The `ValidateSet` attribute in PowerShell allows you to specify a set of valid values for a parameter, ensuring that the input provided to the command is restricted to pre-defined options.
Here’s a code snippet that demonstrates its use:
function Test-Color {
param (
[ValidateSet("Red", "Green", "Blue")]
[string]$Color
)
Write-Host "You chose the color: $Color"
}
# Example usage
Test-Color -Color Green
What is `ValidateSet`?
`ValidateSet` is a validation attribute used in PowerShell to define a set of accepted string values for a parameter. Its primary function is to limit users to a specific set of values, ensuring that only valid inputs are accepted. This enhances the reliability of scripts by minimizing the chances of errors caused by invalid entries.
Using `ValidateSet` offers several benefits, including:
- Parameter validation at the time of input.
- Enhanced user experience through autocompletion features in PowerShell environments.
- Improved command reliability, reducing the likelihood of runtime errors in scripts.
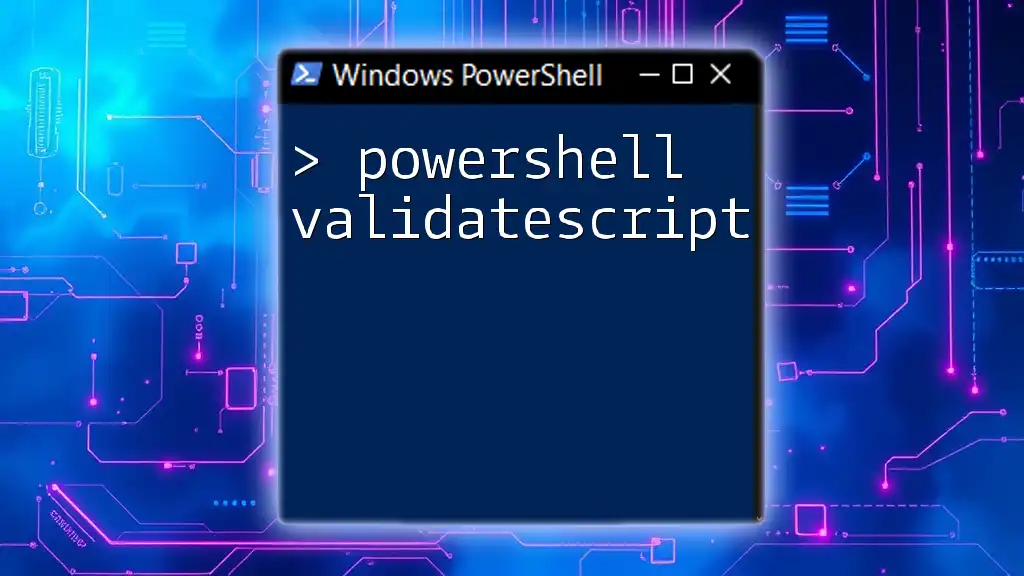
How to Implement `ValidateSet`
Basic Syntax
The basic syntax for using `ValidateSet` in a PowerShell function is straightforward. It involves applying the attribute above the parameter declaration. Here's the basic structure:
[ValidateSet("Option1", "Option2", "Option3")]
param (
[string]$MyParameter
)
Examples of Using `ValidateSet`
Example 1: Basic Implementation
To understand how `ValidateSet` operates, let’s consider a simple function that allows users to select a color:
function Select-Color {
param (
[ValidateSet("Red", "Green", "Blue")]
[string]$Color
)
"You selected $Color"
}
In this example, if a user tries to input a color outside of the specified options (e.g., "Yellow"), PowerShell will return an error, ensuring that only valid inputs are accepted.
Example 2: Complex Parameters
You can also use `ValidateSet` in functions with multiple parameters. Here is an example that allows the selection of both fruit and its size:
function Select-Fruit {
param (
[ValidateSet("Apple", "Banana", "Cherry")]
[string]$Fruit,
[ValidateSet("Small", "Medium", "Large")]
[string]$Size
)
"You selected $Fruit of size $Size"
}
In this case, both `$Fruit` and `$Size` are restricted to predefined values, ensuring the function operates as intended.
