The `Test-Path` cmdlet in PowerShell checks whether a specified path exists, returning a boolean value indicating its presence.
Test-Path "C:\Temp\example.txt"
What is Test-Path?
The `Test-Path` cmdlet in PowerShell is a powerful tool used to determine whether a specified path exists. This path can refer to various resources, including files, directories, registry keys, or even environment variables. Understanding how to use `Test-Path` effectively can enhance your scripting capabilities and help prevent errors when dealing with file and directory operations.
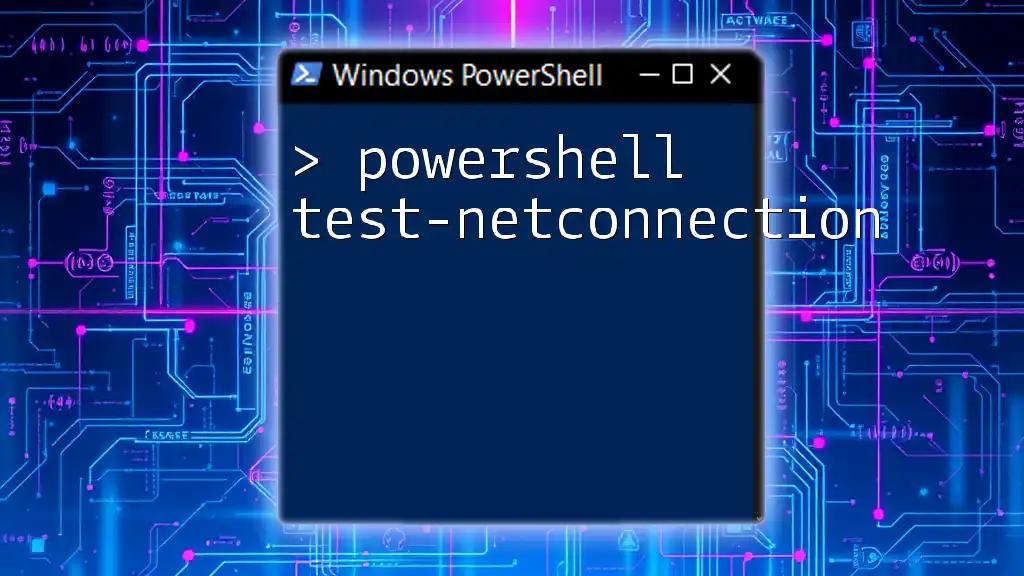
Syntax of Test-Path
Basic Syntax
The basic structure of the `Test-Path` command is straightforward:
Test-Path -Path <path>
This command will evaluate whether the specified `<path>` exists and return a Boolean value—`True` if the path exists and `False` otherwise.
Parameters
While the command syntax above provides a basic usage of `Test-Path`, there are key parameters worth noting:
- -Path: This parameter specifies the file system path you want to check for existence.
- -LiteralPath: When this parameter is used, it treats the path as a literal string, meaning it won’t interpret any wildcard characters. This is essential when the path includes characters, such as `?` or `*`, that could be misinterpreted.
- -IsValid: This parameter is useful for checking if the provided path is valid without actually checking if the path exists. It's particularly beneficial when validating paths inputted by users or scripts.

How Test-Path Works
Behind the Scenes
When you execute the `Test-Path` cmdlet, PowerShell checks the specified path against the file system or registry. The cmdlet quickly traverses the file system hierarchy, returning a Boolean result to indicate the presence of the specified item. Understanding that it returns `True` or `False` allows developers to incorporate this logic into conditional statements, making decision-making in scripts more effective.
