In PowerShell, a parameter set allows you to define different groups of parameters for a function or cmdlet, enabling users to specify different combinations of options depending on their needs.
Here's a code snippet demonstrating a parameter set in a function:
function Test-ParameterSet {
[CmdletBinding(DefaultParameterSetName='SetA')]
param (
[Parameter(Mandatory=$true, ParameterSetName='SetA')]
[string]$NameA,
[Parameter(Mandatory=$true, ParameterSetName='SetB')]
[int]$NumberB
)
switch ($PSCmdlet.ParameterSetName) {
'SetA' { Write-Host "Name: $NameA" }
'SetB' { Write-Host "Number: $NumberB" }
}
}
What are Parameter Sets in PowerShell?
PowerShell parameter sets are a fundamental feature that allows you to define groups of parameters for a cmdlet, ensuring that only a specific set can be used in a single invocation. The core idea behind parameter sets is to create clear and intuitive command structures, which help users understand how to interact with the cmdlet without confusion.
When defining a cmdlet, you can specify different parameter sets that group distinct parameters. This ensures that depending on the use case—such as creating a new user or deleting an existing one—only the relevant parameters are available for inputs, thus improving usability.
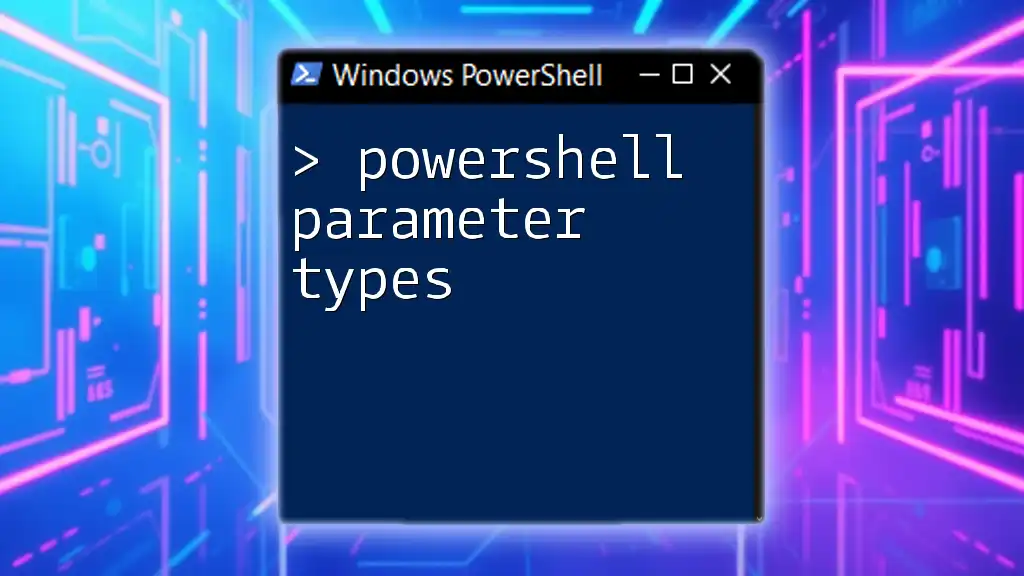
How to Define Parameter Sets
Creating a parameter set is a straightforward process. You use the `Parameter` attribute to define the sets. The structure of a simple cmdlet with two parameter sets might look like this:
[CmdletBinding()]
param (
[Parameter(ParameterSetName='Set1')]
[string]$FirstName,
[Parameter(ParameterSetName='Set2')]
[string]$LastName,
[string]$FullName
)
In this example, there are two distinct sets (`Set1` and `Set2`), each associated with a different parameter. The user can either provide a first name or a last name, but both cannot be supplied simultaneously. The `$FullName` parameter is always available to hold whatever string might be provided.
This structure is instrumental for guiding user input and preventing errors caused by inappropriate parameter combinations.