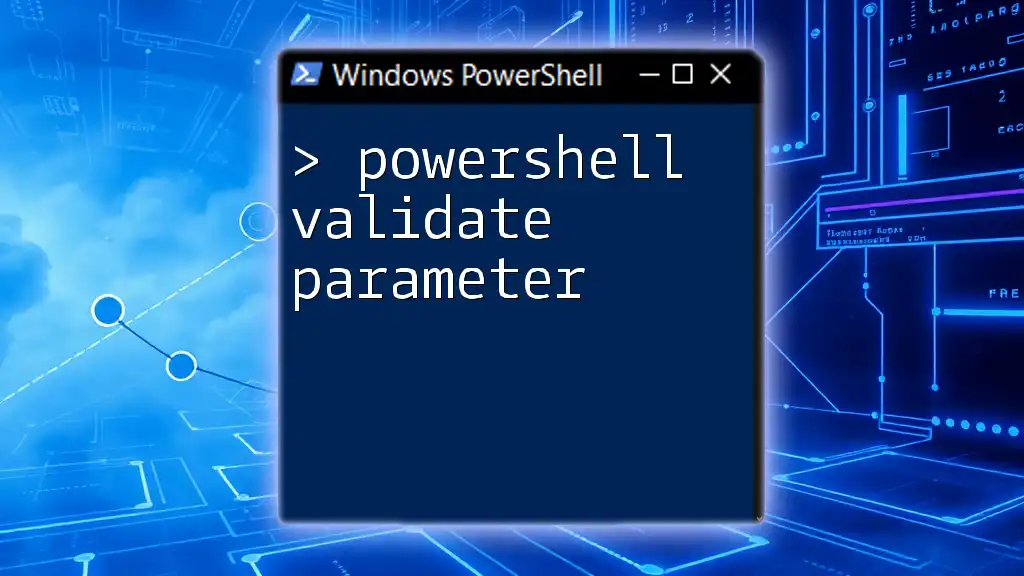
How `ValidateSet` Enhances User Experience
Enforcing Parameter Validation
One significant advantage of using `ValidateSet` is its ability to enforce parameter validation effectively. By limiting the inputs to predefined values, it helps prevent runtime errors and unexpected behavior in your scripts. This is especially crucial in a production environment where reliability is paramount.
Autocompletion in PowerShell ISE and VSCode
When using `ValidateSet`, you also provide autocompletion suggestions in PowerShell Integrated Scripting Environment (ISE) and Visual Studio Code. As you start typing a function that includes parameters defined with `ValidateSet`, the ISE or VSCode will automatically suggest available options. This feature not only enhances user experience but also accelerates the scripting process.
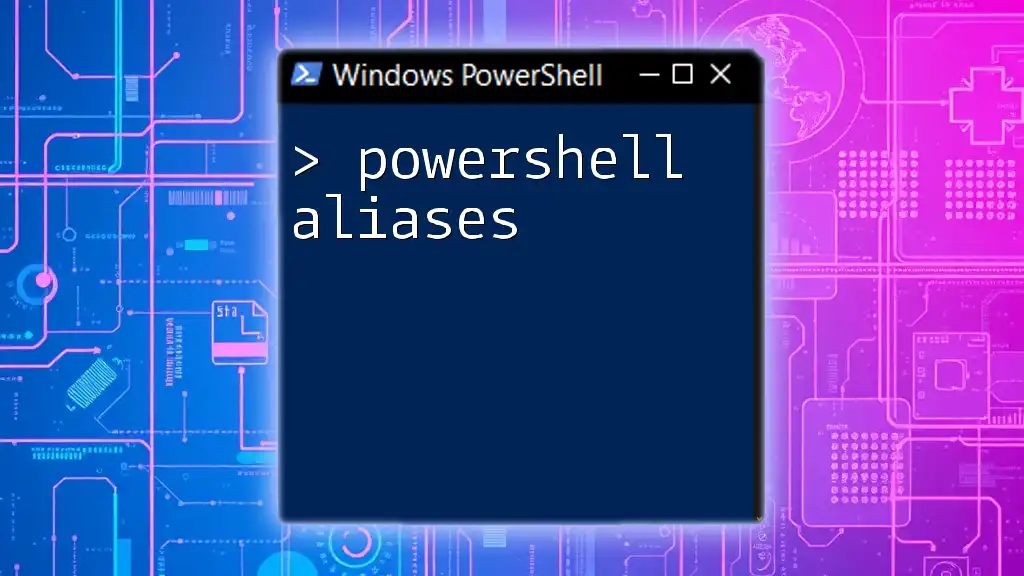
Customizing `ValidateSet` Options
Using Arrays for Dynamic Values
You can customize `ValidateSet` even further by utilizing variables and arrays. This is especially helpful when the valid options may change based on external factors. Here’s how you can create a dynamic set:
$validOptions = @("Option1", "Option2", "Option3")
function Select-Option {
param (
[ValidateSet($validOptions)]
[string]$Option
)
"You selected $Option"
}
In this example, the options for `ValidateSet` come from an array, allowing for greater flexibility in what values are accepted.
Case Sensitivity and `ValidateSet`
It’s important to note that PowerShell parameters are case-insensitive by default. However, in cases where case sensitivity is a requirement, you can design your function accordingly. PowerShell doesn’t currently have a direct way to enforce case-sensitive `ValidateSet`, so it's essential to manage expectations around parameter handling carefully.