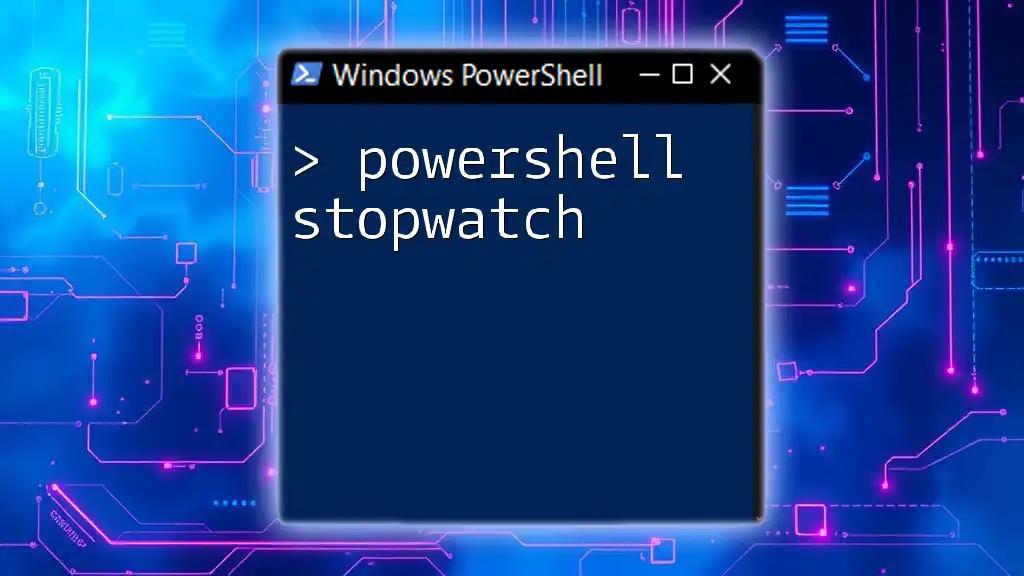
Common Use Cases
Checking File Existence
One of the most common applications of `Test-Path` is verifying whether a specific file exists. For instance, if you need to check for a config file before executing a script, you can use the following code snippet:
if (Test-Path -Path "C:\example\file.txt") {
Write-Host "File exists."
} else {
Write-Host "File does not exist."
}
This snippet evaluates the presence of `file.txt` in the specified path, providing immediate feedback on its existence.
Validating Directories
Similarly, checking for the existence of a directory can be done with ease using `Test-Path`. Here’s an example:
if (Test-Path -Path "C:\example\directory") {
Write-Host "Directory exists."
} else {
Write-Host "Directory does not exist."
}
By verifying directory existence, scripting becomes more robust and less prone to errors related to file paths.
Handling Wildcards
`Test-Path` also supports wildcard characters, allowing for flexible path validation. For example, if you want to check if any `.txt` files exist within a directory, you can run:
if (Test-Path -Path "C:\example\*.txt") {
Write-Host "There are text files in the directory."
}
This capability can save time and streamline the process of managing multiple files.
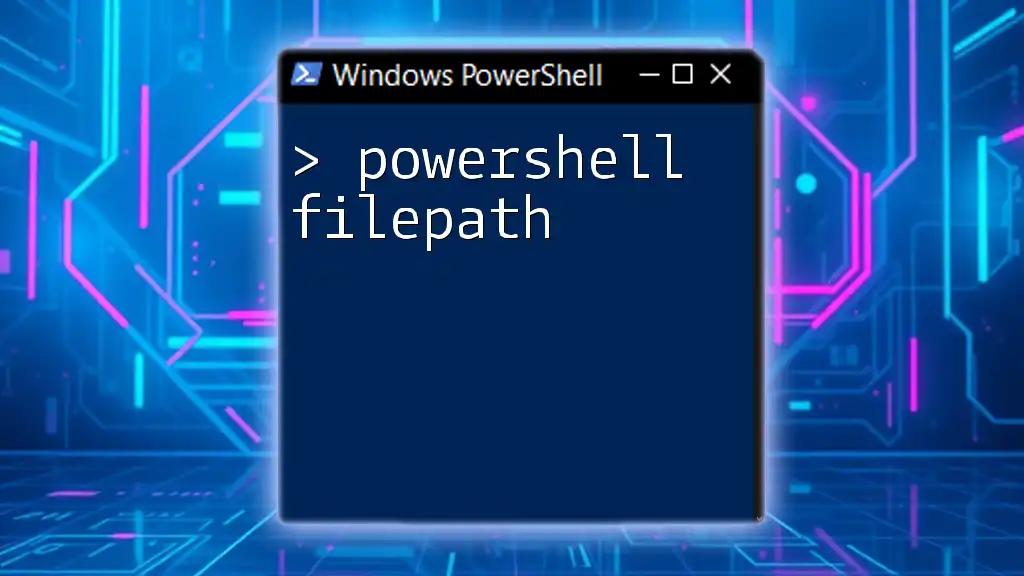
Working with Other Data Types
Checking for Registry Paths
Another important aspect of `Test-Path` is its ability to check paths in the Windows registry. For instance, you can verify if a particular registry key exists with the following snippet:
if (Test-Path -Path "HKCU:\Software\ExampleKey") {
Write-Host "Registry key exists."