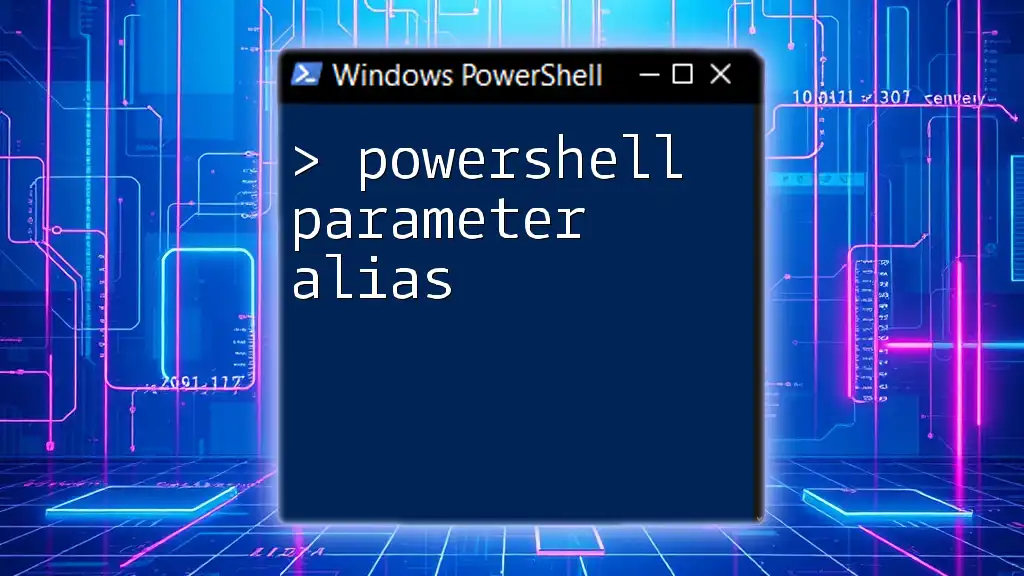
Using Parameter Sets with Cmdlets
Creating Cmdlets with Parameter Sets
The creation of cmdlets with parameter sets allows for improved command functionality. A more complex example can illustrate this:
[CmdletBinding()]
param (
[Parameter(ParameterSetName='Add')]
[string]$Name,
[Parameter(ParameterSetName='Remove')]
[string]$NameToDelete,
[alias('DisplayName')]
[string]$OutputName
)
In this cmdlet, there are two parameter sets: `Add` and `Remove`. Users can either add a name or remove one, ensuring that both actions cannot be invoked simultaneously. Using aliases, such as renaming `$OutputName`, adds further flexibility.
Why Parameter Sets Matter
Parameter sets enhance user experience by clarifying how different parameters interact within the context of the cmdlet. By having them, users can easily understand which parameters are applicable to their use case without wading through extensive documentation. Thus, clear parameter set design can lead to fewer mistakes and increased efficiency.
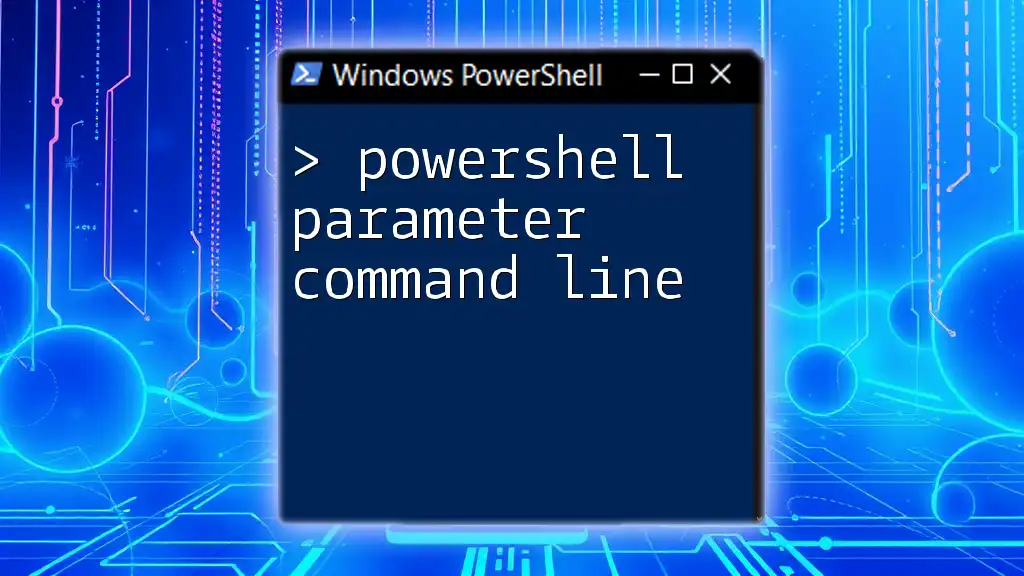
Best Practices for Designing Parameter Sets
When designing parameter sets, consider the following best practices:
-
Clear Naming Conventions: Parameter set names should be intuitive and descriptive, reflecting the functionality they enable. This ensures users can quickly identify which parameters they need to utilize.
-
Minimize Parameter Sets: Avoid excessive parameter sets to keep cmdlet design simple and easier to maintain. Aim for a balance that covers functionality without cluttering the command interface.
-
Maintain Compatibility: Ensure that your parameter sets and cmdlets remain backward-compatible, especially if they become part of a larger framework. This prevents issues when older scripts are executed in newer environments.
-
Edge Case Testing: Test your cmdlets for potential edge cases where parameters interact unexpectedly. This can help prevent runtime errors that could frustrate users.
