A PowerShell hashtable is a collection of key-value pairs that allows you to store and retrieve data efficiently in a structured format.
Here's a simple example of how to create and access a hashtable in PowerShell:
$myHashtable = @{'Name'='John'; 'Age'=30; 'City'='New York'}
Write-Host "Name: $($myHashtable['Name']), Age: $($myHashtable['Age']), City: $($myHashtable['City'])"
What is a Hashtable?
A hashtable is a data structure that stores key-value pairs, allowing for efficient retrieval and management of data. Each unique key corresponds to a specific value, enabling fast access. Unlike arrays, which are indexed numerically, hashtables use strings or any type of data as identifiers, making them extremely versatile in PowerShell.
Why Use Hashtables in PowerShell?
Using hashtables in PowerShell provides several advantages:
- Efficiency: Quick access to data by keys compared to searching through arrays.
- Flexibility: Supports diverse data types as keys and values, accommodating various use cases.
- Organization: Enables logical grouping of related data, useful in configuration management or storing metadata.
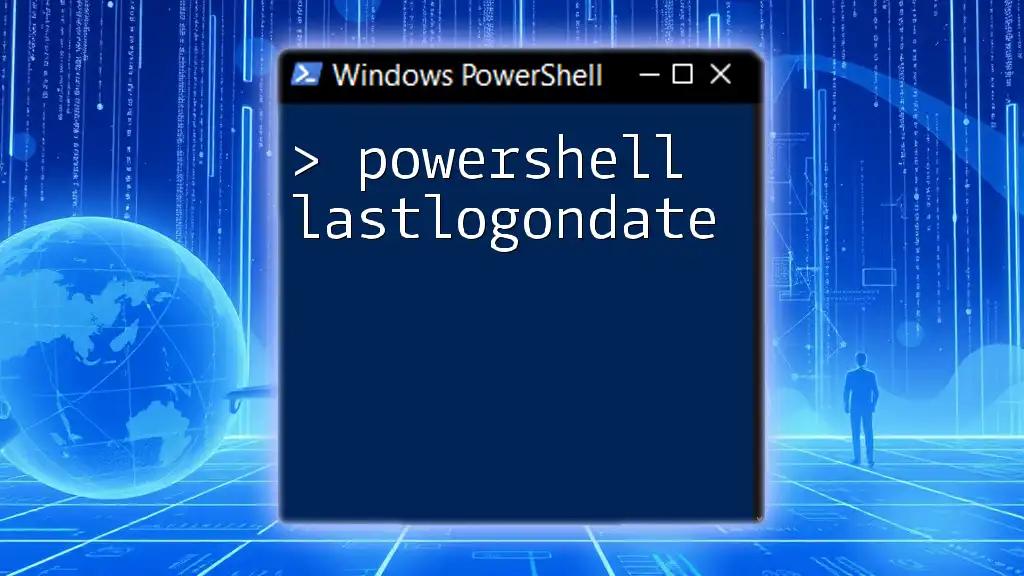
Understanding Hashtables
Key Concepts
At its core, a hashtable consists of keys and values. A key is a unique identifier that allows you to retrieve the associated value. Hashtables manage data in a manner similar to dictionaries in other programming languages, ensuring each key only exists once.
Creating a Hashtable
To create a hashtable in PowerShell, you utilize the @{} syntax, which creates an empty hashtable.
$myHashtable = @{}
Adding Key-Value Pairs
You can easily add key-value pairs to your hashtable using the following syntax:
$myHashtable["Key1"] = "Value1"
$myHashtable["Key2"] = "Value2"
In this example, Key1 maps to Value1, and Key2 maps to Value2. This allows you to store related pieces of data efficiently.
Accessing Data
Retrieving Values from a Hashtable
Accessing values is straightforward. You reference the key, and PowerShell returns the associated value. For example:
$value = $myHashtable["Key1"]
If Key1 exists, $value will contain Value1.
Checking for Existence of Keys
Before accessing a value, you might want to check if the key exists in the hashtable to avoid potential errors:
if ($myHashtable.ContainsKey("Key1")) {
"Key1 exists!"
}
This ensures the integrity of your script and prevents runtime errors related to missing keys.
Modifying Hashtables
Updating Values
To update an existing value in a hashtable, simply reassign the value to the key:
$myHashtable["Key1"] = "NewValue"
The value associated with Key1 is now NewValue.
Removing Key-Value Pairs
If you need to remove a key-value pair, the Remove method comes in handy:
$myHashtable.Remove("Key1")
This line deletes Key1 and its corresponding value from the hashtable.