}
This approach can be particularly useful for applications that depend on specific settings stored in the registry.
Verifying Environment Variables
You can also use `Test-Path` to validate the presence of environment variables. For example, when checking if an environment variable named `MY_VARIABLE` exists, you can do the following:
if (Test-Path -Path env:MY_VARIABLE) {
Write-Host "Environment variable exists."
}
This allows your script to adapt based on system configurations and user preferences.
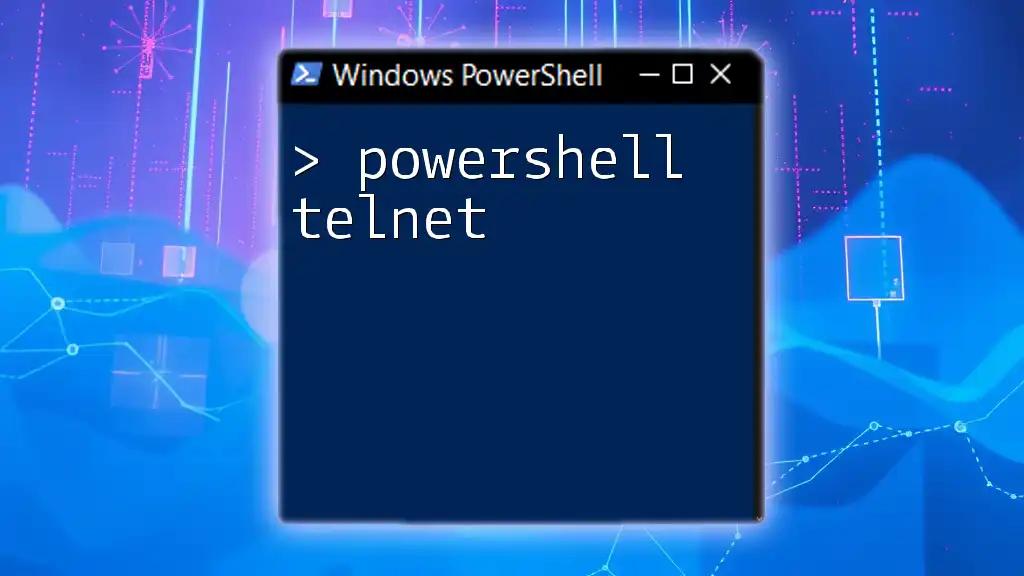
Common Pitfalls and How to Avoid Them
Misusing Paths
One common mistake when using `Test-Path` is providing an incorrect path format. To troubleshoot this issue, always ensure that the path is correctly spelled and follows the syntax conventions of your operating system. Utilizing quotes around paths, especially those with spaces, is imperative to avoid syntax errors.
Case Sensitivity
It's also essential to recognize that some file systems are case-sensitive. For instance, paths in Linux are sensitive while Windows paths are generally case-insensitive. When scripting across platforms, keep this in mind to avoid unexpected failures.
