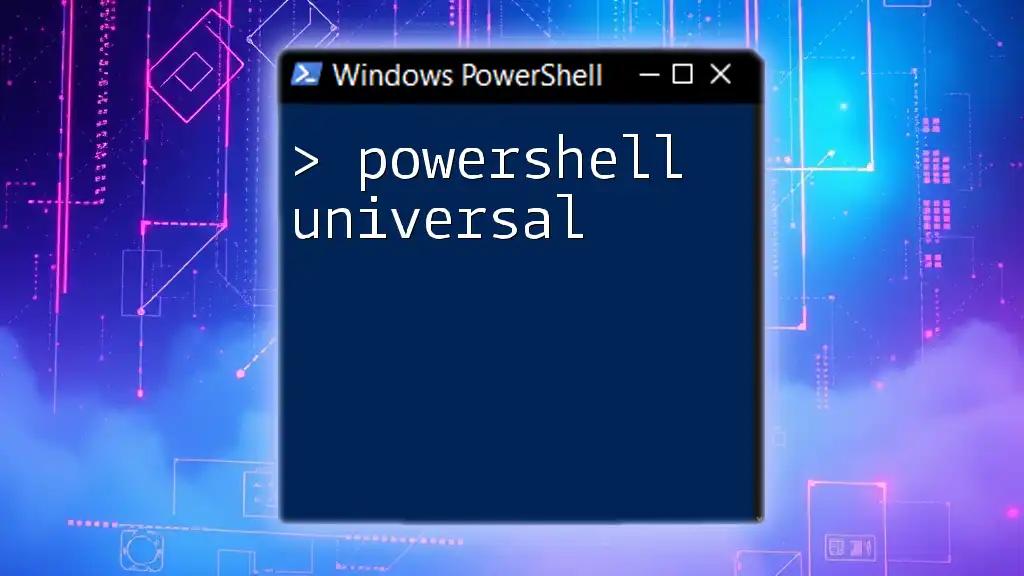
Advanced Error Handling Techniques
Using the `ErrorRecord` Object
The `ErrorRecord` object is generated during an error and contains detailed information about the error, including the source and message. You can access properties of the `ErrorRecord` object to get a detailed context around an error.
try {
Get-Item "C:\NonExistentFile.txt"
} catch [System.IO.FileNotFoundException] {
$errorInfo = $_.Exception
Write-Host "File not found: $($errorInfo.Message)"
}
In this snippet, we catch a specific type of exception and print more informative details about the file that was not found. This can be beneficial for targeted error messages.
Implementing Graceful Recovery
When writing scripts, considering how to recover from errors can greatly enhance the robustness of your code. Implementing strategies such as retries or alternative actions when an error occurs can prevent script failure due to transient issues.
For instance, if an attempt to read a file fails, the script could prompt the user to correct the filename or specify an alternative file path. By designing your scripts with these contingencies in mind, you can make them more resilient.
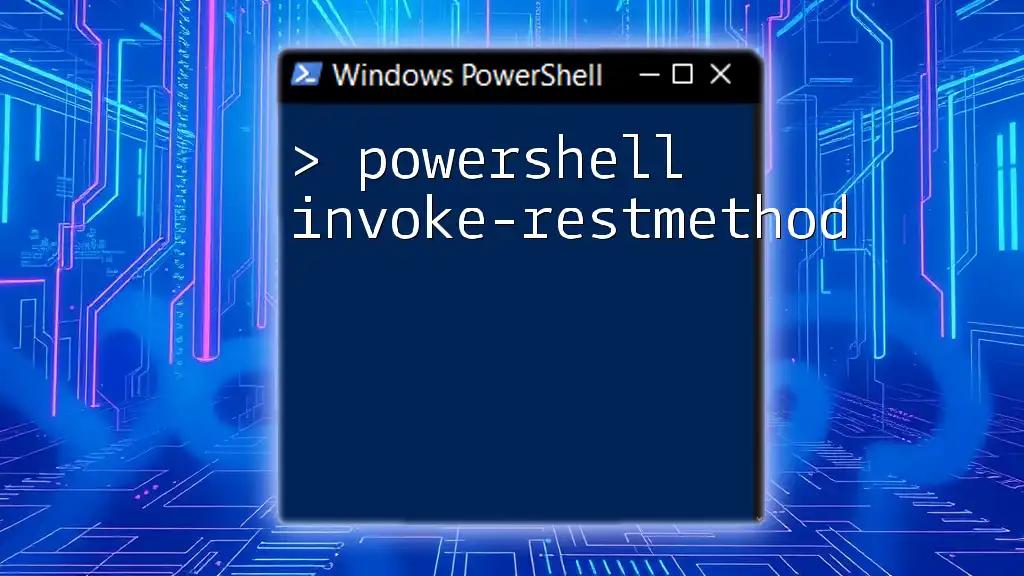
Conclusion
Effective PowerShell error handling is an essential skill for anyone looking to automate tasks or manage systems efficiently. Through the correct implementation of error handling techniques such as `try`, `catch`, and `finally`, using the `$Error` array, and customizing error actions, you can create robust scripts that fail gracefully and provide clear feedback to users. By leveraging debugging options and logging errors, you ensure that you can easily track and resolve issues as they arise. Embrace these techniques and take your PowerShell scripting to the next level!

Additional Resources
For further exploration, consider reviewing the official PowerShell documentation on error handling, engaging in community forums, and utilizing tutorial resources to deepen your understanding and proficiency in managing errors within PowerShell scripts.