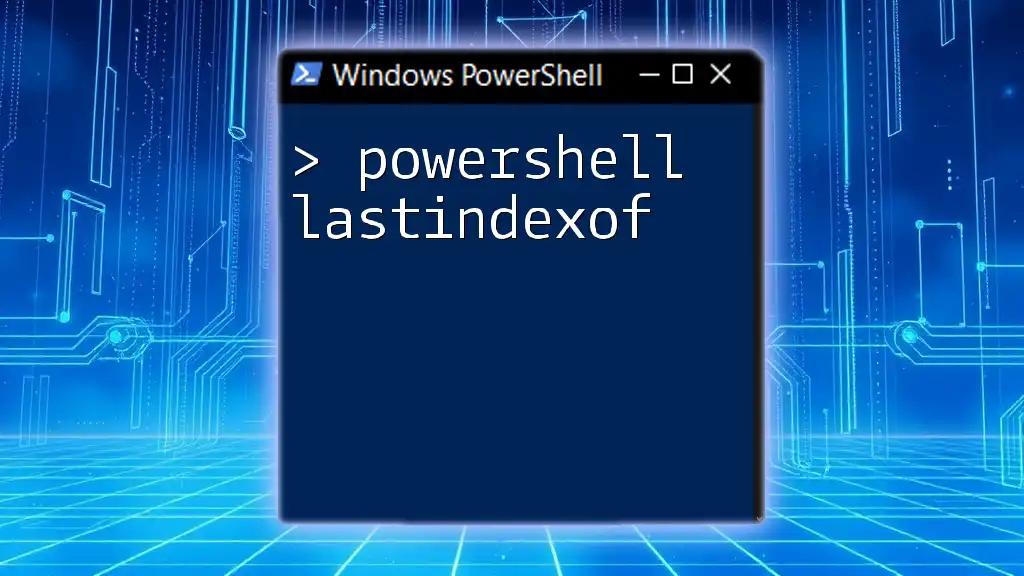
Advanced Hashtable Usage
Nested Hashtables
You can create complex data structures using nested hashtables. This enables you to group related information even further.
$nestedHashtable = @{
"OuterKey" = @{
"InnerKey1" = "InnerValue1"
"InnerKey2" = "InnerValue2"
}
}
In this example, OuterKey contains another hashtable, giving you multi-layered data organization.
Hashtable and PSObject
Integrating hashtables with PowerShell objects (PSObjects) allows for more sophisticated data management. You can construct an object from a hashtable like this:
$person = New-Object PSObject -Property $myHashtable
This approach is helpful when you want to utilize hashtables to represent object properties dynamically.
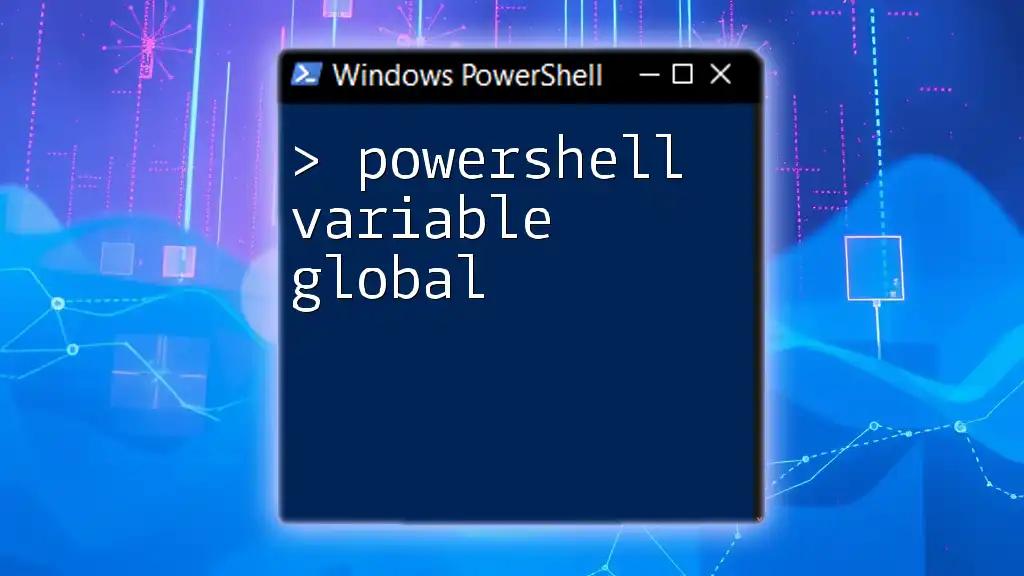
Common Use Cases for Hashtables
Configuration Management
Hashtables are ideal for storing application configurations. You can easily manage settings for an application in one place:
$appConfig = @{
"AppName" = "MyApplication"
"Version" = "1.0"
"DebugMode" = $true
}
Here, appConfig holds important information to be accessed throughout your script.
Data Manipulation
You can utilize hashtables to aggregate and manipulate data from various sources. For instance, if you are pulling user information from different systems, you can collect and organize it using a hashtable.
Script Parameters
Hashtables can also serve as efficient parameters in functions. This allows for a flexible way to pass multiple arguments:
function MyFunction {
param (
[hashtable]$InputHashtable
)
# Do something with InputHashtable
}
By using hashtables as function parameters, you enable dynamic input handling, which is particularly useful in larger scripts.
