In PowerShell, the `Get-ChildItem` command, commonly known as `ls`, is used to list the contents of a directory or folder. Here's a simple code snippet to illustrate this:
Get-ChildItem
Understanding Lists in PowerShell
What are Lists?
In PowerShell, a list is a collection of items that can be managed together. Lists are particularly useful for storing multiple values or objects in a single variable, allowing you to easily manipulate, iterate, and manage large sets of data efficiently. They provide a level of functionality above basic arrays by allowing for dynamic resizing and various manipulation methods.
Types of Lists in PowerShell
PowerShell supports several types of lists, each with its own benefits:
-
Typed Lists vs. Untyped Lists: Typed lists enforce a specific data type for their elements, while untyped lists can hold any type of item. Utilizing typed lists can help prevent errors and improve script reliability.
-
ArrayList: The ArrayList class provides a variable-sized array implementation that allows you to add and remove items dynamically. This type is particularly useful when the number of items in your list is not known in advance.
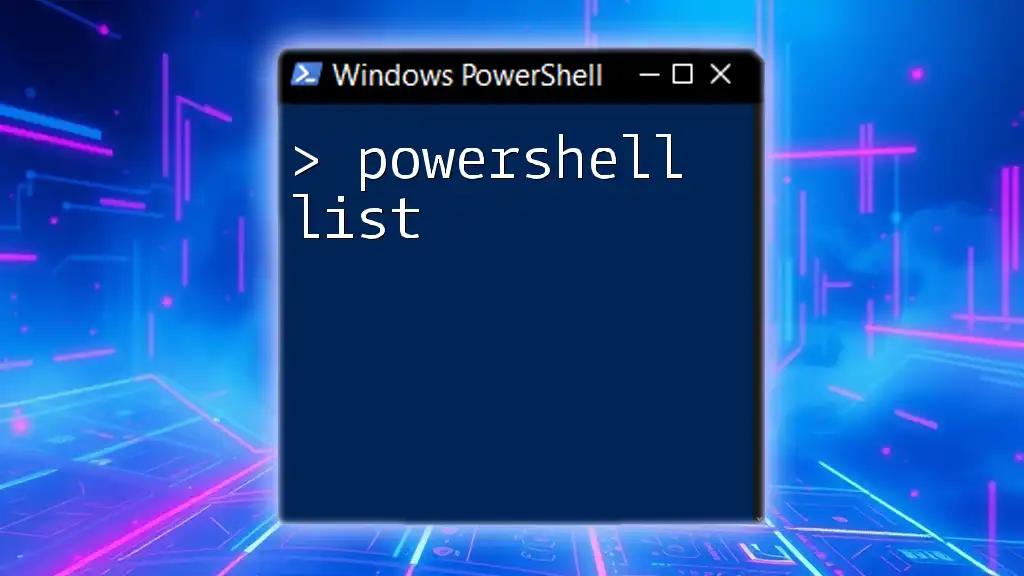
Creating Lists in PowerShell
Initializing a List
To create a new, empty list in PowerShell, you can use the `ArrayList` class. Here’s how to create it:
$myList = New-Object System.Collections.ArrayList
This line initializes `$myList` as an empty list that you can expand on as needed.
Adding Items to a List
To add items to your list, utilize the `Add()` method. This appends items to the end of the list:
$myList.Add("Item1")
$myList.Add("Item2")
Using the `Add()` method is straightforward and allows for quick population of your list with different elements.
Inserting Items at Specific Positions
If you need to add an item at a specific index rather than at the end, you can use the `Insert()` method. Here’s an example:
$myList.Insert(1, "InsertedItem")
In this example, "InsertedItem" will be added at index 1, pushing the existing item at that position (if any) to the next index.