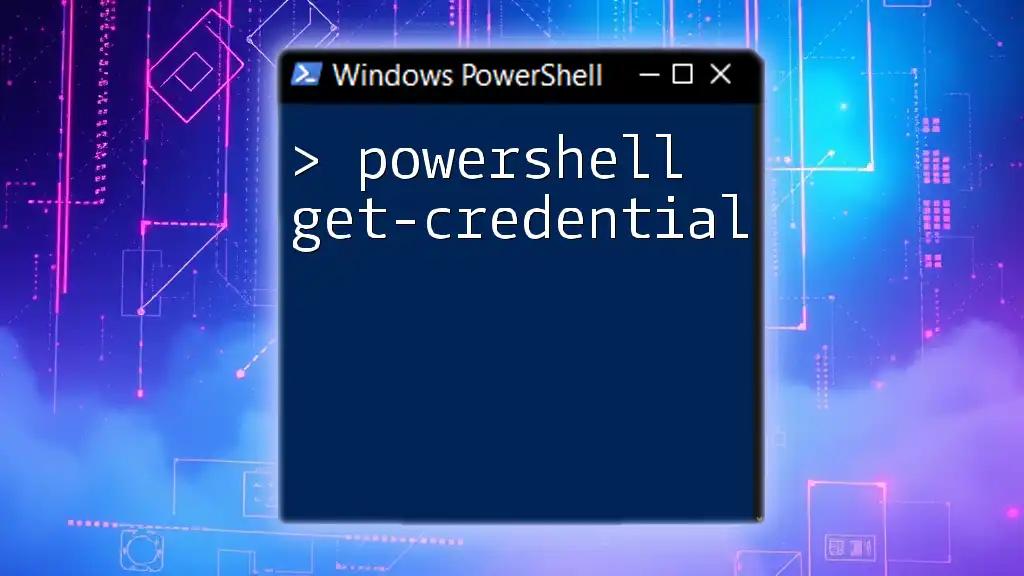
Best Practices for Using Test-Path
Combining with Other Cmdlets
Leveraging `Test-Path` alongside other cmdlets can significantly enhance script efficiency. For example, when iterating through files in a directory, you can check if each file exists before proceeding:
$files = Get-ChildItem "C:\example"
foreach ($file in $files) {
if (Test-Path -Path $file.FullName) {
Write-Host "$($file.Name) exists."
}
}
This approach ensures that your script runs smoothly without encountering errors due to missing files.
Error Handling
Incorporating error handling with `Test-Path` can further secure your scripts. Using try/catch blocks, you can gracefully manage any unexpected issues:
try {
if (Test-Path -Path "C:\example\file.txt") {
# ... execute code
}
} catch {
Write-Host "An error occurred: $_"
}
This technique fosters a more reliable scripting environment by allowing you to respond to errors proactively.
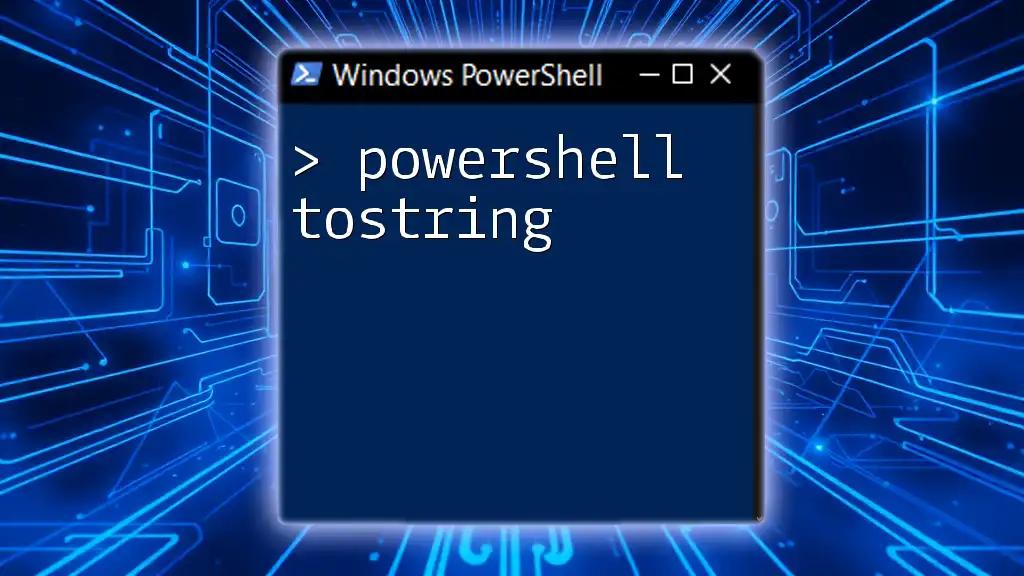
Conclusion
In summary, mastering the `Test-Path` cmdlet is essential for any PowerShell user looking to streamline their file and environment management processes. By understanding its syntax, applications, and best practices, you can significantly enhance your scripting effectiveness. Practice using `Test-Path` in various scenarios to become more comfortable and effective in your PowerShell scripting endeavors.

Additional Resources
For a deeper understanding, consider checking out the official Microsoft documentation on `Test-Path` and explore other PowerShell resources for further learning. Engaging with community forums and PowerShell user groups can also provide valuable insights and tips from fellow scripters.