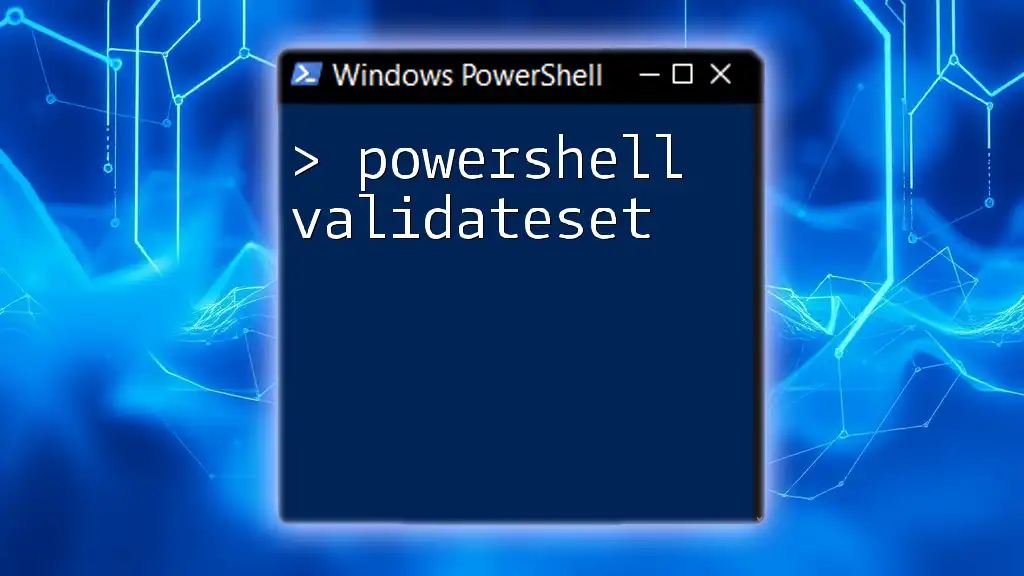
Common Scenarios and Examples
Scenario 1: Using Multiple Parameter Sets in Real World Scripts
Consider a user creation script with parameter sets that conditionally allow input based on the task at hand:
[CmdletBinding()]
param (
[Parameter(ParameterSetName='UserCreation')]
[string]$UserName,
[Parameter(ParameterSetName='UserDeletion')]
[string]$UserId
)
In this case, the cmdlet would require either a user name for creating a new user or a user ID for deleting an existing one. By preventing the user from inputting both parameters, the cmdlet ensures clarity in its function.
Scenario 2: When to Use Parameter Sets
Understanding when to use parameter sets is crucial. For example, in a script where user privileges are being updated, you might have sets defined for `Admin` and `Regular` users. By allowing certain parameters only under specific sets, developers can tailor input directly to real-world usage patterns, minimizing confusion regarding what they can supply.
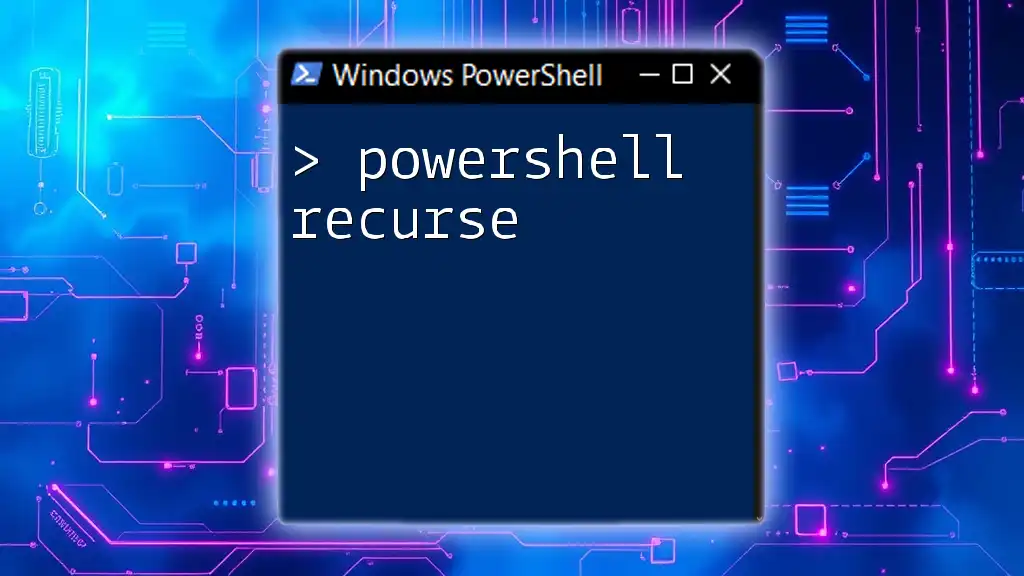
Troubleshooting Parameter Sets
While working with parameter sets, you may encounter common errors. It often helps to add error handling within your scripts to catch these issues early. For instance, if a user tries to invoke incompatible parameters, a clear error message should be thrown.