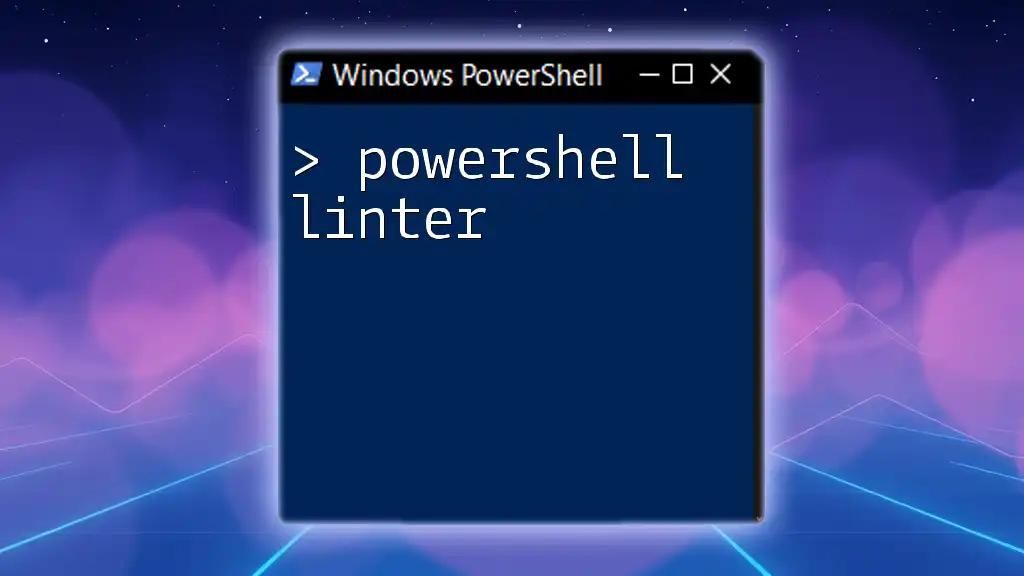
Accessing and Modifying List Items
Indexing and Retrieving Items
PowerShell lists allow direct access via indexing. For instance, you can retrieve an item like so:
$myItem = $myList[0]
This retrieves the first item in the list, demonstrating the concept of zero-based indexing where the first element is always at index 0.
Modifying Items in a List
To change the value of an existing item, simply assign a new value to the desired index:
$myList[0] = "ModifiedItem"
This method is efficient, allowing you to update values directly. It’s important to note that this operation does not change the size of the list; it only alters the content at the specified index.
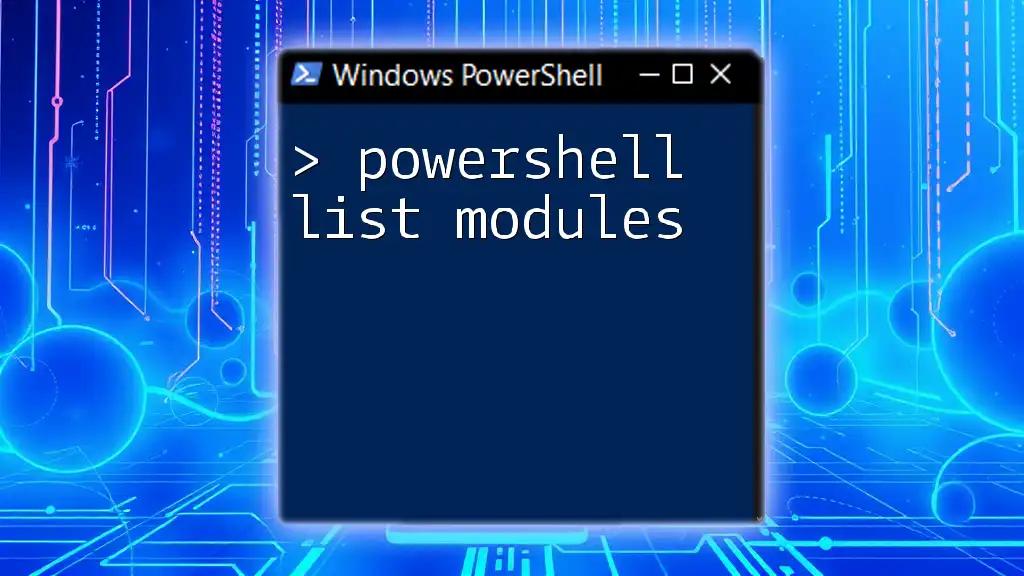
Removing Items from Lists
Removing by Value and Index
PowerShell lets you remove items both by their value and by their position in the list. To remove an item by value, use:
$myList.Remove("Item1")
Alternatively, if you know the index, you can remove an item like so:
$myList.RemoveAt(0)
Understanding the difference between these two methods can aid in effectively managing lists, especially in scripts that manipulate data dynamically.