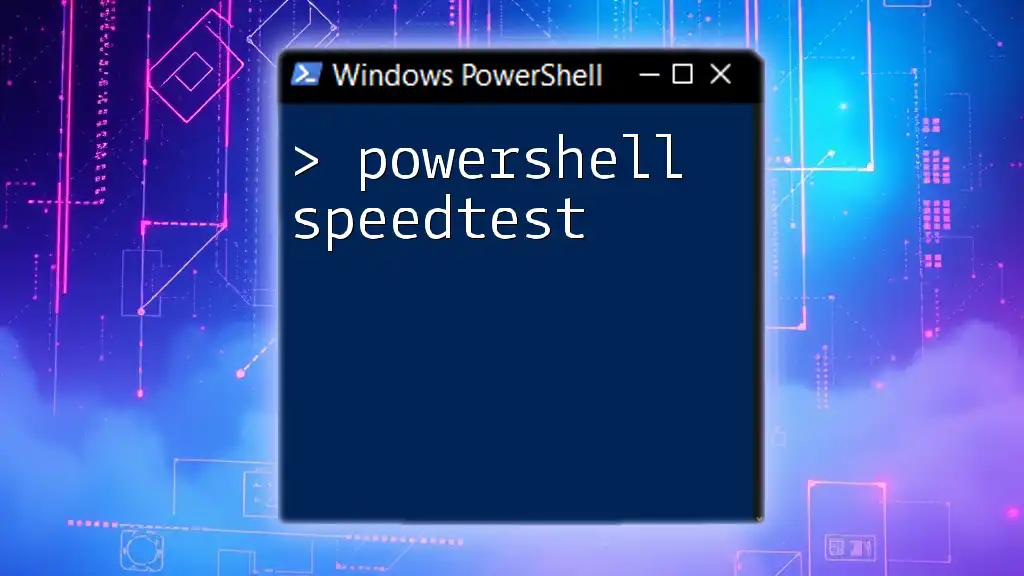
Best Practices for Using `ValidateSet`
When to Use `ValidateSet`
Using `ValidateSet` is particularly useful when you have a fixed set of options that users need to select from. Here are a few scenarios when it is appropriate to implement `ValidateSet`:
- When creating functions that require specific command arguments.
- In scripts intended for a controlled environment where predictable parameters are critical.
- For cmdlets that command user input; defining acceptable values enhances usability.
Avoiding Common Pitfalls
While `ValidateSet` is powerful, it's important to be aware of common pitfalls:
- Don't overuse it: Applying `ValidateSet` to every parameter can make a function unnecessarily rigid. Use it judiciously.
- Ensure clarity: Clearly communicate the valid options to users within help documentation or comments.
- Handle errors gracefully: Prepare for erroneous input by providing useful error messages or guidance.
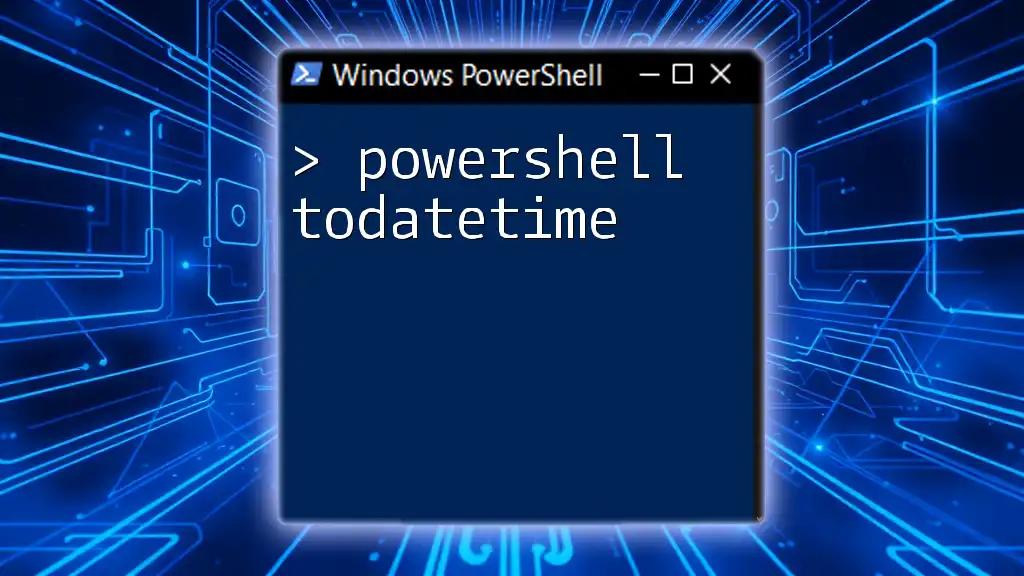
Conclusion
Incorporating `ValidateSet` in your PowerShell scripts enhances usability, enforces reliable inputs, and prevents common errors. By understanding how to implement and customize `ValidateSet`, you can create more effective and user-friendly PowerShell functions. As you explore your PowerShell journey, consider adopting these practices to improve parameter validation in your scripts.