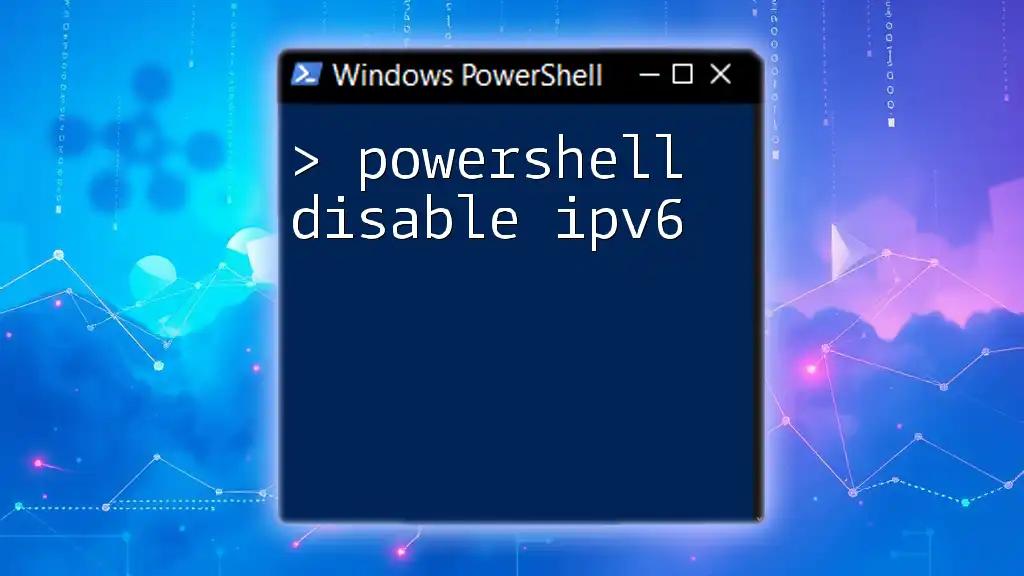
Best Practices
When to Use Hashtables
Choosing between arrays and hashtables largely depends on how you intend to access your data. If you need quick lookups based on unique identifiers, hashtables are your best option. For sequential data retrieval, arrays will suffice.
Performance Considerations
While hashtables offer speedy retrieval and organization capabilities, they do come with overhead. For very large datasets, consider memory usage, and evaluate whether other data structures may serve your purpose better.

Conclusion
In the realm of PowerShell scripting, understanding the concept of hashtables and their practical applications can significantly enhance your scripting efficiency and abilities. They provide the needed flexibility and organization for various tasks, from configuration management to data handling. Embrace the power of hashtables, experiment with them, and take your PowerShell skills to the next level!
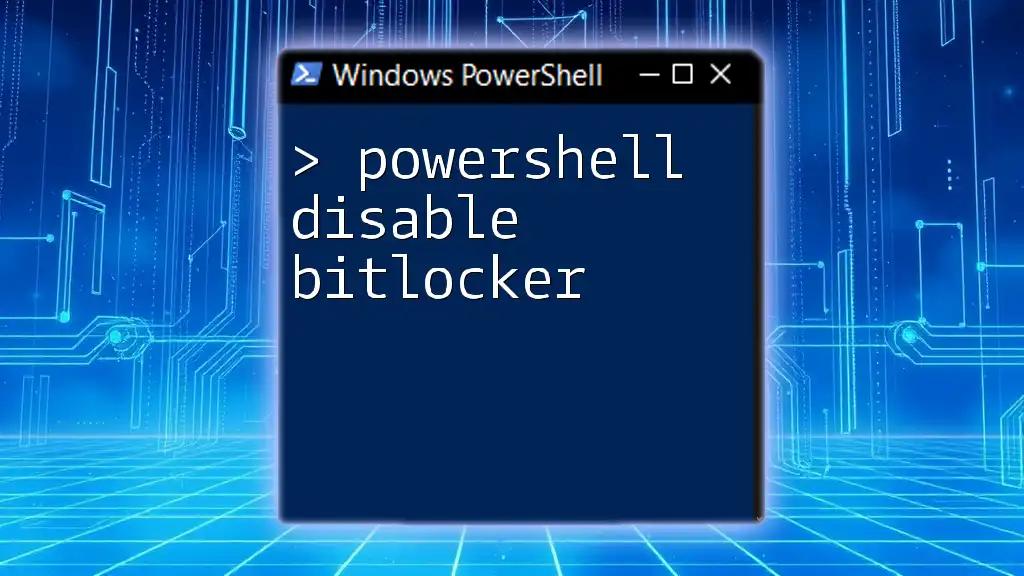
Additional Resources
- Official PowerShell Documentation for deeper insights into hashtables and other advanced features.
- Explore community forums and tutorials for diverse perspectives and use cases.
- Consider recommended books for comprehensive learning about PowerShell scripting techniques.