Clearing a List
At times, you may want to empty the entire list without deleting the variable itself. This can be done using the `Clear()` method:
$myList.Clear()
Utilizing `Clear()` is particularly beneficial when you intend to reuse the same list structure throughout your script without needing to initialize a new variable.
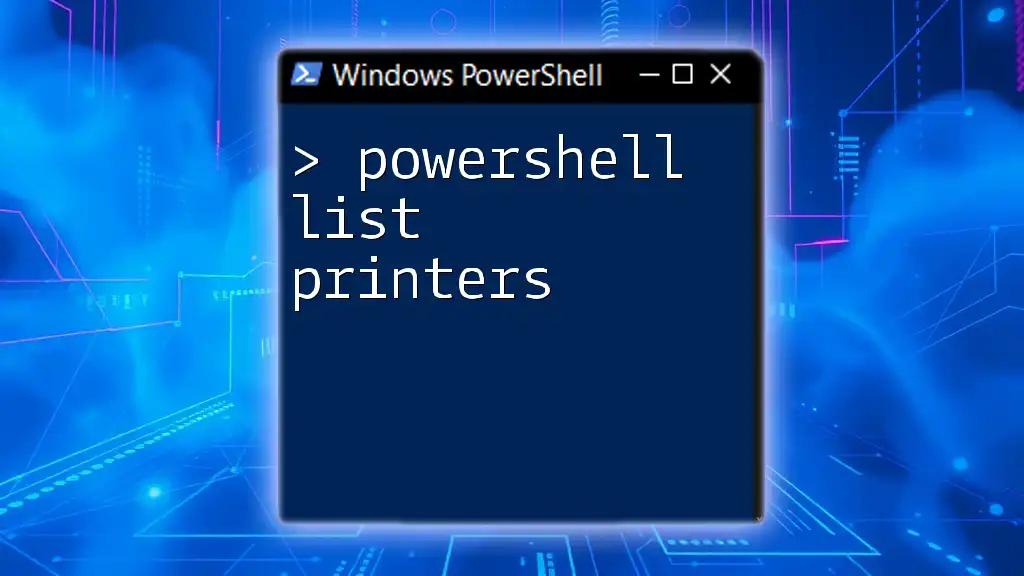
Useful List Methods
Common Methods and Their Uses
PowerShell lists come with several built-in methods that enhance their functionality:
-
Contains: This method checks if a specific item exists in the list:
$myList.Contains("Item2")
This returns `True` or `False`, making it easy to conditionally execute code based on the presence of an item.
-
Count: To determine the number of items currently in the list, use:
$itemCount = $myList.Count
This method is particularly useful in loop control structures or when you need to assess whether the list is empty before proceeding with operations.
Looping Through a List
Iterating over a list is simple using a `foreach` loop. Here’s how it looks:
foreach ($item in $myList) {
Write-Host $item
}
This snippet prints each item in the list to the console, providing an efficient way to process all elements.