To debug parameter set problems, check for:
- Incompatible parameters defined within the same set.
- Missing required attributes for parameters.
- Logical errors that arose from how parameters were grouped.
A diagnostic example might include adding verbose output to your cmdlets, allowing users to better understand which parameter set is being invoked.
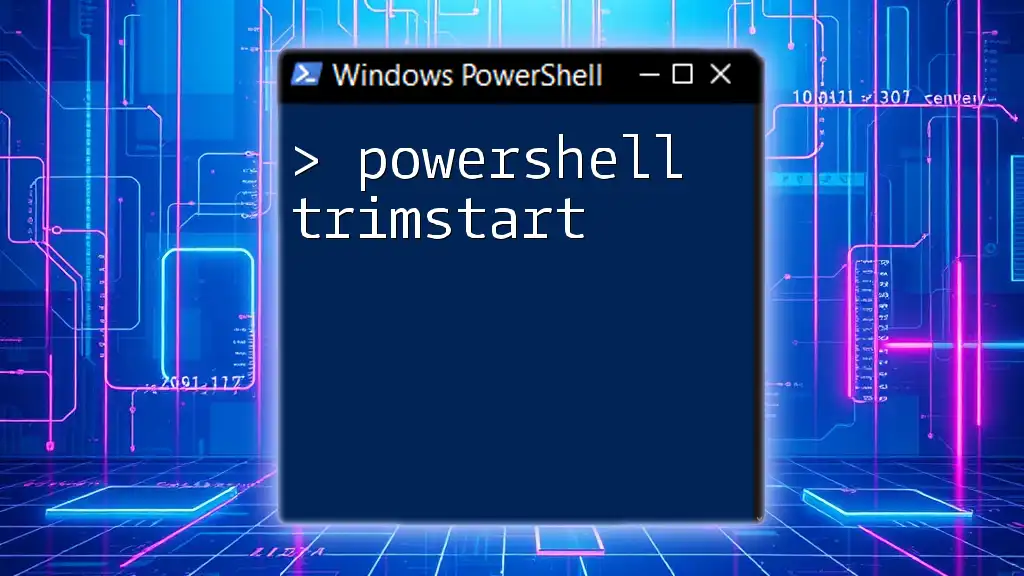
Conclusion
Understanding PowerShell parameter sets is crucial for any PowerShell developer aiming to write effective scripts. By clearly defining sets and using them wisely, you enhance both the usability and clarity of your cmdlets. Experimentation is key: use these concepts in your scripts to witness firsthand how well-structured parameter sets improve user interactions and reduce errors.
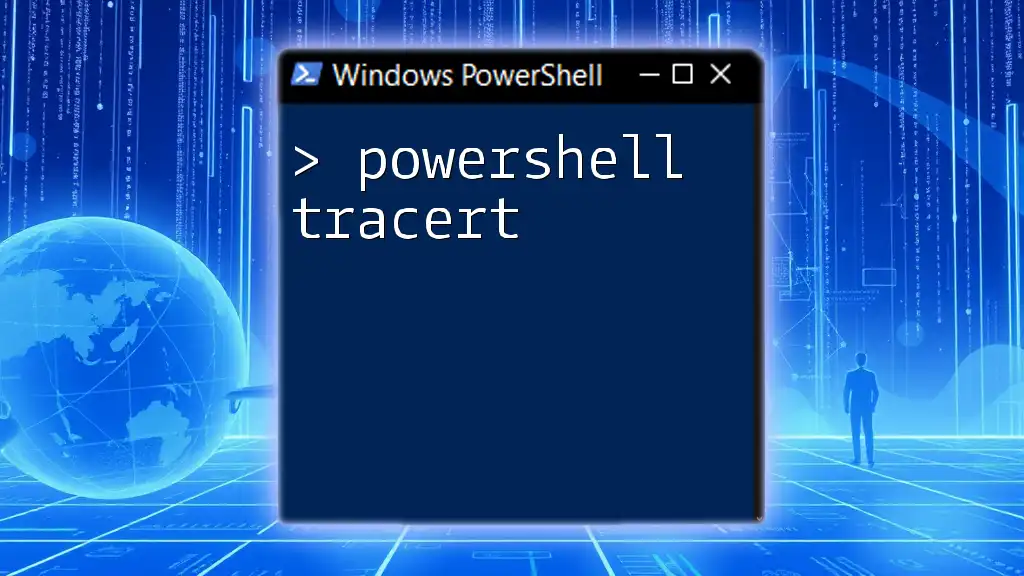
Call to Action
As you dive deeper into PowerShell, consider exploring additional resources to enrich your understanding of scripts and commands. Stay engaged by subscribing for the latest tutorials and guides that can elevate your PowerShell skills to new heights. Your feedback and experiences are also invaluable, so don't hesitate to share your insights with us!