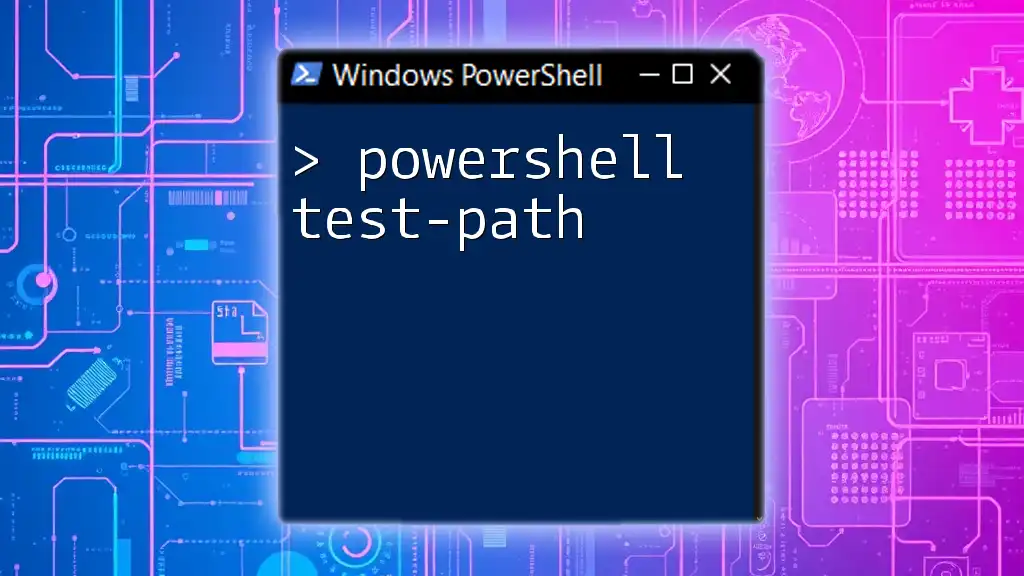
Additional Resources
For further exploration of `ValidateSet` and PowerShell functions, the official PowerShell documentation is an invaluable resource. Look for additional articles that delve into the nuances of parameter validation techniques to broaden your understanding.
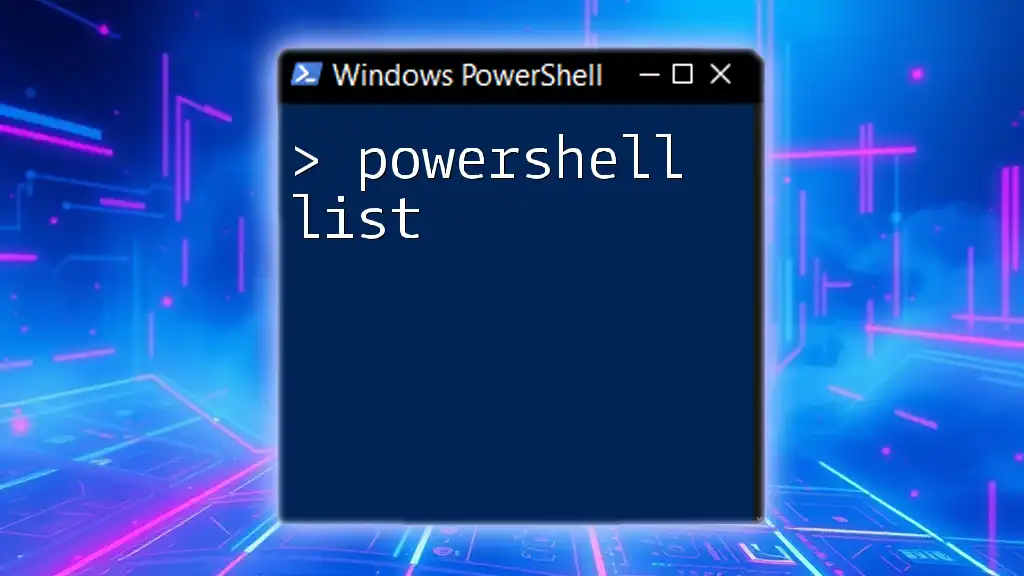
FAQs
Q: Can I use `ValidateSet` with integers?
A: `ValidateSet` is typically used with strings. However, it is possible to use it with strings that represent integer values.
Q: What happens if a user provides an input that's not in the `ValidateSet`?
A: PowerShell will throw an error indicating that the input is not valid, guiding the user to input a correct value from the specified set.
By effectively utilizing `PowerShell ValidateSet`, you can improve your scripts' functionality and user experience, making the PowerShell scripting environment more accessible to all users.