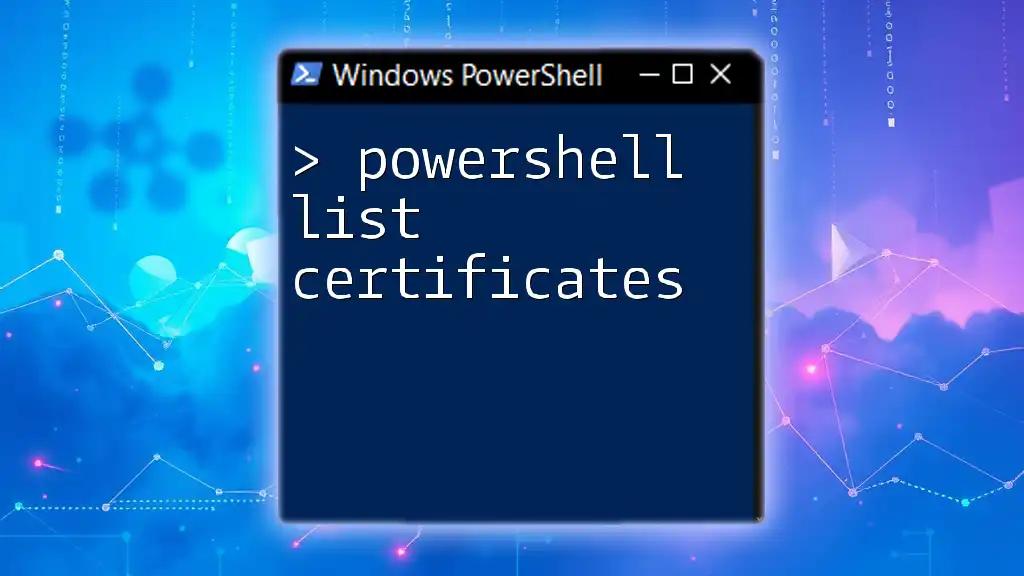
Best Practices for Using Lists
When to Use Lists vs. Arrays
Choosing between lists and arrays depends largely on your needs. Lists offer dynamic resizing, meaning you can add or remove items without needing to create a new collection. Arrays, while faster and more lightweight, have a fixed size and require you to know the quantity of items in advance.
Performance Considerations
While using lists is more flexible, keep in mind that they can have a performance overhead compared to arrays, particularly when dealing with large sets of data. For maximum efficiency, opt for the smallest structure that meets your requirements and utilize lists when you need dynamic resizing or complex data manipulation.
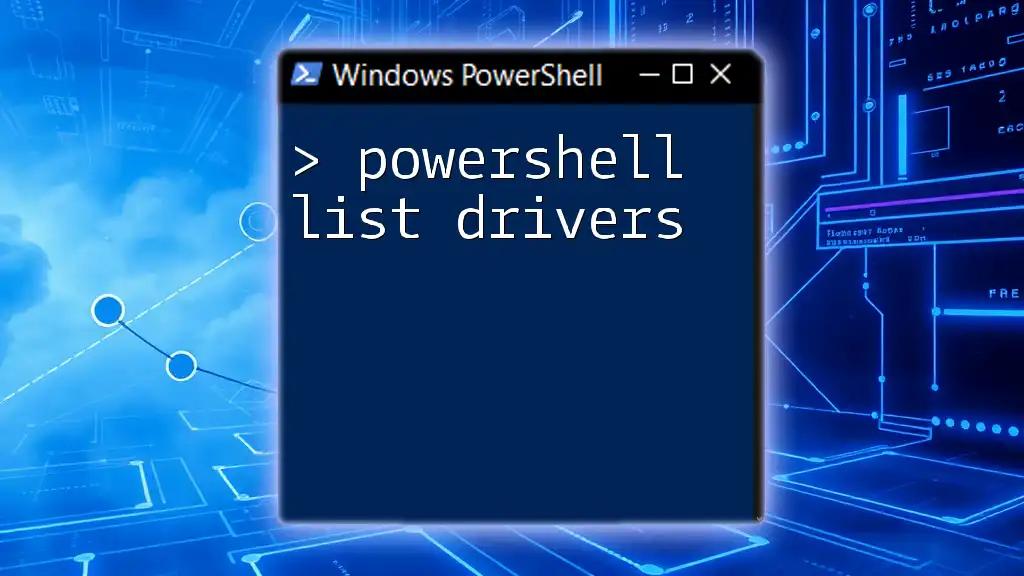
Real-World Applications of Lists in PowerShell
Automating Tasks
Lists are invaluable when automating tasks in PowerShell. You can gather lists of servers, users, or resources you need to manage and loop through them to apply configurations, collect data, or generate reports. This efficiency transforms tedious manual tasks into streamlined processes.
Managing Data Collections
Using lists to hold collections of related data simplifies operations. For example, if you’re collecting input from users or external data sources, you can store each record in a list to systematically process and query it later.
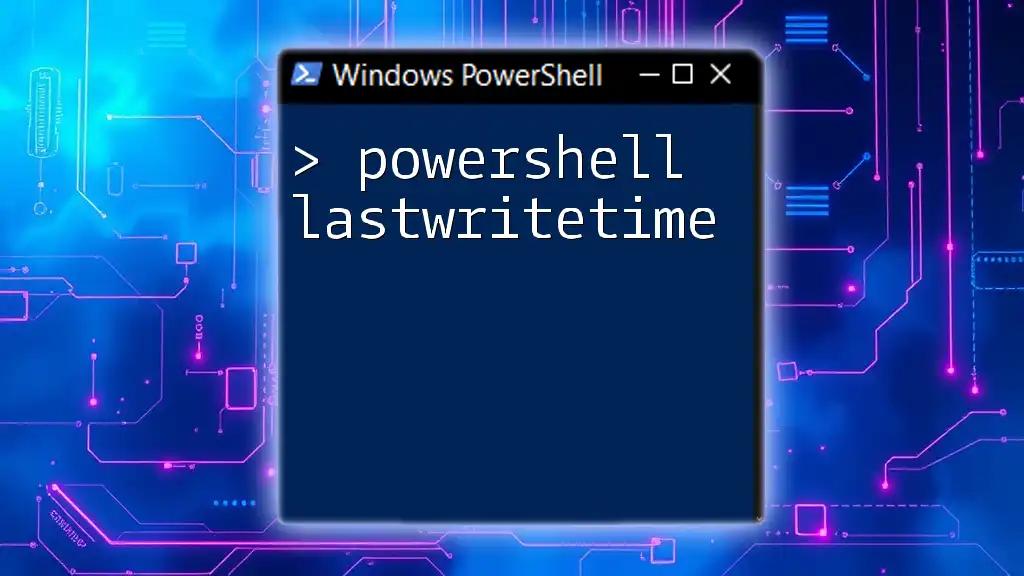
Conclusion
In summary, mastering PowerShell lists is essential for anyone looking to leverage the full power of PowerShell scripting. By learning how to create, manipulate, and iterate through lists, you can significantly enhance your scripting efficiency and effectiveness. Explore the capabilities of lists in your scripts and watch your productivity soar!
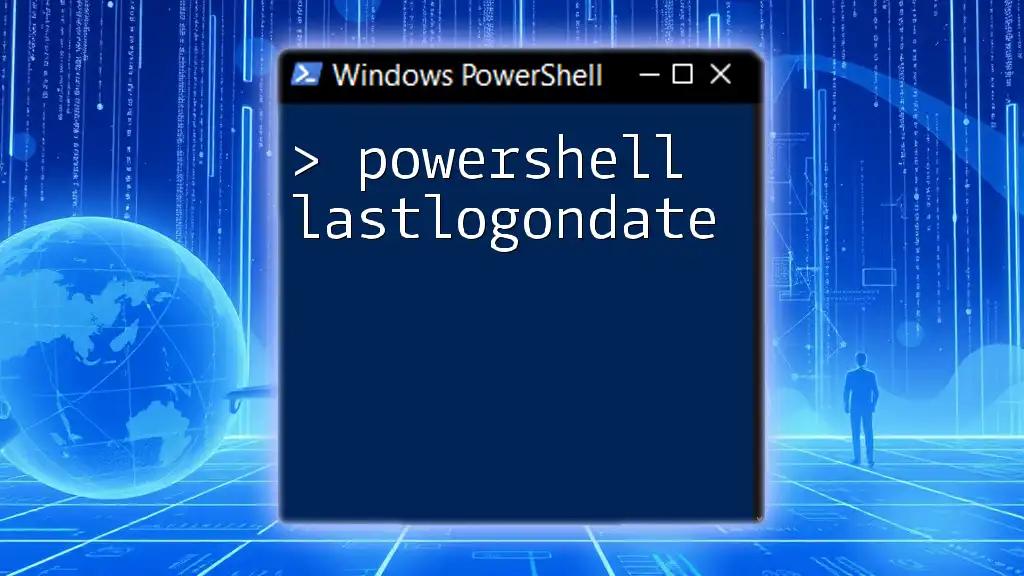
Additional Resources
For further exploration of lists in PowerShell and for best practices in scripting, consider diving into the official PowerShell documentation and joining community forums where experts gather to share their insights and tips.